Greetings to all aspiring coders, developers, and game creators! The world of programming languages is vast and exciting, and one of the most powerful and widely-used languages out there is C++. Wondering what C++ is and what you can do with it? Keep reading to find out!
Table of contents
What is C++?
C++ is a high-level programming language. To simply put it, high-level language is closer to human language and can be relatively easier to comprehend and write. C++ is a descendant of the C language but boasts a myriad of enhancements, making it a more robust and versatile tool in software and game development.
What is C++ used for?
Globally acclaimed, C++ is the go-to language for software development. It forms the foundation for operating systems, file systems, and databases. But that’s not all. If you dream of developing compelling video games, knowing C++ could be your ticket to make it come true. Many leading game development engines like Unreal Engine heavily depend on C++.
Why Should I Learn C++?
Apart from software and game development, learning C++ offers several benefits:
- Performance: C++ allows low-level access to memory. This ensures better control of system resources and enhances program performance.
- Large community: C++ has a vast and helpful community. You’ll find plenty of resources, tutorials, and forums to help you navigate the language.
- Great foundation: C++ provides an excellent foundation for learning other programming languages. Concepts like variables, loops, and data structures that you learn here can easily be applied to other languages.
Learning C++ can dramatically elevate your coding skills and open up a world of possibilities. From game development to building high-performance software, C++ could be your stepping stone to achieving these goals.
Understanding C++ Basics
Before diving into code, let’s understand some basic terms and syntaxes of C++.
Print Statements
The first thing you would want to do when learning a new language is print a statement. In C++, we do this using ‘cout’.
#include using namespace std; int main() { cout<<"Hello, World!"; return 0; }
Variables
Variables are used to store data. Naming a variable is important, and C++ has a strict rule: variable names must start with a letter or underscore.
#include using namespace std; int main() { int num = 5; cout<<num; return 0; }
Data Types
C++ supports various data types. It can hold an integer, a decimal number, or a character. Here are examples of different data types:
#include using namespace std; int main() { int a = 5; float b = 7.5; char c = 'z'; cout<<a; cout<<b; cout<<c; return 0; }
Control Statements
We use control statements to determine the flow of a program. The ‘if-else’ statement is the most basic form:
#include using namespace std; int main() { int a = 5; if (a > 3) { cout<<"Number is greater than 3."; } else { cout<<"Number is less than 3."; } return 0; }
These are some of the basic terms and syntax that are used in C++. Practicing these will give you a head start in your C++ journey. In the following section, we will dive deeper and understand some more complex coding structures.
Loops in C++
Loops are instrumental because they allow us to repeatedly execute a block of code. Let’s delve into the three types of loops in C++.
For Loop:
#include using namespace std; int main() { for(int i = 1; i <= 5; i++) { cout<<i; } return 0; }
While Loop:
#include using namespace std; int main() { int i = 1; while(i <=5) { cout<<i; i++; } return 0; }
Do While Loop:
#include using namespace std; int main() { int i = 1; do { cout<<i; i++; } while(i <=5); return 0; }
Functions
Functions in C++ are used to perform specific tasks. Functions help in code reuseability, improving code readability and manageability.
#include using namespace std; void welcome() { cout<<"Welcome to Zenva Academy!"; } int main() { welcome(); return 0; }
Classes and Objects in C++
C++ is an object-oriented programming language, which means it revolves around ‘objects’. An object is an instance of a class (like a blueprint), and it holds both data and functions.
#include using namespace std; class HelloWorld { public: void display() { cout<<"Hello from Zenva!"; } }; int main() { HelloWorld obj; obj.display(); return 0; }
Exception Handling
Unpredicted errors may emerge during the execution of a program. Exception handling in C++ allows us to manage these errors and ensure smooth operation of the program.
