Ever had a day when you can’t decide what game to play, what to code, or which Python concept to grasp next? Think it would be neat if your computer could take those decisions with some logic on its own? That’s the magic of Python’s “if” statement! It allows our code to simulate decision-making, thereby automating many tasks. This tutorial guides you through the fundamentals of Python’s “if” statement, explaining its engaging, valuable, and useful aspects.
Table of contents
What is Python’s If Statement?
Python’s “if” statement is a conditional statement that implements decision-making. It delivers output actions based on specified conditions. If a condition holds true, the statement will execute a block of code. It’s as simple as saying “If it’s cloudy, I’ll stay inside and code.”
Python’s “If” statement is a prime component in creating interactive programs and games. It introduces logic into our code, allowing it to make decisions just like we do in our daily lives.
Mastering Python’s “if” statements represents a significant stride in your coding journey. It’s a key step towards writing sophisticated and dynamic programs. And the best part? It’s a concept that is transferrable to nearly every other programming language. Acquiring this skill will make you more versatile and nimble as a programmer.
Getting Started with Python’s If Statement
Python’s “if” statements are pretty straightforward. You start with the key word “if”, followed by your condition, and then a colon. Let’s build a very simple program using an “if” statement.
x = 50 if x > 10: print("Yes, x is greater than 10!")
This code simply checks if variable x is greater than 10. If so, it prints “Yes, x is greater than 10!”.
Introducing Else
What if we want our program to do something else, when x is not greater than 10? That’s where Python’s “else” statement comes in needed.
x = 5 if x > 10: print("Yes, x is greater than 10!") else: print("No, x is not greater than 10.")
In this example, since x is not greater than 10, the “else” part of the statement triggers, and the output reads: “No, x is not greater than 10.”
Elif For Multiple Conditions
Let’s say we want to program our very own adventure game. We can use Python’s “elif” statement to cover more than just two possibilities. This gives us greater flexibility and creativeness with our codes.
weather = "sunny" if weather == "rainy": print("Stay home and code.") elif weather == "sunny": print("Go out and enjoy the day, then code.") else: print("Keep calm and continue coding.")
In this example, since the weather is “sunny”, the program doesn’t execute the “if” or “else” statements but the “elif” condition, hence the output reads: “Go out and enjoy the day, then code.”
Nesting If Statements
Much like in our everyday life decision-making process, Python’s “if” statements can be nestled within each other to make more complex decisions.
x = 20 y = 10 if x == 20: if y == 10: print("Both conditions are true!")
Here, the code checks if x is equal to 20; if true, it proceeds to check if y equals 10. If both the conditions are true, it prints: “Both conditions are true!”.
So now you know Python’s ‘if’ statement and how it behaves in different conditions. This directly helps you design more dynamic and customizable programs.
Using Operators in If Statements
You can use comparison, logical and membership operators in if statements to make more complex decisions in your Python programs.
Comparison Operators
Comparison operators are used to compare two values. Let’s experiment with a couple of examples.
x = 5 y = 10 if x x: print("y is greater than x") if y != x: print("y and x are not equal")
Logical Operators
Logical operators provide a means of making decisions based on multiple conditions. Python supports “and”, “or” and “not” logical operators. Let’s see them in action.
x = 5 y = 10 z = 20 if x x: print("Both conditions are True") if x z: print("At least one of the conditions is True") if not(x == y): print("x is not equal to y")
Membership Operators
Operators such as “in” and “not in” can be used to check if a particular item exists in a container such as list, tuple, etc.
languages = ["Python", "Java", "C++"] if "Python" in languages: print("Python is in the list!") if "Ruby" not in languages: print("Ruby is not in the list!")
As seen, the if statement in combination with these operators can lead to some very intriguing and dynamic code structures.
Python’s If Statement in Functions
You can also use Python’s “if” statement within a function. This allows us to create flexible functions that can adjust their behavior based on the arguments they receive.
def greet(name, time_of_day): if time_of_day == "morning": return "Good morning, " + name else: return "Hello, " + name print(greet("Alice", "morning")) print(greet("Bob", "evening"))
This function, depending upon the time of the day it receives, will greet the person accordingly.
Leveraging If Statements in Loops
If statements can be strategically used in loops to control the flow of iteration based on certain conditions.
for i in range(1, 10): if i%2 == 0: print(str(i) + " is even") else: print(str(i) + " is odd")
In this program, we are using a for loop to check if the number is odd or even and print it accordingly.
As we explore Python’s if statement, we find that the possibilities it brings to programming are immense. Whether it’s simplifying the flow of code or making programs more dynamic, if statements are powerful tools every Python programmer should master.
What’s Next in Your Python Journey?
The world of Python has so much more to offer, and we’re here to guide you every step of the way. Python holds key leveraging positions in numerous industries, including space exploration, MedTech, and robotics. This underscores its powerful capabilities and its invaluable role in today’s tech world. Where to head next in your Python journey?
One resource we highly recommend is our comprehensively designed Python Mini-Degree.
This mini-degree is a collection of courses that provides a deep dive into Python programming. You’ll learn everything from coding basics, algorithms, object-oriented programming, to game development and real-world app development. The best part? You’ll be learning by creating your own games, solving problems using algorithms, and building real-world applications through step-by-step projects.
This mini-degree allows students to learn at their own pace without any time limits or deadlines. It introduces industry-relevant skills that are highly sought after in the job market. Our curriculum is regularly updated to keep you abreast of the latest industry practices. Moreover, our students have successfully changed their careers, landed their dream jobs, or started their own businesses after completing our courses.
Our Python courses are meticulously designed to cater to both beginners and experienced programmers, making us a one-stop learning platform for all things Python.
Conclusion
As we conclude, we hope this deep dive into Python’s if statement has not only improved your Python skills but also enkindled in you the unquenchable fire to keep learning. With every new Python concept and function you master, you know you are one step closer to creating engaging games, advanced web applications, or even intuitive AI systems, all catered to make lives better and simpler.
So don’t wait! Start your in-depth learning adventure with the expertly crafted Python Mini-Degree at Zenva, and step into the fascinating world of Python programming. Your passion and our guidance will make an unbeatable combination for coding success. Happy learning!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
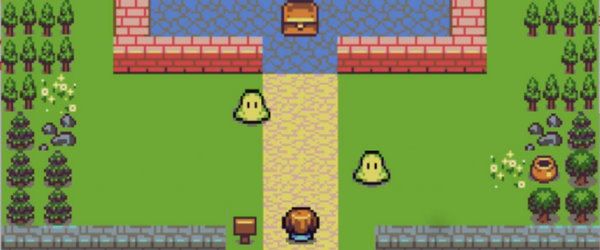
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.