Welcome, budding game developers and code enthusiasts! Today’s journey is an exciting dive into the world of Unity and C#. In this article, we’ll unravel the mystery behind creating games using these incredible tools, exploring the potential they offer and the unique experiences you can create with them.
Table of contents
What is Unity C#?
So, let’s get started with the basics. Unity is a powerful game development platform used to create 3D and 2D games, VR experiences, and simulations. C#, on the other hand, is a popular, versatile programming language that Unity employs to provide us with the ability to script game mechanics, control the game’s flow, and respond to user actions.
Why Should You Learn Unity C#?
Now you may ask, “Why should I learn Unity C#?. Well, here’s why:
- Unity C# is a potent combination that opens the door to the gaming industry.
- Knowing C# will not limit you to Unity. It’s a highly valuable language in a variety of software development fields.
- Programming in Unity using C# enables you to create any feature you dream of, offering full control and flexibility.
Mastery of these tools will not just make you a game developer, but a creator of immersive, interactive experiences. So, let’s roll up our sleeves and start our coding adventure!
Getting Started with Unity C#
Before we dive into coding, ensure you have Unity installed on your computer. If you haven’t, head over to the Unity website and download the Unity Hub to manage and install different versions of Unity. Remember to install an appropriate IDE too, like Visual Studio, which typically comes bundled with the Unity installation.
Creating a New Project
Once your setup is complete, let’s begin by creating a new project:
1. Open Unity Hub. 2. Click on "New". 3. Select a template. For beginners, the "3D" template is a great starting point. 4. Give your project a name and select a location to save it. 5. Click on "Create".
You’re now inside the Unity editor, ready to create your new game.
Creating Your First Script
Now, let’s create our first C# script in Unity:
1. Right-click in the Project panel. 2. Select "Create" > C# Script. 3. Name your script. For our tutorial, let's call it "HelloWorld". 4. Double click on the script to open it in Visual Studio.
Your new script now looks like this:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class HelloWorld : MonoBehaviour { // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { } }
Understanding Basic Unity C# Functions
The two functions in our script, Start() and Update(), are among the most commonly used functions in Unity C#:
- Start() function: The Start() function runs once when the game starts, or when the script is enabled. It’s usually reserved for initial setup, like setting initial variable values.
- Update() function: The Update() function runs once per frame. It’s generally used to update game elements, react to user input, or run anything that needs to be updated frequently.
Here’s an example of how you might use these functions:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class HelloWorld : MonoBehaviour { void Start() { Debug.Log("Hello, World!"); } void Update() { if(Input.GetKeyDown(KeyCode.Space)) { Debug.Log("You pressed the Space key!"); } } }
In this example, our Start() function logs “Hello, World!” to the console when the game begins. In the Update() function, we check if the user has pressed the Space key. If they have, we log “You pressed the Space key!” to the console.
There you have it – we’ve created our first script with basic input detection! In the next section, we’ll build further on this foundation.
Enhancing Game Mechanics with Unity C#
Having explored basic input detection, we can proceed to more advanced topics. Let’s talk about variables and Unity’s Rigidbody system, enhancing our simple Hello, World! game with movement.
Using Variables
Variables in C# allow us to store and manipulate data in our code. Let’s define a speed variable to control how fast our object moves:
public class HelloWorld : MonoBehaviour { public float speed = 10f; void Update() { if (Input.GetKey(KeyCode.W)) { Debug.Log("You pressed the W key!"); transform.Translate(Vector3.up * speed * Time.deltaTime); } } }
In this updated script, we declare a new public float variable speed and set it to 10f. This variable can now be accessed and modified from the Unity editor, helping us adjust our game to the perfect feel.
We also modified the if statement in the Update() function. When the W key is pressed, our object moves upward at the speed set by our variable. We multiply by Time.deltaTime to make the movement smooth and framerate independent.
Working with Unity’s Rigidbody System
Unity’s Rigidbody system takes the complexities of physics and packs them into a simple, powerful system that we can use with just a few lines of code.
First, we must attach a Rigidbody component to our game object in Unity. In Unity’s inspector pane, click “Add Component” and select the Rigidbody component.
Now, let’s tweak our code to use the Rigidbody for movement:
public class HelloWorld : MonoBehaviour { public float speed = 10f; private Rigidbody rb; void Start() { rb = GetComponent<Rigidbody>(); } void Update() { if (Input.GetKey(KeyCode.W)) { Debug.Log("You pressed the W key!"); rb.AddForce(Vector3.up * speed); } } }
In the Start() function, we now get a reference to the Rigidbody component attached to our game object. In the Update() function, instead of using transform.Translate, we’re now using Rigidbody’s AddForce function to move our game object upward.
Note how we retain our “speed” variable to control movement speed. The difference is that, with Rigidbody, our movement is now affected by physics such as gravity and drag.
There you have it! Learning Unity C# unlocks your gaming world, giving tools to create a range of exciting games and experiences. With Zenva, we aim to make education engaging and accessible, so don’t hesitate to dig into our other courses or ask questions. We’re here to guide you on your coding journey.
Moving Beyond Basic Movement
At this point we’ve delved into creating scripts, detecting input, and simple movement with Unity’s Rigidbody system. Now, let’s venture past this foundation and explore how we can make characters jump, rotate, and interact with our game environment.
