Welcome to the fascinating world of command line arguments, a dimension of programming that unlocks powerful ways to interact with our software. As you embark on your coding endeavors or hone your advanced development skills, understanding the mechanics of command line arguments is essential. This knowledge paves the way to enhanced flexibility and a deeper grasp of how programs operate under different conditions. Stick with us, and you’ll learn how to harness command line arguments to give your applications new levels of dynamism and user interactivity.
Table of contents
What Are Command Line Arguments?
Command line arguments are inputs that are passed to a program when it is executed from a command-line interface (CLI). They are used to influence the behavior of the program by providing it with specific information or instructions right as it starts. Imagine you’re telling a video game character what equipment to start with or setting the difficulty level before the game begins – that’s what command line arguments do for your program.
What Are Command Line Arguments Used For?
They serve various purposes, from specifying configuration settings to passing data files for the program to process. Each argument can be thought of as a key piece to the puzzle that shapes how your software functions at runtime, just like choosing the game mode at the beginning of a match.
Why Should I Learn About Command Line Arguments?
As a developer, command line arguments are invaluable for crafting versatile applications. They provide the means to tailor software execution without altering the code. For game developers, they can add replayability and customization options to their games. Moreover, for data scientists and automation engineers, these arguments can streamline batch processing and task automation. All in all, learning about command line arguments is a step toward mastering the art of programming.
With this foundational understanding, we’ll next explore practical examples that illustrate how command line arguments can be used in various programming contexts, with a specific focus on Python. Whether you are a beginner looking for a solid start or an experienced coder aiming to refresh your knowledge, command line arguments are an essential tool for your developer’s toolkit. Let’s dive in!
Basic Command Line Argument Parsing in Python
Python provides a built-in module called sys that allows us to access command line arguments. The argv attribute of the sys module is a list in Python, which contains the command-line arguments passed to the script. Let’s see a simple example:
import sys print("Script Name:", sys.argv[0]) for i in range(1, len(sys.argv)): print(f"Argument {i}:", sys.argv[i])
This script will print the name of the script itself (accessible as sys.argv[0]) and all the arguments passed to the script.
Try running this Python script from the command line and pass some arguments:
python script.py arg1 arg2 arg3
You should see output similar to:
Script Name: script.py Argument 1: arg1 Argument 2: arg2 Argument 3: arg3
Using argparse for Advanced Argument Parsing
While the sys module is straightforward, Python also has the argparse module, which offers more advanced options for command line argument parsing. This module supports the creation of user-friendly command-line interfaces and permits you to specify what data type each argument should be, provide default values, and much more.
Begin by importing the module and creating a parser object:
import argparse parser = argparse.ArgumentParser(description="This script processes command line arguments.")
Next, let’s add some arguments with different data types and options:
parser.add_argument('integers', type=int, nargs='+', help='an integer for the accumulator') parser.add_argument('--sum', dest='accumulate', action='store_const', const=sum, default=max, help='sum the integers (default: find the max)')
Then, we parse the arguments and use them in our script:
args = parser.parse_args() print(args.accumulate(args.integers))
Run this script with the appropriate command:
python script.py 1 2 3 4 --sum
You should see the sum of the integers passed as arguments:
10
If you omit ‘–sum’, it will return the maximum value instead, by default.
Handling Optional Arguments
Optional arguments give your users flexibility. In argparse, optional arguments are prefixed with either “-” or “–“. Here’s how to define optional arguments:
# An optional argument with a default value parser.add_argument('-f', '--file', type=str, default='default.txt', help='The file to process') # A flag that, when present, stores a boolean value parser.add_argument('-v', '--verbose', action='store_true', help='Increase output verbosity')
With this setup, you can run the script without specifying a value for these options:
python script.py 5 6 -v
Or specify a file:
python script.py 2 4 -f data.txt
Both -f and –file are synonymous, and the verbose flag -v will set the corresponding variable to True if included.
Working with Argument Groups
For more complex command line applications, you might want to group related arguments together. The argparse module allows you to create argument groups for better help message organization:
parser = argparse.ArgumentParser(description="Example of using argument groups.") group = parser.add_argument_group('Group 1') group.add_argument('--foo', help='foo help') group2 = parser.add_argument_group('Group 2') group2.add_argument('--bar', help='bar help')
This will display two separate groups in the help message, each with its respective arguments.
