Diving into the world of programming, a code library becomes an essential toolkit much like a Swiss Army knife for developers. It’s your go-to resource for frequently used routines, scripts, and functions that can make coding more efficient and your programs more effective. Aspiring coders and seasoned pros alike find value in a well-crafted library: it saves time, fosters code reuse, and helps in maintaining a consistent coding style across different projects.
Table of contents
What Is a Code Library?
A code library is a collection of pre-written code that can be reused in multiple programming projects. Think of it as a virtual bookshelf filled with resources that you can pull from to solve common problems in programming. Libraries contain sets of functions, methods, and classes that provide a way to harness functionality without the need to write code from scratch.
What Is It For?
Libraries serve as an invaluable resource for developers to:
- Accelerate the development process by using tried and tested code blocks.
- Reduce bugs and errors by relying on well-documented and community-vetted solutions.
- Improve collaboration among team members by sharing a common set of tools and practices.
- Focus on the unique aspects of their projects, rather than reinventing the wheel.
Why Should I Learn It?
By understanding and leveraging code libraries, you can drastically improve your programming prowess. Here’s why learning to use them effectively is so valuable:
- Efficiency: Reusing code saves time, allowing you to deliver projects faster.
- Knowledge Sharing: Libraries often encapsulate complex logic that you can learn from.
- Quality: They help maintain a higher standard of coding by using community-approved methods.
- Versatility: Knowing various libraries can make you more adaptable to different programming tasks.
Whether you’re starting your coding journey or looking to streamline your development process, getting to grips with code libraries is an invaluable step in becoming a proficient programmer.
Getting Started with Code Libraries
To effectively work with code libraries, it’s essential to understand the basics of including them in your projects. Here’s how you can start with some of the most common programming languages:
Python – Importing a Module
In Python, a library is often referred to as a module. You can include it using the `import` statement:
import math print(math.sqrt(16)) # Outputs: 4.0
JavaScript – Using an External Library
JavaScript libraries can be added within the HTML file through a “ tag, or imported in a JavaScript file using `import` statement in modern JS:
<!-- In your HTML file --> <script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.20/lodash.min.js"></script> // In your JavaScript file console.log(_.shuffle([1, 2, 3, 4]));
Basic Library Operations
Once a library is imported, you can start using its functions and objects. Here are some basic operations from popular libraries:
Manipulating Arrays in JavaScript with Lodash
Lodash is a JavaScript utility library that makes it easier to work with arrays, numbers, objects, strings, etc.
console.log(_.compact([0, 1, false, 2, '', 3])); // Outputs: [1, 2, 3] console.log(_.concat([1], 2, [3], [[4]])); // Outputs: [1, 2, 3, [4]]
Working with Dates in Python with datetime
`datetime` is a Python library for dealing with dates and times, allowing you to create, manipulate, and format date and time objects.
from datetime import datetime # Get the current date and time now = datetime.now() print(now) # Formatting a date print(now.strftime("%Y-%m-%d %H:%M:%S"))
Scientific Computing in Python with NumPy
NumPy is a fundamental library for scientific computing in Python, providing support for large multi-dimensional arrays and matrices, along with a collection of high-level mathematical functions.
import numpy as np # Creating a NumPy array arr = np.array([1, 2, 3, 4, 5]) print(arr) # Performing operations on NumPy arrays print(arr * 2)
Integrating Libraries with Functions
Libraries can be nested within functions to create reusable blocks of code that perform a specific task utilizing the library’s capabilities.
Creating a Plot with Matplotlib in Python
Matplotlib is a plotting library for Python which allows you to create a wide variety of static, animated, and interactive plots.
import matplotlib.pyplot as plt def plot_data(x, y): plt.plot(x, y) plt.xlabel('X-axis label') plt.ylabel('Y-axis label') plt.show() plot_data([0, 1, 2, 3], [0, 1, 4, 9])
By leveraging these code examples, you’re now familiar with the basics of including and using functionalities from a variety of code libraries. Stay tuned for the next part of our tutorial where we’ll delve into more advanced use cases and reveal powerful tips to get the most out of code libraries.
