Embarking on a journey into the world of programming can be as thrilling as discovering a new universe. For those who are starting to learn how to code or even seasoned developers looking to expand their toolkit, understanding the role and functionality of a Software Development Kit (SDK) is crucial. As we unravel the mysteries of SDKs, think of them as swiss army knives for your next digital creation, essential for building software efficiently and effectively.
Table of contents
What is a Software Development Kit (SDK)?
An SDK is a collection of software tools and libraries that developers use to create applications for specific platforms or devices. Like the pieces of a puzzle, an SDK includes everything you might need to complete a software project, from debugging tools to ready-to-use code samples.
What is it for?
SDKs serve a dual purpose; they not only provide the building blocks to ease the development process but also ensure that applications seamlessly integrate with the platform they’re destined for. Let’s imagine programming like constructing a robot; the SDK is the box that contains all the parts, manuals, and tools you need to assemble it, with instructions tailored specifically for a robotic arm or maybe a laser eye.
Why Should I Learn About SDKs?
Being versed in SDKs is like having a passport for worldwide travel in the land of technology. It enables you to build software across various platforms, from desktops to mobile devices, and opens up new possibilities for problem-solving and innovation. Aligning with industry standards, SDKs can propel your projects ahead, allowing you to stand on the shoulders of tech giants and achieve faster and more reliable development cycles.
A strong understanding of SDKs is an invaluable asset for aspiring developers and industry veterans alike. It ensures that your journey in software development is both current and competitive.
Now that we’ve explored the fundamentals of what an SDK is and why we should incorporate it into our learning, let’s jump into some practical examples.
Exploring the Essentials of an SDK
To truly appreciate the convenience an SDK provides, let’s start by setting up a simple development environment. Consider we’re creating a mobile application; we would need to download the respective SDK for iOS or Android. Let’s look at some fundamental components and how they can be used.
SDK Tools: These are command-line tools that let us interact with the device. For example, Android SDK comes with a tool called ‘adb’, the Android Debug Bridge. Here’s how to start the adb server and connect to a device:
adb start-server adb devices
API Libraries: These libraries provide interfaces to interact with the necessary features of the devices or platform. Suppose you’re working with the Twitter SDK; you might use its API to fetch tweets.
TwitterApiClient twitterApiClient = TwitterCore.getInstance().getApiClient(); StatusesService statusesService = twitterApiClient.getStatusesService(); Call<List<Tweet>> call = statusesService.homeTimeline(50, null, null, null, null, null, null);
Documentation and Samples: Most SDKs come with extensive documentation and sample projects to help you get started. For instance, if you are working with Google’s Firebase SDK, you might find examples like initializing Firebase in your application:
public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); // Setup Firebase FirebaseApp.initializeApp(this); } }
IDE Extensions or Plugins: SDKs may include plugins for Integrated Development Environments (IDEs) such as Visual Studio or Android Studio, to streamline the development process.
Integrating SDKs in Projects
An SDK’s value is fully realized when seen in the context of a real-world project. Let’s say we’re creating a cross-platform mobile app using Flutter. We would utilize the Flutter SDK to not only create our app but to also ensure it is compatible across multiple devices.
Here are some examples of using the Flutter SDK:
// Importing the Flutter SDK import 'package:flutter/material.dart'; // Running the app void main() => runApp(MyApp()); // Define the 'MyApp' widget class MyApp extends StatelessWidget { @Override Widget build(BuildContext context) { return MaterialApp( title: 'Welcome to Flutter', home: Scaffold( appBar: AppBar( title: Text('Welcome to Flutter'), ), body: Center( child: Text('Hello World'), ), ), ); } }
For platform-specific SDKs, you might need to include native code to use certain features that are unique to that platform. For instance, accessing the camera on an Android device:
public class TakePhotoActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_take_photo); // Intent to open camera Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(takePictureIntent, REQUEST_IMAGE_CAPTURE); } }
Last but not least, we must not forget about dependency management for these SDKs. If you’re working in a Java environment, you might use Maven or Gradle. Here’s how you can include the Google Maps SDK for Android using Gradle:
dependencies { implementation 'com.google.android.gms:play-services-maps:17.0.0' }
In the upcoming part, we will dive deeper and explore how these SDK examples interplay with the creation of fully-fledged features in an application, as well as best practices for a smoother development process.
