Learning to create games involves understanding various important concepts and one of them is collision detection. In the gaming world, making sure entities react when they interact with each other is critical. It is what makes the game realistic and interactive. Welcome to our pygame collision detection tutorial, where play, learning, and code intertwine to equip you with the necessary expertise in this exciting Python feature.
Table of contents
What is Pygame Collision?
The pygame module is a cross-platform set of Python libraries designed for game development. Collision detection in pygame is the programming strategy of detecting when two or more objects collide, or meet, in the game environment.
Why is Pygame Collision Significant?
Pygame collision is a concept that brings our games to life. Without collision detection:
- Balls would drift through walls.
- Spaceships would fly through planets.
- Characters could walk through obstacles, making the game less realistic and engaging.
Hence, for anyone passionate about game development, understanding and implementing collision detection in pygame is a vital skill to have in your toolkit.
Benefits of Mastering Pygame Collision
While pygame collision may seem complex, the advantages of mastering it far outweigh the perceived challenges.
- Improve Game Dynamics: It enhances the dynamics of a game making it more exciting and engaging.
- Realistic Gaming Environment: It provides interactivity and the ability to navigate real-world scenarios.
- Expand Your Toolkit: Numerous games, both 2D and 3D, employ collision detection, making the skill quite valuable.
Our tutorial today seeks to break down this concept in an accessible way for both beginners and advanced coders. Stay tuned and embrace the fun side of learning about pygame collision detection.
Setting Up the Pygame Environment
Before we work with collision detection, we need to set up our pygame window. Here is a basic example to create a window with a title and specific dimensions:
import pygame pygame.init() win = pygame.display.set_mode((800, 600)) pygame.display.set_caption("Collision Detection in Pygame")
In this code snippet, we’ve initialized Pygame and set the window dimensions to 800 pixels across and 600 pixels down.
Creating Game Entities
Next, let’s define our game objects. For simplicity, we’ll use rectangles as our game objects:
rec1 = pygame.Rect(50, 50, 50, 50) rec2 = pygame.Rect(150, 150, 50, 50)
In this example, we’ve defined two rectangles: rec1 and rec2. Each rectangle is defined by its x and y positions (top left corner), as well as width and height. The first two arguments represent the coordinates of the top left corner, and the latter two represent the rectangle’s width and height, respectively.
Rendering Game Entities
Before testing a collision, we need to display our rectangles. We’ll do this by filling our window with a color and then drawing our rectangles on it:
win.fill((0, 0, 0)) pygame.draw.rect(win, (255, 0, 0), rec1) pygame.draw.rect(win, (0, 255, 0), rec2) pygame.display.update()
Here, we first fill the window “win” with black. Then, the red rectangle “rec1” and the green rectangle “rec2” are drawn on top. Finally, we update the display so the rectangles become visible.
Moving Game Entities
Furthermore, we can animate our game entities to make the interaction more engaging:
keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: rec1.move_ip(-2, 0) if keys[pygame.K_RIGHT]: rec1.move_ip(2, 0) if keys[pygame.K_UP]: rec1.move_ip(0, -2) if keys[pygame.K_DOWN]: rec1.move_ip(0, 2)
In this snippet, we’re moving rec1 based on the arrow keys pressed. The “move_ip” method moves the Rectangle’s position by the amount you pass in. Remember to include this inside a main game loop to keep the window running and responsive to user’s input.
That’s it for part two, in the next part, we’ll focus on the actual collision detection.
Pygame Collision Detection
With our Pygame environment set and our entities in motion, let’s dive into the actual collision detection. Pygame makes it incredibly easy to check for collisions between its Rect objects with the “colliderect” method. Here’s how it’s done:
if rec1.colliderect(rec2): print('Collision detected!')
This code will print “Collision detected!” each frame that the two rectangles are in contact. However, printing to the console dozens of times a second isn’t quite what we want. Usually, we would perform some action like bouncing the rectangles away from each other.
Bouncing the Rectangles
To bounce the rectangles, we’ll need to decide what happens based upon their direction. Let’s assume that rec1 is moving from left to right. When it hits rec2, we’ll want to start moving it in the opposite direction. To do so:
dx, dy = 2, 0 if rec1.colliderect(rec2): dx *= -1 rec1.move_ip(dx, dy)
In this snippet, we’ve defined a direction vector `(dx, dy)`. Whenever a collision is detected, we invert the `dx` direction before moving `rec1`, causing it to bounce back.
