Welcome to this tutorial on Python and Game Mechanics! This is the perfect place to delve into the world of game development and Python programming. We’ve tailored this guide to be suitable for beginners, although veterans may also find this information to be a great refresher or reference point!
Table of contents
What is Python?
Python is an easy-to-learn, interpretative and high-level programming language that is popular for a multitude of tasks, including game development. With its clean syntax and powerful features, it’s the perfect choice for beginners.
Decidedly, you may wonder why you should use Python for game mechanics. Well, Python has a rich ecosystem of libraries and frameworks that simplify the game development process. These libraries include Pygame, Python Arcade, and Panda3D, just to name a few!
Acquiring Python skills for game mechanics ensures you are well-prepared to handle the logistics and structure in designing a game. You learn how to code movements, create game loops, spend less time debugging, and more time creating!
By learning Python and Game Mechanics, you will be joining a large and supportive community of developers and will be at the forefront of an industry that consistently seeks new talents!
Now that the introductions are out of the way, let’s move ahead and dive into some exciting Python coding!
Beginning with Python and Game Mechanics
One common feature in games is a scoring system. Let’s look at how you could implement this in Python.
# Define initial score score = 0 # Function to increment score def increment_score(): global score score += 1 print("Current score:", score) # Call function to increase score increment_score()
This is a simple yet essential game mechanic! We’ve just used Python to create a scoring system. The concept of ‘functions’ is applied, which is a powerful feature in Python, allowing us to reuse pieces of code. In this example, we’ve made a function that, when called, increases the score of the game.
Moving Forward with Python and Game Mechanics
Now, let’s try to code a basic character movement. This is a key gameplay mechanic used in numerous video games, from platformers to RPGs. Let’s create a simple game where a character can move left or right.
# Create class for game character class GameCharacter: def __init__(self): self.position = 0 # Method to move left def move_left(self): self.position -= 1 # Method to move right def move_right(self): self.position += 1 # Create a character character = GameCharacter() # Move character right character.move_right() print("Character Position:", character.position) # Output: Character Position: 1
We’ve just created a game character who can move left and right using Python’s class system and methods. Python shines in its ability to create interactive mechanics in a quick and straightforward way.
Setting Up the Game
Let’s start by creating a simple game setup. We’ll initialize a game character at a position and create a function to display the character’s current position.
class GameCharacter: def __init__(self, name): self.name = name self.position = 0 def display_position(self): print(self.name, "is at position", self.position) character = GameCharacter("Bob") character.display_position() # Output: Bob is at position 0
Reacting to Player Input
Now, let’s make our character Bob move when a player inputs either ‘left’ or ‘right’.
# Add 'move' functionality to GameCharacter class class GameCharacter: def __init__(self, name): self.name = name self.position = 0 def display_position(self): print(self.name, "is at position", self.position) def move(self, direction): if direction == "left": self.position -= 1 elif direction == "right": self.position += 1 self.display_position() character = GameCharacter("Bob") character.move("left") # Output: Bob is at position -1
By reacting to player input, we are making the game interactive! Now, ‘Bob’ can move in the direction the player inputs.
Adding Obstacles
Let’s add some complexity to the game by introducing obstacles. If our character Bob encounters an obstacle when moving right, we’ll have him stop and then print an alert message.
#Add 'obstacle' functionality to GameCharacter class class GameCharacter: def __init__(self, name): self.name = name self.position = 0 self.obstacles = [2, 3, 5] # Define positions of obstacles def display_position(self): print(self.name, "is at position", self.position) def move(self, direction): if direction == "left": self.position -= 1 elif direction == "right": if self.position + 1 in self.obstacles: print("Obstacle encountered! Can't move right.") return self.position += 1 self.display_position() character = GameCharacter("Bob") character.move("right") # Output: Bob is at position 1 character.move("right") # Output: Obstacle encountered! Can't move right.
We’ve added another layer to the game – obstacles! This creates a more challenging gameplay experience.
Power-ups and Health
Another common game mechanic involves power-ups that improve the player’s abilities or health. Let’s add a health attribute to our character Bob, and a power-up that increases his health when spotted.
#Adding Power-ups and Health to GameCharacter class class GameCharacter: def __init__(self, name): self.name = name self.position = 0 self.health = 100 self.power_ups = [4] # Position of power-ups def display_position(self): print(self.name, "is at position", self.position) def move(self, direction): if direction == "left": self.position -= 1 elif direction == "right": if self.position + 1 == self.power_ups[0]: self.health += 50 print("Power-up collected! Health is now", self.health) self.position += 1 self.display_position() character = GameCharacter("Bob") character.move("right") # Bob moves to position 1 character.move("right") # Bob moves to position 2 character.move("right") # Bob collects power-up, health increases
With that, we introduced the concept of power-ups, and now ‘Bob’ can increase his health when he encounters a power-up!
Setting Up Levels
Adding different levels to our game will improve its depth. Levels can be categorized by different challenges, enemies and rewards. Let’s start by creating a basic level structure.
