In the fast-paced world of software development, managing the changes to code effectively is crucial. Whether you’re embarking on your first programming venture or you’ve been coding for years, understanding Source Code Management (SCM) can revolutionize the way you work on projects. It’s a fundamental tool that ensures stability, collaboration, and a historical record of your work all in one. This tutorial aims to unveil the mysteries of SCM, making it an accessible and invaluable resource in your coding arsenal.
Table of contents
What is Source Code Management?
Imagine working on a complex puzzle with many pieces constantly shifting. Now, envision having an assistant who meticulously records each move you make, allowing you to step back and analyze, or revert any change if needed. SCM acts as this meticulous assistant for your code. It’s a system that tracks modifications in computer programs, allowing multiple developers to work on different aspects of the same project without conflict.
What is SCM for?
SCM is used for version control – it manages changes to documents, computer programs, large websites, and other collections of information. With the immense amount of detail in modern software projects, keeping track of what was changed, when, and by whom becomes an essential practice. This allows for collaborative work environments, where features can be developed, bugs fixed, and experiments conducted alongside the main codebase without risking its integrity.
Why Should I Learn SCM?
- Efficient Collaboration: SCM systems allow multiple developers to work seamlessly together, merging their changes into a shared codebase.
- History and Documentation: Every change is logged with a timestamp and authorship information, making it easy to track the evolution of the software and understand the reasoning behind each piece of code.
- Error Control: If an error slips through, SCM can help you easily identify and revert the problematic changes, minimizing the impact of mistakes.
- Branching and Merging: Feature branches allow developers to work on new capabilities without disturbing the main code, merging them only when they’re ready for prime time.
Source Code Management is more than a safety net; it’s an enabler of creative and technical freedom, ensuring even the most complex projects can be tackled with confidence. Let’s delve into the practical applications and see SCM in action with Python as our companion for code examples.
Setting Up a Repository
To begin using Source Code Management with Python, the first step is to set up a repository. A repository is like a project folder for your code, but with SCM capabilities. Here’s how you can do it using Git, one of the most popular SCM systems:
git init
Running git init
in your project’s root directory initializes a new Git repository. After initializing the repository, we need to tell Git which files to track.
git add git add .
The first command adds a specific file to the staging area, while the second one adds all files in the directory. Remember, changes are not recorded until you commit them:
git commit -m "Initial commit"
Committing Changes
As you make changes to the code, you need to commit these changes to record them in your repository’s history. Here’s how to commit a new feature in your Python code:
# Let's say you've added a new function to your Python script. def new_feature(): print("This is a new feature.") # You would then track changes and commit them. git add my_script.py git commit -m "Add new_feature function"
Each commit has a message that describes what you did, which is useful for documentation purposes.
Inspecting the History
One of the key advantages of using SCM is the ability to inspect the history of your project. With Git, you can view the commit log to see what changes have been made:
git log
For a more condensed view of the log, showing one line per commit, you might use:
git log --oneline
To view the actual changes made, you can use the git diff command:
# To view unstaged changes to your code git diff # To view the changes that are staged but not yet committed git diff --staged
Branching and Merging
Branches are essentially parallel versions of your code. You can develop new features in isolation and later merge them back into the main branch. Here’s how to create a new branch and switch to it:
git branch new_feature git checkout new_feature
You can also combine these two commands into one:
git checkout -b new_feature
Once your feature is complete, you can merge it back into your main code. Note that you should switch to the branch you want to merge into first:
git checkout main git merge new_feature
Merging can sometimes result in conflicts when the same part of the code was changed on both branches. You will need to manually resolve these conflicts before completing the merge.
These foundational SCM commands lay the groundwork for efficient and controlled development. In the next part of our tutorial, we’ll delve into more advanced SCM features and how they can further enhance your workflow.
As we dive deeper into the world of Source Code Management with Git, it’s vital to understand how to make use of the following advanced features. These will help refine your development process and enhance your ability to handle complex project scenarios. We’ll explore examples such as reverting changes, stashing your work, handling remote repositories, and tagging important milestones.
Reverting Changes
Sometimes, you’ll make changes that you later decide to undo. Git allows you to revert changes that you’ve committed. This can be done by using the commit’s hash (which you can find using git log
):
git revert 1a2b3c4d
A new commit will be created that undoes the changes from the specified commit. If you need to undo uncommitted changes, git checkout
can help:
git checkout -- my_script.py
This will revert changes to the file back to its last committed state.
Stashing Changes
When you need to switch branches but aren’t ready to commit your current work, you can use git stash
. This will remove the changes and store them so you can reapply them later:
git stash git checkout other_branch # do some work on other_branch git checkout original_branch git stash pop
The git stash pop
command applies the stashed changes back to your working directory.
Working with Remote Repositories
Often, you’ll want to store your code remotely (for example on GitHub) for backup or sharing purposes. First, you’ll need to add a remote repository:
git remote add origin https://github.com/your_username/your_repo.git
Then you can push your code to the remote repository:
git push origin main
And fetch updates from it:
git pull origin main
If you’re working with others, you’ll frequently pull changes they’ve pushed to the remote repository to keep your local version up to date.
