Welcome coders! This time, we’re diving into a fundamental topic that often doesn’t get the limelight it deserves – CSV files in Python. However, as plain as it may sound, it’s a significant aspect of programming and data handling for a coder of any level.
Table of contents
What are Python CSV Files?
CSV, or Comma-Separated Values, is a simple file format that is used to store tabular data, like a spreadsheet or a database. Files in the CSV format can be imported to and exported from programs that store data in tables. In Python, CSV files are dealt with in a more programmer-friendly way.
Why Should You Learn it?
In the world of data science and web development, CSV files play a critical role as they are used to store and transport data. Whether you are dealing with a massive dataset or just need to save player data for your next game, knowing how to work with CSV files is a skill that every Python programmer should have in their toolkit.
Working with CSV files may not sound as thrilling as developing a game or creating a cutting-edge AI, but it’s one of those everyday tasks that you’re likely to encounter quite often. So, let’s tackle it now!
Opening a CSV File in Python
Opening a CSV file in Python is similar to opening a regular file and can be accomplished using the built-in open()
function. Let’s take a look at a simple example:
csv_file = open('myfile.csv', 'r') print(csv_file.read()) csv_file.close()
In this example, we’re opening the file 'myfile.csv'
in read mode (‘r’), reading its contents with the read()
method, printing the contents, and finally closing the file with close()
.
Reading CSV Files with the csv Module
Python provides a CSV module to handle CSV files. To read a CSV file using the csv module, we can use its reader function:
import csv with open('myfile.csv', 'r') as csv_file: csv_reader = csv.reader(csv_file) for row in csv_reader: print(row)
This code opens the CSV file and then initializes a CSV reader, which is used to read the contents of the file. We then loop through each row in the file and print it. Note how we are using with
to handle this file, which takes care of closing the file as soon as we’re done with it.
Writing to a CSV File
We can also write to a CSV file using the csv module. Here we’ll use writer
function:
import csv with open('myfile.csv', 'w') as csv_file: csv_writer = csv.writer(csv_file) csv_writer.writerow(["column1", "column2"]) csv_writer.writerow(["data1", "data2"])
Just like the reader, we instantiate a CSV writer using the csv module’s writer function. Then, we use the writerow()
method to write rows into the CSV file.
Reading and Writing Dict with csv Module
Sometimes, dealing with rows as lists can be less efficient, especially when dealing with large amounts of data. Python offers DictReader and DictWriter classes under the csv module to read and write data in the form dictionary objects:
import csv with open('myfile.csv', 'r') as csv_file: csv_reader = csv.DictReader(csv_file) for row in csv_reader: print(row)
import csv with open('myfile.csv', 'w') as csv_file: fieldnames = ['column1', 'column2'] csv_writer = csv.DictWriter(csv_file, fieldnames=fieldnames) csv_writer.writeheader() csv_writer.writerow({'column1': 'data1', 'column2': 'data2'})
The fieldnames
parameter is a list of keys that identify the order in which values in the dictionary should be written. The writeheader()
method writes a row with the field names (as specified by the fieldnames).
As you can see from these examples, CSV file handling in Python is made accessible and straightforward with the csv module. So, let’s start implementing it!
Manipulating Data with CSV Files
Reading and writing are just the beginning. Often, you’ll want to manipulate the data you read in from a CSV file. So, let’s look at how to do that.
import csv names = [] ages = [] with open('myfile.csv', 'r') as csv_file: dict_reader = csv.DictReader(csv_file) for row in dict_reader: names.append(row["Name"]) ages.append(row["Age"]) print(names) print(ages)
In the above example, we read a CSV file with columns “Name” and “Age” and load them into separate lists. This will allow us to perform operations or analysis on those arrays as needed.
Filtering Data from a CSV File
Data analysis often involves filtering and exploring data. Let’s look at how we can do this with CSV files in Python:
import csv with open('myfile.csv', 'r') as csv_file: dict_reader = csv.DictReader(csv_file) filtered_data = [row for row in dict_reader if int(row["Age"]) > 20] print(filtered_data)
Above, we read a CSV file and filter the data to include only those rows where the “Age” field is greater than 20.