# Defining a Level Class class Level: def __init__(self, level_number): self.level_number = level_number self.is_completed = False def complete_level(self): self.is_completed = True print("Level", self.level_number, "completed!") level1 = Level(1) level1.complete_level() # Output: Level 1 Completed
Adding Combat Mechanics
Adding combat mechanics can make games more engaging and stimulating. Let’s assume that Bob will encounter an enemy at a certain position, and when they meet, a battle ensues.
#Adding Combat Mechanics to GameCharacter class import random class GameCharacter: def __init__(self, name, health): self.name = name self.position = 0 self.health = health self.enemies = [5] # Define positions of enemies def move(self, direction): if direction == "right": self.position += 1 if self.position in self.enemies: self.fight() def fight(self): damage = random.randint(1, 50) self.health -= damage print(self.name, "fought an enemy and received", damage, "damage!") print("Current health:", self.health) character = GameCharacter("Bob", 100) character.move("right") # Bob moves to position 1 character.move("right") # Bob moves to position 2 character.move("right") # Bob fights an enemy at position 5
Enhancing Combat with Power-ups
Power-ups can enhance the player’s ability to fight. Here, Bob will pick up a power-up that doubles his strength in the next battle.
# Enhancing Combat with Power-ups in GameCharacter class class GameCharacter: def __init__(self, name, health): self.name = name self.position = 0 self.health = health self.enemies = [5] # Define positions of enemies self.power_ups = [4] # Position of power-ups self.strength = 2 def move(self, direction): if direction == "right": if self.position + 1 in self.power_ups: self.strength *= 2 print("Power-up collected! Strength is now", self.strength) self.position += 1 if self.position in self.enemies: self.fight() def fight(self): damage = random.randint(1, 50) self.health -= damage/self.strength print(self.name, "fought an enemy and received", damage/self.strength, "damage!") print("Current health:", self.health) character = GameCharacter("Bob", 100) character.move("right") # Bob moves to position 1 character.move("right") # Bob moves to position 2 character.move("right") # Bob collects power-up, strength increases character.move("right") # Bob fights an enemy at position 5
Adding an Enemy Character
Games become more engaging if the enemy characters have their own characteristics and abilities. Here, let’s assume that instead of losing a random amount of health, Bob will compete with an enemy character in battle.
#Adding an enemy character class GameCharacter: def __init__(self, name, health, strength): self.name = name self.position = 0 self.health = health self.strength = strength def move(self, direction): if direction == "right": self.position += 1 if self.position in enemy.positions: self.fight(enemy) def fight(self, enemy): damage = enemy.strength self.health -= damage print(self.name, "fought an enemy and received", damage, "damage!") print("Current health:", self.health) class Enemy: def __init__(self, name, positions, strength): self.name = name self.positions = positions self.strength = strength enemy = Enemy("EvilMonster", [5], 25) character = GameCharacter("Bob", 150, 2) character.move("right") # Bob moves to position 1 character.move("right") # Bob moves to position 5 and fights with EvilMonster
Where to Go Next?
Congratulations! You have made it this far in our journey of Python and game mechanics, building an understanding of levels, combat, powerups, and artificial intelligence enemies. So what comes next? This is not the end of your learning journey, but rather a beginning, a springboard into wider horizons.
Looking to dive deeper into Python or switch careers? Our Python Mini-Degree is an in-depth bundle course perfect for you!
- Get into the nitty-gritty of Python programming, covering everything from the basics to advanced topics
- Understand algorithms and object-oriented programming
- Experiment with game and app development using popular libraries like Pygame, Tkinter, and Kivy
- Flexible learning options and project-based assignments for hands-on experience
- Access to constant updates corresponding to the latest industry trends
- Equip yourself with high in-demand skills in Python, the most preferred coding language in various fields, particularly in data science
For a broader perspective, check out our Python Courses Collection.
This collection offers a wide range of courses in Python programming, covering specific topics such as data analysis, machine learning, and more. All courses, like the rest of Zenva’s offerings, offer project-based learning and support from experienced mentors.
Conclusion
Throughout this tutorial, we’ve explored the fascinating side of Python and its application in game mechanics. We’ve demonstrated how Python, with its simplicity and flexibility, can be used to create engaging game mechanics. From building scoring systems to coding character movements, from setting up exciting levels to shaping thrilling combats, with Python, the game development journey is always engaging.
But don’t let the exploration stop here! We, at Zenva, are always here to fuel your learning journey further. So why not check out our Python Mini-Degree to deepen your knowledge and skills? Keep going, keep learning, and remember, the beautiful thing about learning is that no one can take it away from you!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
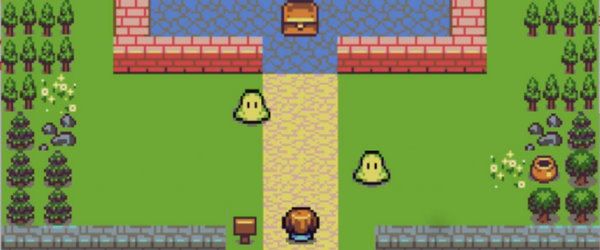
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.