Tagging Releases
When you reach a milestone, such as a release, tagging allows you to mark a specific point in your repository’s history as important. Creating a tag is straightforward:
git tag v1.0.0 git push origin --tags
The first command creates a tag in the local repository, and the second command pushes the tags to the remote repository.
Advanced SCM techniques like these help you navigate coding projects with precision and confidence. Incorporating them into your development routine will significantly improve your control over the codebase and collaboration with team members. Stay tuned for our next section, where we’ll tie all these concepts together and explore how to overcome common source code management challenges.
Now that we’ve seen how to manage your source code using Git, let’s continue by exploring additional commands and functionalities that can help tackle common challenges in software development. We’ll walk through the examples involving the inspection of changes, handling complex merges, syncing your repository, and dealing with tags and branches.
To begin with, let’s delve into a powerful Git tool, the git bisect command, which can be used to find the commit that introduced a bug:
git bisect start git bisect bad # mark the current commit as bad git bisect good commit-hash-here # mark the last known good commit # Git will now automatically checkout a commit halfway between the good and bad commits # Test this commit, then mark it as 'good' or 'bad' and Git will continue by halving the range git bisect good # or 'git bisect bad', depending on the test results # Once the culprit is found, reset the bisect session git bisect reset
When it comes to handling complex merges, it’s essential to understand merge strategies. Sometimes a merge conflict can’t be automatically resolved and requires manual intervention. In such cases, you’ll see something like this:
<<<<<<< HEAD your changes here ======= someone else's changes here >>>>>>> feature_branch
You’ll need to decide which changes to keep, make the necessary edits, remove the conflict markers, and then add and commit the resolved file:
git add resolved_file.py git commit -m "Resolve merge conflict by incorporating both features"
Syncing your repository with remote changes is another critical habit. You can fetch updates without merging them using the git fetch command:
git fetch origin
This command updates your remote-tracking branches. It’s a safe way to see what others have committed. You can then merge these fetched changes into your branch:
git merge origin/main
Alternatively, if you want to fetch and merge in one go, you can use git pull:
git pull origin main
This will fetch the changes from the origin’s main branch and merge them into your current branch.
Managing tags and branches efficiently also plays a significant role in a well-maintained repository. To delete a remote tag that’s no longer needed:
git push origin --delete <tagname>
For deleting a local branch that has been merged and is no longer in use:
git branch -d <branchname>
If the branch hasn’t been merged and you still want to delete it, a forceful approach is needed:
git branch -D <branchname>
Remember, Source Code Management is as much about the tools you use as it is about the practices you implement. Staying organized, committing often with clear messages, keeping your work synced, and cleaning up tags and branches are all best practices that lead to a clean and efficient project workflow. By mastering these practices and commands, you’ll be well-equipped to handle even the most demanding software development challenges.
Continuing Your Programming Journey with Zenva
The landscape of programming is vast and constantly evolving. Mastering Source Code Management is just the beginning. If you’re keen to elevate your skills further and dive deeper into programming, especially with Python, Zenva’s Python Mini-Degree is an excellent next step to consider. This comprehensive suite of courses takes you through from the basics to more advanced topics, providing a well-rounded education in this powerful and versatile language. Ready to expand your knowledge and challenge yourself? Explore the Python Mini-Degree here.
For those looking to broaden their expertise beyond Python, our range of Programming courses cover a multitude of technologies and skills which can help you stay ahead in the fast-paced world of technology. Whether iOS app development or data science pique your interest, Zenva has got you covered with over 250 courses designed to take your abilities from beginner to professional. Begin exploring all the possibilities and fortify your programming prowess with our curated courses here.
At Zenva, we champion self-paced learning, giving you control over your educational journey. The practical, project-based curriculum allows you to build a portfolio as you learn, making your newfound skills as tangible as they are valuable. So, wherever you are in your programming journey, remember that with Zenva, you can go from beginner to professional. Keep coding, keep creating, and never stop learning.
Conclusion
Embarking on your coding odyssey equipped with the knowledge of Source Code Management is like setting sail with a compass and a map. It ensures that no matter how turbulent the waters of software development become, you can navigate through with confidence and collaboration. As you continue to build, innovate, and create, SCM will be a steadfast ally in preserving your work’s integrity and fostering a harmonious coding environment.
Remember, this is only the beginning. With resources like Zenva’s Python Mini-Degree, as well as a plethora of other programming courses at your fingertips, your journey towards mastery is just one click away. Keep pushing boundaries and leveling up your skill set – your future self will thank you for the investment in knowledge. Explore the Python Mini-Degree and propel your programming career to new heights here.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
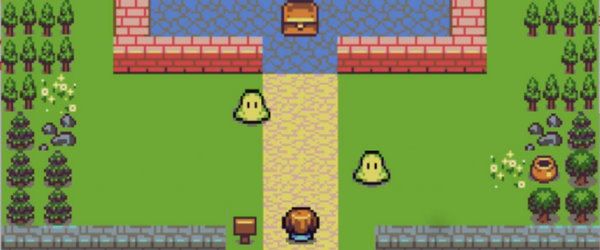
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.