Updating Data in a CSV File
Sometimes, you may want to modify data in a CSV file. One straightforward way to do this is to load the data, modify it in the script, and write it back to the CSV file:
import csv data = [] with open('myfile.csv', 'r') as csv_file: dict_reader = csv.DictReader(csv_file) for row in dict_reader: row["Age"] = int(row["Age"]) + 1 data.append(row) fieldnames = ['Name', 'Age'] with open('myfile.csv', 'w') as csv_file: dict_writer = csv.DictWriter(csv_file, fieldnames=fieldnames) dict_writer.writeheader() for row in data: dict_writer.writerow(row)
In this example, we read a CSV file, increment the “Age” field by 1 for all rows, and write the data back to the CSV file.
Dealing with Large CSV Files
What happens when you’re dealing with extremely large CSV files that won’t fit into memory? As developers, we don’t want our scripts to crash or consume excessive memory, which becomes crucial when dealing with large datasets. Fortunately, Python’s CSV reader objects are iterable, allowing us to read large files line by line, as shown below:
import csv with open('large_file.csv', 'r') as csv_file: csv_reader = csv.reader(csv_file) for row in csv_reader: print(row)
When coding, remember to pay attention to memory consumption and runtimes, especially when dealing with large amounts of data. Python offers a variety of memory-efficient ways to handle CSVs and other data sources.
Where to Go Next
Now that you have a solid understanding of how to handle CSV files with Python, it’s time to think: what’s next? Data handling and manipulation is a vital part of the vast landscape of programming, but there is so much more to explore!
Consider checking out our Python Programming Mini-Degree. Our Python Mini-Degree is a comprehensive collection of courses that generously covers both the basics and the advanced aspects of Python programming. This easy-to-follow curriculum includes creating engaging games, formulating algorithms, and developing real-world applications – all rolled into one exciting package! To boost understanding and facilitate active learning, the courses also feature quick challenges and in-course quizzes.
We understand that learners come from diverse backgrounds and cater to a variety of skill levels – whether you’re taking your first steps into coding or are a seasoned developer. Hence, you can start with entry-level courses and proceed at your pace. With the courses regularly updated to keep up with the industry’s evolution, learning Python has never been easier.
If you’ve got aspirations to publish games, land a job, start a business, or switch careers, the Python Mini-Degree could be a great place to start. With certified instructors and the flexibility to learn at any time and on any device, the Zenva journey is tailored to suit each learner’s needs. Plus, on completion of the courses, certificates are awarded as a testament to your newly acquired skills.
Go a step further and explore our entire collection of Python courses. From fundamentals to complex processes, our courses are designed to relay a clear, comprehensive understanding of Python programming. Start your journey with us today, and take your learning experience to the next level!
Conclusion
Whether you are a beginner setting foot into the vast world of coding or an experienced developer looking to strengthen your skills, handling and manipulating CSV files is a crucial skill to have. As we’ve seen, Python’s simplicity and power can undoubtedly bring any of your coding projects to life!
A solid grasp of Python is the new literacy in the modern workforce. As you move forward, we encourage you to take up our carefully crafted Python Programming Mini-Degree. This expansive and comprehensive learning journey will empower you to create games, develop apps, and manipulate data, among so much more. Leap across your learning hurdles and join us, as together we pave the way for your continued growth and success in the realm of coding.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
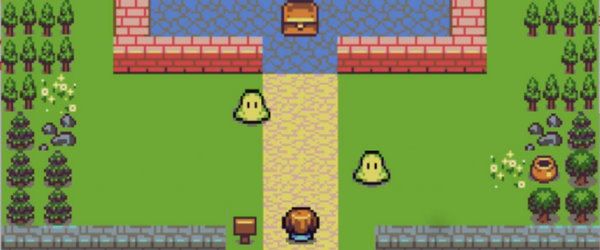
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.