Welcome to the first step in your journey to mastering modern C++. Our aim with this tutorial is to provide you with a comprehensive introduction to effective modern C++. This tutorial seeks to make the often complex aspects of C++ much more accessible, and is designed to be both engaging and valuable in your ongoing coding education. Rest assured, by the end, you’ll not only understand the fundamentals but feel more confident utilizing modern C++ in your projects.
Table of contents
What is Modern C++?
Modern C++ refers to the latest standards and practices embraced by the C++ community. This is a constantly evolving collection of features and techniques, designed to allow developers to write cleaner, more efficient, and more maintainable code.
What is it Used For?
C++ is a powerful and versatile language. With modern C++, you can develop desktop and mobile applications, video games, and even high-performance software for scientific computing. Its wide-ranging usability makes it a cornerstone in software development.
Why Learn Modern C++?
Understanding modern C++ is critical for several reasons:
- Efficiency: Modern C++ enhancements provide the developer with the ability to write clean, efficient and maintainable code.
- Opportunity: C++ powers much of the software industry, meaning a broad range of career opportunities for those proficient in modern C++.
- Future-proof skills: By learning modern C++, you are making an investment in a dynamic, constantly evolving language that will stay relevant in future technology trends.
In the following sections, we will delve into practical coding examples to help you get a feel of the language’s modern application. By focusing on game mechanics and analogous scenarios, we aim to provide you with an engaging and interactive study experience. Stay tuned!
Setting Up the Environment
To get started with coding in modern C++, we first need to ensure your coding environment is set up correctly. We’ll be using an integrated development environment (IDE).
// On windows g++ --version // On Linux gcc --version
You can either download GCC directly or use package managers depending on the system. If it’s not installed, follow your system’s usual installation procedure to install.
Basic Syntax
With the environment ready, let’s dive into some actual coding. We’ll start with the basic syntax of Modern C++.
// This is a simple C++ program #include int main() { std::cout << "Hello, world!"; return 0; }
In the above code:
#include
is a preprocessor command which includes the iostream standard library. Thelibrary is used for input/output operations.
int main()
is the main function where program execution begins.std::cout << "Hello, world!";
prints the string “Hello, world!”.return 0;
ends program execution.
Data Types
The next element of modern C++ to understand is data types. Let’s examine some of the basic data types in C++:
// Defining integer data type int myNum = 5; // Defining float data type float myFloatNum = 5.99; // Defining double data type double myDoubleNum = 9.98; // Defining character data type char myLetter = 'D'; // Defining boolean data type bool myBoolean = true; // Defining string data type std::string myText = "Hello";
Control structures: If-Else
To demonstrate control structures, let’s look at a classic If-Else code snippet:
int main () { int x = 10; int y = 20; if ( x > y ) { std::cout << "x is greater than y"; } else { std::cout << "y is greater than x"; } return 0; }
This code compares the two values and prints out a statement depending on the result.
That covers the second part of our tutorial. The third part will delve deeper into the world of C++. Stay tuned!
Loops in C++
Loops are a key aspect of coding. Here is an example of a for loop, but modern C++ also supports while and do-while loops.
// Program to print numbers from 1 to 10 #include int main () { for(int a = 1; a <= 10; a++) { std::cout << a << std::endl; } return 0; }
In the above code, the loop will continue to increment the variable a by one and print its value, as long as a is less than or equal to 10.
Functions in Modern C++
Functions are reusable pieces of code that perform a specific task within a program. Here’s a simple function that adds two numbers together:
int addition (int a, int b) { int r; r=a+b; return r; } int main () { int z = addition (5,3); std::cout << "The result is " << z; }
In this program, we define and then call a function within the main function. It outputs “The result is 8”.
With modern C++, we can define multiple functions with the same name but different parameters, known as function overloading. This allows for more flexible code.
Arrays and Vectors in Modern C++
An array is a series of elements of the same type, whereas a vector in C++ is a dynamic array that can grow and shrink in size.
Here’s an example of an array:
// Define an array of 5 elements int myNumbers[5] = {1, 2, 3, 4, 5}; // Access element number 2 int secondNumber = myNumbers[1];
And here’s how you’d define a similar vector:
// Include vector library #include // Define a vector std::vector myVector = {1, 2, 3, 4, 5}; // Access element number 2 int secondNumber = myVector[1];
Notice that vectors are much more flexible, as their size can be changed dynamically.
Modern C++ and Object Oriented Programming
We finish our tutorial by explaining Object-Oriented Programming (OOP) in modern C++, which has extensive support for classes and objects.
