Welcome to this comprehensive tutorial on Python control flow. In this tutorial, we’re going to delve deep into the inner workings of Python, a widely-used and powerful programming language. Whether you’re a newbie on your coding journey, or an experienced developer looking to brush up your skills, this tutorial has something for you. So, let’s get started!
Table of contents
What is Python Control Flow?
In Python, and indeed any programming language, control flow is an essential concept. It’s how we determine the order in which the instructions, or ‘statements’ in our code are executed. This allows us to dictate how our program will respond to different conditions and inputs – it’s the brain behind your code, so to speak.
Python control flow is used to handle complex logic and decision making in a program. With the use of statements such as loops and conditional if..else, we can create dynamic programs that go beyond linear execution and react differently depending on various factors. This is crucial in game development, data analysis, AI, and essentially any application that requires interactive or dynamic behaviour.
Why should I learn Python Control Flow?
Understanding control flow in Python is fundamental to mastering the language and enhancing your programming skills. Whether you aim to explore the exciting world of game development, delve into the intricacies of AI, or shift your career to a more tech-oriented field, a solid grasp of control flow will take you far. It opens up opportunities for creating more complex, efficient, and dynamic code, making your programs smarter and more interactive. Besides, Python is loved for its readability and ease of learning; thus, understanding its control flow is a manageable task that yields great results.
Python Control Flow Basics
Now let’s get our hands dirty with some actual code. First, we’ll show you how to use conditional statements in Python.
Conditional Statements
The most basic form of control flow in Python is the if-elif-else statement, which allows the program to evaluate whether certain conditions are met and to perform different actions based on the results.
temperature = 25 if temperature > 30: print("It's too hot!") elif temperature < 10: print("It's too cold!") else: print("The weather is perfect!")
In this code, the program checks the value of the ‘temperature’ variable and prints a different message based on its value.
Loops
Loops are used to run a section of code multiple times. The loops in Python are for and while loops.
For Loop
for i in range(5): print("Loop iteration: ", i)
This for loop runs 5 times, printing the iteration number each time.
While Loop
count = 0 while count < 5: print("Count: ", count) count += 1
This while loop also runs 5 times and performs a similar operation.
Using Break and Continue
Sometimes, we might need to exit a loop prematurely or skip one iteration. Python provides the break and continue statements for this purpose.
Break:
for i in range(10): if i == 5: break print(i)
The program exits the loop as soon as i equals 5.
Continue:
for i in range(10): if i == 5: continue print(i)
Here, when i equals 5, the program skips that iteration and continues with the next one.
The possibilities with Python’s control flow mechanisms are endless. We hope this tutorial gives you a good start to explore more!
Nesting Control Statements
Control statements can be nested within one another to create more complex logic structures.
for i in range(5): for j in range(i): print('i:', i, 'j:', j)
This code runs two nested loops. The outer loop runs 5 times, and for each iteration of the outer loop, the inner loop runs a number of times equal to the current value of i from the outer loop.
Else Statements in Loops
You may not know this, but Python loops can have an optional else clause, which is executed when the loop finishes normally (i.e., it’s not broken by a break statement).
for i in range(5): print(i) else: print("Loop finished successfully!")
This loop prints numbers from 0 to 4, and then prints “Loop finished successfully!” because the loop completed its full run without encountering a break statement.
List Comprehensions
Python features a powerful tool known as list comprehension that allows you to create and manipulate lists in a single, succinct line of code.
squares = [x ** 2 for x in range(10)] print(squares)
This creates a list of squares of numbers from 0 to 9.
The pass Statement
Finally, Python has a special statement known as pass. The pass statement does nothing—it serves as a placeholder when a statement is syntactically required but you don’t want any code to execute.
for i in range(5): if i == 3: pass print(i)
Here, when i equals 3, the pass statement ensures that the program does nothing for that iteration. As you see, Python control flow is both flexible and powerful, and understanding it is crucial to developing your Python skills!
Where to go next
Well done on making it this far! You’ve armed yourself with a fundamental aspect of Python, but don’t stop here. There are innumerable ways in which these concepts can be used and various avenues to explore. As you continue to learn and grow as a programmer, you’ll encounter the need for a firm grasp on control flow time and time again.
If you’re excited to learn more, check out Zenva’s Python Mini-Degree program. It’s a comprehensive collection of courses that help you delve further into Python programming. From coding basics to complex algorithms, from game development to app programming, this rich resource spans a multitude of topics to boost your learning journey.
The beauty of the Python Mini-Degree is that it’s designed for both beginners and more experienced programmers. Our courses are meticulously curated and updated regularly to keep up with industry developments and to provide you with the most relevant learning content.
Additionally, we also invite you to check out our broader Python course offerings. Whether you’re seeking to transition your career, expand your skillset, or simply quench a thirst for learning, we’ve got you covered.
Conclusion
Python control flow is the heart of any program, driving the logic and functionality. Understanding it well will empower you to build more complex, intelligent, and user-friendly applications. We hope that this tutorial has given you a comprehensive overview as well as some practical insights into Python control flow. Remember, the journey of programming is continuous, and every step you take in learning and practicing will take you closer to your goal.
Are you ready to build more advanced programs, games or even your own applications? Join the thrilling journey with Zenva’s Python Mini-Degree program. Diverse topics, expert instructors, an active community, and real-world projects await you. Take the leap and start your journey with us today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
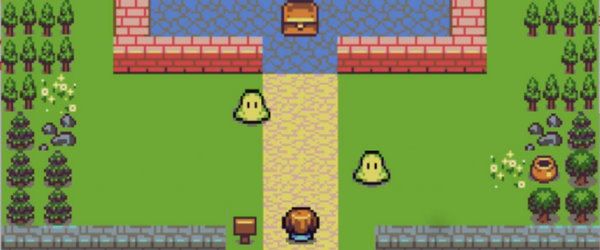
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.