Today we step into the fascinating world of Lua coroutines. In this comprehensive tutorial, we’ll demystify this topic, exploring its applications, and showcasing its usefulness within the coding journey. We invite you to indulge your curiosity, add another notch to your coder’s belt, and discover how Lua coroutines can add efficiency and flexibility to your programming endeavors.
Table of contents
What is Lua Coroutine?
Lua Coroutine is a feature within the Lua programming language designed to manage the flow of a program. Rather than running a program from start to finish without interruption, coroutines allow a running code block to be paused and resumed, providing an incredible advantage when dealing with various tasks simultaneously.
Why Learn Lua Coroutine?
Lua Coroutine provides a vital benefit – the ability to multitask within a program. By using coroutines:
- One can interchangeably alternate execution between different tasks in a program.
- Complex operations can be simplified, as we can structure them into approachable sequences.
Thus, adding Lua Coroutine to your skill set will indeed enrich your coding prowess, enabling you to write more structured, efficient, and clean code.
Let’s dive in and start experimenting with Lua coroutines, unraveling their potential and usage.
Creating Coroutines in Lua
Creating a coroutine in Lua is done by calling the coroutine.create() function, which takes in a function as its argument. Let’s look at an example code snippet to understand this:
mycoroutine = coroutine.create(function () print("Hello!") end) coroutine.resume(mycoroutine)
Upon running this code, it simply prints out “Hello!”. Let’s move on and explore more about coroutine status and coroutine yield.
Understanding Coroutine Status and Coroutine Yield
Coroutine status in Lua is used to determine the status of a coroutine. It can be dead (if the coroutine has finished its execution or has not started at all), running (if it is currently running), or suspended (if it is currently suspended).
Here is an example demonstrating coroutine status:
mycoroutine = coroutine.create(function () print("Hello!") end) print(coroutine.status(mycoroutine)) -- This will print "suspended" as the coroutine is yet to be executed. coroutine.resume(mycoroutine) -- The coroutine is now resumed. print(coroutine.status(mycoroutine)) -- This will print "dead" as the coroutine has completed its execution.
Coroutine yield is a feature that allows the suspension of a coroutine from its running state. Let’s see it in action in this code snippet:
mycoroutine = coroutine.create(function () for i=1,10 do print(i) coroutine.yield() end end) for i=1,10 do coroutine.resume(mycoroutine) -- This resumes the coroutine each time, allowing the print statement to be executed. end
This script would print values from 1 to 10, with each value being printed when the coroutine is resumed.
Resuming Coroutines with Parameters
Coroutines can also accept parameters. Resuming a coroutine with a parameter entails passing a parameter to the function within the coroutine. Here’s an example:
mycoroutine = coroutine.create(function (x,y) print(x+y) end) coroutine.resume(mycoroutine,10,20) -- This will print "30" as the coroutine function adds the parameters.
This script will print “30”, the sum of the two parameters passed to the coroutine function when it’s resumed.
Cooperative Multitasking with Lua Coroutines
The true power of Lua coroutines comes out when they are used for cooperative multitasking. This allows multiple coroutines to manage their schedules themselves, yielding control in a collaborative routine. Lua doesn’t preemptively take control, rather the coroutines voluntarily relinquish it using ‘coroutine.yield()’.
Let’s consider an example of simulating multiple computational tasks with coroutines:
task1 = coroutine.create(function () for i=1,5 do print("Task 1 - processing "..i) coroutine.yield() end end) task2 = coroutine.create(function () for i=1,5 do print("Task 2 processing "..i) coroutine.yield() end end) for i=1,5 do coroutine.resume(task1) coroutine.resume(task2) end
Here, task1 and task2 are simulated as separate computational tasks. They are run in an alternating manner, demonstrating cooperative multitasking.
Handling Coroutine Errors
Lua also allows us to handle errors that occur within coroutines. This can be done by using the ‘pcall()’ or ‘xpcall()’ Lua functions. Below is a simple example:
mycoroutine = coroutine.create(function () assert(nil, 'This is an error') end) status, err = coroutine.resume(mycoroutine) if not status then print('Caught an error: ',err) end
Running this script will display ‘Caught an error: This is an error’. The error within the coroutine is caught and printed out.
Coroutine Utilities
Lua provides several utility functions offered in the coroutine library. A few crucial ones include coroutine.running() and coroutine.wrap().
The coroutine.running() function returns the running coroutine and a boolean which signifies whether the coroutine is the main one:
co = coroutine.create(function () print(coroutine.running()) end) coroutine.resume(co)
The coroutine.wrap() function is similar to coroutine.create(), but returns a function instead. Calling this function resumes the coroutine:
co = coroutine.wrap(function () print("Hello from coroutine") end) co()
Running this script will print “Hello from coroutine”. Once the coroutine created using coroutine.wrap() terminates, the subsequent calls to this coroutine function will not restart it.
