Welcome to our tutorial on Lua operators. As game developers or simply enthusiasts, understanding the role of operators in a coding language is essential for creating effective and efficient scripts. Today, we’re diving deep into Lua operators, an integral part of this popular scripting language used in games like Roblox.
Table of contents
What are Lua Operators?
Operators in Lua help perform operations on variables and values. Quite like mathematical operators, but in the context of game scripting, these operators can manipulate numerical, string, and boolean data to determine game behavior.
Importance of Learning Lua Operators
Why learn Lua operators, you may ask? Firstly, Lua is not just an ordinary coding language. It’s a scripting language widely used in game development, especially in Roblox. Having a solid understanding of Lua operators aids in writing efficient game logic and interactive scripts.
Secondly, grasping Lua operators arms you with the ability to make your game elements interact with each other. Whether it’s a trigger that starts a race when a player steps on it or a countdown timer for a bomb explosion, Lua operators play a central role in executing these tasks effectively.
Join us as we unravel the world of Lua operators, piece by piece, in an engaging and beginner-friendly way. Our aim is to simplify complex concepts so that regardless of your coding experience, you’ll be able to incorporate these operators into your game projects proficiently. Let’s dive in!
Basic Lua Operators
First, we’re going to tackle the most basic yet fundamental operators that Lua has to offer – Arithmetic Operators. With these, we can manipulate number values to perform standard mathematical operations:
local num1 = 10 local num2 = 5 -- Addition local sum = num1 + num2 -- This will give us the sum: 15 -- Subtraction local difference = num1 - num2 -- This will give us the difference: 5 -- Multiplication local product = num1 * num2 -- This will give us the product: 50 -- Division local quotient = num1 / num2 -- This will give us the quotient: 2
Relational Operators
Relational Operators allow us to compare two values. Let’s look at some examples:
local num1 = 10 local num2 = 5 -- Equal local isEqual = num1 == num2 -- This will give us false, as 10 is not equal to 5. -- Not equal local isNotEqual = num1 ~= num2 -- This will give us true, as 10 is not equal to 5. -- Greater than local isGreater = num1 > num2 -- This will give us true, as 10 is greater than 5. -- Less than local isLess = num1 < num2 -- This will give us false, as 10 is not less than 5.
Logical Operators
Logical operators in Lua follow the principles of Boolean Algebra which only has two possible results: true or false. Let’s demonstrate each of them:
local bool1 = true local bool2 = false -- And local andResult = bool1 and bool2 -- This will give us false, as both values are not true. -- Or local orResult = bool1 or bool2 -- This will give us true, as one of the values is true. -- Not local notResult = not bool1 -- This will give us false, as it reverses the original boolean value.
In the next section, we will delve deeper and learn how to maximize the usage of these basic Lua operators in games design!
Working with Assignment Operators
Assignment operators are used to assign values to variables. However, Lua also offers shorthand coding with combined assignment and arithmetic operators. We’ll get to those in a minute.
First, let’s look at a basic assignment:
local num1 = 10 -- Here, '=' is used to assign the value 10 to the variable num1
Now, let’s dive into shorthand assignments:
local num1 = 10 num1 += 5 -- Equivalent to num1 = num1 + 5 print(num1) -- This will print 15 num1 -= 2 -- Equivalent to num1 = num1 - 2 print(num1) -- This will print 13 num1 *= 3 -- Equivalent to num1 = num1 * 3 print(num1) -- This will print 39 num1 /= 3 -- Equivalent to num1 = num1 / 3 print(num1) -- This will print 13
This makes coding more streamlined without compromising the readability of the code.
Table Manipulation with Lua Operators
Tables in Lua can be manipulated through a set of operators. By understanding these, you can efficiently store and manipulate data in your games.
Let’s start by creating a table:
local myTable = {"Apple", "Banana", "Cherry"} -- Creates a table with three elements
We can manipulate this table in different ways:
-- Accessing table elements print(myTable[1]) -- This would print "Apple" -- Modifying a value in the table myTable[1] = "Avocado" print(myTable[1]) -- This would now print "Avocado" -- Adding new elements to the table table.insert(myTable, "Dragonfruit") print(myTable[4]) -- This would print "Dragonfruit"
By now you’re getting the hang of this, right? At Zenva, we believe that by making these small steps on your journey, you can become a proficient game developer from anywhere in the world!
In the next segment, we will examine how Lua operators can be applied in creating engaging gameplay scenarios, pushing the boundaries of your coding skills in a fun and interactive way. Stay tuned!