Understanding these different facets of SDKs paves the way for us to leverage their full potential in our development projects. In a practical scenario, this might translate into invoking specific functionalities provided by the SDK to enhance our applications. Let’s delve into some more code examples to illustrate this.
Imagine you’re working on an iOS application using Apple’s SDK; you may want to implement a feature that uses the device’s accelerometer:
import CoreMotion let motionManager = CMMotionManager() if motionManager.isAccelerometerAvailable { motionManager.startAccelerometerUpdates(to: .main) { (data, error) in guard let data = data else { return } // Use the accelerometer data } }
Integration with third-party services like social media platforms is a common requirement. If you’re using the Facebook SDK for a web application, initializing and logging in a user could be as simple as:
window.fbAsyncInit = function() { FB.init({ appId : 'your-app-id', cookie : true, xfbml : true, version : 'v8.0' }); FB.login(function(response) { if (response.authResponse) { console.log('Welcome! Fetching your information.... '); FB.api('/me', function(response) { console.log('Good to see you, ' + response.name + '.'); }); } else { console.log('User cancelled login or did not fully authorize.'); } }); };
Here’s an example of integrating with a payment API using Stripe’s SDK to make a charge:
const stripe = require('stripe')('your-secret-key'); stripe.charges.create({ amount: 2000, currency: 'usd', source: 'tok_amex', description: 'My First Test Charge (created for API docs)', }, function(err, charge) { // asynchronously called });
If creating cloud-based applications interests you, you might use Amazon Web Services (AWS) SDK to interact with their plethora of services. For example, creating a new item in DynamoDB would look like:
const AWS = require('aws-sdk'); const docClient = new AWS.DynamoDB.DocumentClient(); var params = { TableName :'yourTable', Item: { 'YourPrimaryKey': 'Key', 'attribute': 'value', // ... more items to add } }; docClient.put(params, function(err, data) { if (err) console.log(err); else console.log(data); });
An application’s performance can be greatly improved by handling tasks in the background. Android SDK allows you to do this with Services. Below is an example of starting a background service:
public class MyService extends Service { @Override public IBinder onBind(Intent intent) { return null; } @Override public int onStartCommand(Intent intent, int flags, int startId) { // Execute your tasks here return START_NOT_STICKY; } }
Lastly, if you are developing games or real-time interactive content, you might find yourself using the Unity SDK. Below is how to move a game object every frame:
using UnityEngine; public class MoveObject : MonoBehaviour { void Update() { transform.Translate(new Vector3(1, 0, 0) * Time.deltaTime); } }
As we continue to explore the diverse landscape of software development, we at Zenva encourage learners to dive deeper into SDKs—their intricacies and nuances—to create applications that are not only code-efficient but embrace the full spectrum of device capabilities and services.
Going further in our exploration of SDKs, let’s delve into more specialized uses. For instance, augmented reality (AR) has been an exciting frontier for developers. Using Apple’s ARKit SDK, you can create immersive AR experiences on iOS devices. Here’s how you could set up an AR session:
import ARKit let arView: ARSCNView = ARSCNView(frame: .zero) arView.session.run(ARWorldTrackingConfiguration())
Moving towards machine learning, TensorFlow’s SDK enables developers to run machine learning models on mobile and edge devices. Here’s how to load and run a model to classify an image:
import tensorflow as tf // Load a pre-trained model let model = tf.keras.models.load_model('path_to_model') // Load an image to classify let img = tf.keras.preprocessing.image.load_img(image_path, target_size=([224, 224])) let img_array = tf.keras.preprocessing.image.img_to_array(img) let img_batch = np.expand_dims(img_array, axis=0) // Predict the class of the image let prediction = model.predict(img_batch) print(prediction)
Within game development, SDKs can help handle multiplayer aspects, like networking. Unity’s networking SDK, UNet, simplifies the process. Below is how you would synchronize a player’s position in a multiplayer game:
