Welcome to this exciting journey into the world of C# programming! If you thought coding was a distant and challenging domain, we’re here to help you navigate through the intricate paths of C# with simple, engaging, and game-focused examples. Let’s unravel the magic of C# together!
Table of contents
What is C#?
C# is a modern, object-oriented programming language developed by Microsoft in the early 2000s. Its versatility and robust features make it a popular choice not just for Windows desktop applications, but also for mobile apps and gaming development.
Why Should You Learn C#?
It’s a question worth asking: why learn C#? Well, here are some compelling reasons:
- Job opportunities: Given its widespread use, there’s a high demand for skilled C# developers across diverse industries.
- Our gaming future: With Unity 3D (one of the world-leading game engines) relying heavily on C#, it’s an essential skill for any aspiring game developer.
- Strong foundational knowledge: Learning C# helps strengthen your understanding of key programming concepts applicable to other languages as well.
What Can You Do with C#?
C# can be wielded for a myriad of applications including building desktop applications, developing mobile apps, creating web applications, and, quite interestingly, designing video games. Thanks to the Unity 3D game engine which uses C# as its primary scripting language, you can bring the most vivid and immersive gaming experiences to life!
Next, we’ll leap straight into the coding sections where we unravel the applications of C# with some fascinating code examples. Let’s get started!
Getting Started with C#
Let’s dive right into the fantastic world of C# programming. We’ll start off with the very basics of C# – writing your very first “Hello, World!” program :
using System; class Program { static void Main() { Console.WriteLine("Hello, World!"); } }
In this C# program, we’re simply printing out “Hello, World!” on the console. The Console.WriteLine() function is inbuilt in C# and is used to output (write) a line of text.
Data Types and Variables
Understanding data types and variables is fundamental to programming in any language. Let’s see how these work in C#:
int age = 25; double height = 5.9; bool isProgrammingAwesome = true; string name = "Zenva";
In this example, we define four variables with different data types – int (integer), double (floating-point number), bool (boolean value), and string (text).
Conditional Statements
Conditional statements in C# allow our programs to make decisions. Let’s look at a simple “if-else” statement:
int a = 10; int b = 20; if (a > b) { Console.WriteLine("a is greater than b"); } else { Console.WriteLine("b is greater than a"); }
In this program, we’re checking if the value of ‘a’ is greater than ‘b’. If it is, the line “a is greater than b” will be outputted. If not, “b is greater than a” gets printed.
Loops in C#
Loops are used to repeatedly execute a block of code until a certain condition is met. A simple “for” loop in C# looks like this:
for (int i = 0; i < 10; i++) { Console.WriteLine(i); }
This loop starts with i equal to 0, and continues to print the value of i as long as i is less than 10, incrementing i by 1 on each iteration.
Working with Arrays
Arrays in C# are used to store multiple elements in a single variable. Let’s see a simple array example:
int[] numbers = { 10, 20, 30, 40, 50 }; foreach (int number in numbers) { Console.WriteLine(number); }
This piece of code defines an array of integers and subsequently prints each integer using a “foreach” loop.
Functions in C#
Functions are reusable pieces of code that perform specific tasks. Let’s define a simple function that adds two numbers:
static int Addnumbers(int a, int b) { return a + b; } static void Main() { int result = Addnumbers(5, 10); Console.WriteLine(result); // Outputs: 15 }
This program includes a function Addnumbers() that takes two integer parameters and returns their sum. In the Main() method, we call this function and print the result.
Along with defining your own functions, C# comes with a variety of inbuilt functions. For example, let’s use the Math.Max() function to find the maximum of two numbers:
int maxNumber = Math.Max(5, 10); Console.WriteLine(maxNumber); // Outputs: 10
In this snippet, we’ve used the Max() function from the Math class to find and print the maximum of two numbers.
Classes and Objects
Firmly rooted in object-oriented programming, C# uses classes and objects for structuring data and code. Let’s create a simple class:
class Vehicle { public string brand; public string color; } class Program { static void Main() { Vehicle myCar = new Vehicle(); myCar.brand = "Audi"; myCar.color = "Black"; Console.WriteLine(myCar.brand); // Outputs: Audi Console.WriteLine(myCar.color); // Outputs: Black } }
In this program, we’ve created a class named ‘Vehicle’ with two properties. We’ve then created an object from this class, set its properties, and printed them on the console.
Exception Handling
Exception handling in C# allows us to handle runtime errors or exceptions, ensuring our applications can recover gracefully from unexpected issues.
try { int[] numbers = {1, 2, 3}; Console.WriteLine(numbers[5]); } catch (Exception e) { Console.WriteLine("An error occurred: " + e.Message); }
This code attempts to access the 6th element of a 3-element array, which throws an exception. The ‘catch’ block handles the exception and prints a custom error message.
With this, we’ve covered some major aspects of C# programming. Remember – consistent practice is key to mastering any programming language, so keep coding!
