Welcome to this compelling guide to the Python Switch Function! Today, we are venturing into the world of Python, a language acclaimed for its versatility and user-friendliness, but curiously lacking in a native switch function.
Table of contents
What is a Switch Function?
A switch function is a flow-control structure available in several high-level programming languages. It allows for cleaner, more readable code when dealing with multiple conditional statements.
Python, notably, does not come with a built-in switch function. But don’t fret – it turns out that Python’s impressive flexibility offers clever workarounds to implement a similar functionality.
Knowing how to create and use a Python version of a switch function is a critical skill, especially if you’re switching from a language that included this function natively. It streamlines your conditional statements and makes your code cleaner and more efficient. If you like seeing your code run smoothly and efficiently, then this tutorial is an exciting journey you definitely want to embark on.
Creating your own Python-style switch cases can be massively helpful in game development and other coding projects. It’s useful for managing different states or modes within your game or app, from character actions to menu selections, giving you more control and simplifying your code.
Creating a Python Switch Using Dictionaries
Let’s dive right into the heart of it: how to implement a Switch-style function in Python using dictionaries.
def switch_case(value): return { 'case1': 'This is the first case', 'case2': 'This is the second case', 'case3': 'This is the third case', }.get(value, 'Invalid case')
In this example, our function switch_case takes a value as input and uses it to return a corresponding case statement from the dictionary. If the value doesn’t match any of the cases, it returns ‘Invalid case’.
Python Switch with Functions
For more complex scenarios where each case performs a different function, we can use Python’s first-class function objects. Instead of strings, we’ll map our cases to individual functions.
def case1(): return 'This is the first case' def case2(): return 'This is the second case' def default(): return 'Invalid case' def switch_case(value): return { 'case1': case1, 'case2': case2, }.get(value, default)()
Notice how we’ve assigned the functions themselves (not their return values) to the cases in our dictionary. If none of the cases match, our dictionary will return the default function.
Python Switch Function With Parameters
Sometimes, our switch cases will need to accept parameters. How do we handle that?
def case1(name): return f'This is the first case, {name}' def case2(name): return f'This is the second case, {name}' def default(name): return f'Invalid case, {name}' def switch_case(value, name): return { 'case1': case1, 'case2': case2, }.get(value, default)(name)
In this version, our functions accept a name parameter, which gets passed in when the function is called inside the switch_case function.
Python Switch Equivalent with Class Methods
The final variant that we are discussing today proposes using class methods instead of functions. It may be beneficial when using OOP (Object-oriented programming) in your Python projects.
class Switcher: def default(self): return 'Invalid case' def case1(self): return 'This is the first case' def case2(self): return 'This is the second case' switcher = Switcher() method = getattr(switcher, value, switcher.default) result = method()
In this example, we’ve made a class Switcher with our cases defined as methods. We use getattr() to retrieve the method corresponding to our value. If no match is found, it defaults to the default method.
Python Switch Function with Enumeration
For situations where our case values are enumerated types (like game states or status codes), we can further optimize our switch function using Python’s Enum class.
from enum import Enum class StatusCode(Enum): OK = 'case1' NOT_FOUND = 'case2' def switch_case(value): return { StatusCode.OK: 'This is the first case', StatusCode.NOT_FOUND: 'This is the second case', }.get(value, 'Invalid case')
With this structure, instead of hardcoding case strings, we use enumerated types for better readability and error prevention.
Switch-case Using Lambda Functions
We can bring our Python switch-case function to the next level of simplicity and compactness by using anonymous (lambda) functions instead of named ones.
switch_case = { 'case1': lambda: 'This is the first case', 'case2': lambda: 'This is the second case', 'default': lambda: 'Invalid case', } case = 'case1' result = switch_case.get(case, switch_case['default'])()
Here, we replaced regular functions with lambda expressions, creating a truly Pythonic switch-case scenario in a very few lines of code.
Creating Nested Choices with Python Switch Function
If you lean towards complex structures and algorithms and love challenges, nested switch functions are a splendid choice. Here’s an example:
def switch_case(outer_value): def inner_switch_case(inner_value): return { 'inner_case1': 'This is the first inner case', 'inner_case2': 'This is the second inner case', }.get(inner_value, 'Invalid inner case') return { 'case1': 'This is the first case', 'case2': inner_switch_case, }.get(outer_value, 'Invalid case')
In this case, our function switch_case contains an inner function, inner_switch_case. If ‘case2’ is chosen, a second switch function triggers, expanding the possible outcomes.
Python Switch Function With Objects
Finally, let’s see how to combine a Python switch function with object-oriented principles for dynamic case-to-method resolution.
class Switcher: def case1(self): return 'This is the first case' def case2(self): return 'This is the second case' def default(self): return 'Invalid case' class App: def __init__(self): self.switcher = Switcher() def execute(self, value): method = getattr(self.switcher, value, self.switcher.default) return method() app = App() result = app.execute('case1')
In this example, we have an App class that uses our Switcher class to execute a particular method based on an input value.
Congratulations! You’ve covered quite the journey, learning about Python’s lack of a native switch function and exploring several practical ways to implement it yourself. We hope you’ve found this information useful and are ready to start optimizing your Python scripts with enhanced readability and responsiveness. Keep practicing and happy coding!
Moving Forward: Keep Learning with Zenva
Mastering Python’s switch function is a great stride towards honing your programming skills. But don’t stop there!
Our Python courses include coding basics, object-oriented programming, game development, algorithms, and much more. There’s truly something for everyone!
To provide a comprehensive learning experience, we’ve designed the Python Mini-Degree, a collective set of Python-focused courses covering a broad array of topics.
Start with the basics of coding, then move on to object-oriented programming, algorithms, game development, and app development. The Python Mini-Degree also paves the way to artificial intelligence by letting you create your AI chatbots! The best part? You’ll learn by building your own projects such as games, apps, and chatbots. Our Python Mini-Degree is not just about learning, but about applying what you learn.
Our lessons are interactive, featuring quizzes, live coding sessions, and more. The instructors are experienced coders and game developers, bringing a real-world perspective to the learning experience.
Start your journey today and become a professional in Python, game development, AI, and much more with Zenva. We’re here for you every step of the way, helping you earn certificates, boost your career, and unlock your potential.
Conclusion
You’ve proven your determination and initiative by reaching the end of this tutorial. We’re confident that the knowledge you’ve gained today will enable you to refine your Python scripts through cleaner and more efficient code, thereby giving you the upper hand in your programming journey.
And remember, learning never ends – especially in the tech world. We firmly believe that it’s a continuous journey and hope you’ll join us in further exploring the fascinating realms of Python, game development, AI, and more. Let’s craft codes that inspire, shall we?
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
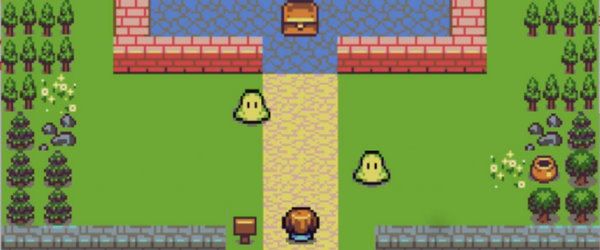
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.