Welcome to this tutorial on one of the most basic yet crucial concepts in C++ – the “cout” statement. It might seem simple on the surface, but mastering how to use it appropriately and creatively is a significant step towards becoming proficient in C++. Stay with us as we delve into the exciting world of C++ and help you learn quickly and effectively. We are confident you will find this article engaging, comprehensive, and beneficial.
Table of contents
What is the “Cout” in C++?
In C++, Cout stands for Console Output. It is the statement used to display data on the console or the output screen. The term “cout” might feel a bit unusual, but it’s just a short form for “character out”. The usage of cout forms the backbone of C++ programming, particularly for beginners making their initial forays into the language.
Why should you learn it?
Cout in C++ plays a vital role for a programmer. It helps you interact with your code by displaying results, debug information, or messages to the console. Understanding how to use cout effectively becomes even more critical when working on large projects or sophisticated systems. Here’s why you should master it:
- It provides a quick and easy way to display outputs or results.
- It aids in debugging by allowing you to trace code execution.
- Understanding cout creates a solid base for learning other advanced aspects of C++.
In the world of gaming, cout can be used to print game scores, player instructions, and even to develop simple text-based adventure games! Its uses are limited only by your imagination.
What is it for?
The primary use of cout is to output data to the console or the output screen. Whether you want to display a simple message, the result of a mathematical operation, or game scores, cout is your go-to statement in C++. It’s versatile, easy-to-use, and an essential tool in a programmer’s toolkit. So, understanding how to use “cout” effectively opens you up to various possibilities in C++.
Basic Use of Cout
Let’s start our learning journey by looking at the most straightforward use of the cout statement. Here’s a simple example:
#include <iostream> using namespace std; int main(){ cout << "Hello, Zenva!"; return 0; }
In this snippet, “Hello, Zenva!” will be displayed on the console. Notice the special characters ‘<<'. These are insertion or stream operators and they are used to insert the data which comes after them into the cout.
Adding More Elements to Cout
Another important feature of cout is that it allows you to add multiple elements in one line. Let’s see how:
#include <iostream> using namespace std; int main(){ cout << "Learn with " << "Zenva"; return 0; }
In this example, the output will be ‘Learn with Zenva’. Here, we’ve used the same ‘<<' operators to join two strings.
Using Cout with Variables
C++ programmers can also use cout to display the value of variables of any data type. Here’s a simple example demonstrating the idea:
#include <iostream> using namespace std; int main(){ int score = 100; cout << "Your game score is: " << score; return 0; }
This example illustrates how to use cout with an integer variable. The output will be ‘Your game score is: 100’. By inserting the variable name immediately after the insertion operator, we tell C++ that we want to display the value of that variable.
Sending Multiple Lines to the Output
Lastly, let’s learn how we can display multiple lines of text with cout. We can use ‘\n’ or endl:
#include <iostream> using namespace std; int main(){ cout << "Welcome to Zenva's" << endl << "C++ Programming Course!"; return 0; }
In this case, ‘Welcome to Zenva’s’ and ‘C++ Programming Course!’ will be displayed on two separate lines. The endl keyword serves as a newline character and moves the control to the next line.
We have covered the fundamentals of using cout in C++. Now it’s time for some practice! Remember, keep it fun and engaging, and do not hesitate to experiment with various elements while using cout. After all, the best learning is through doing! Let’s dive deeper in the next section!
Formatting Output with Cout
Now that we’ve got the basics covered, we can start looking at how to use cout for more complex outputs. Let’s talk about formatting.
#include <iostream> using namespace std; int main(){ int score_1 = 95; int score_2 = 86; cout << "Your Game Scores:\n" << "First Score: " << score_1 << "\nSecond Score: " << score_2; return 0; }
In this snippet, the ‘\n’ is used to add a new line where we need to. This helps keep the output clean and readable.
Using Cout with Conditions
We can also use cout to display the result of conditional statements.
#include <iostream> using namespace std; int main(){ int score = 90; if(score >= 90){ cout << "Excellent!"; } else { cout << "Keep Trying!"; } return 0; }
In this example, because the score is more than or equal to 90, the output would be ‘Excellent!’. This comes in handy when creating more complex games or applications, and you want to print different messages based on different conditions.
Using Manipulators in Cout
Manipulators are used in cout to format the output. There are several manipulators available in C++, but for now, let’s focus on setw() and setprecision().
#include <iostream> #include <iomanip> using namespace std; int main(){ double score = 96.4556; cout << setw(10) << setprecision(3) << score; return 0; }
In this code snippet, the setw(10) manipulator sets a width of 10 characters for the output and the setprecision(3) manipulator sets the number of digits to be displayed after the decimal point to 3. Hence, the output we will get is ‘ 96.456’.
