Table of contents
Welcome to the Exciting World of C++
Are you interested in learning programming? Are you intrigued by game development? If your answer is yes, then one language punches above its weight in these arenas and more, and that is C++. C++ is a powerful, high performance and versatile language used to build game engines, desktop apps, and even operates in system/software development.
What is C++?
C++ is a statically-typed, free-form, multiparadigm, compiled, general-purpose programming language. Known for its performance and efficiency, C++ allows the programmer a high degree of control over system resources and memory. The language was developed by Bjarne Stroustrup as an extension to the C language, with added classes and objects – bringing in the flavor of object-oriented programming.
Why Learn C++?
Here are some reasons why picking up C++ would be a wise decision:
- Performance – C++ allows developers direct control of system resources and memory, leading to highly efficient code.
- Game Development – It is a primary language used in the more computational parts of many game engines like Unreal Engine.
- Widely Used – Many popular desktop applications and databases use C++ because of its performance and memory-handling benefits.
- Highly Paid Jobs – Proficiency in C++ can open up great career opportunities in high-paying roles across various industries.
So, if you are ready to dive into the world of coding, let’s start our journey!
Setting up and Your First C++ Program
Before we start coding, you’ll need to set up a C++ compiler. There are many options out there but a popular one for beginners is Code::Blocks, which is available for free.
Once you’ve got your compiler set up, it’s time to write your first program.
#include int main() { std::cout << "Hello World!"; return 0; }
Here’s the breakdown of what’s happening in this simple program:
- #include tells the compiler to include the input/output stream library.
- int main() declares the main function of the program. This is where our program starts.
- std::cout << "Hello World!" prints “Hello World” to the console. `std::cout` is the standard output stream.
- return 0; ends the main function and “returns” a value to the system. By convention, a return of 0 denotes successful execution.
Variables and Data Types
Variables in C++ work as containers to hold data. When you create a variable, you must specify its type, which determines the size and layout of the variable’s memory.
#include int main() { int age = 30; double averageScore = 89.6; char grade = 'A'; std::cout << "Age: " << age << " Average Score: " << averageScore << " Grade: " << grade; return 0; }
In this example:
- int for integer values, double for floating point values, and char for storing individual characters.
- age variable stores 30, averageScore stores 89.6, grade stores ‘A’.
- The << operator is used to pass more than one piece of data to std::cout.
Control Flow
C++ uses control structures that allow for more complicated execution paths. Let’s take a look at how `if`, `else` and `for` loop work.
#include int main() { int num = 6; if (num < 10) { std::cout << "Number is less than 10"; } else { std::cout << "Number is 10 or more"; } for(int i=0; i<5; i++){ std::cout << i << "\n"; } return 0; }
In this code example:
- The if statement checks if num is less than 10, and if it is, it prints “Number is less than 10”.
- If num is not less than 10, it executes the code in the else statement, printing “Number is 10 or more”.
- The for loop starts by initializing `i` to 0. It continues running as long as `i` is less than 5, and after each loop, `i` is incremented by 1. The loop will print the numbers 0 through 4 to the console.
Functions in C++
Functions are a group of statements that perform a specific task. The real power of C++ programming lies in its ability to define and use functions. Consider the following example:
#include using namespace std; // Function declaration int add(int, int); int main() { int sum; sum = add(5, 10); // Function call cout << "Sum: " << sum; return 0; } // Function definition int add(int a, int b) { return a + b; }
In the above code:
- The function `add` is declared before `main()`, and defined after.
- `add(5, 10)` is the function call in `main()`. The values `5` and `10` are the actual parameters.
- In the function definition, `a` and `b` are the formal parameters.
- The computation result of `a + b` gets returned to the variable `sum` in `main()`.
Let’s have a look at arrays and how they can be handled in functions.
#include using namespace std; void printArray(int arr[], int size) { for (int i=0; i<size; i++) { cout << arr[i] << " "; } } int main() { int nums[] = {10, 20, 30, 40, 50}; printArray(nums, 5); return 0; }
In this example:
- We declare an array `nums` with values from 10 to 50.
- We then call the function `printArray` and pass the array `nums` and its size.
- The `printArray` function outputs each element of the array on the console separated by a space.
Object-Oriented Programming in C++
C++ is known for its feature of object-oriented programming which includes concepts like classes, objects, inheritance, polymorphism etc. Let’s jump into the basic concept of classes and objects.
#include using namespace std; // Class creation class Car { public: string brand; string model; int year; }; int main() { // Create an object called car1, and specify values for its member variables Car car1; car1.brand = "Ford"; car1.model = "Mustang"; car1.year = 1966; cout << car1.brand << " " << car1.model << " " << car1.year; return 0; }
This example introduces the following concepts:
- Class: The user-defined data type, which holds its own data members and member functions, which can be accessed and used by creating an instance of that class, i.e, an object.
- Object: An instance of a class.