Here’s an example of a simple class:
class Car { public: std::string brand; std::string model; int year; }; int main() { Car myCar; myCar.brand = "BMW"; myCar.model = "X5"; myCar.year = 1999; std::cout << myCar.brand << " " << myCar.model; return 0; }
This code creates a new Car object called myCar, and then sets the brand, model and year fields. It finally outputs “BMW X5”.
Going through these examples should provide you with a solid foundation in modern C++. We look forward to seeing you unleash your newly acquired skills in your projects.
Advanced Concepts in Modern C++
Having covered the basics of modern C++, let’s now move forward and delve into some of the advanced concepts that make C++ a powerful and flexible programming language.
Pointers, References and Memory Management
One of the key features of C++ is direct memory management. This is largely done through pointers and references. Here’s an example of using pointers:
// Define an integer variable int a = 20; // Define a pointer variable int* p; // Store address of a in pointer variable p = &a; std::cout << *p; // prints the value stored at the addressed pointed to by the pointer.
Pointers can be powerful but dangerous. It is important to use them with care, and only when necessary.
Const in Modern C++
The `const` modifier can make sure that variables aren’t changed accidentally. Here is an example of how to use `const`:
int main() { const int yearEstablished = 2010; std::cout << "Year established: " << yearEstablished << std::endl; return 0; }
Exceptions and Error Handling
Here’s an example of the try/catch/finally syntax that Modern C++ uses for handling exceptions:
double division(int a, int b) { if( b == 0 ) { throw "Division by zero error!"; } return (a/b); } int main() { int x = 50; int y = 0; double z = 0; try { z = division(x, y); std::cout << z << std::endl; } catch (const char* msg) { std::cerr << msg << std::endl; } return 0; }
Templates in C++
Modern C++ uses templates to define functions and classes that operate on different data types without having to reprogram for each type. Here’s an example of a function template:
template T maximum(T a, T b) { return (a > b) ? a : b; } int main() { int maxInt = maximum(3, 5); double maxDouble = maximum(3.0, 5.0); std::cout << "Maximum Int:" << maxInt << std::endl; std::cout << "Maximum Double:" << maxDouble << std::endl; return 0; }
The `maximum` function works on both `int` and `double` types and returns the maximum of two numbers.
That covers the key advanced concepts of modern C++. Mastery of these will provide you with a solid foundation to tackle any coding project or challenge. Our next and final part of this tutorial will look at the use of modern C++ in real-world applications.
Where to Go Next?
Armed with the knowledge from our guide, you’re now ready to explore the world of modern C++, but learning doesn’t stop here! There is always more to discover, as programming languages like C++ constantly evolve and progress. We encourage you to continue pushing your boundaries and fortifying your coding abilities.
A fantastic place to continue this adventure is our C++ Programming Academy. The program offers courses that cover essential topics such as program flow and object-oriented programming, as well as more specialized knowledge in areas like the SFML game development library. Our curriculum is project-based, allowing you to acquire hands-on experience by creating your own games and building a portfolio of real projects.
Entry barriers? None! The C++ Programming Academy is designed for you to start from the basics and gradually work towards more complex concepts at your own pace. So, whether you’re stepping into the coding world for the first time or have initial knowledge, we’ve got you covered.
We also recommend exploring our broad range of C++ courses – there’s plenty to learn with over 250 coding, game development, AI, and data science modules taught by our certified instructors.
Remember, every coding session, every program, every bug you fix takes you one step closer to mastery. Embrace the journey with us at Zenva – where we take you from beginner to professional. Happy Coding!
Conclusion
And there you have it! With this comprehensive guide, we’ve walked you through the exciting world of modern C++. From setting up your programming environment to diving into complex concepts like pointers, exception handling and templates, we’ve shone a light on the power and flexibility that C++ offers.
As you ready yourselves for the magnificent world of coding, always remember what Steve Jobs once said: “The people who are crazy enough to think they can change the world are the ones who do.” So don’t back away from challenges. Join us on this exciting journey and be the world-changer we believe you can be! Venture further with our C++ Programming Academy to unlock more knowledge and coding prowess. Let’s code, learn, and thrive together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
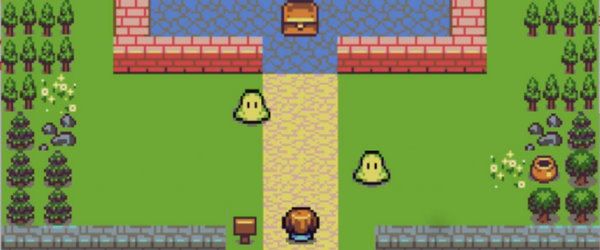
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.