using UnityEngine; using UnityEngine.Networking; public class PlayerController : NetworkBehaviour { [SyncVar] private Vector3 syncPos; [SerializeField] Transform myTransform; [SerializeField] float lerpRate = 15; void FixedUpdate() { TransmitPosition(); LerpPosition(); } void LerpPosition() { if (!isLocalPlayer) { myTransform.position = Vector3.Lerp(myTransform.position, syncPos, Time.deltaTime * lerpRate); } } [Command] void CmdProvidePositionToServer(Vector3 pos) { syncPos = pos; } [ClientCallback] void TransmitPosition() { if (isLocalPlayer) { CmdProvidePositionToServer(myTransform.position); } } }
In web development, handling user authentication securely can be streamlined using SDKs like Auth0. With the Auth0 SDK, you can easily authenticate a user and manage their session:
import { Auth0Client } from '@auth0/auth0-spa-js'; const auth0 = new Auth0Client({ domain: 'YOUR_DOMAIN', client_id: 'YOUR_CLIENT_ID' }); await auth0.loginWithRedirect({ redirect_uri: window.location.origin }); auth0.isAuthenticated().then(function (isAuthenticated) { if (isAuthenticated) { auth0.getUser().then(function (user) { console.log('Hello, ', user.name); }); } });
VoIP and messaging platforms like Twilio provide SDKs to enable features such as sending SMS or making calls directly from an app. Here’s an example of sending an SMS with Twilio’s Node.js SDK:
const twilio = require('twilio'); const client = new twilio('ACCOUNT_SID', 'AUTH_TOKEN'); client.messages.create({ body: 'Hello from Twilio!', to: '+12345678901', // Text this number from: '+10987654321' // From a valid Twilio number }) .then((message) => console.log(message.sid));
For data visualization in large web applications, tools like D3.js are essential. D3’s API, considered as an SDK for data-driven documents, enables complex visualizations. Here’s a snippet for creating a basic bar chart:
import * as d3 from 'd3'; const data = [80, 120, 60, 150, 200]; const svg = d3.select('body').append('svg') .attr('width', 400) .attr('height', 300); svg.selectAll('rect') .data(data) .enter().append('rect') .attr('x', (d, i) => i * 80) .attr('y', (d) => 300 - d) .attr('width', 70) .attr('height', (d) => d);
With Zenva, our goal is to provide learners with the knowledge and resources needed to harness the power of various SDKs, allowing you to innovate and create applications or services that significantly contribute to technological advancements. By getting comfortable with SDKs and their various applications, you open up a world of possibilities for your coding projects.
Continuing Your Learning Journey
As you’ve begun to unpick the world of Software Development Kits, remember that this is just the first step in what can become a rich and diverse career in tech. While SDKs offer a tremendous advantage in specialized areas of development, a solid foundation in programming is crucial to making the most of these toolkits.
We at Zenva would love to support you as you build that foundation, and our Python Mini-Degree is an excellent place to continue your journey. With a curriculum that’s designed for both beginners and those looking to expand their skills, these courses will arm you with the knowledge of Python—a versatile language that’s pivotal in areas ranging from web development to artificial intelligence.
If you’re eager to expand your expertise beyond Python, or would like to explore more aspects of programming, be sure to check out our vast collection of Programming courses. These courses align with the latest industry trends and will empower you to become a proficient coder, gear up for the next big tech wave, or simply make your ideas come to life. The path of learning never truly ends, and with Zenva, you can go from beginner to professional at your own pace. Let’s code the future together!
Conclusion
In the digital world, SDKs are the magic boxes that contain the spells you need to conjure applications that enthrall and functions that empower. They are essential in your quest to bring code to life, regardless of the platform or device. With each SDK mastered, you not only broaden your capabilities but also deepen your understanding of the underlying technology that drives innovation.
We invite you to join us at Zenva, where we champion the continuous pursuit of knowledge and skill. Dive into our Python Programming Mini-Degree and open the door to mastering SDKs and beyond. Your adventure into code is only as limitless as your curiosity, and we’re excited to be part of your journey to becoming a coding wizard. The future of technology awaits, and together, we will shape it!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
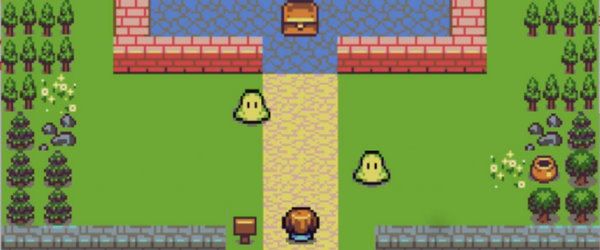
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.