Welcome to this tutorial on GDScript arrays! Whether you’re a seasoned coder or you’re just starting on your coding journey, this comprehensive guide aims to engage you with accessible and practical examples. We’ll delve into the nitty-gritty of these fantastical data handling tools and illustrate how significant they are in the world of coding, specifically in game development. Read along to experience the magic of GDScript arrays and discover their infinite possibilities!
Table of contents
What are GDScript Arrays?
GDScript is the scripting language developed and utilized by Godot Engine, a popular open-source game engine. An array in GDScript, much like in other programming languages, is a data structure that can store a fixed-size sequential collection of elements of the same type. These elements can be of any datatype, and they could be mixed in the same array.
Why are GDScript Arrays Important?
Arrays come in handy when you want to organize and manipulate a fair amount of data in an efficient way. They’re an essential aspect of coding and play a significant role in developing game mechanics in Godot. As a game developer, you’ll often find yourself working with arrays to handle various aspects of your game, such as storing multiple player scores, inventory items, and enemy positions. Therefore, mastering GDScript arrays can greatly streamline your game development process and open up a world of possibilities for what you can build!
What are GDScript Arrays Used For?
GDScript arrays are used extensively throughout game development. For instance, you may use arrays to manage character inventories, moving platforms, or levels in your game. Anytime you’re dealing with numerous items of the same kind, there’s a high likelihood you’ll find arrays to be an efficient solution to your problem.
Navigating GDScript Arrays
Perhaps the easiest way to understand how GDScript arrays work is by diving right in and looking at some simple examples. Below, we are creating an array and filling it with integers.
var my_array = [10, 20, 30, 40, 50]
Once you’ve created an array, you might want to access a specific piece of data stored in it. This can be done with indices, which range from 0 to (array size – 1). Here’s how you can fetch the second element in our array:
var my_value = my_array[1] print(my_value) # This prints: 20
If you want to change the value of an element in the array, you do it this way:
my_array[1] = 25 print(my_array) # This prints: [10, 25, 30, 40, 50]
Deleting and Adding Elements
Arrays in GDScript are dynamic, meaning you can add or remove elements from them. To remove an element from a specific position, you can use the remove() method:
my_array.remove(1) print(my_array) # This prints: [10, 30, 40, 50]
You can also use the push_back() method to add a new element to the end of the array:
my_array.push_back(60) print(my_array) # This prints: [10, 30, 40, 50, 60]
And the reverse function pop_back(), which not only removes the last element but also returns it. This function is frequently used in game development to manipulate data stacks:
var popped_value = my_array.pop_back() print(popped_value) # This prints: 60 print(my_array) # This prints: [10, 30, 40, 50]
Additional GDScript Array Methods and Functions
Next up, let’s explore some additional methods and functions that are important for array management in GDScript.
To work out how many elements are in an array, you can use the size() function. This can be especially useful in ‘for’ loops when iterating over each item in an array:
print(my_array.size()) # This prints: 4
Checking if an array is empty is very straightforward with the empty() function:
print(my_array.empty()) # This prints: False
You can use the insert() method to add value at a specific position. This method accepts two parameters: the index at which the value is to be inserted and the value itself. Here’s an example:
my_array.insert(2, 25) print(my_array) # This prints: [10, 30, 25, 40, 50]
If you’re unsure whether a certain value is in your array or not, you can use the has() method to confirm. If the value is in the array, this function will return true, if not it will return false:
print(my_array.has(25)) # This prints: True print(my_array.has(60)) # This prints: False
Finally, we can find the index of a specific value in an array using the find() function. It will return the position of the first occurrence of the value. If the value is not found, it will return -1:
print(my_array.find(25)) # This prints: 2 print(my_array.find(60)) # This prints: -1
Sorting, Reversing, and Duplicating GDScript Arrays
It’s often helpful to sort the elements of an array in ascending order. Luckily, GDScript comes with a built-in method sort() to do this:
my_array.sort() print(my_array) # This prints: [10, 25, 30, 40, 50]
If you need your elements in descending order, you can sort the array first and then reverse it using the reverse() method:
my_array.sort() my_array.reverse() print(my_array) # This prints: [50, 40, 30, 25, 10]
There may be situations when you want to create a copy of an array, a duplicate so to speak. Note that simply assigning a variable to another array will not produce a copy; both variables will refer to the same array. Instead, use the duplicate() method to create a wholly separate copy:
var my_second_array = my_array.duplicate() print(my_second_array) # This prints: [50, 40, 30, 25, 10]
Multi-Dimensional GDScript Arrays
Most of what we’ve talked about so far revolves around one-dimensional arrays, but GDScript also supports multi-dimensional arrays. These are essentially arrays that contain other arrays. Here’s a common application of this concept:
var my_2d_array = [[1, 2], [3, 4], [5, 6]] print(my_2d_array[1][0]) # This prints: 3
In this example, we create an array of arrays (a 2D array) and then print the first element of the second array within it.
There you have it! From basic operations to multidimensional arrays, this guide covers a large portion of what you need to know about GDScript arrays. With practice and application, you’ll master GDScript arrays and their functionality in no time! Keep experimenting and see how these versatile data structures can streamline your game development process.
Remember, at Zenva, we provide top-notch resources and courses for learning GDScript and a whole other spectrum of programming languages. Join us to elevate your knowledge and programming skills!
Where to Go Next
With a basic understanding of GDScript arrays, you’re well on your way to becoming proficient in game development with Godot! But, the journey doesn’t stop here. To level-up your coding skills and broaden your understanding of Godot, we highly encourage you to keep exploring, practicing, and learning.
For instance, our Godot Game Development Mini-Degree is a substantial, all-embracing set of courses that delve deep into building cross-platform games with Godot. The lightweight engine is suitable for both beginners and advanced developers, teaching you how to work with a flexible, node-based system and the GDScript language.
This comprehensive curriculum covers various game development topics such as using 2D and 3D assets, implementing gameplay control flow, managing player and enemy combat, applying item collection mechanisms, developing UI systems, and constructing various game genres like RPGs, RTS, survival, and platformers. This curriculum includes projects in which you can build a portfolio showcasing real Godot projects.
Godot 4 is free, open-source, and supported by a robust community. The courses are suitable for beginners and experienced programmers, offering flexible learning options and the ability to practice coding in-browser. The curriculum is suitable for first-time learners and the courses are facilitated by instructors certified by industry leaders.
If you’re inclined towards more personalized learning, you can examine our dedicated array of Godot Courses, designed for an all-around mastery of this game engine.
Remember, with Zenva, you can go from beginner to professional. We offer over 250 comprehensive courses in programming, game development, and AI, that allow you to earn certificates, create games, and learn coding at your own pace. Whether you’re an absolute novice or have already mastered the basics, there’s always something new to learn with us! Continue your journey, conquer coding and game development, boost your career, and above all, never stop learning!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
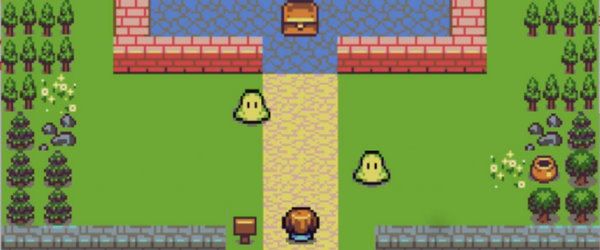
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.