Welcome to our comprehensive tutorial on Roblox obby scripting. This engaging guide aims to provide both novices and seasoned coders with a valuable stepping stone to building their own obstacle course — commonly known as “obby” — games within the immersive world of Roblox.
Table of contents
Demystifying Roblox Obby Scripting
Before we launch into the formative sections of our coding tutorial, let’s take a moment to understand what Roblox obby scripting entails. Obby, short for obstacle, in Roblox refers to a popular game where players have to navigate through various obstacles to reach a goal. The excitement lies not just in playing the game, but also in bringing one to life using Roblox Lua scripting, a powerful tool that allows you to control the behavior of your obby.
The Worth of Learning Roblox Obby Scripting
Learning to script obbies in Roblox is not only fun, but it’s a valuable skill that can open doors to an exciting future in game development. As the digital world increasingly infuses with our reality, gaining an understanding of this interactive language can enhance both your problem-solving skills and creative thought process. Whether you’re aiming for a career in game development or simply finding a rewarding hobby, enriching your coding arsenal with Roblox obby scripting is a definite front runner.
Getting Started With Roblox Obby Scripting
To get right into Roblox obby scripting, it’s imperative we first set up our development environment. Once you are successfully logged into Roblox Studio, create a new baseplate. This serves as the main ground that will host your obby.
--Create new part (platform in the game) local part = Instance.new("Part", workspace) --Add the position and size of the part part.Size = Vector3.new(10,1,10) part.Position = Vector3.new(0,0.5,0) part.Anchored = true part.BrickColor = BrickColor.new("Bright red")
Laying Out the Obstacles
Once your baseplate is ready, let’s create some obstacles. To do this, we will design a function that generates platforms within specified parameters. The script below creates a platform at a given point.
--Function to create a platform function createPlatform(position, size, color) local part = Instance.new("Part", workspace) part.Size = size part.Position = position part.Anchored = true part.BrickColor = BrickColor.new(color) end
Using the function above, we can create platforms in different locations with various sizes and colors. Use the code below as an example of creating various platforms:
--Create platforms using the method stated above createPlatform(Vector3.new(10, 0.5, 10), Vector3.new(10, 1, 10), "Bright red") createPlatform(Vector3.new(20, 0.5, 20), Vector3.new(10, 1, 10), "Bright blue") createPlatform(Vector3.new(30, 0.5, 30), Vector3.new(10, 1, 10), "Bright yellow")
Adding Player Interaction
The next step is scripting player interaction to our obby game. We start by adding a script that detects when a player touches a platform:
--Add a script that activates when a Part is touched part.Touched:Connect(function(hit) --We want to check if it's a human who touched the Part. local humanoid = hit.Parent:FindFirstChild("Humanoid") --If humanoid isn't nil, that means a human touched the Part. if humanoid then print("Platform has been touched by a player!") end end)
From here, let’s introduce a function that tracks when a player fails an obstacle and responds by respawning the player at the beginning.
function playerDies(player) --Grab the player's character local character = player.Character --If character isn't nil, that means the player has a character in the workspace if character then --Grab humanoid from character local humanoid = character:FindFirstChild("Humanoid") --If humanoid isn't nil, that means the player's character is a humanoid if humanoid then --Kills the humanoid (makes the humanoid's Health reach 0) humanoid.Health = 0 end end end
Each concept we’ve discussed here sets you on the path to scripting exciting and dynamic obby games on Roblox. Remember, practice is key to becoming proficient, so keep experimenting and enhancing your obbies with every lines of code!
Enhancing Player Interactions
Now that we can detect player interaction and respawn a player, let’s script additional interactions. Below is an example of a script that makes a player jump when they touch a platform:
part.Touched:Connect(function(hit) local humanoid = hit.Parent:FindFirstChild("Humanoid") if humanoid then --Make the player jump humanoid.Jump = true end end)
Let’s take a step further by adding scripts that increase the player’s running speed when they touch a particular platform:
part.Touched:Connect(function(hit) local humanoid = hit.Parent:FindFirstChild("Humanoid") if humanoid then --Increase the player's running speed humanoid.WalkSpeed = 50 end end)
Fine-Tuning Our Obby Game
As you become more comfortable with scripting player interactions, you can begin fine-tuning your obby game. One way to achieve this is by scripting the game to produce sounds or visual effects, adding more life into your game.
The following example shows how to incorporate sound into your game:
--Add the sound to the workspace local sound = Instance.new("Sound", workspace) sound.Volume = 0.5 sound.SoundId = "rbxassetid://145204128" --Play the sound when a player touches a platform part.Touched:Connect(function(hit) local humanoid = hit.Parent:FindFirstChild("Humanoid") if humanoid then sound:Play() end end)
Another fun modification involves manipulating the original size of platforms upon interaction:
part.Touched:Connect(function(hit) local humanoid = hit.Parent:FindFirstChild("Humanoid") if humanoid then part.Size = part.Size * 2 end end)
Furthermore, you can add a scripting function that will create parts periodically for players to climb on:
-- this part needs to be put in a while loop while wait(3) do createPlatform(Vector3.new(math.random(10, 300), 0.5, math.random(10, 300)), Vector3.new(10, 1, 10), "Bright red") end
The script above ensures parts spawn every 3 seconds in random positions, adding dynamic and unpredictable elements to your obby game.