Advanced Use Cases of Code Libraries
Automating Web Browsing with Selenium
Selenium is a powerful tool for controlling web browsers through programs and performing browser automation. It is especially useful for testing web applications and scraping web data.
from selenium import webdriver driver = webdriver.Firefox() driver.get("http://www.example.com") # Find an element by its name attribute and type something into it search_box = driver.find_element_by_name('q') search_box.send_keys('Zenva') # Submit the form search_box.submit() driver.quit()
Creating RESTful API Requests with Axios in JavaScript
Axios is a promise-based HTTP client for the browser and Node.js, perfect for making HTTP requests to fetch or save data.
const axios = require('axios'); // Fetch data from a URL axios.get('https://api.example.com/data') .then(response => { console.log(response.data); }) .catch(error => { console.error('An error occurred:', error); }); // POST request to send data axios.post('https://api.example.com/data', { key: 'value' }) .then(response => { console.log('Data saved:', response); }) .catch(error => { console.error('Cannot save data:', error); });
Data Visualization with D3.js
D3.js is a JavaScript library for manipulating documents based on data, enabling complex data visualization capabilities.
// Assume we have an SVG element with width and height defined const svg = d3.select('svg'); const data = [4, 8, 15, 16, 23, 42]; // Create bars for a simple bar chart svg.selectAll("rect") .data(data) .enter().append("rect") .attr("width", 20) .attr("height", d => d * 10) .attr("x", (d, i) => i * 25) .attr("y", d => 100 - d * 10);
Password Hashing in Python with bcrypt
Security is paramount when storing user information. bcrypt is a Python library for hashing passwords in a secure manner.
import bcrypt # Generate a salt salt = bcrypt.gensalt() # Hash a password with the salt hashed = bcrypt.hashpw('my_secret_password'.encode('utf-8'), salt) print(hashed) # Check that an unhashed password matches one that has previously been hashed if bcrypt.checkpw('my_secret_password'.encode('utf-8'), hashed): print("It's a match") else: print("It's not a match")
Creating Command-Line Interfaces (CLI) with Click in Python
Click is a Python package for creating beautiful command line interfaces in a composable way. It allows for easy building of command line tools.
import click @click.command() @click.option('--count', default=1, help='Number of greetings.') @click.option('--name', prompt='Your name', help='The person to greet.') def hello(count, name): """Simple program that greets NAME for a total of COUNT times.""" for _ in range(count): click.echo(f"Hello, {name}!") if __name__ == '__main__': hello()
By integrating these advanced code examples into your projects, you can see how libraries add extensive functionality and help solve complex problems with less effort. Remember, the real skill in using libraries lies in knowing which one fits your needs and how to harness its potent features effectively.Incorporating functionality from various code libraries not only streamlines your workflow but also opens up a world of possibilities for what you can achieve with your code. Below are some more sophisticated examples highlighting how to integrate different libraries into your projects.
Data Analysis with Pandas
Pandas is a game-changer for data manipulation and analysis in Python. It provides structured data operations for DataFrames, which are similar to spreadsheets or SQL tables.
import pandas as pd # Create a DataFrame from a CSV file df = pd.read_csv('data.csv') # Perform data summarization using groupby grouped_data = df.groupby('column_name').sum() # Filtering data filtered_data = df[df['column_name'] > 100]
Network Requests with Python’s Requests Library
The Requests library in Python is the de facto standard for making HTTP requests, famous for its simplicity and elegance.
import requests response = requests.get('https://api.example.com/data') if response.status_code == 200: data = response.json() print(data) else: print('Failed to retrieve data')
Real-time Applications with Socket.IO
Socket.IO enables real-time, bidirectional, and event-based communication between web clients and servers.
