Welcome to an integral part of your game development journey – learning C#. This powerful yet friendly language is the backbone of many popular games and applications. If you’ve been seeking an engaging and comprehensive guide to kickstart your coding adventure in C#, you’ve come to the right place!
Table of contents
What is C#?
C# is a simple, modern, and object-oriented programming language. Developed by Microsoft, it is widely favored for its expressiveness and ease of use, making it hugely popular among beginners and seasoned programmers alike.
What is C# for?
C# is a versatile language that has various applications, including building Windows applications, creating websites with ASP.NET, and, most importantly for us game enthusiasts, developing games using the Unity engine.
Why should you learn C#?
Learning C# opens up a myriad of opportunities. For one, with knowledge of C#, you can harness the full power of Unity, which is a leading game development platform. Additionally, C# skills are highly sought after in the job market, tipping the scales in your favor when seeking tech roles.
Getting Started with C# – Basics
As we begin our journey, the simple yet essential part is understanding the syntax and the core concepts of C#. We’ll kick off with the basic constructs such as variables, data types, operators, and control structures.
Variables and Data Types
In C#, variables are utilized to store data temporarily in our program. When declaring a variable, you must specify its type. Let’s look at some code examples.
// Declaring an integer variable int myNumber = 10; // Declaring a string variable string myString = "Hello, Zenva learners!";
C# comes with various built-in types like int (integer), float (floating-point number), bool (boolean), and string (text).
Working with Operators
Operators are handy symbols that perform certain operations on one or more values (operands). The three main types of operators in C# are arithmetic, comparison, and logical operators.
// Arithmetic operator int sum = 5 + 10; // results in 15 // Comparison operator bool result = 5 > 10; // results in false // Logical operator bool combinedResult = (5 3); // results in true
Control Structures
In any programming language, control structures are essential for controlling the flow of the program. C# provides several control structures such as “if” statements, “switch” statements, and loops like “for” or “while”.
// if statement if (myNumber > 5) { Console.WriteLine("The number is greater than 5."); } // for loop for (int i = 0; i < 5; i++) { Console.WriteLine(i); }
Through these examples, we’re slowly shaping up our understanding of the C# language. This programming journey may seem challenging, but at Zenva, we are dedicated to making it a rewarding and thrilling ride!
Working with Arrays and Lists
Ranges of data in C# can be handled through arrays and lists. They help organize data in structure and provide ways to iterate through them.
// Array example int[] numbersArray = new int[5] {1, 2, 3, 4, 5}; // List example List<int> numbersList = new List<int>() {1, 2, 3, 4, 5};
Looping over the elements in arrays or lists can be done using “foreach” loop.
foreach (int number in numbersArray) { Console.WriteLine(number); }
Functions and Methods
Functions, also known as methods in C#, are blocks of code designed to perform a particular task. They can be called by its name. Let’s declare a simple function that adds two numbers.
static int AddNumbers(int a, int b) { return a + b; }
Now, we can call this function and use the result.
int result = AddNumbers(5, 10); Console.WriteLine(result); // Outputs 15
Classes and Objects
As an object-oriented language, C# encapsulates codes within classes, providing a blueprint for creating objects with properties and methods.
public class Dog { public string name; public string breed; public void Bark() { Console.WriteLine(name + " says: Woof Woof!"); } }
Creating an object from a class is simple.
Dog myDog = new Dog(); myDog.name = "Sparky"; myDog.breed = "Golden Retriever"; myDog.Bark(); // Outputs "Sparky says: Woof Woof!"
With these concepts in mind, you’re well on your way to mastering C#. Remember, the key to learning programming is consistency and practice. Here at Zenva, we’re committed to guiding you on each step of your coding journey!
Working with User Inputs
Being able to interact with users via input is a crucial aspect of any application. In C#, we can use the Console.ReadLine() method to get user input as a string. Let’s see it in action:
Console.WriteLine("Enter your name: "); string userName = Console.ReadLine(); Console.WriteLine("Hello " + userName + "!");
Using Complex Control Structures
While we’ve covered the basics of control structures, C# offers more complex tools to handle code flow. Let’s deep dive into some of them.
Do-while loops are similar to while loops, but they check the condition after the loop has run once, ensuring the block of code gets executed at least once.
int x = 5; do { Console.WriteLine(x); x++; } while(x < 10);
The “switch” statement is another control structure that helps validate various conditions and execute code blocks based on those conditions.
int dayOfWeek = 5; switch (dayOfWeek) { case 1: Console.WriteLine("Monday"); break; case 2: Console.WriteLine("Tuesday"); break; // ... And so on until 7 default: Console.WriteLine("Invalid day of the week"); break; }
Classes – Advanced Concepts
Understanding classes often involves grasping some more complex concepts such as constructors, inheritance, and polymorphism.
A constructor is a special method inside a class used to initialize objects. It’s called when an object of that class is created.
public class Dog { public string name; public string breed; // Constructor for Dog public Dog(string name, string breed) { this.name = name; this.breed = breed; } public void Bark() { Console.WriteLine(name + " says: Woof Woof!"); } } Dog myDog = new Dog("Sparky", "Golden Retriever"); // Using the constructor myDog.Bark();
As complex as learning C# might seem, knowing it will extend your toolset and open doors to exciting opportunities. In game development, for instance, understanding C# unlocks the maximum potential of the Unity engine. Zenva believes in your journey as a programmer and will be here to illuminate your path!
Where to Go Next – Continuing Your Learning Journey
Now that you’ve acquired fundamental knowledge of C#, it’s time for the next exciting step in your game development journey – diving into Unity. With C# being the primary language of Unity, your newfound skills will enable you to harness the full potential of this powerful game engine.
At Zenva, we provide a comprehensive Unity Game Development Mini-Degree that offers a collection of courses on building cross-platform games with Unity. This collection covers everything from game mechanics and animation to enemies AI. A leading game engine, Unity is capable of creating 2D, 3D, AR, and VR games. Its use extends beyond gaming, bridging to industries like architecture, film, education, automotive, healthcare, and engineering.
If you wish to explore a broader collection of resources, check out our dedicated Unity courses. Learning with Zenva offers you the flexibility and the tools to journey from beginner to professional, seamlessly and effectively.
Stay curious, keep learning, and remember – the most successful journey starts with a single step. Your first step with Zenva may just be the beginning of your adventure in the world of game development!
Conclusion
In the dynamic realm of programming, languages like C# offer powerful tools to scrape the surface of reality and sculpt imaginative worlds. Your journey through this tutorial has laid the foundation for further exciting adventures in game development, app creation, and beyond.
Remember, mastering this art requires patience, practice, and passion. At Zenva, we are committed to supporting you on every step of this quest, providing high-quality content and interactive platforms. Continue your learning experience with our comprehensive Unity Game Development Mini-Degree, catapulting your C# skills into the engaging and creative world of Unity game development. Let us help you transform from a novice coder to a sorcerer of skill and creativity!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
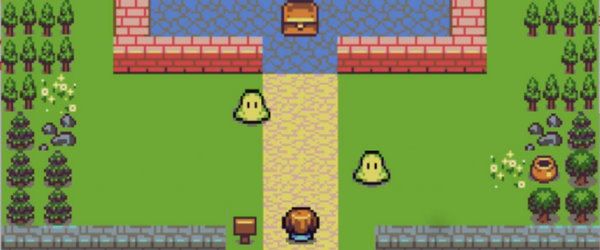
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.