Welcome to our comprehensive tutorial on Python Enumeration! Beyond just a core aspect of Python programming, the concept of enumeration is a cool, powerful, and incredibly useful tool for handling different kinds of tasks in Python. And the best part? It’s easy to understand and use, even for beginners.
Table of contents
What is Enumeration in Python?
Enumeration in Python is a built-in function that allows you to add a counter to an iterable object. The item’s index is also returned, along with the item itself. In essence, it provides a more efficient way to iterate over sequences.
What is Enumeration Used For?
When dealing with looping constructs in Python programming, there comes a time when we need the position or index of an item in a sequence. Be it list, string, or array, knowing the element’s position can come in handy in a multitude of operations, from sorting and searching to manipulating data. This is where enumeration comes in!
Why Should You Learn Enumeration?
Firstly, enumeration plays a vital role in enhancing the readability of your code, which is crucial when you’re working in a team or even when you’re dabbling with personal projects. Secondly, enumeration offers a more Pythonic approach to handling iterations. It’s clean, effective, and efficient. And finally, it often simplifies complex operations by providing an easy way to simultaneously access an element and its index in a sequence.
Whether you’re an experienced coder or just starting on your journey, getting to grips with enumeration will level up your Python game. So, let’s dive in and learn more about this fascinating aspect of Python programming.
Part II: Basic Usage of Enumeration in Python
Let’s begin by implementing a basic example of enumeration. Here we’ll create a list and iterate over it using the enumerate() function.
fruits = ['Apple', 'Banana', 'Grapes', 'Pear', 'Cherry'] for i, fruit in enumerate(fruits): print("The index of {} is {}".format(fruit, i))
In the above code, enumerate() adds a counter to the fruits list and returns it in a form of enumerate object that we can loop over to get both the elements and their indexes.
Using Start Parameter in Enumeration
Python’s enumerate() function also allows us to specify the starting index of the counter. We can do this using the start parameter. Let’s see an example:
fruits = ['Apple', 'Banana', 'Grapes', 'Pear', 'Cherry'] for i, fruit in enumerate(fruits, start=1): print("The index of {} is {}".format(fruit, i))
In the above example, we used a start parameter of 1, so the counter starts from 1 instead of the default 0.
Part III: Advanced Enumeration Techniques
Now let’s move ahead and tackle some more advanced applications of the enumerate() function.
Enumerating Over Strings
Remember, enumeration in Python isn’t just limited to lists. You can enumerate over any iterable, including strings. Let’s witness the magic:
text_string = "Zenva" for i, letter in enumerate(text_string): print("The index of {} is {}".format(letter, i))
Getting Index and Value in a Dictionary
What if we had a dictionary and we want both keys and values indexed? That’s possible too! Here’s how:
# create a dictionary seasons = {'Spring': 'March', 'Summer': 'June', 'Fall': 'September', 'Winter': 'December'} # use enumerate on the dictionary items for i, (key, value) in enumerate(seasons.items()): print("The index of season {} which starts in {} is {}".format(key, value, i))
The above script will print the index of each key-value pair in the dictionary.
By understanding and mastering these techniques using enumeration in Python, you will make your programming journey a smoother one. Happy coding!
Part IV: Enumeration in List of Tuples
We can iterate over a list of tuples using enumeration. Such as:
student_list = [('John', 'A'), ('Sarah', 'B'), ('Tom', 'A'), ('Emma', 'C')] for i, (name, grade) in enumerate(student_list): print("The student {} got grade {} and has index {}".format(name, grade, i))
In this example, we used tuple unpacking within the enumerate() function to separate elements within the tuples.
Enumeration and List Comprehension
Enumeration can also be used with list comprehension, a powerful Python feature that allows you to create lists from existing lists by applying an expression. Look at the following example:
fruits = ['Apple', 'Banana', 'Grapes', 'Pear', 'Cherry'] indexed_fruits = [(i, fruit) for i, fruit in enumerate(fruits)] print(indexed_fruits)
In the above example, we used enumeration inside a list comprehension to generate a new list of tuples, where each tuple includes the index and the corresponding element from the original list.
Enumeration in Nested Loops
If you want to use enumeration in nested loops, that’s possible too!
outer_list = [['John', 'Sarah', 'Tom'], ['Apple', 'Banana', 'Grapes']] for i, inner_list in enumerate(outer_list): for j, item in enumerate(inner_list): print("The index of {} in outer list {} is {}.".format(item, i, j))
This example showcases the usage of enumeration in nested loops and how they provide more control over indexes in complex data structures.
Conditional Checks with Enumeration
Enumeration can also work with conditional statements seamlessly. Let’s see an example:
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry'] for i, fruit in enumerate(fruits): if fruit.startswith('b'): print("The index of the first fruit starting with 'b' is", i) break
Here we are using enumeration and a conditional check together to halt the loop as soon as we encounter the first fruit starting with ‘b’.
Enumeration is a wonderful tool in Python that helps us not only to keep track of elements but also makes our code cleaner and efficient. Now that you know how it works, it’s time to put your newfound knowledge into practice. Happy learning!
Where to Go Next?
You’ve learned the powerful utility of enumeration in Python, and you’re on the right track in your coding journey. Naturally, you might be asking, “Where do I go from here?” We’re here to guide you through that.
Our comprehensive Python Mini-Degree is the perfect platform for you to learn Python programming, taking you from beginner to professional. This set of in-depth courses covers a plethora of topics ranging from coding basics, algorithms, object-oriented programming, to game development and app development.
Why choose our Python Mini-Degree?
- You get to learn by doing. The mini-degree contains hands-on projects like creating your own games, implementing your algorithms, building real-world apps, and even developing an AI medical diagnosis bot.
- Python is a widely demanded skill in the job market, especially in the fields of data science. Mastering Python can open doors for numerous career opportunities.
- The learning content is continuously updated, ensuring your training remains relevant in keeping with industry practices.
Whether you’re aiming to land your dream job, thinking about a career change, or seeking to launch your own business, our Python Mini-Degree is designed to arm you with the necessary skills and knowledge.
What After Basic Python?
If you’re past the basics and looking to delve into intermediate Python, we’ve got you covered as well. Check out our advanced Python courses, designed to cater to learners seeking to deepen their expertise in Python. Detailed, practical, and comprehensive, our courses are an excellent resource for dedicated learners.
At Zenva, we’re proud to have supported over 250 high-quality courses geared towards empowering individuals by teaching them the skills they need to thrive. Consider us your partners in this journey to success. Start learning today and see where your coding skills take you!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
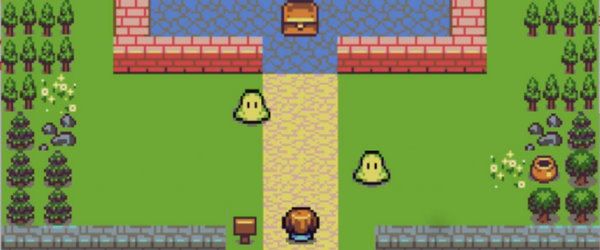
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.