Welcome to this insightful journey into the world of GDScript signals. This versatile language component is a must-learn for every aspiring game developer looking to master Godot Engine. It’s simple, yet powerful nature brings a new dimension to your game development toolkit.
Let’s delve into this intriguing subject and discover what makes GDScript signals a game-changer in the creation of interactive and dynamic games.
Table of contents
What are GDScript Signals?
Signals in GDScript serve as an integral messaging system within Godot Engine. They offer an efficient way to handle in-game events and interactions without heavily relying on frequent polling or tightly coupled scripts.
The Utility of GDScript Signals
Imagine setting a trap in your game that signals an enemy’s presence once triggered. Or perhaps, you want a ninja character to signal the player’s score increment whenever it successfully sneaks past guards. That’s where GDScript signals come in. They enable you to seamlessly orchestrate various aspects of your game mechanics, making them interact and respond to each other.
Why Learn GDScript Signals?
A solid understanding of GDScript signals is fundamental for creating games that are advanced and immersive. They help in circumventing the pitfalls of excessive polling, fostering optimized performance and simplicity in your code.
Their event-driven design pattern cuts down dependencies between scripts, fostering code flexibility, maintenance, and reusability. As a result, you create a complex network of game interactions that can be tweaked easily without causing unwanted side effects.
Basics of Emitting and Connecting Signals
To understand signals, we must first understand how to emit and connect them. Typically, this entails defining a signal, emitting the signal when an event transpires, and connecting the signal to a method that will be triggered upon the signal occurrence.
# Step 1: Define a signal signal game_over
This chunk of code simply declares a signal named ‘game_over’.
# Step 2: Emit the signal emit_signal("game_over")
When the game conditions dictate that it should end (for instance, when the player’s health drops to zero), the ‘game_over’ signal is emitted using the method above.
# Step 3: Connect the signal to a method connect("game_over", self, "_on_game_over")
Finally, we connect this signal to a method. In this case, we are instructing the game to trigger the ‘_on_game_over’ method when the ‘game_over’ signal is emitted.
Advanced Signal Uses
We have explored how to emit and connect signals. Now let’s examine some more advanced use cases you could implement in your games.
Gathering data from a signal:
signal player_scored(score) # Player scores emit_signal("player_scored", current_score) # Handler Function func _on_player_scored(score): print("Player scored " + str(score) + " points")
In this case, the ‘player_scored’ signal carries additional data– the player’s score. When the signal is emitted, the ‘current_score’ data is passed over to the method connected to the signal, which then handles it appropriately.
Multiple connections:
# Add multiple handlers to a signal connect("game_over", self, "_on_game_over") connect("game_over", self, "_save_game") func _on_game_over(): print("Game Over") func _save_game(): print("Saving game")
This code shows that a single signal can be connected to multiple methods. Here, the ‘game_over’ signal triggers the ‘_on_game_over’ method and the ‘_save_game’ method.
Disconnecting Signals
Godot Engine allows us to disconnect signals that we no longer need. Maybe you need the signal connected only for a certain duration, or you’d like to stop a specific action from being triggered. Here’s how to do it:
disconnect("game_over", self, "_on_game_over")
The ‘disconnect’ function in the code above signifies that whenever the ‘game_over’ signal is emitted, the ‘_on_game_over’ method will no longer be triggered.
You can also check if a signal is connected:
if is_connected("game_over", self, "_on_game_over"): print("Signal is connected")
Built-in Node Signals
One of the beneficial aspects of GDScript signals is that they are not exclusive to custom game events. Godot Engine has a wealth of built-in node signals that can be harnessed to enrich your game mechanics further.
For instance, to detect a button press, you can employ Godot’s built-in ‘pressed’ signal:
func _ready(): $Button.connect("pressed", self, "_on_button_pressed") func _on_button_pressed(): print("Button pressed")
In this example, whenever the button is pressed, the ‘_on_button_pressed’ method is activated, thereby printing ‘Button pressed’ to the console.
Concept of Groups and Signals
In Godot Engine, the “Group” concept can incorporate signals to cultivate more dynamic and versatile interactions. By adding nodes to a group, we are able to emit signals to multiple nodes at once.
# Adding nodes to a group $Enemy1.add_to_group("enemies") $Enemy2.add_to_group("enemies") # Emit signal to all nodes in a group get_tree().call_group("enemies", "emit_signal", "notify")
The code above projects the ‘notify’ signal to all nodes in the ‘enemies’ group. Thus, the signal isn’t confined to a single node, but it’s accessible to an entire group of nodes, resulting in broader impact and interactive opportunities in your game.
