In the ever-evolving world of coding and game development, understanding core elements of a language like C# becomes paramount. A fundamental component to grapple with is the `int32` data type. As we delve into the fascinating realm of `int32`, you will find its versatility and pivotal role in game mechanics to be both engaging and invaluable.
Table of contents
What is `int32`?
`Int32` is a value type in C#. It is a signed integer data type that can hold values ranging from -2,147,483,648 to 2,147,483,647. Capable of storing a fair amount of data, `int32` is robust and versatile.
What is it used for?
Being one of the base data types in C#, `int32` permeates every corner of programming in the language. It is primarily used to store numeric values and can be instrumental in controlling game elements like player scores, enemy counts, or level markers in games.
Why learn `int32`?
As a fundamental data type in C#, understanding `int32` opens doors to higher complexity codes and can significantly improve your coding fluency. Furthermore, knowing how to correctly utilize `int32` can help optimize your game’s performance by ensuring you are not unnecessarily consuming memory resources.
Working with `int32` – Basic examples
Understanding how to declare and manipulate an `int32` variable forms the cornerstone of your C# learning journey. Let’s explore the same through the following examples:
First, declaring an `int32` variable can be as simple as:
int number = 999;
You can even declare multiple `int32` variables in a single line as shown below:
int number1 = 500, number2 = 200, number3 = 300;
Manipulating `int32` variables involves operations like addition, subtraction, multiplication, or division. See the examples below:
int number1 = 20; int number2 = 10; // Addition int result = number1 + number2; // result is now 30 // Subtraction result = number1 - number2; // result is now 10 // Multiplication result = number1 * number2; // result is now 200 // Division result = number1 / number2; // result is now 2
Further Operations with `int32`
Apart from basic arithmetic operations, you can perform other significant operations like modulo operation or increment/decrement using `int32`.
The modulo operation gives the remainder of a division operation:
int number1 = 15; int number2 = 4; // Modulo int result = number1 % number2; // result is now 3
The increment and decrement operators add or subtract 1, respectively, from the variable value:
int number = 50; number++; // number is now 51 number--; // number is now 50 again
In the next section, we’ll put all these basics to the test with more complex examples. Stick with us to improve your mastery over `int32`!
Delving Deeper – `Int32` in Real World Scenarios
As we step up a bit further into the learning curve, let’s look at how `int32` can be effectively used in more complex programming scenarios. Remember, as a coder, your creativity is boundless when it comes to using `int32` to solve challenging problems!
Determining the maximum or minimum of two numbers:
int number1 = 30; int number2 = 50; int max = (number1 > number2) ? number1 : number2; // Output: The maximum number is 50 Console.WriteLine($"The maximum number is {max}");
In this example, we have used the conditional (ternary) operator to detect the higher number.
Using `int32` in loops:
for (int i = 0; i < 5; i++) { Console.WriteLine(i); }
Here, we utilize `int32` as a counter in a for loop, essential when you want to iterate over a block of code a certain number of times.
Handling exceptions with `int32.TryParse`:
string input = "1234"; int number; bool success = Int32.TryParse(input, out number); if (success) { Console.WriteLine("Conversion successful"); } else { Console.WriteLine("Conversion failed"); }
When dealing with user inputs or data from external sources, it’s crucial to ensure the conversion to `int32` is successful. `int32.TryParse` is a useful tool in your toolkit for this endeavor.
Converting `int32` to other data types:
int number = 200; // convert to double double converted = Convert.ToDouble(number); // convert to string string s = number.ToString();
In some cases, you might need to convert `int32` to other data types. The above examples demonstrate how you can do it.
There you have it! By now you should have a pretty solid understanding of `int32` in C#. We’ll continue this journey into C# and its intricacies, delving into other essential topics in future write-ups.
In the meantime, why not put your newfound knowledge to the test? Build something using `int32`, share it with the community, and enhance your learning experience! Happy coding!
Advanced `int32` Operations and Usage
As we continue to delve deeper into the powerful `int32` data type, let’s take a look at some advanced usage cases and operations. This will include working with arrays of `int32`, looping through such arrays, and employing bitwise operators.