When creating scripts that trigger off a variety of command line arguments, it’s essential to structure your code in a way that is not only functional but clear and maintainable. Utilizing the argparse module as shown in these examples will set you on the right path to developing command-driven Python applications with user-friendly interfaces.
As we continue, we’ll delve more deeply in the next part of this series, covering how these principles apply to real-world scenarios and practices, ensuring your expertise with command line arguments keeps growing.
Let’s explore more complex usage scenarios where command line arguments can transform your Python scripts into truly dynamic tools.
Sometimes, you might want to allow for multiple values for a single argument. This functionality can greatly enhance the script’s capability to process input. Using argparse, you can define an argument that can consume multiple values as follows:
# This argument can be specified several times to include multiple values parser.add_argument('--tags', nargs='+', help='List of tags to include')
When the script is run with the –tags option, all subsequent values are gathered into a list:
python script.py --tags adventure multiplayer indie
This can be particularly useful for filtering datasets, querying APIs, or managing multiple resources in a single command line instruction.
Another common use case is to provide mutually exclusive arguments where the presence of one argument invalidates the others. For instance, you might want a script that toggles between different modes but can only operate in one mode at a time:
group = parser.add_mutually_exclusive_group() group.add_argument('--verbose', action='store_true', help='Enable verbose mode') group.add_argument('--quiet', action='store_true', help='Enable quiet mode')
With a mutually exclusive group, users must choose either –verbose or –quiet and cannot specify both.
python script.py --verbose # or python script.py --quiet
Sub-commands are a feature when your script is meant to handle several different functions and each function might require its own set of arguments. Here’s how you might set up a script with sub-commands using argparse:
subparsers = parser.add_subparsers(dest='command') # Create the parser for the "a" command parser_a = subparsers.add_parser('a', help='a command help') parser_a.add_argument('bar', type=int, help='bar help') # Create the parser for the "b" command parser_b = subparsers.add_parser('b', help='b command help') parser_b.add_argument('--baz', choices='XYZ', help='baz help')
Now the script can be run with different sub-commands, each with their particular arguments:
python script.py a 12 # or python script.py b --baz X
This might be similar to git’s own interface, where git fetch and git push are sub-commands with their own specific arguments.
For scenarios where you might want to handle argument parsing errors or provide custom error messages, argparse allows for overriding the default behavior:
def error_handler(message): print(f"ERROR: {message}") parser.print_help() sys.exit(2) parser.error = error_handler
And finally, let’s look at using environment variables as arguments. In some situations, you might prefer to not pass sensitive information, like passwords, directly through the command line:
import os parser.add_argument('--password', default=os.environ.get('MYAPP_PASSWORD'))
Now, if MYAPP_PASSWORD is set in the environment variables, the script will use it as the default value for the –password argument. This is a secure and common practice for handling credentials.
python script.py --password # If MYAPP_PASSWORD is set, it will use that value; otherwise, it prompts for a password.
Throughout this exploration, we’ve seen how even simple command line tools can provide rich and intuitive interfaces for users. With Python’s powerful argparse and the previously mentioned sys modules, you’re equipped to build scripts that are not only easy to use but also robust in handling a variety of input configurations.
To reach this level of proficiency in Python, learning with Zenva can be a game-changer. We offer a wide range of courses that cover the foundational knowledge all the way to advanced skills—perfect for turning the potential of command line arguments into a meaningful asset in your coding toolkit. Transform your ideas into reality as you grow to become a Python maestro with us by your side.
Let’s dive further into the world of command line arguments in Python, exploring additional ways in which you can enhance your command line interfaces (CLIs). These examples continue to build on the argparse module, a versatile and powerful tool in Python’s standard library designed to facilitate the parsing of command line arguments.
When developing a CLI, you might want to assign special meaning to certain argument values. The argparse module allows you to do this through the use of custom action classes. Here’s an example of defining a custom action that would convert a passed argument into uppercase:
import argparse class UppercaseAction(argparse.Action): def __call__(self, parser, namespace, values, option_string=None): setattr(namespace, self.dest, values.upper()) parser.add_argument('echo', action=UppercaseAction) args = parser.parse_args() print(args.echo)
Now, if you passed a string to the argument, it would automatically convert to uppercase:
python script.py hello world # Output: HELLO WORLD
The argparse module also provides support for type conversion of the command line arguments. You can specify the type of an argument so that the input is automatically converted to your desired type:
parser.add_argument('--number', type=int, help='An integer number') # Now, if we provide a string that represents an integer, it is automatically converted:
python script.py --number 123 # '123' becomes an integer 123
Often, CLI tools require configuration files. Instead of passing numerous arguments through the command line, you can use arguments to specify the path to a file and read configurations from there:
import json parser.add_argument('--config', type=str, help='Path to the configuration file') args = parser.parse_args() if args.config: with open(args.config, 'r') as f: config = json.load(f) print(config)
By designating a config file, you can significantly streamline the command line instructions:
python script.py --config settings.json
Furthermore, you can combine the use of environment variables and default argument values to create fallback mechanisms for your script:
parser.add_argument('--user', default=os.environ.get('USER', 'default_user'))
In this case, the script uses the USER environment variable if it exists; otherwise, it falls back on a default value.