Lua coroutines provide powerful tools for managing and simplifying complex asynchronous tasks. They are an indispensable tool for any Lua developer. Happy coding!
Coroutine Yield And Resume With Parameters: A Deep Dive
Lua coroutines live up to their true potential when we blend the power of coroutine.yield() and coroutine.resume(). While coroutine.yield() suspends the coroutine, it also sends back values to coroutine.resume(), which further supplies values back to coroutine.yield().
Let’s discern this concept with a simple illustrative script:
mycoroutine = coroutine.create(function (x) print("Coroutine received:", x) print("Coroutine returning: ", coroutine.yield(x+10)) -- Add 10 to x and yield. end) status, value = coroutine.resume(mycoroutine, 20) -- The coroutine receives a parameter. -- The status now indicates whether the coroutine is successfully resumed, and value carries the yielded value. print("Resume returned:", value) status, value = coroutine.resume(mycoroutine, value*5) -- Resuming again with a new parameter. print("Resume returned:",value)
This script will first print ‘Coroutine received: 20’. Then, when we yield, it generates ‘Coroutine yielding: 30’ and subsequently ‘Resume returned: 30’. Finally, it resumes and returns nil.
Coroutine.running() and coroutine.isyieldable()
There’s more to coroutines! Lua provides useful utilities: coroutine.running() and coroutine.isyieldable().
– coroutine.isyieldable() returns true if the running coroutine can yield.
Here are a couple of examples:
mycoroutine = coroutine.create(function () print("Coroutine running?", coroutine.running()) print("Coroutine yieldable?", coroutine.isyieldable()) end) coroutine.resume(mycoroutine) print("Out of coroutine. Coroutine running?", coroutine.running())
This script prints ‘Coroutine running? true’ and ‘Coroutine yieldable? true’ when within the coroutine. When outside the coroutine context, it prints ‘Coroutine running? false’.
Coroutine.wrap() with Parameters
Coroutine.wrap() can also accept parameters in the same way as coroutine.resume(). The parameters are passed to the coroutine function.
mywrap = coroutine.wrap(function(x,y) print(x+y) end) mywrap(10,20) -- This will print "30" as the coroutine function adds the parameters.
This script readily sums the parameters and prints “30”, effectively demonstrating coroutine.wrap() with parameters.
Coroutine with Error Handling
Taking a leap further, let’s handle errors occurring within the co-routines using ‘xpcall()’ :
mycoroutine = coroutine.create(function () local function err_handler(err) print("Caught an error: ", err) end xpcall(function () assert(nil, "This is another error") end, err_handler) end) coroutine.resume(mycoroutine)
Here, the error is caught within the coroutine itself using an error handler function and ‘xpcall()’.
Overall, with these examples, we’re confident you’ve earned substantial grasp over Lua coroutines. Remain curious, keep experimenting, and may the code be with you!
Continue Your Learning Journey With Zenva
After this deep dive into Lua coroutines, you might be wondering what’s next on your learning journey. At Zenva, we offer a wide range of courses to expand your knowledge and skills even further. A great next step could be our Roblox Game Development Mini-Degree, where the principles you learned here are an integral part of programming games.
Our Roblox Mini-Degree is a comprehensive collection of project-based courses that cover game creation with Roblox Studio and Lua across various genres. Not only do you learn about game designing in different genres like obstacle courses and FPS games, but also delve deeper into multiplayer features, leaderboards, and diverse ways of monetizing your game creations. This Mini-Degree equips you with valuable skills applicable to the wider game development industry, which is always on the lookout for skilled developers.
For learners interested in a broader exploration, we recommend checking out our Roblox collection. With Zenva, you can efficiently navigate from beginner to professional stages of your learning journey. We take great pride in keeping our courses regularly updated, ensuring that your learning aligns with the latest industry trends. So what are you waiting for? Let’s keep learning!
Conclusion
In this tutorial, we’ve journeyed through the intriguing world of Lua coroutines. They’re magnificent tools that can introduce immense flexibility and efficiency into your code. We trust that this knowledge will fortify your coding skills and prove effective in your future projects, gaming or otherwise.
Now that you’ve got Lua coroutines under your belt, we invite you to take your skills to the next level with Zenva’s Roblox Game Development Mini-Degree. Make your games come alive with Lua scripting and create fantastic experiences for game enthusiasts! Keep exploring, keep learning, and remember – the code is your canvas.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
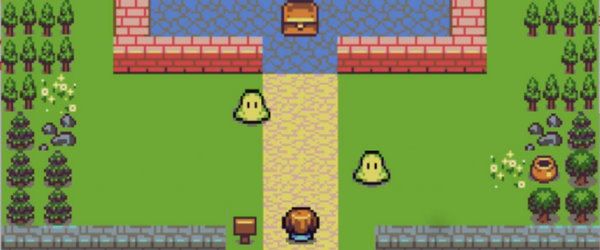
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.