Welcome to our definitive guide on Pygame Text. This engaging tutorial will equip you with the knowledge needed to implement text into your Pygame projects, allowing for a more interactive and immersive gaming experience. Whether you’re a seasoned coder or a novice beginning your coding journey, this article promises to deliver value and intrigue.
Table of contents
What is Pygame Text?
Pygame Text is essentially the way we handle and manipulate written content in Pygame, a popular open-source library for Python. This module allows developers to utilize text in their gaming applications, enabling them to build dialogue systems, scoring displays, instructional cues, and other text-based features.
What is it for?
In any game, be it simple or complex, text plays a pivotal role. It forms the basis for setting narratives, communicating game progress and instructions, displaying scores, and enhancing overall user interaction. The magic of text equips a game with ‘a voice’, making it more engaging and interactive.
Why should I learn it?
Learning Pygame Text is an essential step in your game development journey for several reasons:
- It enables you to build better communication within your games.
- Text can provide hints, display scores, and set the tone of your game.
- Mastering Pygame Text can improve the overall user experience of your game, making it more enjoyable and immersive.
Whether you’re an indie developer looking to spice up your pet project, or a student polishing your game development skills, the relevance and significance of Pygame Text cannot be overstated. We believe that mastering this skill will be an asset to your programming toolkit. Rest assured, learning and eventual mastery is an enjoyable journey, not an uphill battle.
Getting Started with Pygame Text
Let’s start with the basics and learn how to display a simple piece of text.
Firstly, we need to define the font and size of the text. Pygame has an inbuilt method for this, which we can call like this:
font = pygame.font.Font('freesansbold.ttf', 32)
In the above code, ‘freesansbold.ttf’ is the font that we want to use, you can replace it with any font of your choice. The number 32 in the code is the size of the font.
Now that we have our font, we’re ready to render the text. With Pygame, we generate a surface that contains our text. This is achieved using the ‘render()’ function. Here is how you can do this:
text = font.render('Hello World', True, (0, 0, 0), (255, 255, 255))
We have created a text saying ‘Hello World’. The second argument ‘True’ enables anti-aliasing making the text smoother. The next tuple (0, 0, 0) is the RGB color of the text (in this case, black), and the last argument (255, 255, 255) is the background color of the text (in this case, white).
The final step is to blit the surface onto the screen. You can control the coordinates of where to display this text. For example:
screen.blit(text, (250, 250))
Changing Text Color and Background
Colors in Pygame are expressed as RGB tuples. We can easily change the color of the text and the background by modifying these values:
text = font.render('Hello World', True, (255, 0, 0), (0, 125, 125))
In the code above, the text color is changed to red and background color to turquoise. You can experiment with different color combinations to get the best visual impact for your game.
Implementing Text without Background
If you want text without any background (so that it can blend with the game background), you can do so by not specifying the background color in the ‘render()’ method:
font = pygame.font.Font('freesansbold.ttf', 32) text = font.render('Hello World', True, (255, 255, 255)) screen.blit(text, (250, 250))
In the above example, we’re rendering the ‘Hello World’ text in white without any specific background. As a result, the background takes the color of the screen where we blit the text.
Just like this, you can create dynamic, interesting and engaging text effects in your Pygame projects, making them more interactive and fun.
Animating Text
Animating text, such as making it move across the screen, can add excitement and make your game more dynamic. Let’s create an example:
font = pygame.font.Font('freesansbold.ttf', 32) text = font.render('Hello World', True, (255, 255, 255)) x = 0 y = 250 while True: screen.fill((0, 0, 0)) screen.blit(text, (x, y)) pygame.display.update() x += 1
In this example, the ‘Hello World’ text moves towards the right. Inside the while loop, we continually update the x-coordinate causing the text to move. Each iteration of the loop moves the text one pixel to the right, creating a smooth animation.
Updating Text
Another common requirement in games is to update text dynamically, such as a score that updates whenever a player earns points. Here’s how you can do this:
font = pygame.font.Font('freesansbold.ttf', 32) score = 0 while True: score_text = font.render(f'Score: {score}', True, (255, 255, 255)) screen.fill((0, 0, 0)) screen.blit(score_text, (10, 10)) pygame.display.update() score += 1
In this example, we create a score that is continuously incrementing while we keep updating the score text on the screen.
Different Fonts and Sizes
Different aspects of the game might require different fonts and sizes. Pygame allows us to easily implement this:
font1 = pygame.font.Font('freesansbold.ttf', 32) font2 = pygame.font.Font('comic-sans.ttf', 48) text1 = font1.render('Hello', True, (255, 255, 255)) text2 = font2.render('World', True, (255, 255, 255)) screen.blit(text1, (100, 100)) screen.blit(text2, (100, 200))
In this example, the first text ‘Hello’ appears in font size 32, while the second, ‘World’, appears in a larger font size, 48.