Working with Strings
Strings are used to store text in C#. We provide a simple example of string manipulation:
string greeting = "Hello from Zenva"; Console.WriteLine(greeting.Length); // Outputs: 15 Console.WriteLine(greeting.ToUpper()); // Outputs: HELLO FROM ZENVA Console.WriteLine(greeting.IndexOf("Zenva")); // Outputs: 10
Here, we use some inbuilt string functions to find the length of the string, convert it to uppercase and find the index of a substring within the string.
Working with Dates
C# offers extensive support to handle dates and times. Here, we demonstrate how you can work with dates:
DateTime currentDate = DateTime.Now; Console.WriteLine(currentDate); // Outputs: The current date and time DateTime specificDate = new DateTime(2022, 2, 28); Console.WriteLine(specificDate); // Outputs: 02/28/2022 00:00:00
This code snippet shows how to get the current date and time and how to create a specific date.
File I/O
C# allows us to interact with files for various operations such as reading from files, writing to files, and more. Here’s an example of writing to and reading from a file:
string filepath = "testFile.txt"; // Write to a file System.IO.File.WriteAllText(filepath, "Hello, Zenva!"); // Read from a file string content = System.IO.File.ReadAllText(filepath); Console.WriteLine(content); // Outputs: Hello, Zenva!
These lines of code create a text file, write a message to it, read the message back, and then print it on the console.
Handling Events
Events in C# are a key part of developing interactive applications such as GUIs and games. A simple example of creating and triggering an event can be as follows:
public delegate void Notify(); // delegate public class ProcessBusinessLogic { public event Notify ProcessCompleted; // event public void StartProcess() { Console.WriteLine("Process Started!"); // some code here.. OnProcessCompleted(); } protected virtual void OnProcessCompleted() { ProcessCompleted?.Invoke(); } } // Use the above class ProcessBusinessLogic bl = new ProcessBusinessLogic(); bl.ProcessCompleted += bl_ProcessCompleted; bl.StartProcess(); public static void bl_ProcessCompleted() { Console.WriteLine("Process Completed!"); }
In this code, we declare a delegate and an event within a class. Elsewhere, we subscribe a method to the event and trigger it.
Working with Databases
C# integrates well with databases such as SQL Server and MySQL. Here’s a simplified example of how you can create and work with a SQL Server database using C# and ADO.NET:
using System.Data.SqlClient; string connectionString = "Data Source=ServerName;Initial Catalog=DatabaseName;User ID=UserName;Password=Password"; SqlConnection conn = new SqlConnection(connectionString); conn.Open(); SqlCommand cmd = new SqlCommand("SELECT * FROM MyTable", conn); SqlDataReader reader = cmd.ExecuteReader(); while (reader.Read()) { Console.WriteLine(reader[0]); } conn.Close();
This snippet establishes a connection with a SQL Server database, executes a SQL query to fetch all data from a table, reads through the results, and then closes the connection.
Remember, trying out lots of different code snippets and creating your own variations is the best way to learn. Happy coding!
Where to Go Next?
Once you have grasped the essential concepts of C#, the fascinating world of game development awaits! Unity, one of the most popular game engines around, uses C# as its primary scripting language, making your newly acquired C# skills a stepping stone into creating your very own games.
We invite you to step into the exciting realm of game creation with our Unity Game Development Mini-Degree. This comprehensive program covers a gamut of game development aspects – from understanding game mechanics to creating intricate enemy AI. The project-based courses are accessible around the clock, allowing you the flexibility to learn at your own pace!
For a broader array of Unity-related courses, feel free to explore our Unity course collection. Whether you are a beginner or a seasoned developer, Zenva will help you take your coding skills to new heights. Remember, with Zenva, you are always on a path to sharpen your skills – from beginner to professional. Keep learning and keep creating!
Conclusion
C# is a feature-rich and versatile language that is widely applied from building web applications to crafting next-gen games. Its elegant syntax and powerful object-oriented principles make it a delightful language for beginners and experienced coders alike. The ubiquitousness of C# across the software development landscape offers promising career pathways and exciting opportunities.
Ready to take the leap and dive deeper into the world of C# and game development? Join us at Zenva with our Unity Game Development Mini-Degree. Unleash your creativity, build your dream games, and become the game creator you always wanted to be. Happy coding, and even happier game creating!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
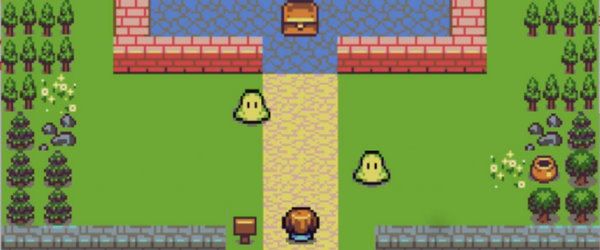
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.