Using Cout inside Functions
Lastly, let’s see how we can use cout inside a function in C++.
#include <iostream> using namespace std; void displayMessage() { cout << "Welcome to Zenva!"; } int main(){ displayMessage(); return 0; }
Here, we’ve declared a function named ‘displayMessage’ that contains a cout statement. When we call this function in the main function, it executes the code inside it, displaying ‘Welcome to Zenva!’ on the screen. This can be very useful when working with larger codebases where the same message needs to be printed multiple times.
The possibilities with cout are vast and exciting. As we mentioned earlier, the best way to learn is by doing. So, don’t stop here. Continue experimenting with cout functions, exploring its various applications, and mastering its uses. Happy learning!
Advanced Use of Cout with Objects
C++ follow simple but expressive syntax for output operations on objects. Using cout with objects is an important feature, especially when working with Object-Oriented Programming.
#include <iostream> using namespace std; class Player { public: string name; int id; }; int main(){ Player player1; player1.name = "Zenva Player"; player1.id = 1; cout << "Player Name: " << player1.name << "\nPlayer ID: " << player1.id; return 0; }
In the above code, we’ve defined a simple Player object with name and id as attributes. Later, we print these details to console with cout.
Using Cout with STL Containers
We can also use cout to print out elements of an STL container, such as a vector or a list:
#include <iostream> #include <vector> using namespace std; int main(){ vector<int> scores = {85, 90, 96}; for(int i=0; i<scores.size();i++){ cout << scores[i] << "\n"; } return 0; }
This demonstrates how to use cout to print the elements of a vector. This can be very handy when dealing with data structures.
Using Cout in Loops
Loops and cout often go hand in hand. Here’s a simple demonstration using a for loop:
#include <iostream> using namespace std; int main(){ for(int i=0; i<5; i++){ cout << "Count: " << i << "\n"; } return 0; }
As loop’s counter increases, for each iteration we print the current value to the console using cout.
Advanced Formatting with Cout
We can also use cout for some advanced output formatting, such as right or left alignment of output or setting a fill character:
#include <iostream> using namespace std; int main() { int score= 1234; cout << setw(10) << score << endl; // Right alignment (default) cout << left << setw(10) << score << endl; // Left alignment cout << setfill('*') << setw(10) << score << endl; // Setting a fill character return 0; }
We are now nearing the end of our tour with the cout statement in C++. We hope the code examples and explanations will encourage you to explore further and try out unique coding practices. As you continue to build your understanding, try to use cout with various data and control structures, classes and objects to print into the console screen. The journey to mastering C++ is an exciting one, and understanding cout in-depth takes you one step closer to it!Now that you’ve made your first steps into the world of C++ and understood the importance of the cout statement, it’s time to bolster your skillset and continue your journey in programming.
We encourage you to check out our C++ Programming Academy. This suite of five comprehensive courses will deepen your understanding of C++, game development, and working with graphics and audio using SFML. Each course is project-based and designed to fast-track your development skills. Whether you’re a beginner with no prior coding experience or an experienced programmer seeking to broaden your abilities, our academy can provide you with the knowledge you need. Our courses are flexible and can be accessed 24/7, and upon completion, certificates are available to display your achievements.
Furthermore, for a broader collection of courses, be sure to explore our C++ Courses on the Zenva Academy! Over 250 courses are available at your pace to boost your programming career. Remember, every new thing you learn takes you one step closer to becoming a pro! Your journey with Zenva is full of exciting discoveries! Enjoy the ride and happy learning!
Conclusion
And that’s a wrap on mastering the “cout” statement in C++. With consistent practice and an appetite for exploring its various applications, it won’t be too long before you find yourself leveraging the cout command to create fantastic projects and solve complex problems!
Remember, this is just one aspect of grasping C++. There’s a world of exciting concepts awaiting you in our C++ Programming bundle. Dive in today and continue your learning journey with Zenva!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
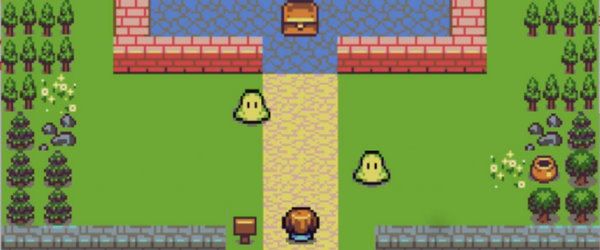
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.