Multiline Text
What if you want to display paragraphs or multilines of text? You can accomplish that by splitting the text into lines and then rendering each line separately:
font = pygame.font.Font('freesansbold.ttf', 32) paragraph = 'Hello\nWorld\nThis is Pygame' lines = paragraph.split('\n') for i, line in enumerate(lines): text = font.render(line, True, (255, 255, 255)) screen.blit(text, (50, 50 + 40 * i))
Here, we use ‘\n’ to denote a new line and split the text accordingly. We then blit each line at a different y-coordinate, effectively creating multiline text.
With these examples and explanations, we aim to provide a comprehensive guide to help you master the use of Text in Pygame, thus making your games more vibrant, interactive and captivating. Keep practicing, and happy coding!
Adding Shadows to Text
Adding a shadow behind your text can make it easier to read, and also gives it a slick, polished look. Let’s create an example:
font = pygame.font.Font('freesansbold.ttf', 32) text = font.render('Hello World', True, (255, 255, 255)) shadow = font.render('Hello World', True, (0, 0, 0)) screen.blit(shadow, (252, 252)) screen.blit(text, (250, 250))
Here, we are rendering the “Hello World” text twice. The shadow version is displayed two pixels down and to the right of the text. This will make the ‘white’ text appear to have a ‘black’ shadow.
Rotating Text
Rotation is a common theme in animations within game design. You can rotate text by using the ‘pygame.transform.rotate()’ function:
font = pygame.font.Font('freesansbold.ttf', 32) text = font.render('Hello World', True, (255, 255, 255)) rotated_text = pygame.transform.rotate(text, 45) screen.blit(rotated_text, (250, 250))
In the above example, our text will be displayed, rotated 45 degrees.
Scaling Text
Pygame also allows you to scale surfaces, which is helpful when you want to animate text growing or shrinking:
font = pygame.font.Font('freesansbold.ttf', 32) text = font.render('Hello World', True, (255, 255, 255)) for i in range(100): scaled_text = pygame.transform.scale(text, (i*5, i*5)) screen.blit(scaled_text, (250, 250))
In this example, we’ve made the text ‘Hello World’ progressively increase in size, giving a zooming-in effect.
Rendering Text with Alpha Transparency
Alpha transparency is useful when you want the text to blend with the game background. Pygame supports this feature, allowing developers to create text with different levels of opacity:
font = pygame.font.Font('freesansbold.ttf', 32) text = font.render('Hello World', True, (255, 255, 255)) for i in range(256): text.set_alpha(i) screen.blit(text, (250, 250))
Here, we progressively increase the text’s alpha value from 0 (completely transparent) to 255 (completely opaque).
Displaying Non-English Text
To display text in other languages, we’ll use unicode strings. Ensure that the chosen font supports the unicode characters you intend to use. For this example, we’ll write some text in Japanese:
font = pygame.font.Font('freesansbold.ttf', 32) text = font.render(u'こんにちは世界', True, (255, 255, 255)) screen.blit(text, (250, 250))
If you run the code, you’ll see “こんにちは世界” being rendered, which means “Hello World” in Japanese.
Through these examples, you should now have a solid understanding of the wide array of textual manipulations available in Pygame. From basic text display, to animations and multilingual expressions, Pygame Text provides the developer with a powerful toolset for enhancing player communication and in-game experience.
Where to go next?
Now that you’ve got a taste of what Pygame Text has to offer, the question is: where to go next? While there’s always more to learn and explore with Pygame, you also might want to expand your Python horizons further. Through consistent practice and relentless learning, true mastery of any skill is attainable.
We, at Zenva, provide an array of programming courses suitable for beginners and professionals alike. To continue your Python journey, we encourage you to explore our Python Mini-Degree. This comprehensive compilation of Python courses covers a broad range of topics, from basic coding concepts to advanced game development and app creation. The curriculum is designed to let you learn at your own pace, while creating real-world projects to form a robust programming portfolio.
For an even broader selection of Python courses, feel free to peruse our Python courses selection. At Zenva, our mission is to provide high-quality education that’s accessible and efficient, helping you achieve your career goals, start your business, or simply follow your passion. Happy learning!
Conclusion
Congratulations on your first steps into the vibrant world of Pygame Text! Text plays an instrumental role in any game, narrating the story, communicating information, and enhancing player engagement. As you continue to explore and experiment, you’ll find more creative ways to incorporate and manipulate text, adding depth and polish to your games.
Continue your journey with our Python Mini-Degree. Remember, the key to mastering any skill is consistent practice and commitment. And with Zenva, you’re not alone. Our expertly crafted courses are here to guide you, every step of the way. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
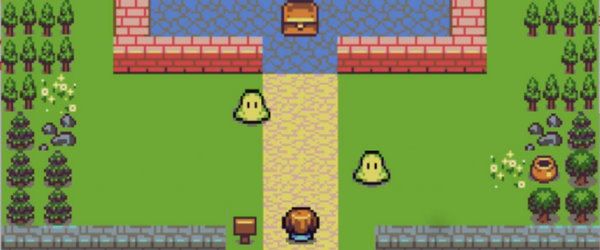
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.