- Public: A keyword to specify that members (attributes and methods) of the class are accessible from outside the class.
- In the `main()`, an object `car1` was created. The members of the object are accessed using the dot operator (.).
Embrace the power of C++ and continue exploring more features and libraries to unlock its full potential. With consistency and practice, you’ll swiftly move from a beginner to an expert level programmer.
Advanced C++ Concepts
As you continue to develop your programming skills, there are more advanced C++ concepts that you’ll encounter. These will not just help make your programming more efficient, but also enable you to better understand how different parts of your code work
Inheritance and Polymorphism
C++ allows classes to be derived one from another, inheriting attributes and methods. This feature is known as Inheritance. Derived classes can override or extend functionalities of base classes, enabling a concept called Polymorphism.
#include using namespace std; // Base class class Vehicle { public: string brand = "Ford"; void honk() { cout << "Tuut, tuut! \n"; } }; //Derived class class Car: public Vehicle { public: string model = "Mustang"; }; int main() { Car myCar; myCar.honk(); cout << myCar.brand + " " + myCar.model; return 0; }
In this example:
- Vehicle is the base class and Car is the derived class.
- The derived class inherits the member variable `brand` and the member method `honk()` from the base class.
- An object `myCar` from the Car class can access the `honk()` method inherited from the Vehicle class.
File I/O
Reading from or writing to a file is another key operation that C++ allows.
A tiny example follows:
#include #include using namespace std; int main () { ofstream myfile; myfile.open ("example.txt"); myfile << "Writing this to a file.\n"; myfile.close(); return 0; }
In the above code:
- A new file “example.txt” is opened using myfile.open() method.
- The string “Writing this to a file.” is written to “example.txt” using the `<<` operator.
- The file is closed using myfile.close().
Exception Handling
Exception handling in C++ is a process to handle runtime errors, so normal flow of the program can be maintained.
#include using namespace std; int main () { int x = 50; int y = 0; int z; try { if(y == 0) { throw "Divide by zero error"; } z = x/y; cout << z; } catch(const char* e) { cerr << "Error: " << e; } return 0; }
In this example:
- The code inside `try` will be executed.
- If an error occurs, the error message will be passed to the `catch` block and the program won’t crash.
- The `throw` keyword is used to throw an exception when the condition `y==0` occurs.
STL – Standard Template Library
STL is a powerful feature in C++ that provides predefined templates for a set of generic classes and functions.
#include #include using namespace std; int main () { vector vec; // Creating a vector of integers vec.push_back(10); vec.push_back(20); vec.push_back(30); for (int x : vec) { cout << x << "\n"; } return 0; }
Here:
- A vector `vec` is defined which is similar to a dynamic array and can resize itself automatically when an element is inserted or deleted.
- Elements `10`, `20`, and `30` are inserted into the vector using push_back().
- The elements are printed using a for-each loop.
Continue exploring and practicing the depth and power of C++. Having a strong command of C++ will not only build your programming confidence but will provide opportunities to dig deep into system design, game development and much more.
What’s Next in Your C++ Journey?
Your C++ learning journey doesn’t stop here. There’s an exciting world of object-oriented programming, data structures, algorithms, and advanced features to explore. You might be interested in our C++ Programming Academy. This academy offers comprehensive courses, beginning from the basics of C++ and advancing to creating games in less than 4 hours. Practical challenges and quizzes fortify your learning and make the courses engaging and interactive.
Keep Learning and Growing with Zenva
With over 250 supported courses, Zenva Academy provides a wide array of learning paths ranging from programming and game development to AI. Whether you are an absolute beginner, or an experienced coder wanting to learn new techniques, Zenva has content tailored to your level of expertise. Our high-quality and latest updated content can help you go from beginner to professional at your own pace.
Don’t forget to check out our broader collection of C++ courses as well, where various other facets of C++ are explored.
At Zenva, we believe in transforming students into creators. Happy learning and creating!
Conclusion
Embarking on a learning journey with C++ provides endless potential to shape your future. Whether in video game development, system design, or even AI, the paths are as limitless as your imagination. Equip yourself with the knowledge and tools to not just participate in the world of technology, but to also be a creator and innovator.
Take the plunge today with Zenva Academy’s C++ programming courses. Empower yourself with the opportunity to learn at your own pace and from the comfort of your own environment. Your road to mastering C++ starts here, at Zenva. The world is waiting for what you will create.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
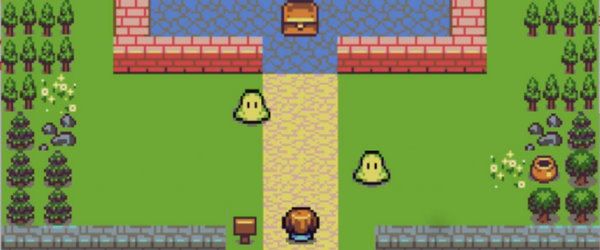
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.