Python is a powerful and versatile programming language that’s used in a wide range of applications. From game creation to automating tasks, understanding Python can open the door to an array of new opportunities. One key aspect of Python that beginners and experienced coders alike need to master is memory management, which will be our focus today. The ability to manage memory efficiently can have a significant impact on the performance of your Python code. This tutorial is designed to make this complex topic accessible and engaging.
Table of contents
What is Python Memory Management?
Python memory management handles how memory is assigned and de-allocated. Every time we create a Python object, like a list or a string, space is allocated in the computer’s memory to store it. On the flip side, when objects are no longer in use, Python’s memory manager steps in to clear these objects from memory.
Why Should I Learn About Python Memory Management?
So, why is learning about Python’s memory management such a big deal?
- Firstly, understanding Python’s memory management can dramatically improve your code’s efficiency. It helps you write code that utilizes memory more effectively, reducing lags and improving overall performance.
- Secondly, it makes you a better programmer overall. Handling memory efficiently is a skill applicable across different programming languages and platforms.
- Finally, it helps you debug your code. If your program is running slow or crashing, it’s often due to memory-related issues. Understanding how Python uses memory can help you pinpoint what’s causing the problem.
Now that we’ve covered what Python memory management is and why you should learn about it, let’s dive in and look at how Python actually manages its memory.
Python Memory Management Basics
Python’s memory management can be divided into three main parts: Heap memory, Stack memory, and Python’s built-in garbage collector.
Heap Memory
Heap memory is where Python stores objects. Every time you create a Python object, that object is stored in heap memory. Here’s an example:
string = "Hello, Zenva learners!"
In the above example, a Python string object is created and stored in heap memory.
Stack Memory
Stack memory is where Python keeps track of what’s happening in your code. Each function call in your program creates a new stack frame in stack memory. This stack frame is a sort of ‘memory box’ that keeps track of the function’s local variables, what the function’s doing, and where it should return to once it’s done.
def greet(): message = "Hello, Zenva learners!" print(message) greet()
In this example, when the greet() function is called, Python creates a new stack frame in stack memory. This stack frame keeps track of the message variable and what the greet() function is doing.
Garbage Collector
Python automatically clears objects from memory once they’re no longer needed, using its built-in garbage collector. Consider this example:
message = "Hello, Zenva learners!" message = "How's your learning journey?"
In this snippet, when we assign a new value to the variable message, the original string object (“Hello, Zenva learners!”) is no longer accessible. The garbage collector then steps in and clears this original string object from memory.
Controlling the Garbage Collector
In Python, the gc module provides the ability to manually control the garbage collector. Here’s a quick example:
import gc gc.disable() # Garbage collector is now disabled message = "Hello, Zenva learners!" message = "How's your learning journey?" gc.collect() # Manually clears garbage gc.enable() # Garbage collector is now enabled again
In this example, we first disable the garbage collector. We then manually clear garbage using the gc.collect() function. Finally, we re-enable the garbage collector.
Managing Memory with Variables
One straightforward way of managing memory in Python is by using variables. When a variable is no longer needed, you can assign the `None` value to it, which allows the garbage collector to clear the object from memory.
message = "Hello, Zenva learners!" message = None
In this example, by setting ‘message’ to `None`, the garbage collector will then have the ability to clear the original string object (“Hello, Zenva learners!”) from memory.
Using `del` Keyword
Python also provides a `del` keyword that you can use to delete a variable, which also allows the garbage collector to clear the object from memory.
message = "Hello, Zenva learners!" del message
In the example above, when ‘del’ is used, Python deletes the reference from the ‘message’ variable to the string object, leaving the object unreachable and eligible for garbage collection.
Understanding Reference Counting
Underpinning Python’s automatic memory management is the concept of reference counting. Each object has a count of the number of references to it. This count is increased when a new reference is made, and decreased when one is removed. When the count reaches zero, the object can be garbage collected.
import sys message = "Hello, Zenva learners!" print(sys.getrefcount(message)) # Outputs: 2 another_message = message print(sys.getrefcount(message)) # Outputs: 3 del another_message print(sys.getrefcount(message)) # Outputs: 2
In this example, `sys.getrefcount()` is used to get the current reference count for the ‘message’. After the ‘another_message’ variable is deleted, the reference count for ‘message’ goes down by one.
Use of `gc` Module
The `gc` module provides functions that you can manipulate to control Python’s garbage collection.
import gc # Returns the number of objects it has collected and deallocated collected = gc.collect() print(f"Garbage collector: collected {collected} objects.")
In the example above, `gc.collect()` is used which returns the number of objects that have been collected and deallocated by the garbage collector.
These examples illustrate Python’s automatic memory management, and how you can interact with it to optimize your programs. Managing memory well can greatly improve your code’s efficiency and make your Python journey much smoother.
Where To Go Next
By now, you should have a solid understanding of Python’s memory management system and how to interact with it. But this is just the beginning of your Python programming journey. To further expand your skills and unlock versatile programming opportunities, consider taking a deeper dive into Python with us at Zenva.
Our Python Mini-Degree is a comprehensive program that thoroughly covers a variety of Python programming topics. By working through this curriculum, you’ll gain an in-depth understanding of Python, including coding basics, algorithms, object-oriented programming, game development, and app development. With an engaging and easy-to-understand approach to instruction, our modules cater to both beginners and experienced coders alike.
This Mini-Degree didn’t earn its name lightly. Across its courses, you get the chance to try your hand at developing your own games, apps, and real-world projects, providing a hands-on way to apply your newly-acquired knowledge. Not to mention, every project you create can make a shining addition to your professional portfolio.
But it doesn’t stop there. We offer a broader collection for you to explore at your pace in our Python Courses. It’s our hearty invitation to you to continue this learning journey with us, and we look forward to guiding you through your Python adventure, from beginner to professional!
Conclusion
Python’s memory management system is an essential pillar of an effective Python developer’s toolkit. Understanding it will not only elevate your Python skills but also provide you with a deeper understanding of programming’s behind-the-scene mechanisms.
The next step towards Python mastery is at your fingertips. Utilize our Python Mini-Degree as your stepping stone towards building powerful, efficient Python code. Set your pace, dive into the diverse course modules, and develop a Python skill set that gives you an edge in today’s coding world. Rome wasn’t built in a day, and neither was any Python developer – but with Zenva, you’re one step closer each day. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
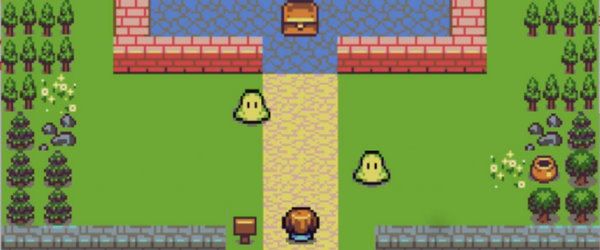
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.