Diving Deeper – Complex Utilizations of Signals
Further expanding on our knowledge of GDScript signals, let’s explore more complex applications and use cases with code examples.
Combining Signals and Areas:
Area2D.connect("area_entered", self, "_on_area_entered") func _on_area_entered(area): print(area.name + " entered")
In the above example, we use GDScript signals in conjunction with Area2D, a fundamental node for creating interactive areas in your games. The signal ‘area_entered’ is emitted when an area is entered, triggering the connected method.
Using Signals with Timers:
$Timer.connect("timeout", self, "_on_timer_timeout") func _on_timer_timeout(): print("Timer has timed out")
In this instance, the Timer node dispatches a ‘timeout’ signal when it finishes counting down to zero. Consequently, when the timer times out, a message is printed to the console.
Changing Scenes with Signals:
signal change_scene connect("change_scene", self, "_on_change_scene") func _on_change_scene(): get_tree().change_scene("res://NewScene.tscn")
This example reveals that signals can be used to transition between game scenes. When ‘change_scene’ is emitted, the attached method shifts the current scene to ‘NewScene.tscn’.
Queuing Free with Signals
One of the primary rules in Godot Engine is to avoid directly freeing nodes during signal emission. Instead, we can resort to the ‘queue_free()’ function in combination with signals to safely cleanup nodes:
signal node_deleted connect("node_deleted", self, "_on_node_deleted") func _on_node_deleted(): queue_free()
To explain this, when the ‘node_deleted’ signal is dispatched, the ‘_on_node_deleted’ method uses ‘queue_free()’ to defer the removal of the node until it’s safe to do so.
Returning Values from Signals
Signals can also return values, enabling you to package and transmit more information. However, keep in mind that only the first method connected to the signal will return a value. Consider the following example:
# Signal definition with return type signal find_closest_node(position) -> Node2D # Emitting the signal and getting the return value var closest_node = emit_signal("find_closest_node", player.position)
Here, the ‘find_closest_node’ signal is defined to return a Node2D type. When the signal is emitted, it calculates the closest node to the player’s current position, then passes this information back via the return value.
Throughout these examples, it’s clear that GDScript signals offer vast possibilities to enrich your game with intricate events and interactive elements, ultimately enhancing the player’s overall experience.
The Journey Continues
After conquering the wonderful world of GDScript signals, you’re ready to move on to the next adventure in your game development journey. The sky’s the limit, and we’re here at Zenva to help you reach new heights.
We encourage you to check out our Godot Game Development Mini-Degree. This comprehensive curriculum dives deep into cross-platform game development using the Godot engine. From rendering graphics to mastering the GDScript programming language, controlling gameplay to creating UI systems, you’ll expand your skillset and start building your own game portfolio. The Mini-Degree offers courses suitable for beginners and, like all our courses, can be accessed online at any time, allowing you to learn at your own pace.
Be sure to also explore our broader collection of Godot Courses to keep honing your skills. With more than 250 courses at Zenva, we cover a range of topics from beginner to professional level. We help you learn coding, game development, AI, and much more. Whether you’re new to coding or already have the basics nailed down, Zenva has content to boost your career and advance your learning journey.
Conclusion
Deciphering the mysteries of GDScript signals, we have unlocked a new layer of potential in our game development journey. With signals, our toolkit is fortified with a powerful messaging system enabling us to build advanced, interactive, and dynamic games in the Godot Engine. Now, using signals, we can weave together game elements creating a rich tapestry of player experiences.
The adventure, however, doesn’t stop here. Let’s dive deeper, further hone skills, and raise the bar of what we can create with our Godot Game Development Mini-Degree. Remember, in the realm of game development, every new skill is another waypoint on your journey to mastery. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
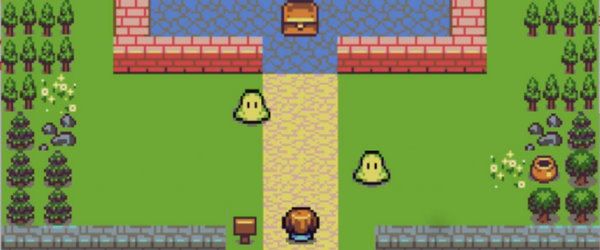
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.