Welcome to this comprehensive tutorial on how to skillfully and effectively utilize the “if” statement in C#. As we embark on this exciting journey, we will shed light on this vital concept, simplifying it with engaging examples and analogies. Whether you are taking your initial steps in the coding journey or are already an experienced programmer, this guide offers valuable insights that you can apply in a wide range of scenarios, primarily in game development!
Table of contents
Understanding the ‘if’ Statement in C#
The ‘if’ statement plays a crucial role in C# programming as it forms the fundamental building block of decision-making structures. Simply put, it translates our everyday ‘if-this-then-that’ logic into the code, enabling your application to make basic decisions based on specific conditions.
Why Is ‘If’ Statement Important?
Mastering the ‘if’ statement in C# allows your code to be smart and dynamic. Without it, our code would be rigid and linear, incapable of adjusting to varying inputs or situations. In the realm of game development, for instance, ‘if’ statements can determine winners or losers based on scores, or control interactive elements like unlocking achievements when certain conditions are met.
Now, let’s delve into the coding part of the tutorial.
Note-Worthy Basics of ‘If’ Statement
Before you dive deep, let’s grasp how an ‘if’ statement takes shape in a C# code. The basic syntax is as follows:
if (condition) { // code to be executed if the condition is true }
The ‘condition’ is put to test. If it evaluates as true, the code within the brackets executes. If not, the program simply skips it.
Let’s look at a simple game scenario:
int playerScore = 100; if (playerScore >= 100) { // Player wins the game }
In this example, if the player’s score is 100 or more, they win the game.
Diversifying Decisions with ‘Else’ and ‘Else If’
For decision making, it’s often required to have more than just one condition. Companions of ‘if’, the ‘else’ and ‘else if’ statements, come in handy then. They help us handle multiple conditions and paths.
Consider a more comprehensive game scenario:
int playerHealth = 50; if (playerHealth == 100) { // Player is at full strength } else if (playerHealth >= 70) { // Player is in good health } else { // Player needs health recovery }
In this gaming case, the program checks the player’s health level and decides the game status accordingly.
Combining Conditions: ‘AND’ and ‘OR’
What if we need to test more than one condition at once? That’s when logical operators come into play. ‘AND’ (&&) and ‘OR’ (||) allow us to evaluate multiple conditions together, adding sophistication to our decision-making structure.
int playerHealth = 50; int gameLevel = 10; if (playerHealth >=70 && gameLevel > 5) { // Player is strong and has crossed initial levels - reward them! }
This ‘if’ statement checks if the player is in good health and has crossed the 5th level. If both conditions are true, the player is rewarded.
Unleash Your Potential with Unity Mini-Degree
Prompted and excited to plunge into the adventure-filled realm of game development? We, at Zenva, stand by you in this endeavour. Our Unity Mini-Degree is the perfect platform that equips you with comprehensive knowledge, spanning from basics to advanced coding, game creation, and even AI.
Conclusion
In this tutorial, we’ve provided a deep dive into the ‘if’ statement in C#, highlighting its importance and use-cases in game mechanics. We took real-life analogies and crafted beginner-friendly examples, ensuring the tutorial meets the needs of both novice and seasoned programmers. The tutorial sincerely aimed at presenting this fundamental concept as engaging, valuable, and supremely useful.
While we’ve covered a considerable ground, the key to mastery is continuous practice and learning. To further sharpen your skills and delve deeper into the fascinating world of game development, feel free to join us in our Unity Mini-Degree. Let’s keep growing and learning together!
Performing Actions Based on Boolean Values
In C#, the ‘if’ statement often uses Boolean expressions – i.e., conditions that are either true or false. Let’s observe some coding examples where the actions are based on Boolean values.
bool isRaining = true; if (isRaining) { // Execute rain effect in the game }
In the above instance, if it is raining (isRaining is true), the game will execute a rain effect.
bool keyFound = false; if (!keyFound) { // Pop a prompt for the player to find the key }
In contrast, here’s an example of where ‘if’ is used with a negative condition. If the key is not found (keyFound is false), the game will prompt the player to find the key.
Using ‘if’ Statements with Strings
While numbers and Booleans are common, ‘if’ statements can also work with strings. It’s essential, especially when we want different outcomes based on text inputs or comparison.
string playerName = "Zenva"; if (playerName == "Zenva") { // Welcome message for Zenva }
In this scenario, if the player’s name is Zenva, a customized welcome message will be displayed.
string gameStatus = "Paused"; if (gameStatus.Equals("Paused", StringComparison.OrdinalIgnoreCase)) { // Code to pause the game }
In the above example, the game is paused if the gameStatus string equals “Paused”, regardless of the case.