Jumping Mechanics
Jumping is a hallmark mechanic in many games, from platformers to first-person shooters. Incorporating a jumping mechanism in our code builds upon what we’ve learnt so far:
public class HelloWorld : MonoBehaviour { public float speed = 10f; public float jumpForce = 5f; private Rigidbody rb; private bool isJumping; void Start() { rb = GetComponent<Rigidbody>(); } void Update() { if(Input.GetKey(KeyCode.W)) { Debug.Log("You pressed the W key!"); rb.AddForce(Vector3.up * speed); } if(Input.GetKeyDown(KeyCode.Space) && !isJumping) { Debug.Log("Jump!"); rb.AddForce(Vector3.up * jumpForce, ForceMode.Impulse); isJumping = true; } } void OnCollisionEnter(Collision other) { if(other.gameObject.CompareTag("Ground")) { isJumping = false; } } }
Here, we’ve added a new float variable, jumpForce, to control the strength of our jump. We also have a bool variable isJumping that determines whether our character is currently jumping.
In the Update() function, we added a new condition. If the player presses the Space key and is not currently jumping, our character jumps up. The isJumping variable then becomes true.
The OnCollisionEnter() function fires when our game object enters a collision with another object. If our character collides with an object tagged with “Ground,” we set isJumping to false, and our character can jump again.
Rotating GameObjects
Rotation can occur upon command or continuously to create effects such as spinning collectibles:
public class HelloWorld : MonoBehaviour { public float rotationSpeed = 90f; void Update() { transform.Rotate(Vector3.up, rotationSpeed * Time.deltaTime); } }
In this simple script, our game object rotates at the set rotationSpeed in degrees per second along the Y-axis. This continuous rotation can make collectibles or other elements of a game more eye-catching.
Shot Mechanics
Often, games require characters to shoot or throw objects. Here’s how we could implement such a feature, spawning a projectile when the player presses the Space key:
public class HelloWorld : MonoBehaviour { public GameObject projectilePrefab; public float shootForce = 20f; void Update() { if(Input.GetKeyDown(KeyCode.Space)) { GameObject projectile = Instantiate(projectilePrefab, transform.position, Quaternion.identity); Rigidbody rb = projectile.GetComponent<Rigidbody>(); rb.AddForce(transform.forward * shootForce, ForceMode.Impulse); } } }
Here we’ve added a new variable, projectilePrefab, which we assign in the Unity editor to the prefab we want to spawn. When the player presses the Space key, we spawn a projectile at the character’s position, apply a Rigidbody to it, and propel it forward.
Interacting with the Environment
Now let’s wrap up with a script enabling our characters to interact with their environments:
public class HelloWorld : MonoBehaviour { private void OnCollisionEnter(Collision other) { if(other.gameObject.CompareTag("Enemy")) { Debug.Log("Hit an enemy!"); Destroy(other.gameObject); } } }
This script logs “Hit an enemy!” and destroys the enemy object when we collide with an object tagged “Enemy”. It’s a simple interaction, but it demonstrates how you can interact with various game objects.
That’s it for this session! Remember, these are just the basics. The possibilities are endless with Unity C#, and you can create anything you imagine with the right knowledge and passion.
Remember to keep exploring, learning, and coding, and don’t forget to have fun along the journey! Stick with us at Zenva, and we’ll provide you with top-notch, accessible, and engaging content to excel in Unity C#, game development, and more!
Mastering the fundamentals is just the first step on your journey to becoming a proficient game developer. As you continue exploring Unity C#, you’ll discover a plethora of tools, mechanisms, and possibilities that this powerful combination offers. There’s a vast and exciting realm to discover!
To help you push your boundaries and excel, we invite you to explore our comprehensive Unity Game Development Mini-Degree. This Mini-Degree provided by Zenva Academy covers essential topics about Unity game development, such as game mechanics, audio effects, and building cinematic cutscenes. It’s designed for all skill levels, and is updated regularly to keep pace with the dynamic game development landscape.
We also offer a broader range of Unity courses that cater to a variety of specific interests and skill levels. With over 250 supported courses, we’re committed to providing valuable and up-to-date programming content. No matter where you’re at in your journey, Zenva is here to support you in becoming a well-rounded, professional developer. Keep exploring, learning, and creating!
Conclusion
We’ve just ascertained the potent possibilities of Unity C#, a gateway that leads you to the dynamic world of game development. With Unity and C#, you can bring your creative visions to life, create immersive games, and take a leap into the flourishing gaming industry.
Remember that this thrilling journey is one of constant exploration and learning. At Zenva, we’re here to accompany you on this path, providing rich, up-to-date resources to help you excel. So why wait? Dive deeper into Unity and C# with our comprehensive Unity Game Development Mini-Degree and start materializing your gaming visions today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
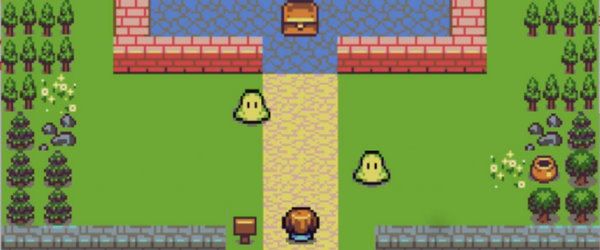
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.