Welcome to this exciting journey into the world of web crawling! In this comprehensive tutorial, we’ll unveil Python’s incredible capabilities for web crawling and showcase how it can empower you to access and process vast quantities of data from the web.
Table of contents
What is Python Web Crawling?
In simple terms, web crawling refers to the automated browsing of web content – like a virtual spider – following links, and gathering data along the way. Python’s rich libraries and powerful functionalities make it an ideal language for carrying out web crawling tasks with efficiency and ease.
Web crawling is arguably one of the most sought-after skills in today’s data-driven world. As an aspiring coder, should you harness the power of Python for web crawling, you’d be able to not only retrieve and analyze massive datasets but also develop insightful applications, tools, and games!
Why Learn Python Web Crawling?
Here’s why Python web crawling should be a key part of your coding repertoire:
- Data Access: Learning Python web crawling enables you to access and analyze vast amounts of data available on the web.
- Job Prospects: Companies across industries are passionate about leveraging the great power of data, escalating the demand for professionals with skills in Python web crawling.
- Diverse Applications: From developing search engines to content aggregation tools and insightful game mechanics, the possibilities are endless!
But that’s not all! Learning Python web crawling is also fun and engaging, as we’ll illustrate in the following sections with game-themed examples.
Getting Started with Python Web Crawling
Before we dive into the examples, ensure that you have Python installed. Additionally, install the ‘requests’, and ‘BeautifulSoup’ libraries, which we’ll be using. You can install them using the following commands:
pip install requests pip install beautifulsoup4
Part 1: Making HTTP Requests in Python
The first step in Python web crawling is to send an HTTP request to the URL of the web page you want to access. Python’s ‘requests’ module provides in-built method called get() for this purpose. Here’s an illustrative example:
import requests response = requests.get('https://www.zenva.com/') print(response.status_code)
Here, ‘https://www.zenva.com/’ is the website URL you want to access, and ‘.status_code’ returns the HTTP response status. ‘200’ signifies a successful HTTP request.
Part 2: Web Scraping with BeautifulSoup
‘BeautifulSoup’ is a Python library for parsing HTML and XML documents. We create a BeautifulSoup object and specify a parser to parse the webpage content.
from bs4 import BeautifulSoup import requests response = requests.get('https://www.zenva.com/') soup = BeautifulSoup(response.text, 'html.parser') print(soup.prettify())
‘prettify()’ method makes the HTML content more readable. Through BeautifulSoup, you can navigate and search the parse tree.
Part 3: Extracting Links
We can use ‘soup’ to extract all the links within <a> tags:
for link in soup.find_all('a'): print(link.get('href'))
This will display every URL found within an <a> element on the page. If the href doesn’t exist, ‘None’ is printed.
Part 4: Scraping Text Data
You can extract all the text that is inside a paragraph <p> tag:
for data in soup.find_all('p'): print(data.get_text())
Here, ‘get_text()’ extracts all the text that is surrounded by HTML tags.
That’s it! You’re now set to begin your own web crawling adventures! Python web crawling provides a wonderful doorway into the world of Big Data, and we at Zenva can’t wait to see what you’ll create.
Part 5: Navigating the Parse Tree
One of BeautifulSoup’s strengths is its ability to navigate a parsed document’s parse tree. We can navigate the HTML tree structure using BeautifulSoup’s tag name. Here’s an example:
soup.title
This prints the title tag and its content. Similarly, if you want the string inside title tag, use:
soup.title.string
Part 6: Finding HTML Elements
Let’s suppose we want to find all instances of ‘p’ tags in the HTML document. We can do this using BeautifulSoup’s ‘.find_all()’ method.
soup.find_all('p')
This will return a list containing each ‘p’ tag and their text content, if any. Equally, you can search for other HTML tags by replacing ‘p’ with your desired tag.
Part 7: Finding HTML Elements with Specific Attributes
You can find HTML tags with specific attributes using BeautifulSoup. Suppose you want to find all tags which contain a specific class name:
soup.find_all('div', class_='className')
This snippet will return all ‘div’ tags that contain the class ‘className’.
Part 8: Extracting All URLs
You can use BeautifulSoup to search for ‘a’ tags and then extract their ‘href’ attribute:
for link in soup.find_all('a'): print(link.get('href'))
This code snippet prints the ‘href’ attribute (or the URL of the link) of each ‘a’ tag in the HTML document.
Part 9: Finding the Parent of an HTML Element
Another great feature of BeautifulSoup is the ability to find the parent of an HTML element:
soup.title.parent.name
This will return the name of the tag that encloses the ‘title’ tag. With these powerful tools at your disposal, the world of Python web crawling and web scraping is your oyster!
Where to go next?
Having taken your first steps into Python web crawling, you might wonder, “What’s next?”
The beauty of the Python language is its expansive nature and multiple applications. Beyond web crawling, it plays a crucial role in areas like data science, machine learning, game development, and app development. And that’s exactly what we offer in our Python Mini-Degree program.
The Python Mini-Degree offered by Zenva Academy is a comprehensive compilation of courses covering Python programming in great depths. It delves into various relevant topics right from coding basics and algorithms to object-oriented programming, game development, and app development.
Our practical learning approach helps students to create their own games, real-world apps, and algorithms. This hands-on experience not only boosts understanding but also helps students build a strong Python portfolio. These skills are especially in high demand in today’s job market, especially in sectors involving data science. Our offering is flexible and accessible at any time, with interactive quizzes and in-browser coding practice to reinforce your learning.
The training concludes with a certificate of completion – a testimony of your new skills, often used by our learners to secure jobs and even initiate their own entrepreneurial ventures.
So, don’t wait any longer. Dive into our Python courses and carry forward your journey to become a Python professional!
Conclusion
To sum up, Python web crawling is your key to unlocking countless doors in the vast digital universe and extracting the infinite data treasures they hold. There’s never been a better time to dive headfirst into Python web crawling, to bolster your skill-set, and step up your coding prowess. We at Zenva are always here to guide and support you on this journey.
Get started with Zenva’s Python Mini-Degree program now and pave the path for your programming triumphs. We can’t wait to see where your Python adventures take you!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
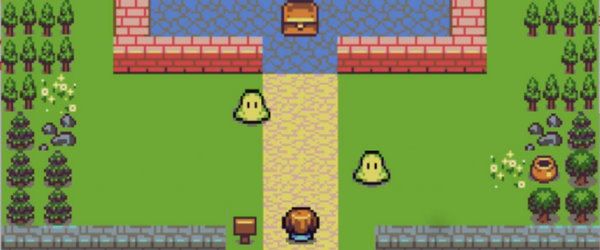
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.