Creating Complex Conditions with Nested ‘if’
For decision-making scenarios that require evaluating more than one sequence of conditions, we use nested ‘if’ statements. As the name suggests, nested ‘if’ involves an ‘if’ statement inside another ‘if’ statement.
int playerHealth = 50; bool shieldActive = true; if (playerHealth < 70) { if (shieldActive) { // Activate shield to protect player } else { // Urgent health recovery needed } }
Here, we first check if the player’s health is less than 70. If so, a nested ‘if’ statement further checks if a protective shield is active. If true, the shield is activated. If not, an alert pops for health recovery.
Combining ‘if’ with Switch-case
if’ statements can also be used before a switch-case when you need to check a particular condition for a variable before moving into multiple choice paths.
string playerClass = "Warrior"; int playerScore = 200; if (playerScore >= 100) { switch (playerClass) { case "Warrior": // Player is a strong Warrior break; case "Mage": // Player is a powerful Mage break; default: // Player class is none of the above break; } }
In this gaming case, if the player’s score is 100 or more, the switch-case helps in further classification based on their character class – Warrior or Mage.
Remember, the real magic unfolds when these concepts are actually put in practice. So, don’t haste; take your own pace and keep practicing as you understand!
Validating Multiple Conditions Using ‘if’ Statement
Your game logic might require checking multiple conditions and performing actions based on their collective output. For such cases, ‘if’ statements can be paired with logical operators like ‘AND'(&&) and ‘OR'(||).
string playerClass = "Mage"; int playerHealth = 75; if (playerClass == "Mage" && playerHealth > 50) { // Activate magic shield }
This piece of code activates a magic shield for the player who is a Mage and his health is above 50.
int gameScore = 500; string bonusItem = "MysteryBox"; if (gameScore > 300 || bonusItem == "MysteryBox") { // Award bonus points }
In the second example, the player is awarded bonus points if either their game score exceeds 300, or they have a MysteryBox.
Understanding Short Circuit Evaluation
The ‘AND'(&&) and ‘OR'(||) operators in C# follow short-circuit evaluation. That means, if the result of an expression can be determined by evaluating the first condition, the subsequent ones aren’t tested.
int playerSpeed = 0; if (playerSpeed != 0 && 100 / playerSpeed > 50) { // Reduce speed }
In this code snippet, the second condition (100 / playerSpeed > 50) will only be evaluated if the first condition (playerSpeed != 0) is true. This prevents a divide-by-zero error.
The Ternary (‘? :’) Operator
The ternary operator is a shorthand for an ‘if-else’ statement and is particularly useful for simple conditional assignments. The syntax is as follows:
condition ? ifTrue : ifFalse
int healthPotion = 6; int healthBoost = (healthPotion > 5) ? 50 : 25;
In this example, if the number of healthPotion is more than 5, player’s healthBoost is set to 50. If not, it’s set to 25.
Limitations of ‘if’ Statements and Alternatives
‘if’ statements can become complex and hard to read when dealing with many conditions. This is where other C# structures like ‘switch’ statements or lookup tables become useful for improving readability and maintainability.
Consider this example:
string characterClass = "Elf"; if (characterClass == "Elf") { /* Code for Elf */ } else if (characterClass == "Orc") { /* Code for Orc */ } else if (characterClass == "Human") { /* Code for Human */ } // And so on for multiple other classes
That can be made more efficient with a ‘switch’ statement as follows:
string characterClass = "Elf"; switch (characterClass) { case "Elf": // Code for Elf break; case "Orc": // Code for Orc break; case "Human": // Code for Human break; // And so on for multiple other classes default: // Code for an unknown class break; }
‘switch’ can make the code much cleaner and easier to read when there are many different conditions to consider.
Remember, programming and game development is at its core about problem solving. It’s essential to understand various tools and concepts, such as ‘if’ statements, so you can select the most effective and efficient solution for any problem your game faces.