Dealing with Multiple Possible Collisions
In a more advanced scenario, your game might have many collidable objects (like an array of blocks). In this case, Pygame offers another method called “collidelist”, which checks if your Rectangle collides with any rectangles inside a list:
blocks = [pygame.Rect(50 * i, 50 * i, 50, 50) for i in range(5)] collision_index = rec1.collidelist(blocks) if collision_index > -1: print(f'Collision with block {collision_index} detected!')
This will check if `rec1` collides with any of the blocks in the `blocks` list. If a collision is detected, it will print a message and tell us the index of the block with which `rec1` collided.
Creating interactive and engaging games with Python has never been so easy! With this newfound knowledge of Pygame collision detection, you can create realistic games and have your objects interact with each other. The sky is the limit! We hope you enjoyed this lesson and look forward to seeing you in the next one!
Handling Collisions with an Array of Blocks
As your game expands, you might find that your player doesn’t interact with a single object. Instead, they interact with multiple objects like an array of blocks. Pygame offers excellent ways to handle such scenarios using the “collidelistall” method.
blocks = [pygame.Rect(100 * i, 100, 50, 50) for i in range(5)] collisions = rec1.collidelistall(blocks) if collisions: print(f'Collisions Detected at {collisions}!')
The method “collidelistall” checks collisions with all the rectangles in the list, unlike “collidelist”. If rec1 colides with any rectangle in the blocks list, it will return a tuple of the indices where the collisions have happened.
Creating a Boundary for Collision
Sometimes, we don’t want to check collision across the entire surface of a rectangle. We can modify the collision boundary of a rectangle using “inflate”. Inflate works by providing positive values to increase the width and height of the rectangle or negative values to decrease it.
rec1.inflate_ip(-10, -10) # Creates a smaller collision boundary if rec1.colliderect(rec2): print('Collision detected!')
In this snippet, the rectangle rec1 will inflate inwards by 10 pixels on each side, thus creating a smaller collision rectangle inside the original.
Mandatory Collision with Borders
In games, we often want to keep our moving objects within the screen or bounding area. Let’s create a border collision:
if not win.get_rect().contains(rec1): print("Out of bounds!")
In this example, we are checking if the whole of rec1 is inside the window (win). If it isn’t, we are printing a message “Out of bounds!”.
Detecting Collisions between Groups
When we have a large number of moving or static objects, it may be more efficient to use Pygame sprite Groups.
group1 = pygame.sprite.Group() group2 = pygame.sprite.Group() ... if pygame.sprite.groupcollide(group1, group2, False, False): print('Collision detected between groups!')
The groupcollide function checks for collisions between all sprites in two groups and returns a dictionary. Each key-value pair in the dictionary contains a sprite from the first group and sprites from the second group it intersects with.
Now you see how Pygame collision detection isn’t difficult or complex. Through combining these principles, you can create compelling games full of action, interaction, and movement. Happy Gaming and Coding!We hope this tutorial sparked your interest in game development and Python programming. As you continue your journey, there are limitless concepts to explore and skills to master.
Zenva offers a wealth of resources designed to guide you through your learning journey, from beginner to professional. One such resource is our Python Programming Mini-Degree, a comprehensive collection of Python programming courses. It covers everything from coding basics to algorithms, object-oriented programming, game development, and app development and is designed to reinforce learning with challenges, quizzes, and live coding lessons.
With Zenva Academy, not only do you learn coding and game development, but you also gain the competence to publish your own games, land jobs and start businesses. Our platform hosts over 250 supported courses, ensuring that learners at all stages have resources to keep growing. Plus, our flexible courses are accessible 24/7 on any device, empowering you to learn at a pace and time that suits you.
Beyond our Python mini-degree, we’ve got a broad collection of Python courses, catering to various aspects of the versatile Python language. Explore these options today and let Zenva be your lifelong learning partner in this exciting tech world.
Conclusion
In summary, collision detection is at the heart of crafting engaging and realistic games. Understanding this in the context of Pygame propels you further along your game development journey. You’ve seen how a few lines of code can dramatically alter the game mechanics, making your games more interactive and fun.
Remember, the learning doesn’t stop here. With endless possibilities and complex games to create, continued learning and practice are key. Our Python Programming Mini-Degree offers a deeper dive into Python, expanding upon what you’ve started here today. We can’t wait to see what creative, interactive games you bring to life!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
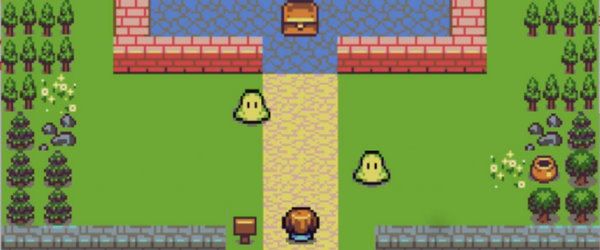
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.