Welcome to the world of C++ development with Eclipse! This tutorial will open up a new realm of opportunities for your coding journey. Starting with the basics, we’ll guide you on how to use the Eclipse IDE for C++ programming.
Table of contents
What is Eclipse C++?
Eclipse is an integrated development environment (IDE) used for computer programming. It contains a base workspace and an extensible plug-in system for customizing the environment. Eclipse is widely used for Java programming, but with the right plug-ins, it can be tailored for C++ development as well.
What is it for?
Eclipse C++ allows developers to write, compile, and execute C++ programs within a single environment. It offers advanced tools for code editing, debugging, and testing, which makes the coding process smoother and more efficient.
Why should I learn to use Eclipse for C++?
Mastering Eclipse for C++ can enhance your productivity significantly. It eliminates the need to switch between multiple tools during the development process. Moreover, Eclipse’s intelligent suggestions and error detection features can help you write cleaner, bug-free C++ code. Most importantly, it’s free to use and supported by a large, active community. So, let’s get started and see what Eclipse C++ has in store for us!
Installation and setup
Before we can use Eclipse for C++, we need to set up our development environment. Firstly, we need to install the Eclipse IDE. Secondly, we have to install the C++ Development Tools (CDT) plug-in.
The steps to install the Eclipse IDE on your computer are:
1. Visit the official Eclipse website. 2. Download the appropriate Eclipse Installer for your operating system. 3. Run the Installer and follow the prompts.
Once the Eclipse IDE has been installed, you need to install the CDT plug-in to enable C++ development.
1. Open Eclipse 2. Go to 'Help' > 'Eclipse Marketplace' 3. In the search bar, type 'CDT' 4. Click 'Go' 5. Find the C++ Development Tools and click 'Install'
Creating a new C++ project
After the setup process, we can now create a new C++ project in Eclipse. Follow the below steps to make a new project:
1. Open Eclipse 2. Click on 'File' > 'New' > 'C++ Project' 3. Give your project a name 4. Choose 'Empty Project' under 'Project type' 5. Select your preferred toolchain (for example, MinGW in Windows) 6. Click 'Finish'
Writing a C++ program
With a new project ready, let’s now write our first C++ program in Eclipse. This example will demonstrate how to create a simple program that prints “Hello, World!” to the console.
1. Right-click on your project in the 'Project Explorer' 2. Select 'New' > 'Source File' 3. Name your file (e.g., "main.cpp") 4. Hit 'Finish' 5. In the new source file, write the following code: #include<iostream> int main() { std::cout << "Hello, World!"; return 0; }
Building and running a C++ program
Finally, let’s build and run your first C++ program.
1. Save your source file by pressing 'Ctrl + S' 2. Right-click your project in the 'Project Explorer' 3. Click 'Build Project' 4. Wait for the build to finish 5. Right-click your project again 6. Click on 'Run As' > 'Local C/C++ Application'
That’s it! The output “Hello, World!” should be displayed in the Console tab. Congratulations on writing, building, and running your first C++ program in Eclipse!
Exploring Basic C++ programming in Eclipse
This section will provide a deeper overview of C++ programming in Eclipse with essential code examples. These examples act as a guide to understand how to write and execute basic C++ instructions in Eclipse.
Data types and Variables
C++ has several data types, including integers (int), floating-point numbers (float), characters (char), and booleans (bool). You can declare a variable with a specific data type as follows:
int a = 10; float b = 5.5; char c = 'G'; bool d = true;
These variables store an integer, a fractional number, a character, and a boolean respectively.
Conditional Statements
C++ allows you to make decisions in your code by using conditional statements. The ‘if’ and ‘else’ statements help in executing certain parts of code based on conditions.
int score = 90; if(score >= 90) { std::cout <= 75) { std::cout << "Very Good!"; } else { std::cout << "Keep trying!"; }
In the above code, the program checks the value of the variable ‘score. If the score is 90 or above, it prints “Excellent!”. If it’s less than 90 but equal to or above 75, it prints “Very Good!”. All other instances will print “Keep trying!”.