What if you want to process arguments conditionally based on their value? Using argparse, you can define a custom type function, which processes the argument right upon parsing:
def even_number(number): number = int(number) if number % 2 != 0: raise argparse.ArgumentTypeError('Only even numbers are allowed') return number parser.add_argument('--even', type=even_number)
This additional layer of validation ensures that the –even argument passed to the script will always hold an even number:
python script.py --even 10 # Valid input, script continues python script.py --even 5 # Raises an error and does not continue
Effective error handling and custom responses are key aspects of user-friendly CLIs. Customize the error message for better user guidance with ArgumentTypeError:
def positive_number(value): ivalue = int(value) if ivalue <= 0: raise argparse.ArgumentTypeError(f"{value} is an invalid positive int value") return ivalue parser.add_argument('--positive', type=positive_number)
By implementing such detailed feedback, you empower users to rectify the error promptly:
python script.py --positive -5 # Output: error: -5 is an invalid positive int value
Through these examples, you can appreciate the versatility of argparse. It proves to be an indispensable framework for building interactive, friendly, and robust CLIs in Python. These snippets just scratch the surface—each project brings unique requirements, calling for tailored implementations of command line argument parsing.
At Zenva, we understand the impact of practical coding experience. Therefore, our courses are designed to tackle real-world problems and utilize these kinds of tools to build meaningful projects. Whether you’re scripting for data analysis, automating tasks, or developing a full-scale application, understanding command line argument parsing with Python is crucial. By learning through us, you’re setting yourself up with the skills to create efficient and user-centric command line applications.
Continuing Your Learning Journey in Python
Congratulations on completing this tutorial! You’ve taken valuable steps in mastering command line argument parsing with Python, a skill that lays the groundwork for building sophisticated and powerful applications. But the learning doesn’t stop here. To further refine your Python expertise and expand your programming knowledge, we warmly invite you to explore our Python Mini-Degree. This comprehensive program is your next step towards proficiency in one of the most versatile programming languages in today’s tech landscape.
The Python Mini-Degree is designed to take you from the basics of coding to the creation of your own projects, including games, applications, and even AI chatbots. With our self-paced, flexible courses, accessible anytime and anywhere, you’re not just learning Python; you’re preparing for a thriving career in programming.
If you’re eager to broaden your programming horizons beyond Python, our catalog of Programming Courses covers an array of topics that can cater to your growing interests and needs. At Zenva, we’re committed to helping you achieve your goals, whether you’re just starting out or looking to enhance your professional skill set. Journey with us to continue shaping your future in the world of tech.
Conclusion
Embarking on your Python programming journey with a clear understanding of command line arguments equips you with a powerful tool for creating high-performance and flexible applications. You now possess the knowledge to craft intricate command line interfaces, breathe life into your software ideas, and tailor programs to your or your users’ specific needs. As you move forward, keep nurturing your skills, as every line of code written is a step towards mastery.
We at Zenva are thrilled to be part of your learning path and to witness your growth as a developer. Our Python Mini-Degree awaits to offer you even more in-depth training, fostering your capabilities and enabling you to conquer new heights in this digital era. Continue your adventure with us, and let’s code a brighter future together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
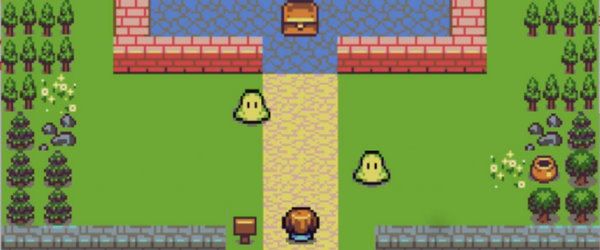
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.