Welcome, coding learners! In this comprehensive article, we are exploring the wonderful world of Pygame drawing. Everything you need to get started is right here, from understanding the basics to creating engaging graphics using code.
Table of contents
What is Pygame Draw?
Pygame draw is a module within Python’s Pygame library especially designed to make 2D games. It includes a set of functions that help you draw shapes like lines, rectangles, and circles directly onto your game window.
Why Use Pygame Draw?
The Pygame draw module offers an easy way to integrate graphics into your game, promoting fun and engaging gameplay. With a few commands, you can create visuals that elevate the gaming experience, from simple objects to complex game elements.
Should I Learn Pygame Draw?
Absolutely! Grasping Pygame draw allows you to add another layer of complexity and entertainment to your game projects. It’s beginner-friendly, interactive, and most importantly, a lot of fun! Learning this tool will not only sharpen your Python skills, but it will also open up a whole new spectrum of possibilities in game design.
Stay tuned as we delve deeper into this exciting module with some coding examples in our next sections!
The Basics of Pygame Draw
Before getting started, you need to install the Pygame library if you haven’t already. Run this command in your terminal to install Pygame:
pip install pygame
Let’s begin by setting up a basic Pygame window:
import pygame pygame.init() win = pygame.display.set_mode((800,600)) pygame.display.set_caption("Pygame Draw Tutorial")
This code initiates a window of 800 pixels width and 600 pixels height with a title “Pygame Draw Tutorial.
Drawing a Line with Pygame Draw
You can use the pygame.draw.line() function to draw a simple line:
color = (255, 0, 0) #color in RGB format start_pos = (0,0) end_pos = (200,200) pygame.draw.line(win, color, start_pos, end_pos) pygame.display.update()
This code will draw a red line from the point (0,0) to the point (200,200) on the window.
Drawing a Rectangle/Square with pygame.draw.rect()
color = (0, 255, 0) #Green color in RGB format top_left_corner = (30,30) width_height = (100,100) pygame.draw.rect(win, color, pygame.Rect(top_left_corner, width_height)) pygame.display.update()
Now we have a green rectangle with its top left corner at the position (30,30) with width and height of 100 pixels each.
Drawing a Circle with Pygame Draw
The pygame.draw.circle() function helps us draw a circle:
color = (0, 0, 255) #Blue color in RGB format center = (400,300) radius = 50 pygame.draw.circle(win, color, center, radius) pygame.display.update()
This will create a blue-colored circle, which is centered at (400,300), with a radius of 50 pixels.
Finally, let’s remember to close the window appropriately when the game ends:
run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False pygame.quit()
These are just some of the basic shapes you can draw with Pygame. Remember to place pygame.display.update() whenever you make changes into the window. Let’s continue creating more complex figures in the next section.
Creating More Complex Shapes with Pygame Draw
Taking our understanding of Pygame Draw further, we can create much more complex shapes and visuals.
Drawing an Ellipse
To draw an ellipse, we use the pygame.draw.ellipse() function. Remember, the ellipse will be drawn inside the rectangle defined by the parameters, so make sure to put suitable values.
color = (0, 255, 255) #Cyan color in RGB format top_left = (500,100) width_height = (200,100) pygame.draw.ellipse(win, color, pygame.Rect(top_left, width_height)) pygame.display.update()
The above code will draw a cyan-colored ellipse inside the defined rectangle.
Drawing a Polygon
Pygame draw also enables us to draw multi-sided shapes or polygons. For that, we use pygame.draw.polygon() function.
color = (255, 255, 0) #Yellow color in RGB format points = [(100,300), (200,200), (300,300), (200,400)] pygame.draw.polygon(win, color, points) pygame.display.update()
This code draws a yellow diamond by connecting the points specified in a clockwise order.
Drawing Arcs
Drawing arcs is also incredibly straightforward with the pygame.draw.arc() function.
color = (255, 0, 255) #Purple color in RGB format top_left = (400,400) width_height = (100,200) start_angle = 0 end_angle = 3.14159 #Pi radians pygame.draw.arc(win, color, pygame.Rect(top_left, width_height), start_angle, end_angle) pygame.display.update()
This code draws a semi-circle (since we’ve provided half of 2π as the angle) with a purple color.