Loops
C++ provides several ways to loop over code including the ‘for’, ‘while’, and ‘do-while’ loops. Below we introduce the ‘for’ loop:
for(int i = 0; i < 5; i++) { std::cout << "Iteration " << i << "\n"; }
This ‘for’ loop will print “Iteration 0”, “Iteration 1”, …, “Iteration 4” on separate lines.
Functions
In C++, we define functions to code reusable blocks. A function can be defined as follows:
void greeting() { std::cout << "Hello, World!"; }
This is a function named ‘greeting’ that will print “Hello, World!” when called. To call this function, simply use its name followed by parentheses in your main code:
int main() { greeting(); return 0; }
When run, this program will output “Hello, World!”.
These are just the basics! Practice these concepts and explore more advanced topics like arrays, pointers, classes, and file handling to further enhance your C++ proficiency in Eclipse.
Arrays and Strings
An array is a fixed-size sequence of elements of the same type. It can be declared as follows:
int numbers[] = {1, 2, 3, 4, 5};
You can access individual items in the array using their index:
std::cout << numbers[0]; // prints 1 std::cout << numbers[3]; // prints 4
A string is essentially an array of characters. It can be declared and manipulated like:
std::string name = "Alice"; std::cout << name; // prints Alice
Pointers
A pointer is a variable that stores the memory address of another variable. Here’s how it works:
int num = 10; int *ptr = # std::cout << *ptr; // prints 10
In this example, ‘ptr’ is a pointer. The symbol ‘*’ is used to denote a pointer. And ‘&’ is used to get the address of a variable. So, *ptr gives the value of the variable whose address the ptr holds, which is ‘num’.
Classes and Objects
OOP (Object Oriented Programming) is a central concept in C++. A class is defined using the ‘class’ keyword:
class Vehicle { public: std::string type; int wheels; void display() { std::cout << "Type: " << type << "\n"; std::cout << "Wheels: " << wheels << "\n"; } };
Here, ‘Vehicle’ is a class, which has two properties (type and wheels) and a method (display). An object of this class can be created and used as:
Vehicle car; car.type = "Car"; car.wheels = 4; car.display(); // prints Type: Car, Wheels: 4
Error Handling
C++ uses exceptions to handle errors and other exceptional events. This is a simple demonstration of exceptions in C++:
try { throw "Error occurred!"; } catch (const char* msg) { std::cerr << msg << std::endl; }
Crystalize these advanced concepts and continue expanding your knowledge for mastering C++ programming in Eclipse.
Where to Go Next?
So, you’ve got the basics of using Eclipse for C++ programming down pat. What’s next? How about really upping your game and truly mastering C++ in all its diversity? We at Zenva are here to help and guide you through this process.
Dive deeper into the world of C++ with our C++ Programming Academy. Designed for both aspirants and seasoned coders, the Academy offers a comprehensive journey covering everything from C++ basics to advanced object-oriented programming, engaging game mechanics concepts, and dealing with graphics and audio with SFML. You’ll also work on enriching hands-on projects, giving you ample opportunity to apply the knowledge you’ve gained.
Beyond specific courses, you can explore our broader collection of C++ related content. Whatever your coding passion – be it game development, AI, or programming, we support a vast portfolio of over 250 practical courses for learners at all levels. Learn coding, create games, earn certificates, and start building the future today with Zenva!
Conclusion
So, are you ready to embark on an exciting journey of coding, creating, and innovating with Eclipse and C++? The possibilities are virtually endless as you move from the basics to becoming a seasoned coder with our C++ Programming Academy.
In this dynamic digital age, coding isn’t just a skill; it’s a superpower. We at Zenva are committed to guiding you on this empowering journey, providing resources, tools, and courses that enable you to optimize your potential. Let’s venture into the bold world of C++ together. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
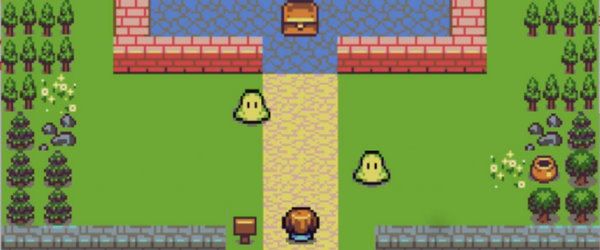
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.