Today, we are venturing into the world of C# and HTML, two powerful tools in the developer’s toolbox. Whether you are a seasoned coder or a beginner taking the first step on your coding journey, C# and HTML can open up exciting opportunities for you. This tutorial will take you through the basics and some intermediate level aspects of these tools, providing you with a robust foundation. We strongly believe that with the right guidance, these tools can turn into a compelling addition to your development skills.
Table of contents
What is C#?
C# is a popular, versatile general-purpose programming language developed by Microsoft. Designed for the .NET framework, C# is widely used in game development, especially in Unity, enterprise software, and more. With its syntax similar to C and Java languages, it simplifies the coding process, making it accessible to beginners while maintaining a powerful platform for advanced coders.
What is HTML?
HTML, or HyperText Markup Language, is the standard language for developing web pages. It provides structure to web content, with the help of tags and attributes that define different parts of a web document. A basic understanding of HTML is essential for all web developers and is often the first stop for beginners exploring web development.
Why Should I Learn C# and HTML?
C# and HTML together offer a versatile combination for game development as well as web applications:
- C# is a go-to language for Unity, a popular game engine, which is used for developing games for consoles, mobile devices, and websites.
- HTML is crucial for developing web applications and websites, making it a critical skill for web development.
- Together, C# and HTML provide a robust foundation for coding, opening up opportunities in a wide range of fields.
Equipped with these languages, you will be able to navigate the world of development with ease and confidence. Whether game or web development is your realm of interest, these tools can help you scale new heights in your career or passion projects. Get ready to dive into the nuances of C# and HTML, and unlock opportunities in this ever-evolving tech landscape.
Basic Syntax in C#
The syntax in C# is intuitive and follows a structured approach, which makes for easy-to-read code. Here are some fundamental aspects:
C# Hello World
using System; public class Program { public static void Main(string[] args) { // This line will print "Hello World!" on the console when run Console.WriteLine("Hello World!"); } }
The code above represents a minimal console application written in C#. The WriteLine function of the Console class is used to print “Hello World!” in the console terminal.
Variables in C#
Variables are essential in any coding language. Here is how to define and use variables in C#:
int a = 5; string b = "Hello"; double c = 3.14159; bool d = true;
In the example above, specific data types are used to define variables of different kinds. We assign an integer to ‘a’, a string to ‘b’, a double precision floating point number to ‘c’, and a boolean to ‘d’.
Conditional Statements in C#
In C#, the most common conditional statements are if/else and switch. Here are examples:
int a = 10; if (a > 5) { Console.WriteLine("The number is greater than 5"); } else { Console.WriteLine("The number is not greater than 5"); }
This ‘if/else’ condition checks if the value of ‘a’ is greater than 5. According to the value of ‘a’, it prints a message on the console.
A ‘switch’ statement can be used to execute different blocks of code based on different outcomes:
int day = 3; switch (day) { case 1: Console.WriteLine("Monday"); break; case 2: Console.WriteLine("Tuesday"); break; case 3: Console.WriteLine("Wednesday"); break; }
This ‘switch’ statement prints the day of the week based on the value of ‘day’.
Loops in C#
In programming, loops are used to repeat blocks of code until a certain condition is met. The basic types of loops in C# are ‘for’, ‘while’ and ‘do while’.
For loop in C#
The ‘for’ loop is handy when you know the exact number of iterations. Here’s an example:
for (int i = 0; i < 5; i++) { Console.WriteLine(i); }
The ‘for’ loop above will print numbers from 0 to 4 in the console.
While loop in C#
The ‘while’ loop continues until the specified condition is true.
int i = 0; while (i < 5) { Console.WriteLine(i); i++; }
The code snippet above performs a similar function as the previous ‘for’ loop but uses a ‘while’ loop instead.
Do-While loop in C#
A ‘do-while’ loop runs the code block once and then continues running it while the specified condition remains true.
int i = 0; do { Console.WriteLine(i); i++; } while (i < 5);
This ‘do while’ loop also prints numbers from 0 to 4, similar to the previous examples.
Functions in C#
Functions are reusable code blocks that perform a specific action. In C#, a function can be defined using the following syntax:
public int AddNumbers(int a, int b) { return a + b; }
In the example above, ‘AddNumbers’ is a function that takes two integers as arguments and returns their sum. Functions can be useful in simplifying complex code and making it more modular and more readable.
HTML
Now that we have covered some primary aspects of C#, let us shift our focus to HTML.