const io = require('socket.io')(server); io.on('connection', (socket) => { console.log('A user connected'); socket.on('chat message', (msg) => { io.emit('chat message', msg); }); socket.on('disconnect', () => { console.log('User disconnected'); }); });
Building Interactive User Interfaces with React
React is a declarative, efficient, and flexible JavaScript library for building user interfaces. It lets you compose complex UIs from small and isolated pieces of code called components.
import React, { useState } from 'react'; function ExampleComponent() { // Declare a new state variable, which we'll call "count" const [count, setCount] = useState(0); return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}> Click me </button> </div> ); }
Creating Command-Line Tools with argparse
Python’s argparse module makes it easy to write user-friendly command-line interfaces. Your programs can define what arguments they require, and argparse figures out how to parse those out of `sys.argv`.
import argparse parser = argparse.ArgumentParser(description="Process some integers.") parser.add_argument('integers', metavar='N', type=int, nargs='+', help='an integer for the accumulator') parser.add_argument('--sum', dest='accumulate', action='store_const', const=sum, default=max, help='sum the integers (default: find the max)') args = parser.parse_args() print(args.accumulate(args.integers))
Advanced Image Processing with PIL/Pillow
Pillow is the friendly PIL fork by Alex Clark and Contributors. PIL (Python Imaging Library) adds image processing capabilities to your Python interpreter.
from PIL import Image, ImageFilter # Open an image file with Image.open('image.jpg') as img: # Apply a filter to the image blurred_image = img.filter(ImageFilter.BLUR) # Save the modified image blurred_image.save('blurred_image.jpg')
Each of these libraries brings a unique set of capabilities that can be combined and leveraged to produce powerful applications. In crafting code and developing projects, the judicious use of such libraries not only exemplifies smart programming but also can give rise to innovative and efficient solutions. Our commitment to quality education at Zenva ensures that when you learn these skills with us, you are armed with the knowledge to utilize these tools to their fullest potential.
Continue Your Learning Journey
Exploring the world of code libraries is just the beginning of your programming odyssey. To further hone your skills and become a proficient Python developer, we encourage you to take the next step with our Python Mini-Degree. This comprehensive program is designed to guide you through Python basics, advance your knowledge on algorithms and object-oriented programming, as well as dive into creating your own games and applications.
Whether you’re just starting out or looking to deepen your programming knowledge, our Python Mini-Degree is structured to provide a balanced learning experience that accommodates your pace. You’ll get hands-on with projects that cement your understanding and contribute to a portfolio that showcases your abilities. If you’re eager to expand your expertise even further, explore our broader range of Programming courses covering various languages and modern development practices.
At Zenva, we believe in empowering you with the skills needed to turn your passion for coding into a career. Join our community of learners and take the next step in your journey towards becoming a versatile and sought-after programmer.
Conclusion
In the ever-evolving landscape of technology, the proficiency to utilize code libraries effectively is undeniably an asset. As you’ve seen, libraries can catapult your coding capabilities, allowing you to create robust and efficient applications with ease. Diving into libraries not only sharpens your technical acumen but also unlocks a treasure trove of possibilities for innovation and creativity in your projects.
Don’t stop here; transform your curiosity into expertise with our Python Mini-Degree and join a community where learning and real-world practice go hand in hand. At Zenva, we’re thrilled to be a part of your journey and can’t wait to see the amazing things you’ll build. Remember, every expert was once a beginner, and with each line of code, you’re shaping your future in the tech world.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
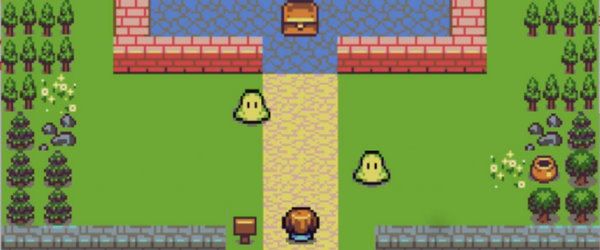
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.