Welcome to this exciting in-depth tutorial on Python variable conditions. Dive into an intriguing world of programming language, where you can spark creativity and enhance problem-solving skills. We’ll explore the very essence of these variable conditions, make it easy to understand, and unravel how they can be used in different scenarios.
Table of contents
What are Python Variable Conditions?
Python variable conditions, often referred to as conditional statements, are commands that perform different computations or actions depending on whether a programmer-specified boolean condition evaluates to true or false. These conditions become the guiding force, determining the pathway the program should follow based on criteria set by the coder.
The purpose of Python variable conditions is to control the flow of execution of the program. They are used to make choices in code and to create interactive programs that can respond differently to different inputs, leading to dynamic and adaptable code.
Why should I learn Python Variable Conditions?
Python variable conditions form the groundwork for logical programming. Mastering these conditions will equip you with a vital toolset enabling you to:
- Design dynamic and responsive code
- Improve code efficiency and execution time
- Create complex algorithms for real-world problem-solving
Furthermore, Python being a popular programming language worldwide, having a firm grasp of its variable conditions will provide a robust foundation to advance your coding ability. In the next sections, we will delve deeper into coding with variable conditions to plug into your programming journey and reinforce your learning process.
Basic Structure of Python Variable Conditions
Before we jump into examples, let’s first examine the basic structure of a python variable condition.
if condition: action elif condition: action else: action
The above code is a basic framework for Python variable conditions. Python evaluates the conditions in order from top to bottom. If the first condition (after the ‘if’) is true, Python performs its corresponding action and skips the rest. If it’s false, Python checks the next condition (after ‘elif’), and so on. If none of the given conditions are true, Python performs the action under the ‘else’ clause.
Understanding Python Variable Conditions Through Examples
Let’s illustrate this with some practical examples:
Example 1: Basic if Statement
x = 10 if x > 5: print("X is greater than 5")
Here, Python checks whether ‘x’ is greater than 5; as it is, it prints “X is greater than 5”.
Example 2: if-else Statement
x = 3 if x > 5: print("X is greater than 5") else: print("X is not greater than 5")
In this case, ‘x’ isn’t greater than 5, so Python executes the code after ‘else’, printing “X is not greater than 5”.
Example 3: if-elif-else Statement
x = 5 if x > 10: print("X is greater than 10") elif x < 10: print("X is less than 10") else: print("X is equal to 10")
Here, Python first checks whether ‘x’ is more than 10; it isn’t, so Python checks the ‘elif’ condition. As ‘x’ is less than 10, it prints “X is less than 10”.
Example 4: Multiple Conditions
x = 3 y = 7 if x > 2 and y > 5: print("Both conditions are true")
In this example, Python checks if ‘x’ is greater than 2 and ‘y’ is more than 5. Since both conditions are true, it prints “Both conditions are true”.
These examples should provide a basic understanding of Python variable conditions. It’s crucial to practice these examples and create your own to solidify your understanding. Remember, understanding the flow and interaction of conditions is key to mastering Python!
More Python Variable Conditions Examples
With the basics grasped, let’s test our skills with more complex examples and elaborate on some additional concepts.
Example 5: Nested if Statements
x = 10 if x > 0: print("X is positive") if x % 2 == 0: print("X is even") else: print("X is odd")
In this example, Python checks if ‘x’ is positive. If it is, Python tells us so, and then checks whether ‘x’ is also even. Because ‘x’ is 10 – a positive, even number – Python prints both “X is positive” and “X is even”.
Example 6: Using Conditions with Lists
fruits = ["apple", "banana", "cherry"] if "banana" in fruits: print("Yes, banana is in the fruits list")
Here, Python checks if “banana” is within the ‘fruits’ list. Since it is, Python prints “Yes, banana is in the fruits list”.
Example 7: The ‘not’ Operator
x = 5 if not x > 10: print("X is not greater than 10")
In this case, Python uses the ‘not’ operator to negate the statement. Python checks if ‘x’ is not more than 10. Because ‘x’ is indeed 5 – less than 10 – Python prints “X is not greater than 10”.
Example 8: The Pass Statement
x = 7 if x > 5: pass
In certain situations, we may want an ‘if’ structure that doesn’t do anything when the condition is true. Here, Python checks if ‘x’ is more than 5. Since it is, Python uses the ‘pass’ statement, which means do nothing – it’s a placeholder command.
Example 9: Using Conditional Expressions (Ternary Operator)
x = 10 print("X is even") if x % 2 == 0 else print("X is odd")
Considered a more compact form of the ‘if-else’ statement, the ternary operator evaluates a condition in a single line, executing the first command if true, the second if false. Here, Python prints “X is even”.
Practice these examples and let your creativity fly by building your own scenarios. It’s the best way to boost your Python prowess!
Where To Go Next: Continue Your Python Journey
You’ve come a great distance on your programming journey by understanding Python variable conditions, but the road doesn’t end here! With Python being such a vast and versatile language there’s always more to learn, more to explore. So how do you keep learning?
At Zenva, we recommend continuing your journey with our Python Mini-Degree. This comprehensive collection of courses takes you deeper into Python programming, covering various important topics such as coding basics, algorithms, object-oriented programming, game development, and app development.
This unique learning path is designed for both beginners and experienced programmers with courses accommodating different skill levels. The project-based nature of our courses helps you to learn by doing, building your own games, algorithms, and applications. This practical approach helps you turn theoretical knowledge into skill sets that can be used in the real world.
To deepen your understanding of Python and its applications, our Python Mini-Degree also covers well-known libraries and frameworks like Kivy, Tkinter, PyGame, and PySimpleGUI.
With the flexibility to access the courses anytime, anywhere, and the opportunity to reinforce your learning through coding challenges and quizzes, our Python Mini-Degree is an excellent resource to keep you moving forward on your coding journey. Our instructors at Zenva Academy are experienced programmers and certified by Unity Technologies and CompTIA, ensuring a high-quality learning experience for you.
If you’re excited to extend your Python skills even further, do have a look at the broader selection in our Python courses. Each course gives you the chance to take the next step on the path to becoming a Python pro, laying a strong groundwork for your future programming ventures.
Conclusion
In this tutorial, we’ve delved into the fascinating universe of Python variable conditions. These conditions form the heart of logical programming, powering dynamic, efficient, and responsive code. With its clear syntax and powerful capabilities, Python is widely used throughout numerous fields, from web development and game design to artificial intelligence and machine learning. Undoubtedly, mastering Python will open doors to a plethora of exciting opportunities.
Whether you’re a beginner testing the waters or an experienced coder looking to expand your skill set, our Python Mini-Degree offers comprehensive, project-based learning designed to turn you into an adept Python programmer. So don’t hesitate! The journey of coding is one filled with creative problem-solving, and the reward of seeing your ideas come to life is unparallel. With Zenva, prepare to evolve as a confident and skilled Python programmer capable of creating impactful projects. Let’s continue our amazing journey into the world of programming together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
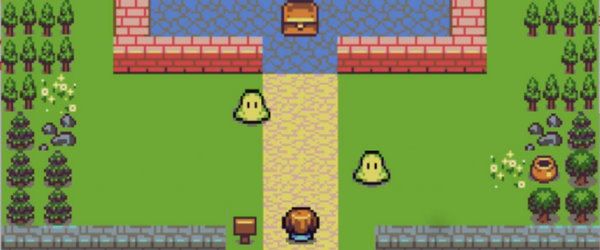
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.