Welcome to this comprehensive tutorial on the Python Interactive Shell! We are certain that by the end of this article, you’ll have a solid understanding of what it is, what it’s used for, and why you should add it to your toolkit of programming knowledge. Our aim is to ensure that you get the most out of the Python Interactive Shell and experience firsthand how it can enhance your coding journey.
Table of contents
What is the Python Interactive Shell?
The Python Interactive Shell is an environment where you can test out Python code. More than a mere code tester, it offers immediate feedback, allowing users to experiment freely. The shell is thus a quick way to validate your code and identify potential issues on the go.
Primarily, Python Interactive Shell is used for the rapid testing and debugging of code. A key feature of the shell is ‘read-eval-print loop (REPL)’, where it reads the code, evaluates it, and prints the output immediately.
Why should I learn it?
There are several convincing reasons to learn about the Python Interactive Shell:
- It’s ideal for practicing Python syntax and experimenting with new coding concepts.
- It enables on-the-spot debugging which accelerates the process of finding and fixing errors.
- It enhances your problem-solving skills as immediate feedback allows you to adjust your solutions in real time.
With these points in mind, learning the Python Interactive Shell can turn out to be an extremely useful endeavour for both beginners as well as experienced coders. The ability to see immediate results of your code without needing to create and run a full program can fine-tune your coding skills in an interactive way.
Now that we’ve covered what the Python Interactive Shell is, let’s get our hands on some code! In the next part of this tutorial, we will explore code examples that will help broaden your understanding further. The journey to Python proficiency is about to get even more interesting!
Getting Started with Python Interactive Shell
To begin, let’s first launch the Python Interactive Shell. Opening it is as straightforward as typing “python” on your command-line interface (CLI) and hitting enter:
$ python
You’ll be greeted with the Python version you’re currently running, as well as the prompt “>>>” which implies that the shell is ready to receive commands.
Running Python Statements
The Python Interactive Shell lets you run Python statements directly. Let’s start with a simple print statement:
>>> print("Hello, Zenva!")
This should output:
Hello, Zenva!
Performing Arithmetic Operations
On the Python Interactive Shell, you can perform simple arithmetic operations like addition, subtraction, multiplication, and division:
>>> 7 + 7 14 >>> 13 - 7 6 >>> 6 * 7 42 >>> 36 / 6 6.0
Creating and Manipulating Variables
You can also create variables and manipulate them as needed:
>>> x = 10 >>> y = 5 >>> z = x + y >>> print(z) 15
Using Built-in Python Functions
The shell facilitates the use of built-in Python functions:
>>> len("Zenva") 5 >>> min(5, 9, 3) 3
With these examples, you can see the power of Python Interactive Shell in offering instant output for your code. It’s an excellent learning tool for both experimenting with Python syntax and debugging snippets of code. In the next section, we’ll delve into more advanced usage of the Python Interactive Shell!
Working with Lists
One of Python’s most powerful data types is the list. Let’s see how we can create and manipulate lists in the Python Interactive Shell:
>>> my_list = [1, 2, 3, 4, 5] >>> print(my_list) [1, 2, 3, 4, 5] >>> my_list.append(6) >>> print(my_list) [1, 2, 3, 4, 5, 6]
Working with Dictionaries
Python dictionaries are a useful data type for storing information in key-value pairs. Here’s how to create and manipulate dictionaries in the shell:
>>> my_dict = {'apple': 1, 'banana': 2, 'cherry': 3} >>> print(my_dict) {'apple': 1, 'banana': 2, 'cherry': 3} >>> my_dict['dragonfruit'] = 4 >>> print(my_dict) {'apple': 1, 'banana': 2, 'cherry': 3, 'dragonfruit': 4}
Handling Errors
One of the benefits of using the Python Interactive Shell is instant feedback when there are errors in your code. Let’s demonstrate this:
>>> 1 / 0 Traceback (most recent call last): File "", line 1, in ZeroDivisionError: division by zero
Using Python Modules
You can even use modules directly in the shell, making it suitable for simple tasks. Here’s an example using the ‘math’ module:
>>> import math >>> math.sqrt(25) 5.0 >>> math.pow(5, 2) 25.0
These examples underscore the fact that the Python Interactive Shell is a powerful environment where you’re free to try out different things, see how Python works, and gain a better understanding of Python’s many nuances. Let’s explore some more advanced features of the Python Interactive Shell in our next section!
Where to go next?
Now that you’ve dipped your toes in the Python Interactive Shell and appreciated its practical utility, what’s next? It’s time to continue expanding your Python knowledge and skills!
To keep learning and augment your coding journey, check out Zenva’s Python Mini-Degree. This comprehensive collection of courses covers everything you need to know about Python programming – from the basics to more complex concepts.
Our Python Mini-Degree is more than just a set of courses. It’s a complete journey through Python programming where you’ll get the opportunity to create games, develop useful apps, and explore Python libraries like Pygame, Tkinter, and Kivy. No matter your current skill-level, we guide you through every step of the way with flexible course material that you can access anytime.
If you’re interested in a broader collection of Python topics, be sure to browse through our Python courses. Here you’ll find a wealth of knowledge that sets you on the path of Python mastery, from beginner to professional.
Conclusion
Congratulations on taking your first steps into the Python Interactive Shell! As you’ve seen, it’s an incredibly versatile tool that allows you to test, debug, and experiment with Python code in real time. This interactive nature makes it a superb learning environment, enabling you to explore the syntax and quirks of Python in ways no traditional program can.
Advancing your skills is just a matter of continued practice and exploration. Our Python Mini-Degree offers a comprehensive curriculum designed to take you from novice to proficient. Whether you’re interested in game creation, app development, or mastering algorithms, this mini-degree has you covered. Remember, every line of code you write brings you one step closer to mastery. Impatient for more? Let’s pick up from where we left and continue your Python journey together! Onwards, to coding glory!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
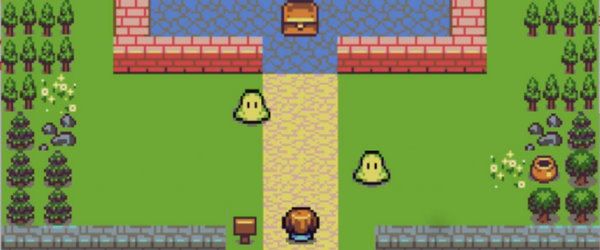
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.