Alright, let’s dive into the world of Python Enums!
Enumerations, also known as enums, are a powerful feature in many programming languages, including Python. They provide a systematic way of dealing with constants, giving you cleaner and more understandable code. But what exactly are Python enums, and why should you learn about them? In this tutorial, we aim to answer these questions and more as we delve into the basics and uses of enums for your Python coding journey.
Table of contents
What are Enums?
In Python, enums are a means of creating symbolic names for constant values. These symbolic names can make your code significantly cleaner and more readable. Instead of dealing with abstract numbers or strings, you can work with clearly defined names that clarify their purpose and value in your program.
Enums are used when a variable can only take one out of a small set of possible values. Think of enums as the ‘settings’ for a game character, where you want the character to exist in one of a limited number of ‘states’. For example, your character’s mood might be ‘happy’, ‘sad’, or ‘angry’ – three states that are best represented by an enum.
Why Should You Learn About Enums?
Learning about enums can greatly enhance your productivity and code readability. Here are a few reasons why learning about enums is beneficial:
- Efficiency: Enums restrict variables to a confined set of potential values, preventing the need for additional logic to constrain your values.
- Readability: Enums make your code easier to read and understand, as the purpose of constants becomes immediate and clear.
- Error Avoidance: Enums help prevent errors and bugs in your code by ensuring that variables cannot take incorrect or unintended values.
In the following sections, we will dive into more depth about Python enums, including some engaging examples to help you understand their use.
Creating Enums in Python
In Python, you can create an enum using the Enum class from the built-in enum module. Here’s a basic example:
from enum import Enum class Mood(Enum): HAPPY = 1 SAD = 2 ANGRY = 3
The Mood enum we created has three elements, each with a unique value.
You can now use this enum in your code:
myMood = Mood.HAPPY print(myMood)
This will print:
Mood.HAPPY
Working with Enum Values and Names
Each enum member has a name and a value which you can access by using the .name and .value attributes:
print(myMood.name) print(myMood.value)
This code will output:
'HAPPY' 1
Iterating Over Enums
Another great thing about enums is they are iterable. This means you can loop over all the members of an enum:
for mood in Mood: print(mood)
This will print:
Mood.HAPPY Mood.SAD Mood.ANGRY
Using Enums in Conditionals
Enums are great to use in conditional statements as they enhance code clarity. Here’s an example:
if myMood == Mood.ANGRY: print("I need to calm down.") elif myMood == Mood.HAPPY: print("I'm feeling great!") else: print("I'm a bit down today.")
With the enum, it is clear what each condition is checking. This greatly improves the readability of your code.
In the third part, we will look at auto enumeration and comparison of enums. Stay tuned!
Auto Enumeration in Python
In Python, you don’t have to manually assign values to the enum members. The auto() function will do that for you. It generates consecutive integer values starting from one.
Here’s an example of auto enumeration:
from enum import Enum, auto class Weather(Enum): SUNNY = auto() CLOUDY = auto() RAINY = auto() SNOWY = auto()
Each member of the Weather enum now has an integer value assigned automatically by Python.
Comparing Enums
One important point to understand about enums is that the comparison is identity-based, not value-based. This means that even if two enum members have the same value, they are not considered equal because they are different instances.
See the example below:
class Colors(Enum): RED = 1 BLUE = 2 class Shapes(Enum): CIRCLE = 1 SQUARE = 2 print(Colors.RED == Shapes.CIRCLE)
This will output:
False
Despite both having a value of 1, Colors.RED and Shapes.CIRCLE are separate enums and are not equal.
Accessing Enum Members
If you need to get an enum member by its name, you can use the following method:
print(Colors['RED'])
This will output:
Colors.RED
Similarly, if you need to get an enum member by its value, you can use:
print(Colors(1))
This will output:
Colors.RED
Continuing Your Python Journey With Zenva Academy
Having dipped your toes into the world of Python with enums, it’s essential to maintain this momentum in order to grow as an aspiring Python developer. At Zenva, we’re committed to providing comprehensive learning paths and resources that cater to different skill levels – from beginners to professionals.
We’d recommend checking out our Python Mini-Degree. This is a systematic compilation of courses designed to guide you in mastering Python programming. Python, due to its simplicity and versatility, makes it a highly sought-after skill in today’s job market.
In our Python Mini-Degree, you’ll grasp various topics including Python basics, algorithms, object-oriented programming, game and app development, and more. The course emphasizes learning by doing, which means you’ll get the opportunity to build real-world games, apps and algorithms, and to create a impressive portfolio of Python projects.
For those of you who are looking to gain a broader understanding of Python, we proudly present our comprehensive collection of Python Courses. Catering to every programmer’s learning needs, these courses range from the basics of Python to the more advanced topics such as data analysis and machine learning.
Conclusion
Congratulations on taking this step in exploring enums in Python and boosting your coding efficiency. Understanding and utilising enums are a huge part of honing your Python skills, making your code expressive, and aligning yourself with professional coding practices.
Continuing your Python journey and nurturing your passion for coding is as accessible as ever with us here at Zenva. With our Python Programming Mini-Degree, you’ll grasp the full spectrum of Python’s potential, making this language an ally in your developer journey. Venture on, coder – we’re excited to share more of Python’s dynamic universe with you.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
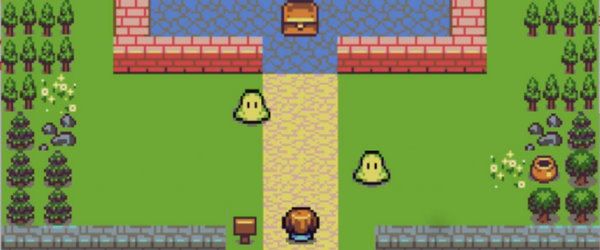
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.