Welcome to our comprehensive guide on Interlocked C#. This article is designed to bring the concept of concurrency and synchronization in code to life for beginners and pros alike. Laced with examples inspired by simple game mechanics, we aim to provide a practical understanding of Interlocked in C#.
Table of contents
What is Interlocked in C#?
The Interlocked class in C# is a powerful feature that aids in writing thread-safe operations. If you haven’t yet explored the world of multi-threading or concurrent computing, don’t worry; the Interlocked class is a good place to start.
Why Should You Learn About Interlocked in C#?
Solving synchronization problems is fundamental when dealing with multi-threaded applications. As you advance in your coding journey, you’ll find more multi-threaded programs, especially in fields like game development and artificial intelligence. The Interlocked class can help you safely perform simple arithmetic and comparison operations even in a multi-threaded environment.
By understanding the Interlocked class, you will master a crucial aspect of multi-threading, which is a valuable skill in today’s concurrent computing world.
Interlocked Basics
Before we dive into code examples, it’s important to understand the basic methods offered by the Interlocked class:
- Increment: This method increases the value of a specified variable by one and stores the result atomically.
- Decrement: Decrement does the opposite, reducing the value of a given variable by one atomically.
- Exchange: This method atomically replaces the value of a specified variable with a new value, and returns the original value.
- CompareExchange: Compares two values for equality and, if they are equal, replaces the first value.
Now let’s look at some examples for better understanding.
Incrementing & Decrementing with Interlocked
Let’s take a look at how we can perform atomic increment and decrement operations with Interlocked. In the following example, we have a shared counter variable among several threads:
// Define a shared variable int counter = 0; // Start multiple threads Parallel.For(0, 1000000, i => { // Increment the counter safely with Interlocked Interlocked.Increment(ref counter); }); Console.WriteLine(counter); // 1000000
In this program, we’re starting a million tasks that all increment the counter. Even though these tasks run concurrently, we can be sure that the counter will reach exactly 1000000 at the end of the program thanks to Interlocked.Increment.
Interlocked.Decrement works in a similar manner. However, please note that if the counter is 0, decrementing it will result in a negative value:
// Define a shared variable int counter = 1000000; // Start multiple threads Parallel.For(0, 1000000, i => { // Decrement the counter Interlocked.Decrement(ref counter); }); Console.WriteLine(counter); // 0
Swapping Variables with Interlocked.Exchange
The Interlocked.Exchange allows us to safely swap the values of two variables across multiple threads. Here’s a simple example:
// Define two shared variables int x = 0; int y = 10; // Swap the values atomically int oldX = Interlocked.Exchange(ref x, y); Console.WriteLine("Old value of X: {0}, New value of X: {1}", oldX, x); // Old value of X: 0, New value of X: 10
In this code snippet, the Interlocked.Exchange method atomically assigns the value of ‘y’ to ‘x’. The old value of ‘x’ is returned and stored in the variable ‘oldX’.
Value Comparisons using Interlocked.CompareExchange
The Interlocked.CompareExchange method compares two values for equality and, if they are equal, replaces the first value. This can be extremely handy for assignment operations that need to be executed conditionally. Here’s an example:
//Define two shared variables int x = 0; int y = 10; //Change the value of 'x' to 'y' only if 'x' is 0 int prevX = Interlocked.CompareExchange(ref x, y, 0); Console.WriteLine("Previous value of X: {0}, New value of X: {1}", prevX, x); //Previous value of X: 0, New value of X: 10
In the above snippet, we only swap the value of x with y if x is equal to 0. This can prevent unwanted assignments in the context of multiple threads.
Just like the other examples shown above, the Interlocked.CompareExchange method ensures atomicity, making it safe to use across all running threads.
Practical Game Example
For a better understanding and context on how to use Interlocked in your games, here’s a simple think-through:
Imagine a racing game where every passing car through a finish line increases a counter. With multiple cars crossing the line at the same time, it’s crucial that the counter addition is thread-safe and does not miss any car. Here’s how the Interlocked class comes into play:
int carCounter = 0; void CarPassesFinishLine() { Interlocked.Increment(ref carCounter); }
Now, even if ‘CarPassesFinishLine()’ is called concurrently by many threads, no car will be missed – every increment operation will be executed in an atomic, thread-safe manner.
Summary
In this comprehensive guide, we discussed the Interlocked class in C#, a critical tool for writing thread-safe operations in multi-threaded applications. It allows us to perform atomic operations and is especially useful in games and AI where concurrency and synchronization matters.