Using Lua Operators in Game Scenarios
Let’s continue our journey by exploring how these Lua operators can be applied to craft captivating game scenarios.
Calculating Game Scores
Imagine you’re developing a game where players earn points by accomplishing tasks.
local gameScore = 0 -- We start with a score of 0. gameScore += 100 -- Player completes a task, so we add 100 points. gameScore += 50 -- Player completes another task; we add 50 more points.
In this scenario, our assignment operator increases the game score by a certain number of points each time a task is completed.
Implementing Game Conditions
Next, suppose we want to show a “Game Over” message whenever the player’s health drops to zero. Here, relational operators come into play:
local playerHealth = 50 -- Player starts with a health of 50. playerHealth -= 50 -- Player gets hit and loses 50 health. -- The 'Game Over' condition. if playerHealth <= 0 then print("Game Over") end
In this case, the script checks if the player’s health is less than or equal to zero. If the condition is met, a “Game Over” message is displayed.
Managing Game Settings
Now, let’s say our game has a “Night Mode”. We need to let players switch it on or off. In this case, Logical Operators provide a method for toggling conditions:
local nightMode = false -- By default, Night Mode is switched off. -- Switching Night Mode on. nightMode = not nightMode print(nightMode) -- This would print "true" -- Switching Night Mode off again. nightMode = not nightMode print(nightMode) -- This would print "false"
With the help of the ‘not’ logical operator, we can switch the boolean value for “Night Mode” from false to true and vice versa.
Storing Inventory Items
Our game also needs an inventory system to store collected items. Here, we can use Lua tables with operators to manage this:
local inventory = {} -- We begin with an empty inventory. -- Adding items. table.insert(inventory, "Sword") table.insert(inventory, "Shield") table.insert(inventory, "Health Potion") -- Checking the first item in the inventory. print(inventory[1]) -- This would print "Sword" -- Changing the second item. inventory[2] = "Magic Shield" print(inventory[2]) -- This would now print "Magic Shield"
By understanding Lua operators, you’ve just created a flexible inventory system for a game! We’ve seen how Lua Operators can make game logic more engaging and interactive, and that’s just scratching the surface. We encourage you to experiment and explore, pushing your new skills to the limit, and always remember at Zenva, we’re here to guide you through every step of your coding journey!
Where to go next?
Having navigated through this comprehensive tutorial on Lua operators, you’re now well equipped with a foundational understanding of scripting in Lua. Now it’s time to take this forward and fully unlock your potential as a game developer, integrating this newfound knowledge into your projects. But you might be wondering – what’s the best way to proceed?
At Zenva, we provide a comprehensive path for learners who want to step up their game development skills and venture deeper into the fascinating world of game coding, creation, and design. A worthy venture you could embark on is our Roblox Game Development Mini-Degree. A complete collection of courses focused on creating various game genres in Roblox Studio using Lua, this Mini-Degree caters to both beginners who’ve recently embarked on their game development journey and experienced developers who want to polish their skills.
And, for those keen to explore all things Roblox, don’t miss out on our broader collection of Roblox courses. With over 250 programming courses available, Zenva covers everything from the basics to advanced topics, allowing you to journey from novice to professional at your own pace.
We look forward to seeing you delve deeper into game development and scripting. Happy coding!
Conclusion
Explorer, you’ve taken a big leap in understanding Lua operators today! This fundamental knowledge about an integral part of Lua coding opens up new possibilities for you to create unique game interactions and elements. Not to mention the technical prowess you gain, putting you ahead on your game development quest.
Don’t let the zeal stop here, though! Take these concepts ahead and deepen your learning with our Roblox Game Development Mini-Degree. Versatile and comprehensive, this program will add untold value on your path as a professional game developer. Take the plunge, and give wings to your coding creativity! We at Zenva are excited to be a part of your game development journey. Happy coding, adventurer!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
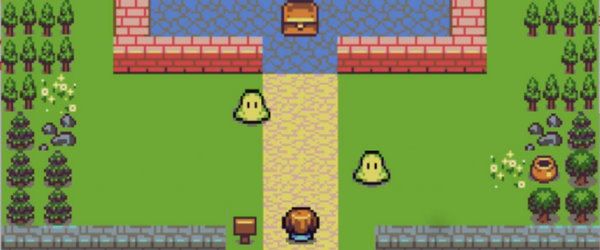
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.