Creating and initializing an `int32` array:
int[] numbers = new int[5] {10, 20, 30, 40, 50};
This code snippet creates an `int32` array with five elements.
Looping through an `int32` array:
int[] numbers = new int[5] {10, 20, 30, 40, 50}; foreach(int number in numbers) { Console.WriteLine(number); }
In this example, we use a `foreach` loop to iterate over an `int32` array, printing each number to the console.
The Bitwise AND Operator (`&`):
int number1 = 12; // in binary: 1100 int number2 = 25; // in binary: 11001 int result = number1 & number2; // result is 8 (in binary: 1000)
Bitwise operators act on the binary representations of `int32` values. The Bitwise AND (`&`) operator gives a 1 in each bit position where both corresponding bits are 1.
The Bitwise OR Operator (`|`):
int number1 = 12; // in binary: 1100 int number2 = 25; // in binary: 11001 int result = number1 | number2; // result is 29 (in binary: 11101)
The Bitwise OR (`|`) operator gives a 1 in each bit position where at least one corresponding bit is 1.
The Bitwise XOR Operator (`^`):
int number1 = 12; // in binary: 1100 int number2 = 25; // in binary: 11001 int result = number1 ^ number2; // result is 21 (in binary: 10101)
The Bitwise XOR (`^`) operator gives a 1 in each bit position where exactly one corresponding bit is 1.
Right Shift (`>>`) and Left Shift (`<<`) Operators:
int number = 12; // in binary: 1100 // Right Shift Operator int result = number >> 2; // result is 3 (in binary: 11) // Left Shift Operator result = number << 2; // result is 48 (in binary: 110000)
The right shift (`>>`) operator shifts all bits towards the right by a certain number of specified bits. The left shift (`<<`) operator, on the other hand, shifts all bits towards the left.
Armed with these advanced `int32` operations and usage examples, you are well on your way to taking your mastery of this fundamental data type to new heights. Continue to practice using `int32` in more advanced scenarios, and don't be afraid to challenge yourself with complex projects. Happy coding!
Where to Go Next?
Congratulations on your progress so far! You’ve taken important strides in understanding `int32` and how it works in C#. As you delve deeper into the path of game development, this knowledge will be an invaluable asset. But your learning journey shouldn’t stop here. Continual involvement and practice are essential in honing your skills and unlocking new avenues in both coding and game creation.
Are you interested in putting your C# coding skills into practice for game development? We invite you to explore our Unity Game Development Mini-Degree. It is a comprehensive collection of courses that introduce game development using Unity, one of the leading game engines in the world. The program covers various aspects such as game mechanics, cinematic cutscenes, and audio effects. By completing the Mini-Degree, you can build a portfolio of Unity games and projects, paving the way for rewarding career opportunities in the game industry.
For a broader look at our offerings, check out our full range of Unity courses. You’ll find a vast selection suitable for both beginners and experienced developers alike.
Remember, with Zenva, you can go from beginner to professional, build your portfolio, and boost your career prospects. Keep up the great work and continue learning. Your game development journey has only just begun!
Conclusion
Mastering `int32` and other fundamental concepts in C# are pivotal stepping stones in your coding journey. Each new topic you conquer takes you one step closer to creating the PC games, mobile apps, VR experiences, or AR interiors you’ve always dreamed of. Remember that each new line of code you write enhances your proficiency, takes you deeper into the captivating world of game development, and endows you with an empowering sense of achievement.
Your next move on this exciting journey? Well, why not put your `int32` skills into action with our Unity Game Development Mini-Degree? The program provides you with a variety of hands-on projects to further hone your C# skills and create your own games. With Zenva, the game development world is your oyster, and we’re here to guide you every step of the way. Happy learning!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
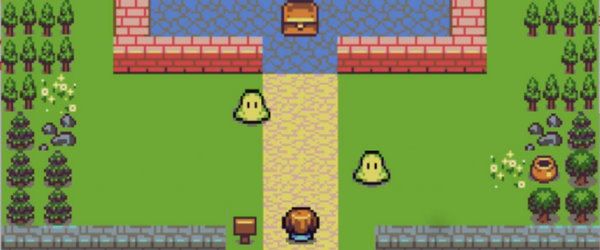
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.