Whether you are just starting your programming journey or you’re a seasoned coder, one thing remains universally essential – the text editor. It’s the arena where ideas become code, and code becomes a functioning masterpiece. Navigating the ocean of programming requires a trusty vessel, and the text editor is just that. It’s the tool that allows you to write, refine, and bring your imaginative concepts to life. If you’re diving into the world of development, understanding and mastering your text editor can elevate your coding skills, efficiency, and enjoyment.
Table of contents
What is a Text Editor?
A text editor is a type of program that allows you to edit plain text—essentially the most basic form of data on a computer. But don’t let its simplicity fool you; for programmers, it’s a powerful workspace for writing and editing code. Unlike word processors, text editors don’t add formatting to text, which is crucial when you’re dealing with programming languages.
What is it Used For?
Text editors serve a multitude of purposes in programming. They enable developers to craft code from scratch, debug existing code, and sometimes manage files and directories. Modern editors also offer syntax highlighting, which makes it easier to distinguish elements of the code, and other helpful tools like line numbering, text searching, and code formatting.
Why Should I Learn to Use it Effectively?
Learning to use a text editor effectively is akin to sharpening your sword before a battle. It can dramatically enhance your coding efficiency by allowing you to navigate and manipulate code faster and with more accuracy. Moreover, knowing how to use the different features and shortcuts available can reduce development time and increase the quality of your work.
Now, let’s step into the world of programming with text editors as we dive into the following sections full of code examples and engaging discussions.
Opening and Creating Files
To begin using your text editor, you must know how to open and create new files. Here’s how you can do it in a command-line based text editor like Vim:
:e filename.txt // Opens an existing file named filename.txt :enew // Opens a new file in a new buffer
In graphical text editors, you can typically find these options under the ‘File’ menu, or you can use shortcut keys like:
Ctrl + O // Open an existing file Ctrl + N // Create a new file
Basic Text Manipulation
Text manipulation is essential for coding. You’ll frequently need to write, delete, cut, copy, and paste text:
i // Inserts text before the cursor (Vim) a // Appends text after the cursor (Vim) dd // Deletes the current line in Vim yy // Copies the current line in Vim p // Pastes copied or cut text after the cursor in Vim
In most GUI text editors, you can use standard shortcuts:
Ctrl + X // Cut selected text Ctrl + C // Copy selected text Ctrl + V // Paste clipboard contents Ctrl + Z // Undo last action Ctrl + Y // Redo last undone action
Searching and Replacing Text
Finding and replacing text is crucial when working with large code files. Here’s how Vim does it:
/text_to_find // Searches for text_to_find in the document :%s/old/new/g // Replaces all occurrences of 'old' with 'new' globally
In GUI text editors, you can usually find and replace text with:
Ctrl + F // Open the 'Find' dialog box Ctrl + H // Open the 'Replace' dialog box
Code Navigation
Moving through your code quickly is pivotal when you’re editing. Knowing these shortcuts can save you a ton of time:
gg // Goes to the first line of the file in Vim G // Goes to the last line of the file in Vim :n // Goes to line number 'n' in Vim Ctrl + G // Shows your current position in the file in Vim
In graphical text editors, you often navigate code with arrow keys, page up/down, or with the mouse scroll wheel. Some also allow jumping to a specific line with:
Ctrl + G or Ctrl + L // Open a 'Go to line' dialog
Stick to these examples and practice them thoroughly. They form the foundation of efficient text editing and will be used over and over again as you program. In the next section, we’ll dive into more advanced features and techniques that can further enhance your coding capabilities.
In continuing with our exploration of text editors, we delve into more advanced features that can significantly streamline your development process. Master these, and you’ll be wielding your text editor like a pro.
Working with Multiple Files
Often, you’ll need to work with more than one file at a time. Text editors like Vim allow you to manage multiple files using buffers, windows, and tabs:
:e file2.txt // Edit another file in a new buffer :bn // Go to the next buffer :bp // Go to the previous buffer :split file2.txt // Split the window and open file2.txt :tabnew file2.txt // Open file2.txt in a new tab
Graphical text editors usually offer tabbed interfaces where you can click to switch between open files, or use shortcuts like:
Ctrl + Tab // Cycle through open files Ctrl + Page Up // Switch to the previous file Ctrl + Page Down // Switch to the next file
Advanced Text Manipulation
Beyond basic text manipulation, you should be familiar with powerful tools like multi-line editing and macros:
Ctrl + V // Start visual block mode in Vim, then I to insert before, A to append after q{register} // Start recording a macro in Vim to the specified register (e.g., qa to start recording to register 'a') q // Stop recording macro in Vim @{register} // Execute macro (e.g., @a for the macro recorded to register 'a')
In GUI editors like Sublime Text or Visual Studio Code, multi-cursor and macro features can dramatically speed up repetitive tasks:
Ctrl + D // Select the next instance of the current selection Alt + Click // Add a new cursor at the clicked location Ctrl + Shift + P, "record macro" // Start recording a macro Ctrl + Shift + P, "run macro" // Run the recorded macro
These advanced manipulations allow for quick changes across large swathes of code without tedious repetition.
Using Snippets and Code Completion
Snippets are small blocks of reusable code that you can insert into your larger code files. Code completion, on the other hand, predicts and suggests possible completions for partially typed words. These features help prevent syntax errors and save time:
{trigger} + Tab // Insert a snippet where {trigger} is the snippet shortcut Ctrl + Space // Trigger code completion in many graphical editors
Most editors come with pre-defined snippets for common tasks, and you can often create your own for repeated patterns specific to your workflow.
