Here’s your engaging tutorial about Python Code Coverage.
Python, the versatile programming language, is a vital tool of choice for many developers. As is the case with any code, ensuring the quality and thoroughness of the code written in Python is fundamental to the overall success of any given project. This is where learning about Python Code Coverage comes into play.
Table of contents
Understanding Python Code Coverage
Python Code Coverage is a form of software testing that gauges the degree to which the source code of a program or an application has been tested. This is quantified in percentages, illustrating how much of your Python code is covered, or exercised, by your test suite.
It’s crucial to learn and implement Python Code Coverage for numerous reasons, such as:
- Quality Assurance: With complete coverage, you flag any part of the code that could possibly lead to bugs.
- Code Refactoring: It gives confidence to refactor the code, safeguarding against unnoticed changes.
- Clear Metrics: It provides a clear metric to indicate the proportion of code base validated by tests.
Essentially, Python Code Coverage is like a game where your objective is to achieve the highest coverage percentage ensuring optimal performance, security, and reliability.
Getting Started with Python Code Coverage
Before we dive into Python Code Coverage examples, let’s set up our environment. Python provides the ‘coverage’ library to aid us in measuring code coverage. Let’s install ‘coverage’ by executing the following command:
pip install coverage
Basic Usage of Coverage
For the basic usage of the coverage command, consider this simple Python script (‘example.py’):
def add(a, b): return a + b def subtract(a, b): return a - b print(add(5, 3))
We have a code (example.py) that contains two functions: add and subtract. But, we have only used the “add” function, not “subtract”. To check the coverage of this code, we can use the “coverage” command:
coverage run example.py
This command generates a ‘.coverage’ data file. To check coverage report, we use:
coverage report -m
The ‘-m’ flag prints the line numbers of the missing statements. Your output would be showing less than 100% coverage, as we entered but did not execute the “subtract” function.
Improving Coverage
To improve coverage, let’s modify example.py to use the “subtract” method.
def add(a, b): return a + b def subtract(a, b): return a - b print(add(5, 3)) print(subtract(5, 3))
If we rerun the “coverage” command as earlier, this time we can see the coverage is 100%.
Excluding Certain Parts
There can be certain parts of your code that you don’t want to test. You can exclude those parts from coverage testing using special comments.
Code example:
def add(a, b): return a + b def subtract(a, b): # pragma: no cover return a - b print(add(5, 3)) print(subtract(5, 3))
In the above code, we have taken the “subtract” function out of the coverage using “# pragma: no cover”. Even though we are using this function, our coverage report will show 100%.
Including Multiple Files
In a real-world project, you’ll likely have multiple Python files. The ‘coverage’ library supports multi-file testing with ease.
coverage run file1.py file2.py
Enabling multi-file testing is as simple as including the other file names in your command.
Generating an HTML Report
In addition to the console, you can generate a handy HTML report of your coverage data.
coverage html
This command creates an ‘htmlcov’ directory, containing an interactive HTML site with your coverage data. In the index.html file, you can see the coverage percentage and drill down by file and line.
Conditional Coverage
Some parts of your code may only be executed under certain conditions. Such scenarios can be tested using Python’s ‘if-elif-else’ statements.
def operation(a, b, operation): if operation == "add": return a + b elif operation == "subtract": return a - b else: pass
By using this structure and creating conditions to test each case, you can ensure 100% code coverage.
print(operation(5, 3, "add")) print(operation(5, 3, "subtract"))
Branch Coverage
Sometimes, line coverage isn’t enough. You might have conditional statements, like ‘if-else’, being partially executed. ‘coverage.py’ offers ‘branch coverage’ for these situations.
To enable branch coverage, add the ‘–branch’ flag:
coverage run --branch myfile.py
This results in a more accurate assessment of your code’s test coverage, accounting for all possible branches and their execution or non-execution.
Configuring Coverage
For more complex setups, ‘coverage.py’ accepts a ‘.coveragerc’ configuration file where you can specify things like data file location, reporting precision, or which files to include/exclude.
Remember, Python Code Coverage isn’t about striving for 100% fulfilment, but about writing better, cleaner, and well-tested code. Understanding the parts of your code tested and working as expected is crucial for reliable software development. Code coverage gives us valuable insight into our code and helps ensure its quality. At Zenva, we believe in producing high-quality code and this is why we place such importance on tools like Python Code Coverage.
Where to Go Next with Python?
You’ve learnt about Python Code Coverage, a vital tool in your programming toolkit. But the landscape of Python is vast and full of exciting possibilities. Wondering where to head next on your Python journey? It’s time to dive deeper into Python and broaden your horizons.
Whether you’re a beginner or a seasoned programmer looking to harness the power of Python, we encourage you to explore our Python Mini-Degree at Zenva Academy. This collection of courses covers Python programming in-depth, offering an exciting blend of coding basics, algorithms, object-oriented programming, game development, and app development.
Our Python Mini-Degree is designed to hone practical skills. The curriculum includes hands-on, interactive lessons, quizzes, and coding tasks. You’ll build your Python portfolio by creating your games, algorithms, and real-world apps.
The advantage of learning Python with Zenva Academy is not just the rich and diverse course content. It’s also about flexibility. You can access courses anytime, anywhere, fitting them around your lifestyle.
You can also further enrich your journey with Python by browsing our Python courses for intermediate users as well.
Conclusion
Python Code Coverage is an invaluable tool in your developer toolkit, fostering better coding practices, spotting potential errors, and boosting code quality. However, it’s just the tip of the iceberg when it comes to the opportunities Python has to offer. From web development to data science, from applications to games, Python is the gateway to a vibrant and satisfying programming career.
Embrace your journey into Python mastery by delving into our Python Mini-Degree or any of our specific Python courses. At Zenva Academy, we help you develop real-world skills through engaging, high-quality content. Empower your coding journey with Zenva and unlock a world of exciting coding opportunities. Your journey into the vast and versatile world of Python starts here!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
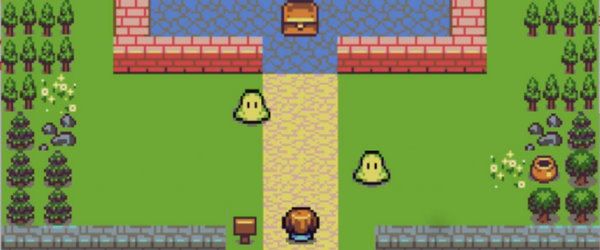
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.