#include using namespace std; int main() { try { int num1, num2; cout<>num1>>num2; if(num2 == 0) { throw 0; } cout<<"Result: "<<num1 / num2; } catch(...) { cout<<"Division by zero is not permitted."; } return 0; }
These advanced concepts offer immense power in creating complex and efficient C++ programs. With practice, you’ll be able to bring your ideas to life and create incredible gaming engines, high-performing software, and much more.
Exploring More with C++
As we delve further into the world of C++, let’s explore data structures and some additional features. We’ll dive into arrays, pointers, and recursion. Ready? Let’s get started.
Arrays
An array is a data structure that holds multiple values of the same data type. They are useful when you need to store multiple related data.
#include using namespace std; int main() { int array[5] = {1, 2, 3, 4, 5}; for(int i=0; i<5; i++) { cout<<array[i]; } return 0; }
Pointers
A pointer in C++ is a variable that holds the memory address of another variable. Pointers can be a bit tricky to understand initially, but they hold immense potential.
#include using namespace std; int main() { int num = 5; int *p; p = # //address of num is assigned to pointer p cout<<"Value of num: "<<num; cout<<"Address stored in p: "<<p; cout<<"Value of *p: "<<*p; //*p dereferences the memory address to get the value return 0; }
Reference Variables
Reference variables are aliases for already existing variables. When a variable is declared as reference, it becomes an alternate name for an existing variable.
#include using namespace std; int main() { int x = 5; int &y = x; //y is reference to x y++; //incrementing y will also increment x cout<<"Value of x: "<<x; return 0; }
Recursion
Recursion in C++ refers to a function calling itself. The classic example of recursion is calculating the factorial of a number.
#include using namespace std; int factorial(int n) { if(n == 0) return 1; else return n * factorial(n - 1); } int main() { int num = 5; cout<<factorial(num); return 0; }
File Handling
In C++, you can read from or write to files via file handling mechanism. Let’s create a file and write some text.
#include #include using namespace std; int main() { ofstream file; file.open("example.txt"); file<<"Welcome to Zenva!"; file.close(); return 0; }
As you continue to practice and understand the core concepts of C++, we believe that you’ll find tremendous opportunities to propel your programming journey. From creating high-performance software to game development, the uses of C++ are tremendous, and the quest for learning should never cease.
Where to Go Next?
So, you’ve embarked on your C++ journey and mastered the basics. But like every great adventure, the learning never stops! It’s now time to take your skills a notch higher. Let us help guide you further along this exciting path.
We invite you to explore our C++ Programming Academy. The academy provides comprehensive courses that stretch from C++ programming basics to more complex areas like object-oriented programming and popular game mechanics. The courses are designed with hands-on learning at their core, allowing you to supplement your theoretical knowledge with practical experience by building simple games.
Not only does learning C++ open up a world of creative possibilities, but it’s also highly sought after in the corporate world, so these skills could truly become your career catalyst.
Whether you’re a beginner or a more experienced coder looking to expand your toolkit, our C++ Courses collection has something for everyone. Take the next step in your learning journey with us today!
Conclusion
Congrats on taking the first steps on what we ensure will be an exciting and rewarding journey into the world of C++. Like any language, it will take time and practice to become fluent, but the benefits of mastering C++ extend far beyond creating incredible software and games – you’ll also take on a new way of thinking and problem-solving that can transform your career.
Thousands of Zenva students have already begun their coding journey, boosting their career prospects, and creating some exciting projects. So what are you waiting for? Join us in our C++ Programming Academy today and let’s unleash your full potential together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
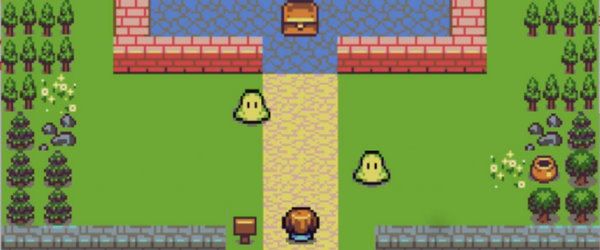
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.