Roblox obby scripting is exciting and expansive. With patience and creativity, you can transform a few lines of code into a dynamic, enjoyable, and engaging game. Practice makes progress, so keep experimenting and pushing the boundaries of what’s possible!
Introducing Physics and Risks to your Obby
A key element of any obby game is the layer of difficulty added by physics and risks involved. This could involve anything from making platforms disappear after a certain time, implementing traps within your game or creating moving platforms. Let’s delve into these topics:
Let’s start by making a platform disappear after a player lands on it, adding a layer of difficulty:
part.Touched:Connect(function(hit) local humanoid = hit.Parent:FindFirstChild("Humanoid") if humanoid then wait(2) part:Destroy() end end)
Next, how about creating traps in your obby? This could be achieved by simply creating a red part in workspace, which when touched by the player, leads to instant death:
--Create the trap local part = Instance.new("Part", workspace) part.Size = Vector3.new(10, 1, 10) part.Position = Vector3.new(10, 0.5, 10) part.Anchored = true part.BrickColor = BrickColor.new("Bright red") --Define what happens when the trap is touched part.Touched:Connect(function(hit) local humanoid = hit.Parent:FindFirstChild("Humanoid") if humanoid then humanoid.Health = 0 end end)
Adding Movement to the Platforms
Adding moving platforms is another great way to write awesome obby games in Roblox. Let’s see how to script this:
--Create a platform to be modified to move local movingPart = Instance.new("Part", workspace) movingPart.Size = Vector3.new(10, 1, 10) movingPart.Position = Vector3.new(10, 0.5, 10) movingPart.Anchored = true --While loop to modify its position constantly while true do movingPart.Position = movingPart.Position + Vector3.new(0, 0, 10) wait(1) movingPart.Position = movingPart.Position - Vector3.new(0, 0, 10) wait(1) end
The script above will create a platform that consistently moves up and down, creating a challenging moving obstacle for players.
Adding Extra Lives to Players
Lastly, when building obby games, you might want to give players the opportunity to earn extra lives. This can be achieved by scripting a special platform that, when touched, grants the player an additional life:
--Create the life-giving platform local part = Instance.new("Part", workspace) part.Size = Vector3.new(10, 1, 10) part.Position = Vector3.new(10, 0.5, 10) part.Anchored = true part.BrickColor = BrickColor.new("Bright green") --Define what happens when the platform is touched part.Touched:Connect(function(hit) local humanoid = hit.Parent:FindFirstChild("Humanoid") if humanoid then humanoid.MaxHealth = humanoid.MaxHealth + 100 humanoid.Health = humanoid.MaxHealth end end)
This script creates a platform that when touched, not only expands players’ maximum health pool by 100 points, it also instantly restores their current health to its maximum. By throwing these benefits to certain strategically placed bases, you can introduce a new layer of strategies to your obby game.
Roblox obby scripting paves the way for an enriching and educationally thrilling journey for prospective game developers of all ages. With some creativity, and a healthy dose of regular practice, you can build captivating obby games that fellow players will find both challenging and delightful. Always remember, the only limit is your imagination!
How to Continue Your Roblox Journey
The wonderful world of Roblox game development is vast and full of exciting opportunities for both upcoming and experienced developers. If you’ve enjoyed diving into the process of scripting obbies and are determined to ramp up your skills further, Zenva has an abundance of resources to assist you on your journey.
We invite you to explore our Roblox Game Development Mini-Degree, a complete collection of courses that delve into various genres of game creation with Roblox Studio and Lua. With this Mini-Degree, you will learn to create obstacle courses, melee combat games, FPS games, and more, while getting adept at implementing multiplayer features and leaderboards. Furthermore, this Mini-Degree is project-oriented, ensuring you can build a robust portfolio while learning – an invaluable asset in the burgeoning field of game development.
In addition to this, you may want to check out our broader collection of Roblox courses, designed to provide flexible learning opportunities for all. At Zenva, we are committed to helping you evolve from a beginner to a professional game developer with our extensive, up-to-date course materials. Step into the world of game creation with us and hone your skills in Roblox scripting!
Conclusion
Embarking on the adventure of creating your own Roblox obby games is a rewarding journey, not to mention an excellent way to learn, innovate, and experiment with the dynamics of game development. As you continue to discover new methods of scripting in Lua and creating immersive games in Roblox, you simultaneously unlock valuable lessons in problem-solving, creativity, and technical proficiency.
Whether you’re just beginning your development quest or seeking deeper understanding of Roblox scripting, Zenva’s Roblox Game Development Mini-Degree offers a comprehensive pathway towards mastery. With our step-by-step instruction, professional teachings, and hands-on projects, we’re confident you’ll emerge with superior knowledge, a solid portfolio, and a budding passion in the realm of game development. Onward into the world of coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
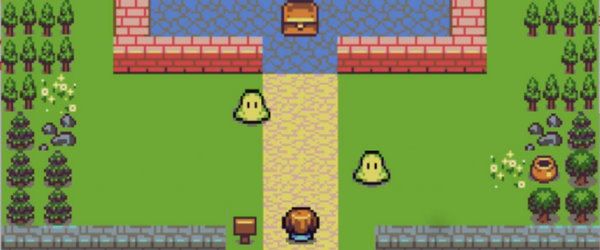
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.