Enhancing Choices with ‘Else-If’ Chain
For more complex decisions where there are several conditions to check, we can create an ‘else-if’ chain. It considers the conditions from top to bottom, and once a true condition is found, its corresponding block of code is executed, and the rest of the chain is ignored.
int playerScore = 85; if (playerScore >= 90) { // Grade A } else if (playerScore >= 80) { // Grade B } else if (playerScore >= 70) { // Grade C } else { // Grade D }
This piece of code is using an ‘else-if’ chain to implement a simple grading logic based on the player’s score. Depending on the score, it will assign a grade to the player, and the rest of the chain will be bypassed.
Understanding ‘If-Else-If’ Ladder
The ‘if-else-if’ ladder is useful for situations where you need to check multiple conditions and execute different code blocks. The keyword ‘else’ attached with the subsequent ‘if’ checks the condition only when the previous conditions fail.
string playerClass = "Wizard"; int playerScore = 90; if (playerClass == "Warrior" && playerScore >= 85) { // Code for a strong Warrior } else if (playerClass == "Wizard" && playerScore >= 80) { // Code for a powerful Wizard } else { // Code for other classes or lower scores }
This ‘if-else-if’ ladder first checks if the player is a strong Warrior (score 85+). If this fails, it checks if the player is a powerful Wizard (score 80+). If both conditions fail, it executes a separate block of code.
Condition Evaluation in an ‘If’ Statement
Remember that in C#, all non-zero and non-null values are considered true, and zero and null are considered false. This can be used to perform actions based on the existence or truthiness of data.
List<string> inventoryItems = new List<string>(); if (inventoryItems.Count) { // Show inventory } else { // Show empty inventory message }
The above block checks if the inventory has items based on the count property. If there are items (Count is non-zero), the inventory will be shown to the player; if not, a message about an empty inventory will be displayed.
‘If’ Statement with Functions
‘if’ statements can also be used with functions. Functions can return values used in conditions, can be called in ‘if’ statement blocks, or can be used for both.
int playerScore = CheckPlayerScore(); if (IsPlayerWinning(playerScore)) { CelebrateVictory(); }
This example first retrieves the player’s score with the CheckPlayerScore() function. This score is then evaluated by the function IsPlayerWinning(), inside the ‘if’ statement’s condition. If the player is winning, the function CelebrateVictory() is called.
Conclusion
If’ statements are the cornerstone of all programming languages, and they play a significant role in C#. It provides the basic decision-making capabilities to our games, making the game logic dynamic. Whether it is to check if a player has scored enough to level up, or see if all the enemies are defeated, ‘if’ statements make it possible.
The crucial part is understanding when and how to use ‘if’ statements. Remember, the power of ‘if’ statements is unlimited when you understand how to use them effectively.
Where to Go Next?
Mastering the ‘if’ statement is a significant step forward in your coding journey, particularly towards game development. However, the world of programming is vast, full of exciting concepts and elements awaiting your exploration. And that’s exactly where our Unity Mini-Degree comes in!
The Unity Game Development Mini-Degree we offer is a comprehensive collection of courses, covering a wide range of topics – from game mechanics and audio effects to cinematic cutscenes. What’s more exciting is that Unity is not only prevalent in the gaming industry but also finds applications in various fields like architecture, healthcare, education, and engineering.
The benefit of learning with us at Zenva extends beyond gaining knowledge. On completion of the courses, you earn certificates, paving your way towards multiple career opportunities in the thriving game industry. So, why wait? Keep learning, and bolster your path to becoming a professional! Besides the mini-degree, you can also explore a broader collection in our Unity content.
Conclusion
Whether you’re building immersive games, design-rich applications or tech-forward innovations, the ‘if’ statement in C# is a basic yet immensely powerful tool in your arsenal. The understanding and skillful application of this concept not only lays a solid foundation of your programming journey, but it also rolls the red carpet to a multitude of opportunities that thrive at the intersection of creativity and technology.
At Zenva, our commitment is to stand by you at every step of this journey and provide you with the necessary training, tools, and guidance. So, waste no time! Leap into the journey by joining our Unity Mini-Degree and unlock your full potential to create, innovate, and inspire!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
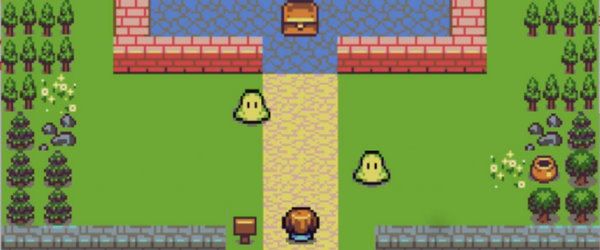
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.