In every discipline, good documentation is crucial. It becomes even more so in the world of programming. As a coder, always remember, code is more often read than written! Understanding how to document your Python code effectively is a key skill to develop in your coding journey, it enhances both readability and reusability of your code.
Table of contents
What is Python Code Documentation?
Python code documentation is about giving clear, precise details about what your code does. This is incredibly useful for anyone who might use your code later on, which could even be you.
Documentation serves as a guide, literally speaking. Not only does it enrich the reader’s understanding of your code, but also results in better code sustainability.
Why Should I Learn It?
Imagine you stumble upon an amazing piece of code on GitHub, but with no documentation. No explanation about what the code does, or how it does it. It’s like getting a map without any street names. You’ll see what’s there, but you wouldn’t know how to navigate. Learning good documentation habits helps prevent this, making your code user-friendly. So let’s dive in to feel the power of well-documented code in Python.
Documenting a Function
A single-line docstring can document a simple function:
def add_numbers(a, b): """Adds two numbers and returns their result.""" return a + b
To access the docstring, use the `.__doc__` attribute.
print(add_numbers.__doc__)
This will print:
“`
Adds two numbers and returns their result.
“`
Documenting a Function with Arguments
You can also document each parameter in the function:
def multiply_numbers(a, b): """ Multiplies two numbers and returns their result. Args: a: The first factor of the equation. b: The second factor of the equation. Returns: The result of the multiplication. """ return a * b
And you can access the docstring the same way:
print(multiply_numbers.__doc__)
Documenting Modules and Classes
You can also use docstrings to document entire modules or classes. Here is an example:
class Calculator: """ A simple calculator. This calculator can add, subtract, multiply, and divide. """ def add(self, a, b): """ Adds two numbers. Args: a: The first number. b: The second number. Returns: The sum of the two numbers. """ return a + b # etc...
Like with functions, to access the class docstrings, you’d use:
print(Calculator.__doc__) print(Calculator.add.__doc__)
Documenting with Single Line Docstrings
Python’s single line docstrings are super simple ways to document your functions, modules or classes. They work best for uncomplicated, clear-cut functions.
def square_root(n): """Take the square root of a number.""" return n**0.5
Documenting with Multi-line Docstrings
When single line docstrings are not enough, you can use multi-line docstrings for detailed documentation.
A typical layout for a multi-line docstrings is as below:
def complex_power(base, exp): """ Calculate the power of a complex number. Args: base : Complex number as the base. exp : Power to be raised to. Returns: Resultant complex number. """ return base ** exp
Documenting Classes
When documenting a class, you should also document its methods, attributes, and exceptions (if any).
class Circle: """ Represents a circle. Attributes: radius : radius of the circle. Methods: area : calculate area of the circle. perimeter: calculate perimeter of the circle. """ def __init__(self, radius): """ Initialize a Circle instance. Parameters: radius: The radius of the circle (default 1.0). """ self.radius = radius def area(self): """ Calculate and return the area of the circle. """ return 3.1415926 * self.radius ** 2 def perimeter(self): """ Calculate and return the perimeter of a circle """ return 2 * 3.1415926 * self.radius
Documenting Modules
Module level docstrings should include a high level description of the module’s purpose, along with any globally available objects.
""" This is the example module. This module is made for demonstration purposes on how to document a module. It contains the Square class for creating square objects. """ class Square: """Creates square objects.""" pass
As you can see, taking time for documentation can make a significant difference in maintaining and reusing code. We hope this tutorial has sparked your interest in always documenting your Python code meticulously!
Where to Go Next?
You’ve just taken your first steps into the powerful world of Python code documentation, but your journey in mastering Python language skills doesn’t stop here.
To keep enhancing your Python knowledge, check out our Python Mini-Degree at Zenva. This comprehensive collection of courses covers a wide range of topics including coding basics, object-oriented programming, game development, and app development. You have the opportunity to learn by creating your own games, algorithms, and real-world apps!
Regardless of whether you’re a beginner just stepping into the programming world or an experienced developer looking to diversify your skillset, this mini-degree is crafted to cater to all levels. Plus, you get to build projects that you can showcase in your Python portfolio.
If you’re looking to delve deeper into the Python language, refer to our broad range of Python courses which are structured to progressively scale your learning curve.
Conclusion
Whether you are a coding novice or a seasoned developer, clear and precise documentation can set you apart. The ability to effectively document and understand your Python code is a crucial skill that will enhance your versatility and value as a coder. It’s a stride that ushers you into the domain of professional programming, allowing your work to shine amongst peers and make significant strides in your coding journey.
Remember, at Zenva, your path to learning never ends. Should you choose to continue on this exciting path of code documentation, or pivot to another challenging and interesting coding topic, we’re here to aid your journey. Our comprehensive Python Mini-Degree is designed to cater to all levels and help you grow as a professional programmer. Join us and take your coding career to new heights! Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
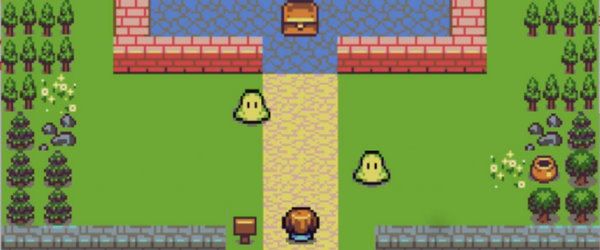
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.