HTML documents are composed of various elements. Each element consists of an opening tag, some content, and a closing tag:
<tagname> Content goes here... </tagname>
Some essential tags in HTML include:
- Hello world – <p>Hello World</p>
- Headline – <h1>This is a Heading</h1>
- Paragraph – <p>This is a paragraph.</p>
- Link – <a href=”https://www.zenva.com/”> Visit Zenva!</a>
By understanding these basics of C# and HTML, you’re taking a significant first step in your coding journey. Continue to explore, experiment, and grow. The world of programming offers endless opportunities, and at Zenva, we are here to guide you every step of the way.
HTML Attributes
HTML elements can contain attributes that provide further information about the element. HTML attributes are always specified in the start (opening) tag. Here’s how you can set attributes:
<tagname attribute="value"> Content goes here... </tagname>
Here’s an example of a paragraph with an id attribute:
<p id="intro">This is a paragraph with an id attribute.</p>
HTML Lists
HTML allows for creating structured lists, both ordered and unordered:
<ul> <li>Coffee</li> <li>Tea</li> <li>Milk</li> </ul>
The code snippet above creates an unordered list using the <ul> tag and individual list items are designated by the <li> tag.
For ordered lists we use the <ol> tag:
<ol> <li>Step 1</li> <li>Step 2</li> <li>Step 3</li> </ol>
The example above makes an ordered (numbered) list.
HTML Tables
HTML tables are defined with the <table> tag, while each row is defined with the <tr> tag. The cells within rows can be defined using the <td>:
<table> <tr> <td>Row 1, Cell 1</td> <td>Row 1, Cell 2</td> </tr> <tr> <td>Row 2, Cell 1</td> <td>Row 2, Cell 2</td> </tr> </table>
HTML Forms
HTML forms are foundational to interactive websites, allowing for data collection from users. Here’s an example of simple HTML form:
<form> <label for="name">Name:</label> <input type="text" id="name" name="name"> <input type="submit" value="Submit"> </form>
In this form, the ‘label’ tag provides a descriptor for the input field, and the ‘input’ tag is used to gather user input.
These examples illustrate how HTML can be used structure web content, create lists, tables and user-interaction forms. Coupled with your C# skills, this understanding of HTML provides a solid foundation for tackling interesting projects in game development and web application arenas.
At Zenva, we believe in making coding accessible and achievable for all enthusiasts, ensuring that you can bring your creative ideas to life. With these robust tools in your repertoire, we pave the way for your success in the world of programming.We’ve now unlocked the door to the exciting world of coding with C# and HTML. But this is just the tip of the iceberg. As you continue your journey, a vast arena of possibilities awaits you. The keys to moving forward are continuously learning and practicing.
At Zenva, we offer a variety of courses that cater to different levels of learners – from beginners to professionals. One such program of note is the Unity Game Development Mini-Degree. This comprehensive program, covering numerous aspects such as game mechanics, cinematic cutscenes, audio effects, and more, is designed to equip you with the skills needed for a career in game development. And remember, Unity is popular not just in gaming, but also in industries such as architecture, film, education, and healthcare. The skills you learn can open doors to various high-paying jobs and opportunities in these booming sectors.
We encourage you to browse our Unity collection for an extensive list of courses that can help you continue your learning journey, taking you from beginner to professional. Keep exploring, keep learning, and let your passion for coding and game development drive you to new heights in the world of programming. With Zenva, you can turn your creativity into reality.
Conclusion
The journey of learning, especially in the world of coding, is an ongoing adventure. Leveraging these fundamental skills in C# and HTML can open up new opportunities in game development, web applications, and beyond. We hope this tutorial has given you a good start on this path.
At Zenva, we are committed to providing high-quality learning resources that help turn learners into makers. Our Unity Game Development Mini-Degree is geared towards supporting you at every step of your coding quest. So why wait? Dive in, start learning, and let the world of programming unfold its wonders to you. Code on!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
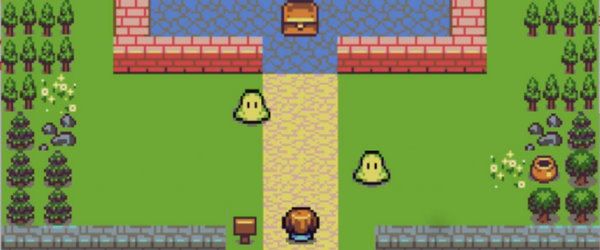
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.