Welcome to this vibrant tutorial, where we aim to tackle the python library, pygame, with a specific emphasis on drawing text. Python pygame enhances your gaming development skills, serving as a practical tool to learn and create media-rich applications including games. Our tutorial will take you through simple, engaging, and practical examples showing how you can utilize pygame in your game development projects.
Table of contents
What is Pygame?
As we delve deeper into this tutorial, it’s important to understand what pygame really means. Pygame is a set of Python modules designed explicitly for video game creation. It’s built on SDL (Simple DirectMedia Layer) library, making it highly portable and enabling it to run seamlessly across multiple platforms.
What is it for?
Essentially, pygame is primarily used for creating 2D games. Whether it’s simple Tetris or the complex science fiction game, pygame provides the requisite tools to bring your creativity to life. It’s incredibly efficient, providing developers with the leeway to focus on game dynamics as opposed to the rudimentary elements of game creation.
Why should you learn it?
Why should you really get to grips with pygame, and specifically, ‘draw text’ in pygame? Firstly, pygame offers a simplistic yet robust structure to design and implement engaging computer games. Drawing text’ in pygame improves the user experience by enhancing interactivity, engagement, and aesthetics in your game.
Using pygame to draw text leads to games with clear instructions, purpose, dialogue, or prompts, ultimately guiding your player through the gaming journey. It gives your game a voice and context, making it far more engaging and immersive.
Stay with us throughout this tutorial and discover the immense benefits pygame can bring into your coding portfolio. Let’s jump straight into it!
Setting up your Pygame environment
First off, you’ll need to install pygame on your Python environment. This operation is rather simple with pip:
pip install pygame
To verify the installation, you can import pygame in Python:
import pygame
Creating a basic Pygame Window
To begin creating your game, you first initiate the Pygame modules with `pygame.init()`. You then define the window size and create the game’s main loop:
import pygame pygame.init() # define the RGB value white = (255, 255, 255) # assigning values to x and y variable x = 400 y = 300 # creating window gameWindow = pygame.display.set_mode((x, y)) # game's main loop running = True while running: gameWindow.fill(white) for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
Adding Text to Your Game
The `pygame.font` module will enable you to add text to the game.
First, you should define the font and size of the text. Then, create the text and its rectangle, placing it on the window:
# define the font font = pygame.font.Font(None, 36) # create the text text = font.render("Hello, Zenva!", 1, (10, 10, 10)) # create the text's rectangle textpos = text.get_rect(centerx=gameWindow.get_width()/2) # place the text on the window gameWindow.blit(text, textpos) pygame.display.update()
Enhancing Text Visibility
To improve the visibility of your text, consider ‘drawing’ a background for it. Here, we’ll show you how to add a rectangle behind your text:
# Draw the rectangle pygame.draw.rect(gameWindow, (200, 200, 200), textpos.inflate(10, 10)) # Place the text on the window gameWindow.blit(text, textpos) pygame.display.update()
The ‘inflate’ method makes the rectangle slightly larger than the text. This enhances visibility by providing a buffer and a distinctive background for your text.
Remember that experimentation is key to mastering pygame. Practice adding different text, shape, and color variations. Happy coding!
Changing Text Properties
Changing the font properties will also significantly change the feel of your game. Let’s adapt font size, boldness, and italics:
fontBig = pygame.font.Font(None, 72) fontBold = pygame.font.Font(None, 36) fontBold.set_bold(True) fontItalic = pygame.font.Font(None, 36) fontItalic.set_italic(True)
With the above code, we have created a larger font, a bold font, and an italicized font.
Custom Fonts
Pygame also enables you to use custom fonts. Ensure you have the font file in the working directory and reference it when creating the Font object:
fontCustom = pygame.font.Font('customfont.ttf', 36)
In this scenario, replace ‘customfont.ttf’ with the name of your own font file.
Animating Text
Moving or animating text is just the same as animating any other Surface object in pygame. You update the location of the rectangle used to place the text.
textpos = text.get_rect(centerx=gameWindow.get_width()/2) speed = 1 running = True while running: gameWindow.fill(white) gameWindow.blit(text, textpos) textpos = textpos.move(speed, 0) if textpos.right > gameWindow.get_width(): textpos.left = 0 pygame.display.update()
A point to note: the move method creates a new Rect object. It does not modify the existing one. Always assign the result back to the original variable to ensure that changes persist.
Rotating Text
Pygame can also rotate text. Keep in mind, however, that rotating text can blur it a bit, especially at smaller sizes or non-90-degree rotations:
font = pygame.font.Font(None, 36) text = font.render("Hello, Zenva!", 1, (10, 10, 10)) textpos = text.get_rect(centerx=gameWindow.get_width()/2) text = pygame.transform.rotate(text, 45) gameWindow.blit(text, textpos) pygame.display.update()
In this example, we have rotated the text by 45 degrees.