Changing Line Thickness
Extra parameters in pygame draw functions can adjust line thickness. Here’s an example:
color = (255, 255, 255) #White color in RGB format start = (400,0) end = (400,600) thickness = 10 pygame.draw.line(win, color, start, end, thickness) pygame.display.update()
This code creates a thick white line across the screen.
As you can see, the possibilities with Pygame Draw are brilliant and near endless. Just with these simple functions, you can create various shapes and objects to enhance your game. Continue experimenting and exploring, and see what amazing things you can create with Pygame!
Exploring More about Pygame Draw
Let’s discover more about the various graphics and styles we can create using Pygame Draw.
Drawing with a Gradient
Pygame does not directly support gradient fills. However, we can achieve the gradient effect with a series of very thin, coloured lines changing progressively.
for i in range(800): pygame.draw.line(win, (0, i//3, i//2), (i, 0), (i, 600)) pygame.display.update()
This block of code creates a gradient from top to bottom.
Drawing Random Pixels
You can also use Pygame Draw for creating randomly colored pixels on the window:
import random colors = [(255,0,0), (0,255,0), (0,0,255), (255,255,0), (255,0,255), (0,255,255)] for _ in range(5000): x = random.randint(0, 799) y = random.randint(0, 599) color = random.choice(colors) win.set_at((x, y), color) pygame.display.update()
This code will randomize 5000 colored pixels on the window.
Displaying Text
While not part of the pygame.draw module, Pygame’s font module allows us to render text onto our display. Here’s how to display some text:
font = pygame.font.SysFont('arial', 30) text = font.render('Hello, Pygame!', True, (255,255,255), (0,0,0)) win.blit(text, (50, 50)) pygame.display.update()
This draws ‘Hello, Pygame!’ on the window in Arial, size 30, in white color, over a black background.
Animating Objects
With Pygame Draw, you can breathe life into your drawings via animation:
color = (255, 255, 255) x = 0 run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False win.fill((0, 0, 0)) pygame.draw.rect(win, color, pygame.Rect(x, 300, 50, 50)) x += 5 if x > 750: x = 0 pygame.display.update() pygame.quit()
This code creates a moving rectangle, that teleports back to the beginning once it reaches the end of the screen.
With each new concept you learn, you’re becoming better at using Pygame. Continue exploring its vast potential to create intriguing and interactive visuals in your game projects. Happy coding!
Where to Go Next?
The world of Pygame drawing is a broad one, offering endless creativity and opportunities. You can bring to life intricate game designs, animate your drawings, and make your programming journey exciting and enjoyable. But don’t stop here!
At Zenva, we are committed to empowering you with the skills and knowledge to become a versatile programmer, and that includes strengthening your Python skills extensively. Jump start your Python journey with our Python Mini-Degree, a comprehensive series of courses that shed light on Python programming at varying levels of difficulty. This collection equips you not only with Python’s fundamentals but also its illustrious roles in data science, game development, and more.
For a broader collection, explore our catalog of Python courses. Zenva’s interactive, self-paced learning resources are designed to boost your career regardless of where you stand in your coding journey. We provide over 250 courses spanning game creation, machine learning, programming, and much more to help you reach your full potential. Let’s continue learning, coding, and improving together!
In the journey of coding and game development, the only limit is the sky. Let’s reach for it together with Zenva.
Conclusion
Congratulations! You’ve now taken a step deeper into the world of game development and coding with Python using Pygame. Bringing your visual ideas to life in a game truly opens the gates for creative exploration.
Remember, every new concept you master continues to add to your strength as a developer. And we at Zenva are always here to fuel that journey. Be sure to continue your learning path with us and check out our Python Mini-Degree. Cherish this amazing journey of creating, coding, and exploring, and let’s continue reaching for the stars together. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
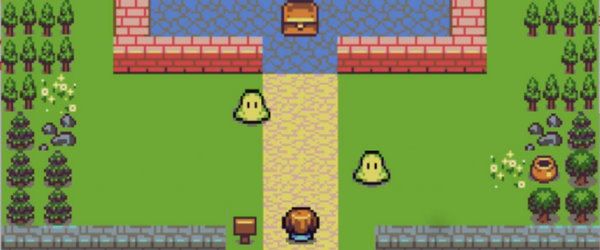
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.