Welcome to an exciting and comprehensive guide to Python Heap in collections. Through this beginner-friendly tutorial, we aim to empower you with a deeper understanding of this crucial concept. Whether you plan to dig into game development, AI, or simple scripting, getting a grip on Python’s Heap module is not just advisable, but essential. Don’t worry, we’ve got you covered!
Table of contents
What is Python Heap in Collections?
The heap module in Python’s collections library introduces functionalities for creating min heap and max heap data structures. Distinct from regular arrays or lists, heaps come with special properties, which make them valuable in various scenarios.
Why Heap is a Must-Learn Topic?
Heaps are especially beneficial when you want efficient data retrieval in your code. This is the key to creating fluid, responsive applications, games, or scripts. To illustrate, in the world of game development, heaps are utilised to manage game states or player objects.
From a broader perspective, understanding heaps is crucial for mastering data structures and algorithms, foundational knowledge for any budding programmer. They also frequently appear in coding interviews, which makes them all the more important to get to grips with.
Moving beyond traditional array manipulation, heaps introduce a more advanced, yet efficient, way of dealing with large datasets. By mastering Heap in Python collections, you’ll be able to construct more robust, efficient and scalable code, opening doors to higher-level programming challenges and projects.
A Look at Basic Heap Operations
Heaps are unique data structures that maintain a certain property in every element. Min heaps store the smallest element at the root, while max heaps store the largest. This makes it possible to quickly find the maximum or minimum value.
Here, we’ll examine several basic operations using Python’s heap module in collections.
Creating a Heap
import heapq # creating an empty heap heap = [] # using heapify() to convert list into heap heapq.heapify(heap) print("Empty Heap:", heap)
This will output an empty heap. But what if we want to create a heap with values?
import heapq # initializing list li = [5, 7, 9, 4, 3] # using heapify() to convert list into heap heapq.heapify(li) print("Heap:", li)
Inserting into Heap
To insert an element, we use the heappush() function, which preserves the heap property.
import heapq # initializing list li = [5, 7, 9, 4, 3] # using heapify() to convert list into heap heapq.heapify(li) # using heappush() to push elements into heap # pushes 6 heapq.heappush(li, 6) print("Heap after pushing:", li)
Removing from Heap
Use the heappop() function to remove and return the smallest element from the heap.
import heapq # initializing list li = [5, 7, 9, 4, 3] # using heapify() to convert list into heap heapq.heapify(li) # using heappop() to pop smallest element print("Popped item:", heapq.heappop(li)) print("Heap after popping:", li)
These are some basic operations that you can perform with heaps in Python’s heap module.
Advanced Heap Operations in Python
After understanding the basic functionalities of Python Heap module, let’s now dig deeper and discover more robust features.
Push and Pop Simultaneously
To push an item on the heap and then pop and return the smallest one, we use the heappushpop() function.
import heapq # initializing list li = [5, 7, 9, 4, 3] # using heapify() to convert list into heap heapq.heapify(li) # using heappushpop() to push and pop items simultaneously print("Popped item :", heapq.heappushpop(li, 2)) print("Heap after puspop:", li)
Pop and Push Simultaneously
Use the heapreplace() function to pop and return the smallest element, and then push a new item onto the heap.
import heapq # initializing list li = [5, 7, 9, 4, 3] # using heapify() to convert list into heap heapq.heapify(li) # using heapreplace() to push and pop items simultaneously print("Popped item :", heapq.heapreplace(li, 2)) print("Heap after replace:", li)
Find the Largest n Elements
To get the ‘n’ largest elements from a heap, the function nlargest() comes in handy.
import heapq # initializing list li = [5, 7, 9, 4, 3] # using heapify() to convert list into heap heapq.heapify(li) # using nlargest to print 3 largest numbers print("Three largest numbers are :", heapq.nlargest(3, li))
Find the Smallest n Elements
Similarly, to fetch the ‘n’ smallest elements, we use the function nsmallest().
import heapq # initializing list li = [5, 7, 9, 4, 3] # using heapify() to convert list into heap heapq.heapify(li) # using nsmallest to print 3 smallest numbers print("Three smallest numbers are :", heapq.nsmallest(3, li))
By understanding the above heap operations, you will be able to effectively utilize the heap module in your Python applications, thereby improving your code’s efficiency.
Where to Go Next?
Adventure is just starting! Python, with its efficient libraries and versatile capabilities, offers so much more to explore and master. As you have embarked on this fascinating journey, the next step is to foster your skills further.
We at Zenva understand the challenges learners face while studying new languages or technologies. Hence, we have curated a comprehensive Python Mini-Degree course, designed to cement your foundation and then gradually elevate your skills.
This Python Mini-Degree is more than a set of tutorials. It offers an inclusive approach to Python programming, involving coding basics, algorithms, and object-oriented programming. Also, you get to do hands-on with game development, and app development.
Features of our Python Mini-Degree
- Rich, step-by-step projects let you create games, algorithms, and real-world apps.
- Designed for beginners, yet includes challenges and quizzes for experienced programmers.
- 24/7 access on any device ensuring flexibility and convenience.
- Video lessons, course printouts, interactive lessons, and practice with source code to reinforce learning.
- Earn your completion certificates to showcase your progress.
If you are looking for a broad collection of Python resources, you can explore our list of Python courses. Regardless of whether you are a beginner or looking to attain a professional mastery level, our courses aim to cater to every level of expertise.
Conclusion
Python’s Heap module opens a world of possibilities for efficient and powerful data manipulation in your code. The journey to becoming a seasoned Python Developer is thrilling, challenging, and empowering. Through this tutorial, we’ve witnessed a glimpse of Python’s prowess, and there’s so much more to discover!
Ready to dive deeper? Join us at Zenva’s Python Mini-Degree course and immerse yourself into a comprehensive learning experience. This is where we bid you adieu and welcome you to a keener, brighter world of coding expertise. Happy Coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
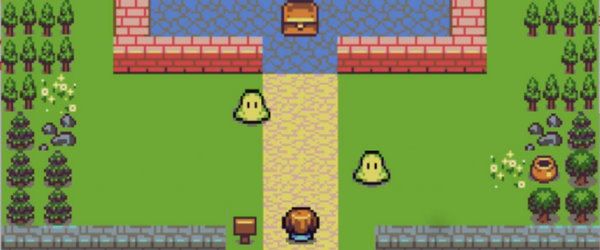
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.