Are you learning C# and have come across an error message that says, “Identifier expected”? You’re not alone. This is a common error experienced by those using this versatile and popular language, but fortunately, it comes with a straightforward solution.
Table of contents
Understanding Identifier Expected Errors
The term “identifier expected” is a type of error message in C# that signifies the compiler is expecting an identifier that doesn’t exist or isn’t defined. An identifier in C# could be anything named by the user, such as a variable, class, function, or method.
Why Do We Get Identifier Expected Errors?
These errors often result from typographical errors, failing to declare an identifier, or using reserved keywords as an identifier. Understanding and fixing these errors are part and parcel of learning C#, and doing so will help make you a better programmer in the long run.
The Importance of Identifiers in C#
Identifiers are a crucial part of C# and programming in general. They help in creating, calling, and making references to various elements of the code. By getting a handle on these common identifier expected errors, you can write cleaner and more effective code.
Common Identifier Expected Error Examples
There are many situations where you might encounter an “Identifier expected” error in C#. Let’s explore each of these scenarios with samples of code.
1. Renaming identifier with a reserved keyword
When you use a keyword as an identifier, the C# compiler will not recognize it as such and throw an error. For example:
int int = 5;
Here we have tried to use ‘int’, which is a keyword, as a variable. To fix this error, we would need to give the identifier a unique name like this:
int num = 5;
2. Not defining an identifier
If you call an identifier that’s not been defined, you’re also likely to see an identifier expected error.
Console.WriteLine(x);
In this code, we’re trying to print a variable ‘x’ that’s not been defined. Here’s the corrected version:
int x = 10; Console.WriteLine(x);
3. Syntax errors
A slight syntax error, such as missing or misplaced characters, can also cause this error message.
int a = 5
In this example, the error arises because of the missing semicolon at the end. Corrected code:
int a = 5;
4. Naming identifiers incorrectly
Identifiers in C# must adhere to certain rules. An identifier must start with a letter, an underscore, or a character followed by letters, digits, or underscores.
int 9lives = 9;
The above identifier is incorrect because it starts with a digit. A correct example would be:
int _9lives = 9;
By keeping these examples in mind and understanding where you went wrong, you can easily overcome the “identifier expected” obstacle and become more proficient in C# programming.
5. Using special characters in identifiers
C# does not allow the use of special characters, except for underscores, in identifiers. Let’s look at an incorrect and correct implementation:
int my-number = 10;
This, as you might guess, would produce an error since we have used a hyphen, which is a special character. A correct version could be:
int my_number = 10;
6. Using whitespaces in identifiers
Using whitespaces in identifiers is not allowed in C#:
int my number = 10;
As above, this would throw an error. We can instead use underscores or employ camelCase to separate words in an identifier:
int my_number = 10; int myNumber = 10;
7. Using namespaces without the “using” keyword
In C#, we are required to use the “using” keyword while using namespaces in order to avoid any confusion:
System.Console.WriteLine("Hello, World!");
Here we’ve used the ‘System’ namespace without the ‘using’ keyword, and hence, we will encounter an error. Here’s how to correct it:
using System; Console.WriteLine("Hello, World!");
By understanding and implementing these fixes, you can easily avoid “identifier expected” errors in your C# code and ensure smoother programming.
C# Identifier Best Practices
Now that we’ve looked at how to fix these errors, it’s also worthwhile accustoming ourselves to some best practices when working with identifiers in C#. By doing so, we can avoid encountering these errors in the first place:
Always use meaningful names for identifiers. This makes it easier to understand the purpose of an identifier and is especially helpful when working in a team context.
Avoid using singly named variables such as ‘a’, ‘b’, ‘x’ except for loop indices and temporary variables.
Do not use special characters and blank spaces in your identifiers.
Do not use C# keywords as identifiers.
By familiarizing yourself with these conventions and understanding the errors and their solutions, you are poised to be more proficient in C# programming.
8. Using Case-Sensitive Identifiers
C# is case-sensitive, and therefore, using different cases for the same identifier can lead to an error.
int myNumber = 1; Console.WriteLine(MyNumber);
In the code above, ‘myNumber’ and ‘MyNumber’ are treated as two different identifiers. To correct this issue, you’ll need to ensure the case is consistent:
int myNumber = 1; Console.WriteLine(myNumber);
9. Over-parenthesizing identifiers
Using an unnecessary extra set of parentheses while naming or calling an identifier can throw an error:
int (myNumber) = 5;
Basically, parentheses should only enclose expressions or method arguments. A correct example would be:
int myNumber = 5;
10. Using the same identifier name in the same scope
Reuse of the same identifier name in the same scope will also give you an error:
int myNumber = 5; int myNumber = 10;
In the above example, we’ve used the same variable name ‘myNumber’ twice in the same scope, which is not allowed. A correct implementation would be to either use a different identifier name or change the values within the same identifier:
int myNumber = 5; myNumber = 10;
11. Using unassigned local variables
If you declare a local variable and try to use it without first assigning it a value, this will also cause an error:
int myNumber; Console.WriteLine(myNumber);
In this example, we’re trying to print the value of ‘myNumber’ which hasn’t been assigned yet. Here’s how to assign a value before using the identifier:
int myNumber = 5; Console.WriteLine(myNumber);
These examples should provide a solid foundation to understand and identify “identifier expected” errors in C#. Remember, the key is to understand the syntax and rules for identifiers in C#. With practice, you’ll minimize these errors, and your overall coding efficiency will upgrade significantly.
Where To Go Next: Enhancing Your C# and Unity Skills
Now that you have a solid understanding of “identifier expected” errors in C#, you’re well on your way to becoming a more efficient programmer. But don’t stop there! Continue enhancing your coding skills and creating amazing things.
We highly recommend our Unity Game Development Mini-Degree. This thorough set of courses covers a lot more than just C# programming; it delves into the Unity engine, which is used for creating games in 2D, 3D, AR, and VR. It’s a popular choice among both AAA and indie developers worldwide. The Mini-Degree will lead you through a variety of topics, including game mechanics, audio effects, cinematic cutscenes, and much more, augmented by project-based assignments.
With more than 250 courses to choose from, there’s something for everyone at every level of expertise at Zenva. Check out our Unity Collection for an even broader range of learning opportunities. Dedication and continuous learning are the key to success in the tech industry. So, keep coding, keep learning, and keep growing with us!
Conclusion
Mastering the art of identifying and resolving “identifier expected” errors propels you further on your journey to becoming a proficient C# programmer. Embrace these challenges as valuable learning moments that contribute to your growth and understanding of coding concepts.
At Zenva, we believe in nurturing your passion for learning and guiding you in your quest for knowledge. As you continue your adventure with C#, remember that our Unity Game Development Mini-Degree is here to expand your horizons even further. Continue exploring, continue learning, and take your programming skills to the next level with us.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
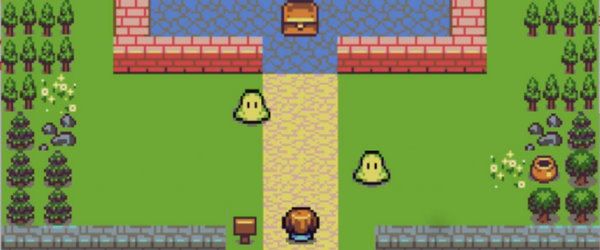
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.