We hope these pygame examples enable you to draw text and enrich the aesthetic appeal of your gaming projects effectively. Practice, understand, and construct more complex game models with Python and pygame to make your video games more engaging.
Handling Text Input
In some situations, capturing user’s text input may be of paramount importance to your game design. Pygame allows for such flexibility. Observe how to draw an input box and record user’s input:
running = True text = '' while running: gameWindow.fill(white) for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: if event.key == pygame.K_BACKSPACE: text = text[:-1] else: text += event.unicode font = pygame.font.Font(None, 50) txt_surface = font.render(text, True, (0, 0, 0)) gameWindow.blit(txt_surface, (50, 50)) pygame.display.update() pygame.quit()
This snippet captures and displays the user’s keyboard input within the game window in real time.
Flickering Text
Creating a flickering effect with your text can add an intriguing dynamic to your game. The trick is to keep track of elapsed time and determine the visibility of the text based on it:
elapsed_time = 0 visible = True clock = pygame.time.Clock() font = pygame.font.Font(None, 50) text = font.render("Get Ready!", True, (0, 0, 0)) textpos = text.get_rect(centerx=gameWindow.get_width()/2) running = True while running: gameWindow.fill(white) if visible: gameWindow.blit(text, textpos) pygame.display.update() elapsed_time += clock.tick(60) if elapsed_time > 500: elapsed_time = 0 visible = not visible for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
In this example, the drawn text flickers every half a second.
Zooming Text
Animating text to give a zooming effect can add another dimension to your game. Here’s how you can implement it:
font = pygame.font.Font(None, 36) text = font.render("Level Up!", True, (0, 0, 0)) textpos = text.get_rect(centerx=gameWindow.get_width()/2) zoomed = pygame.transform.scale(text, (textpos.width*2, textpos.height*2)) running = True while running: gameWindow.fill(white) gameWindow.blit(zoomed, zoomed.get_rect(centerx=gameWindow.get_width()/2, centery=gameWindow.get_height()/2)) pygame.display.update() for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
In this case, the text appears twice as large, giving a ‘zoomed-in’ effect.
Color Changing Text
Finally, changing the color of your text can be a convenient way to grab your player’s attention or signal different game stages:
running = True color = (0, 0, 0) while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False font = pygame.font.Font(None, 50) text = font.render("Game Over!", True, color) gameWindow.fill(white) gameWindow.blit(text, (50, 50)) pygame.display.update() color = (color[0] + 1, color[1] + 1, color[2] + 1) pygame.quit()
In this snippet, the color of the drawn text changes gradually from black to white.
Remember, mastering these pygame skills won’t happen overnight. With regular practice and some creativity, you’ll soon be creating spectacular 2D games using pygame. Happy coding!This Pygame tutorial has set you on the right path to create engaging and creative games with Python. However, the journey just begins here. To boost your learning and enhance the practical application of your skills, we recommend more extensive training.
We strongly encourage you to check out our Python Mini-Degree. This comprehensive collection offers courses in Python programming, covering coding basics, algorithms, object-oriented programming, game development, and app development. Designed for both beginners and more advanced learners, these courses will provide you with step-by-step real-world projects, expanding your skill set at your own pace.
If you wish to explore a more diverse set of Python courses, take a look at our Python Course Collection. With over 250 supported courses, Zenva Academy continues to foster a dedicated community of over a million learners, instructors, and game developers. Keep the journey going, and make Python your second language! Zenva is here to support you in your efforts to become a professional developer.
Conclusion
With Python and Pygame, you are well on your way to building engaging and striking 2D games that can reach audiences worldwide. The skill of drawing text in Pygame is just one of many that you’ll garner as you delve deeper into your game development journey.
We encourage you not to stop at this tutorial. Continue your Python and Pygame exploration with our comprehensive Python Mini-degree, where you can learn other valuable game development skills. At Zenva, we remain your dedicated partner in your coding journey, committed to providing you with high-quality learning resources. Keep practicing and happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
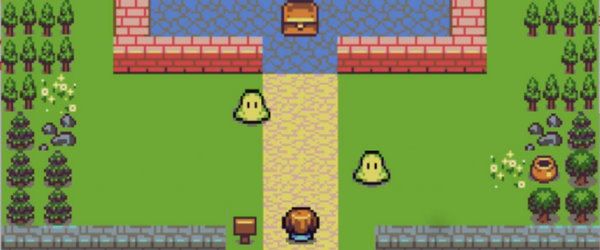
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.