We are stepping into an exciting world today, the realm of C#. It’s a world that beckons beginners and seasoned coders alike with its vast potential, offering an avenue to simplify complex tasks and make your coding aspirations a reality. Sit tight as we dive into this programming language, unveiling its features, purposes, and why it’s an essential tool in a coder’s toolkit.
Table of contents
Getting to Know C#
So, what is C#? Well, C# (pronounced ‘C Sharp’) is a powerful, versatile and modern programming language developed by Microsoft. It is primarily used for building Windows desktop applications and games, but its versatility allows it to be used in web and mobile application development as well.
The Purpose of C#
This dynamic language lets you create pretty much anything! From building immersive games on Unity (one of the largest gaming engines on the planet), to coding powerful server-side applications and services with ASP.NET, C# is your ticket to a whole new dimension of programming.
Why Master C#?
Why should you learn C#? Here are some compelling reasons:
- Power and Flexibility: C#’s rich library and wide-ranging capabilities make it a powerful and flexible language that can be used across platforms.
- Highly Valued: Due to its wide usage across different fields, C# skills are highly valued in the job market.
- Gateway to Game Development: For those excited by the prospect of game creation, C# is the primary language used in Unity game development.
It’s not an exaggeration to say that a solid understanding of C# is your key to unlocking a universe of coding possibilities.
Basic Syntax and Variables
Let’s start our hands-on journey with C# by understanding its basic syntax and variables.
In C#, every statement ends with a semicolon (;). Take a look at this simple code:
Console.WriteLine("Hello, Zenva!");
This line of code, when executed, will print the message ‘Hello, Zenva!’ to the console.
Moving on to variables, these are just named storage spaces where our program can store data. In C#, every variable has a type, a name, and a value. Here’s how you declare a variable:
int num1 = 10;
This line of code declares a variable named ‘num1’ of type ‘int’ (integer), and assigns it the value 10.
Data Types and Operators
In addition to integers, C# has a wide variety of other data types:
double d1 = 20.5;
string str = "Zenva";
bool isLearning = true;
These lines declare a double (floating point number), a string (text), and a boolean (true/false) respectively.
Let’s look at some basic mathematical operations using C# operators:
int sum = num1 + 5; int diff = num1 - 5; int product = num1 * 5; int quotient = num1 / 5; int remainder = num1 % 5;
These operations use the addition (+), subtraction (-), multiplication (*), division (/), and modulus (%) operators respectively.
Control Structures
Control structures help us manage the flow of our code. Let’s look at the ‘if’ statement:
if (num1 > 0) { Console.WriteLine("The number is positive."); }
In this code, if ‘num1’ is greater than 0, then the message “The number is positive.” will be printed.
Similarly, we have ‘loops’ to execute a block of code multiple times. Check out this ‘for’ loop example:
for (int i = 0; i < 5; i++) { Console.WriteLine(i); }
This code will print numbers 0 to 4 to the console.
All these examples just scratch the surface and there is much more to learn about C#. We know it can seem overwhelming at first, but remember – practice makes perfect. As you write more and more code, these concepts will become clear and intuitive. Happy coding!
Methods and Functions
Methods and functions in C# are blocks of code designed to perform a particular task. Packages of reusable code save us time and can ensure accuracy as they reduce repetition.
Here’s a simple C# method:
void SayHello() { Console.WriteLine("Hello, Zenva!"); }
This ‘SayHello’ method, when called, will print the message ‘Hello, Zenva!’ to the console.
Here’s how to call a method in C#:
SayHello();
Functions With Return Values and Parameters
Not all methods are created equal; some return values and accept parameters.
Here’s a C# function that adds two numbers:
int AddNumbers(int num1, int num2) { int result = num1 + num2; return result; }
In this snippet, ‘AddNumbers’ is a function that accepts two integer parameters, ‘num1’ and ‘num2’. It adds these numbers together and then returns the result.