We demonstrated real-life examples, such as incrementing and decrementing counters, swapping variables, and comparing and exchanging variables in a thread-safe way – all thanks to the Interlocked class.
Remember, mastering the Interlocked class can make you a better coder and empower you to create more performant, robust, and interactive games and applications.
Advanced Interlocked Operations
Let’s now delve into some of the more advanced operations that can be achieved with the Interlocked class. These operations allow you to manipulate and control data across multiple threads seamlessly.
Atomic Boolean Toggle
Imagine you have a boolean that needs to be toggled thread-safely. Using Interlocked.CompareExchange(), you can achieve this:
// Define the boolean variable int toggle = 0; // Toggle the boolean atomically Interlocked.CompareExchange(ref toggle, toggle ^ 1, toggle);
This piece of code will swap the value of ‘toggle’ (0 or 1) with the opposite value (1 or 0).
Thread-Safe Double Increment
The Interlocked class in C# doesn’t overlap for the double data type. However, we can simulate an atomic operation for that data type. Here is how you could perform an atomic increment for a double:
// Define the shared double double sharedDouble = 0.0; // Increment the double atomically double toAdd = 1.0; double newValue; do { double old = sharedDouble; newValue = old + toAdd; } while (Interlocked.CompareExchange(ref sharedDouble, newValue, old) != old);
In this example, we used a do…while loop and the Interlocked.CompareExchange() method to increment the shared double atomically.
Thread-Safe Double Decrement
Similarly, you can also decrement a double atomically. Here’s how:
// Define the shared double double sharedDouble = 1.0; // Decrement the double atomically double toSubtract = 0.001; double newValue; do { double old = sharedDouble; newValue = old - toSubtract; } while (Interlocked.CompareExchange(ref sharedDouble, newValue, old) != old);
This is virtually the same as the increment example, but we subtract a value from the shared double instead.
Summary
In this section, we walked through some advanced operations that you can achieve with the Interlocked class. We started with a simple example of an atomic Boolean toggle. We then dove into thread-safe operations for doubles, which require slightly more complex code due to Interlocked lacking direct support for the double type.
With adequate practice and increased familiarity, these operations can soon become second nature to you, saving you countless headaches when dealing with multi-threading in your C# applications and games.
Keep Learning and Growing
Now that you’ve grasped the concept of the Interlocked class in C#, and understood its practical usage in terms of game development, it’s time to expand your knowledge deeper and broader. Game developers need a whole set of skills, and acquiring them can be a fun and fulfilling journey.
We at Zenva offer a range of beginner to professional courses. Learning coding, creating games, understanding artificial intelligence – it’s all here for you to explore. Gain in-depth knowledge, build your strengths, and earn certificates with more than 250 supportive courses we provide. You can go from beginner to professional with us, at your own pace. Both beginners and skilled individuals are welcome to learn.
Consider checking out our Unity Game Development Mini-Degree, an extensive collection of courses covering different aspects of building cross-platform games with Unity, a game engine massively used across the globe. The mini-degree covers various game aspects from mechanics to enemy AIs, animation to cinematic cutscenes, all taught by qualified instructors. These project-based courses let you create a powerful portfolio, adding to your skillset while you learn. For a wider collection, explore our Unity Courses. Seize the opportunity to boost your career in the booming game development industry with us.
Conclusion
In conclusion, the journey to become a skilled game developer may seem daunting, but with the right resources, it can be an enjoyable and enriching experience. Take every step as a new challenge, whether it’s understanding a new concept, like we did today with Interlocked in C#, or creating a game from scratch.
Remember, learning never ends. When you’re ready to take your game development skills to the next level, we at Zenva are always here to assist you. Explore our comprehensive Unity Game Development Mini-Degree and enrich your journey as a game developer. Start your learning adventure with us, and let’s create some amazing games together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
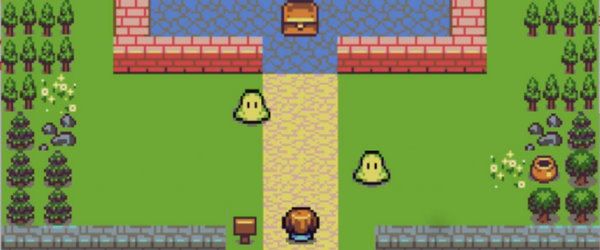
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.