We all know the power of Python as a coding language. But, have you ever needed to interact with a JSON file using this versatile language? In this tutorial, we’ll provide a comprehensive overview on working with JSON files in Python, with plenty of examples along the way.
By the end of this journey, you will understand the mechanics of Python JSON files and how to manipulate data in a fun and engaging way, applicable even in the world of game mechanics.
Table of contents
What is Python JSON?
Python provides a built-in package called `json` to handle JSON files. JSON stands for JavaScript Object Notation and is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate.
It’s a popular data format with diverse uses in data handling. Its application ranges from web development to configuration files, data storage and much more.
Python JSON: Why learn it?
Why should you bother learning about Python JSON files? Here is the exciting part. Imagine creating a game where the player’s data need to be saved for later use. With Python JSON, you can easily implement a mechanism to save game states, player scores, and configuration settings, enhancing the player experience and making your game more professional.
Furthermore, a strong understanding of Python JSON files will open up more possibilities in manipulating and handling data in your coding journey. It’s an essential skill in the toolbox for any Python coder. So, let’s dive in and learn more about Python and JSON.
Python JSON: Handling and Manipulation Basics
1. Importing the json Module
First, to work with JSON in Python, we need to import the built-in `json` module.
import json
2. JSON String to Python Object conversion
Next, let’s look at how to convert a JSON string into a Python object using the `json.loads()` method.
import json json_string = '{"name": "Zenva Student", "courses": ["Python: Basics", "Game Creation: Advanced"]}' python_obj = json.loads(json_string) print(python_obj)
The output would be a Python dictionary:
{'name': 'Zenva Student', 'courses': ['Python: Basics', 'Game Creation: Advanced']}
3. Python Object to JSON String conversion
Likewise, you can convert a Python object into a JSON string using the `json.dumps()` method.
import json python_obj = {"name": "Zenva User", "score": 5, "completed_courses": ["Python Fundamentals", "HTML and CSS"]} json_string = json.dumps(python_obj) print(json_string)
Your output would be a JSON string:
'{"name": "Zenva User", "score": 5, "completed_courses": ["Python Fundamentals", "HTML and CSS"]}'
4. Python Object to JSON File conversion
You can write a Python object into a JSON file using the `json.dump()` function.
import json data = { "player1": { "name": "Zenva", "game": "python learning", "level": 2 } } with open('data.json', 'w') as outfile: json.dump(data, outfile)
This will create a new JSON file, namely ‘data.json’, and writes the JSON string into the file.
Python JSON: Reading a JSON file and JSON into Python Object conversion
1. Reading a JSON File
Using Python, you can read a JSON file and convert it into a Python object with the `json.load()` method.
import json with open('data.json') as json_file: data = json.load(json_file) print(data)
This script will print the contents of the JSON file, ‘data.json’, as a Python object.
These are some of the basics of handling JSON files in Python that can take your data handling capabilities to the next level. The applications of Python JSON are broad and can significantly streamline your coding projects!
Python JSON: More Handling and Manipulation Techniques
1. Pretty-Printing JSON data
The `json.dumps()` function has parameters to make it easier to read the resulting JSON data. The `indent` parameter specifies how many spaces to use as indentation.
import json data = { "playerName": "Zenva Gamer", "completedCourses": ["Python Basics", "Advanced Game Development"] } json_obj = json.dumps(data, indent=4) print(json_obj)
This provides a nicely formatted output for readability:
{ "playerName": "Zenva Gamer", "completedCourses": [ "Python Basics", "Advanced Game Development" ] }
2. Sorting keys in JSON data
You can decide the ordering of keys in the JSON output by using the `sort_keys` parameter of `json.dumps()`.
import json data = { "playerName": "Zenva Gamer", "completedCourses": ["Python Basics", "Advanced Game Development"] } json_obj = json.dumps(data, indent=4, sort_keys=True) print(json_obj)
This time, keys in the output are sorted in ascending order:
{ "completedCourses": [ "Python Basics", "Advanced Game Development" ], "playerName": "Zenva Gamer" }
3. Handling Python Exceptions while working with JSON
You might encounter errors as you work with JSON files. Python raises an exception `JSONDecodeError` if it cannot parse a JSON file.
import json invalid_json_string = '{ "name":"Zenva", "courses:[ "Python", "Game Creation"]}' try: python_obj = json.loads(invalid_json_string) print(python_obj) except json.JSONDecodeError as jde: print("Invalid JSON", jde)
Invalid JSON Expecting property name enclosed in double quotes: line 1 column 23 (char 22)
4. Complex Python objects in JSON
Python’s json module cannot handle complex Python objects like custom classes. By defining `__str__()` in a class, we can control how object serialization takes place.
import json class Player: def __init__(self, name, level): self.name = name self.level = level def __str__(self): return f'Player [name={self.name}, level={self.level}]' player = Player('Zenva', 5) print(json.dumps(str(player)))
5. Using a Custom JSONEncoder
You can define a custom JSONEncoder to handle more complex Python objects, which aren’t serializable by default.
import json from json import JSONEncoder class Player: def __init__(self, name, level): self.name = name self.level = level class PlayerEncoder(JSONEncoder): def default(self, o): return o.__dict__ player = Player("Zenva Gamer", 5) playerJSON = json.dumps(player, indent=4, cls=PlayerEncoder) print(playerJSON)
This technique brings our exploration of Python JSON to a close. With these methods and examples, you’ll be better able to tackle data handling challenges with Python!
Where to Go Next
Your journey doesn’t have to end here! Now that the basics of Python JSON manipulation have been covered, there is still much more to explore and learn with us at Zenva.
One of the most comprehensive resources we offer is our Python Mini-Degree. The Python Mini-Degree is a thorough collection of courses teaching the incredibly versatile Python programming language. Well-known for its simplicity and a wide range of applications, Python is the tool of choice for many professional programmers and data scientists.
This Mini-Degree will guide you through projects where you will create games, algorithms, and practical real-world apps. It includes courses covering coding basics, algorithms, object-oriented programming, game development, and app development using popular libraries like Pygame, Tkinter, and Kivy.
The curriculum is designed to cater to both beginners and experienced learners focusing on a hands-on, practical approach with quizzes and challenges to reinforce your learning. Upon completion of the course, you will receive a certificate to acknowledge your newly acquired expertise and practical skills, which could open doors to job opportunities in various industries.
If you want to delve even deeper, you can explore all our Python Courses. These includes specifically curated courses to help you master the different aspects of Python programming.
Conclusion
Embracing the capabilities of Python JSON is an empowering skill for handling data, whether it’s for web development projects, game creation, or data analysis. We hope you found this tutorial informative and encouraging in your journey towards mastering Python.
As you continue acquiring new Python skills and deepening your understanding, remember that Zenva offers an extensive range of courses perfectly tailored to your needs. Our Python Mini-Degree package is a comprehensive platform that will facilitate your journey to becoming a professional Python programmer. So, why wait? Let’s get coding and see your bright future in Python programming unfold!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
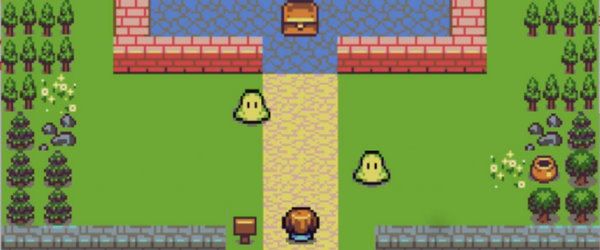
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.