Here’s how you would call that function and print the result:
int sum = AddNumbers(10, 5); Console.WriteLine(sum); // Outputs 15
Handling Errors with Try/Catch Blocks
Error handling is a key aspect of any programming language, and C# is no exception. We can use try/catch blocks to gracefully handle errors.
try { int quotient = 10 / 0; } catch (DivideByZeroException e) { Console.WriteLine("Cannot divide by zero"); }
In this code snippet, attempting to divide by zero will cause a ‘DivideByZeroException’. However, thanks to our try/catch block, instead of crashing, this error is caught, and a sensible message is outputted to the console.
Remember, when diving into C#, having a clear understanding of its syntax and features is essential. However, like every programming language, it is a tool that’s as powerful as the hands guiding it. Therefore, practice what you’ve learned, play with the code, shape it to your needs, and watch as the magic happens. True mastery comes with time and consistent practice. Happy Learning!
Working with Arrays
Let’s explore arrays in C#, a data structure that can store fixed-size sequential collections of elements of the same type.
Declaring an array in C# is straightforward:
int[] arr = new int[3];
This line creates an array named ‘arr’ of size 3.
Assigning values to an array looks like this:
arr[0] = 10; arr[1] = 20; arr[2] = 30;
And to access the values:
Console.WriteLine(arr[0]); // Outputs 10
Simplified Array Initialization
We can use an even more simplified array initialization:
int[] arr = new int[] {10, 20, 30};
Here, we are declaring the array and initializing it with values in a single line.
Looping Over an Array
A ‘foreach’ loop is considered a more elegant way to iterate over an array:
foreach (int num in arr) { Console.WriteLine(num); }
This loop will print all elements in the array to the console.
Working with Lists
Now, let’s use the List collection which is more flexible than an array. Here’s how you declare a list:
List list = new List();
Adding elements is easy:
list.Add(10); list.Add(20); list.Add(30);
And removing elements is just as straightforward:
list.Remove(20);
With C#, you’ve got a lot of control in your hands and how you wield that power is totally up to you. It’s important to continually expand your knowledge, so be sure to research more on C# topics like classes, objects, inheritance, and more. Always keep practicing and pushing your code boundaries. Happy Coding!
Continuing Your C# Journey
Now that we’ve scratched the surface of the C# world, your coding journey is only just beginning. Keep in mind that continued learning is the key to mastery, and remember to apply these basics in different contexts for a better understanding.
With your newfound knowledge of C#, consider defending yourself against AI invaders, creating 2D puzzles, or getting completely immersed in VR—all with the power of Unity, one of the world’s most popular game engines! Hone your skills further with our comprehensive Unity Game Development Mini-Degree. The mini-degree covers a plethora of topics such as game mechanics, animation, audio effects, and more, all suitable for beginners and experienced developers alike.
Need even more game development content? You can gain access to a wide variety of Unity courses in our broader Unity collection.
At Zenva, we support over 250 beginner to professional courses in programming, game development, and AI. Discover that stepping up your skills can be not just an education, but also a fun adventure. So continue to explore, learn, and create with us. Happy Coding!
Conclusion
In this journey through the captivating world of C#, you’ve learned the basics and delved into crucial aspects such as syntax, data types, control structures, methods, functions, arrays, and lists. You’ve seen how this powerful language can extend from creating engaging games to crafting impactful server-side applications.
The potential rewards of mastering C# are substantial. As you continue to build on your skills, don’t forget that the sky is the limit. Now is the time to turn ideas into reality, and we at Zenva hope to be a part of your exciting coding journey. Continue learning with us through our comprehensive Unity Game Development Mini-Degree. Let’s keep creating and coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
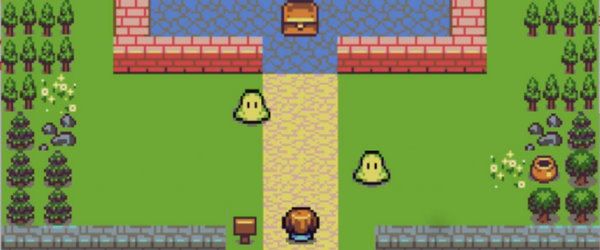
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.