Version Control Integration
Lastly, integration with version control systems like Git is a staple of modern text editors. Directly within your editor, you can perform actions like committing changes and reviewing the history of your project:
:Gstatus // Check the Git status in Vim (with fugitive plugin) :Gcommit // Commit changes in Vim (with fugitive plugin) Ctrl + Shift + G // Open Git panel in Visual Studio Code
This integration streamlines your workflow, keeping all your tools within reach in a central environment.
As you continue to develop your coding skills, leverage these text editor capabilities to increase your productivity. After all, efficient use of your editor is a key step in becoming a proficient programmer. We, at Zenva, value the importance of well-rounded coding expertise, and mastering your text editor plays a significant part in that. Keep practicing, exploring features, and configuring your environment to fit your personal coding style – it’s an investment that will pay dividends throughout your coding journey.
As you grow accustomed to the basic and advanced features of text editors, delving into even more sophisticated functionalities will help you harness the full potential of your chosen editor. In this section, we’ll touch upon several powerful features, supported by appropriate code examples, that can further enhance your productivity and coding prowess.
Regular Expressions and Pattern Matching
Regular expressions (regex) are sequences of characters that form a search pattern, often used for string searching and manipulation. Here’s how you might employ regex within Vim:
/\d+ // Find one or more digits :%s/^[ \t]*//g // Remove leading whitespace from every line /\v // Find an email address pattern using very magic mode
Graphical editors also offer regex search abilities accessible through their search functionalities:
Ctrl + F, Toggle Regex // Enable regex mode and then search patterns
Understanding and using regex can massively accelerate text manipulation tasks and complex searches in your projects.
Customizing the Text Editor
Personalizing your text editor ensures that it aligns with your workflow and preferences, potentially reducing friction and fatigue. Here’s how you can configure the Vim environment:
:syntax on // Enable syntax highlighting :set number // Show line numbers :colorscheme darkblue // Set the color scheme to 'darkblue' :set autoindent // Enable auto-indentation
In GUI editors, customization options are usually found in settings menus, and many allow you to install themes and extensions for added functionality:
File > Preferences > Settings // Access settings (Visual Studio Code example) Extensions > Search for 'Theme' // Search for themes (Visual Studio Code example)
Learning Editor-Specific Commands and Shortcuts
Each editor has a unique set of commands and keyboard shortcuts. Mastery of these can set you apart as an efficient developer. In Vim, you might encounter:
:set wrap // Toggle word wrapping :help {topic} // Get help on a specific topic ZZ // Save and quit
GUI editors also have their own sets of shortcuts, with many allowing customization:
Ctrl + Shift + P // Show command palette (Sublime Text, Visual Studio Code) Ctrl + B // Toggle the sidebar (Sublime Text)
Making the effort to learn and customize these editor-specific commands greatly streamlines the coding process.
Plugins and Extensions
Most modern text editors support plugins or extensions—add-ons that extend functionality. Some popular Vim plugins and their installation commands might look like this:
:PluginInstall <em>plugin-name</em> // For Vim with Vundle package manager
For graphical text editors, you might find and install extensions using the editor’s marketplace or extension library:
Extensions > Search for 'Linters' // Install linters for code quality (Visual Studio Code example)
By tapping into an ecosystem of community-contributed plugins and extensions, you can turn your text editor into an IDE customized for your specific needs and preferences.
Remember, the journey to becoming fluent in your text editor is ongoing. Commit to daily practice with real coding projects, and soon enough, these commands and shortcuts will become second nature. At Zenva, we encourage learners to immerse themselves in their development environments, as this is where the magic of creation comes to life. Keep honing your skills and exploring the depths of your text editor’s capabilities, and watch as your coding transforms from meticulous task to artful craft.
Embark on Your Python Journey
Having honed your text editor skills, you’re well-equipped to tackle the exciting challenges of programming. The next logical step in your journey is to put those skills to use by learning a versatile and powerful programming language like Python. We invite you to explore our Python Mini-Degree, where you can elevate your coding abilities from the ground up.
Our Python Mini-Degree is designed not just for beginners, but also for those who already have a grasp on the basics and want to delve deeper into programming. As you advance through the courses, you’ll build games, algorithms, and real-world applications that will both challenge and showcase your growing expertise. The flexible, device-friendly learning structure allows you to progress at your own pace, ensuring a learning experience tailored to your personal and professional goals.
For learners looking to broaden their horizons beyond Python, our diverse selection of programming courses covers a wide range of topics, tools, and languages to keep you continuously learning. By choosing Zenva, you’re joining over 1 million learners who have enhanced their careers with our courses. Your journey in coding is boundless, and we’re here to support every step of the way. Transform your dedication into development mastery with Zenva.
Conclusion
The road to mastering programming is an adventure of continuous learning and improvement, with your text editor serving as a steadfast companion along the way. At Zenva, we understand the importance of a strong foundation, which is why our Python Mini-Degree is crafted to turn novices into confident programmers, armed with the knowledge to conquer any coding challenge. Whether you’re piecing together your first lines of Python or deploying sophisticated applications, your journey with us is just beginning.
Our comprehensive curriculum ensures you’re not just learning to code—you’re learning to create and solve problems. The skills you gain here will not only make an impact in your personal projects but also propel your professional career forward. Dive into our meticulously structured content, and let us guide you to become a shaper of technology. Embrace the beauty of learning with Zenva, where your potential and our expertise come together to unlock incredible possibilities in the digital world.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
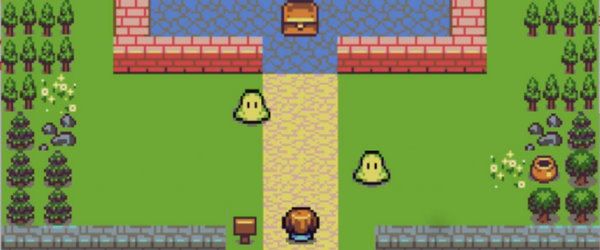
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.