Welcome to this comprehensive guide on the interesting world of Python’s open function. It’s high time we unlocked the powerful potential of this seemingly simple function and understood why it’s a vital component in the toolkit of any budding or experienced developer.
Table of contents
What is the Python Open Function?
This function in Python is part of the standard library. It is used to open a file in read or write mode.
Knowing how to utilize the open function effectively can be a game-changer. Whether it’s to access game data, build configuration files, or even to log information during debug and development stages, it is an essential tool.
Mastering Python’s open function allows you to handle file operations with ease. You could say it’s like knowing how to craft a key that can open a treasure chest of data – a vital skill indeed!
Now that we’ve uncovered what the Python open function is and why it’s an invaluable asset in your developer arsenal, let’s dive deeper into some practical examples to truly grasp its potential. Stay tuned – we’re just getting started.
Using Python Open to Read Files
Let’s jump straight into how you can use Python’s open function to read data from files.
For one, we need to remember the syntax:
file_object = open("filename", "mode")
The ‘filename’ is the name of the file we’re intending to perform operations on. The mode, which is given as a string, tells Python what kind of action we want to carry out.
If we want to just read a file, we’d give the ‘r’ mode:
file = open("testfile.txt", "r") print(file.read()) file.close()
The code above reads the file called ‘testfile.txt’, prints out its contents, and then promptly closes the file. It’s always good practice to close your files once you’re done with them!
Writing to Files with Python Open
Writing to files is as simple as changing the mode to ‘w’ (write). But remember, this will overwrite any existing content in the file:
file = open("testfile.txt", "w") file.write("Hello, Zenva!") file.close()
The ‘w’ mode creates a new file if one with the given name doesn’t exist. However, it only allows writing to the file, and not reading from it.
Appending to Files
What if you wanted to add more data to a file without getting rid of the old stuff? That’s where the ‘a’ (append) mode comes in:
file = open("testfile.txt", "a") file.write("\nWe are learning Python open.") file.close()
Reading and Writing to Files Simultaneously
Finally, we have the ‘r+’ mode which gives us the best of both worlds:
file = open("testfile.txt", "r+") print("Reading initial contents") print(file.read()) print("Writing to the file") file.write("That's it, folks!") print("Reading newly written contents") file.seek(0) print(file.read()) file.close()
This opens a file for both reading and writing and prints out the contents before and after writing to it. We use the “seek” function here to set the file’s current position at the offset (0 in this case).
That wraps up our guide on using the Python ‘open’ function to handle file operations. By getting a grip on this command’s functionality, you’re well on your way to carrying out more complex actions with files. Happy coding!
Opening Files Using Python Open With Block
When dealing with file operations, it’s crucial to ensure that the file is properly closed after use. A neat way to achieve this without explicitly calling the close method is by using the Python open function with a ‘with’ block. This will automatically close the file once the operations within the block are completed.
with open("testfile.txt", "r") as file: print(file.read())
Reading Data Line by Line
Python open function offers a method called ‘readline()’, which allows us to read individual lines in a file, one at a time. Especially handy when working with large files.
with open("testfile.txt", "r") as file: line = file.readline() while line: print(line) line = file.readline()
This will read each line individually, print it, and continue until there are no lines left to read.
Use Python Open to Create a File
If you want to create a file but not open it for writing, you can use the open function with ‘x’ (exclusive creation) mode, which will create the file but return an error if the file already exists.
file = open("newfile.txt", "x") file.close()
Writing Multiple Lines to a File
If you have a list of strings and you want to write each one as a new line in your file, you can use ‘writelines()’. This is different from ‘write()’, which simply writes a single string to the file.
lines = ["Hello, Zenva!", "We are learning Python open."] with open("testfile.txt", "w") as file: file.writelines([f'{line}\n' for line in lines])
This code will write each string in the ‘lines’ list as a new line to the ‘testfile.txt’ file.
If you’re working on large-scale projects or complex games, it’s likely that you’ll be dealing with a lot of file operations. Invest time in truly mastering Python’s ‘open’ function – it’s a staple in any programming toolbox and a skill set worth cultivating.
Where To Go Next With Python Open
Armed with the information in this guide, you’re now well-equipped to handle file operations using Python’s open function. However, your learning journey doesn’t stop here!
Here at Zenva, we believe in extending the boundaries of knowledge. Empowering individuals with coding skills is our mission, and we strive to make that happen from beginner to professional levels of learning.
If you are looking to dive deep into Python programming, consider our Python Programming Mini-Degree. This course collection is a comprehensive guide to Python programming covering essentials like coding basics, algorithms, object-oriented programming, and many more.
You’ll also get to explore game development with Python and creating stunning apps using popular Python libraries and frameworks. The courses feature projects including game creation, app development, and AI chatbot development.
If you are past the basics and consider yourself an intermediate learner, check out our collection of advanced Python courses to further enhance your skills.
Our courses are self-paced and accessible on all modern devices. This means you can learn when it’s most convenient for you, and at a pace that suits your style. With Zenva, the learning experience comes with engaging video lessons, interactive content, and the reward of completion certificates.
Conclusion
There you have it – a deep dive into Python’s powerful and versatile ‘open’ function. Grasping this fundamental concept will boost your file handling capabilities, turning you into a more efficient and proficient Python programmer. From creating and writing to files, to reading and appending to them, and even automating their everyday management – you’ve just unfolded a host of new possibilities with Python.
As you journey further into the world of Python and coding in general, remember that we at Zenva are here to guide and support you on your mission. Whether you’re looking to start fresh, upgrade your skills, or move into a specialized domain – our comprehensive and easy-to-follow courses will be your trusted companions. The world of coding is vast and full of excitement, and we’re thrilled to be part of your journey. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
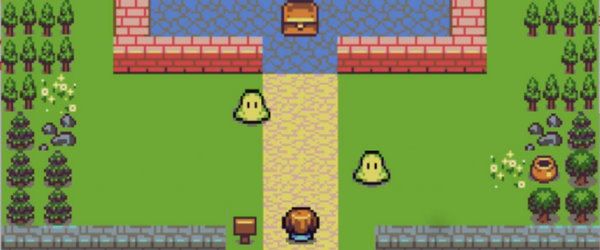
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.