Welcome to our hands-on beginner-friendly tutorial on Lists in C#! Learning coding concepts and advanced features of high-level programming languages like C# can enhance your game development skills beyond measure. With our guidance, you’ll dive into the world of Lists in C#, understand their importance, and learn how to efficiently implement them in your code.
Table of contents
What is a C# List?
In C#, Lists are a type of collection, similar to arrays but with the significant advantage that they can dynamically resize. They provide methods for adding, removing, and finding items with functionality akin to that of an array but with greater flexibility.
Why Should You Learn About Lists in C#?
If you’re building games with Unity, learning how to use Lists is quintessential. With Lists, you can dynamically store and manipulate a set of items, such as game characters, inventory items, or leaderboard scores. This helps in creating interactive, complex, and exciting gaming experiences.
Remember, mastering Lists and other advanced C# features can significantly boost your prospects in the game development industry. It’s always worth upgrading your skillset, especially when the learning resources are designed to make the journey enjoyable and engaging.
Now that we’ve addressed the “what” and “why”, let’s hop right into the coding aspect. Stay with us as we dive into fascinating C# codes and decipher them bit by bit. As we journey along, you’ll find yourself becoming comfortable with C# Lists and related concepts in no time.
Creating Lists in C#
In C#, lists are created by using the List
class that the System.Collections.Generic
namespace provides. Let’s create a simple list of integers:
using System.Collections.Generic; List<int> numbers = new List<int>();
The above code instantiates a list of integers that currently holds no elements.
Adding to a List
To add to a list, you can use the Add()
method. Here’s an example:
numbers.Add(1); // numbers list now holds {1} numbers.Add(2); // numbers list now holds {1, 2} numbers.Add(3); // numbers list now holds {1, 2, 3}
In the above code, you can see that after each Add()
operation, we’re appending a new integer to our list.
Accessing Elements in a List
List elements can be accessed in much the same way as an array, using an index number. Take a look at this example:
int firstNumber = numbers[0]; // firstNumber is now '1' int secondNumber = numbers[1]; // secondNumber is now '2'
A point to note here is that List indices also start at zero, similar to arrays.
Removing Elements from a List
To remove an element from a list, you can use the Remove()
method. For instance:
numbers.Remove(1); // numbers list now holds {2, 3}
The Remove()
operation in the above example removes the first occurrence of ‘1’ from our list.
Alternatively, you can use the RemoveAt(int index)
method to remove the element at a specific index:
numbers.RemoveAt(0); // numbers list now holds {3}
This operation removes the element at index 0.
Counting List Elements
To count the number of elements currently in a list, we use the Count
property. Here’s a simple example:
numbers.Add(4); numbers.Add(5); int count = numbers.Count; // count is 5
In the above case, the Count
property returns the number of elements held in our list, which is 5.
Checking for List Elements
The Contains()
method allows us to check for an element within a list.
bool containsThree = numbers.Contains(3); // containsThree is true bool containsSix = numbers.Contains(6); // containsSix is false
In this case, Contains() returns true if the specified value is in the list, and false otherwise.
Iterating Through a List
To iterate through all the elements of a list, we have some familiar options: the for
loop and foreach
loop.
for (int i = 0; i < numbers.Count; i++) { Console.WriteLine(numbers[i]); }
The foreach
loop offers an easier way to iterate through elements.
foreach (int number in numbers) { Console.WriteLine(number); }
Both of these examples print all the elements of our list to the console.
Clearing a List
The Clear()
method wipes a list clean of all its elements, essentially resetting it.
numbers.Clear(); int countAfterClear = numbers.Count; // countAfterClear is 0
This simply removes all the elements from our list, leaving us with an empty list.
We hope these examples have given you a solid understanding of Lists in C# and their practical implementation. Keep coding, practicing, and challenging yourselves, and you’ll witness incredible growth in your game development journey!
Finding Elements in a List
There will be situations when you need to find the index of an element in a list. The IndexOf()
method serves this purpose:
int indexThree = numbers.IndexOf(3); // indexThree is 2 int indexSix = numbers.IndexOf(6); // indexSix is -1
The IndexOf()
method returns the index of the first occurrence of the specified value in the list. If the value does not exist, it returns -1.
Sorting Lists
Lists provide a handy Sort()
method that sorts all elements in the list. Let’s see how it works:
numbers.Add(5); numbers.Add(3); numbers.Add(1); numbers.Add(4); numbers.Add(2); numbers.Sort(); // numbers list now holds {1, 2, 3, 4, 5}
After sorting, the list now holds the values in ascending order.
We can also sort the list in descending order using the Sort
method and a comparison delegate:
numbers.Sort((a, b) => b.CompareTo(a)); // numbers list now holds {5, 4, 3, 2, 1}
In this case, we’ve sorted the list in descending order.
Converting Array to List and Vice Versa
To convert an array to a list, we can use the List
constructor as shown:
int[] arr = new int[] {1, 2, 3, 4, 5}; List<int> numList = new List<int>(arr); // numList now holds {1, 2, 3, 4, 5}
We can also convert a list back to an array with the ToArray()
method:
int[] numArray = numList.ToArray(); // numArray now holds {1, 2, 3, 4, 5}
Working with List of Objects
The real power of lists can be witnessed when working with objects. Let’s imagine we have a simple Player
class:
public class Player { public string Name {get; set;} public int Score {get; set;} public Player(string name, int score) { Name = name; Score = score; } }
We can create a list of players and add new Player objects to it.
List<Player> players = new List<Player>(); players.Add(new Player("Alice", 1000)); players.Add(new Player("Bob", 800)); players.Add(new Player("Charlie", 1200));
In this way, you can create and manipulate collections of any type of object. Understanding and effectively using lists can unlock a great deal of power and flexibility in your game development schemes!
Where to Go Next?
We at Zenva aim to provide the stepping stones to make your progression in learning smooth and enjoyable. After getting a solid understanding of C# Lists, your next step should be diving further into game development by mastering a powerful game engine like Unity.
For that, our Unity Game Development Mini-Degree would be an excellent place to march forward. This comprehensive collection of courses covers building cross-platform games in 2D, 3D, AR, and VR, with Unity – a popular game engine used globally. The mini-degree introduces various game development skills, storytelling techniques, intermediate coding skills, and optimization strategies. All courses are project-based, equipping you with a robust portfolio of Unity games and projects by the end.
Lastly, for a more expansive selection of learning resources, do check out our Unity Collection. Happy coding and game developing! Remember, with us, beginner or otherwise, you’re always in the right hands.
Conclusion
As you’ve discovered in this tutorial, Lists in C# are essential, flexible, and powerful tools. They help manage dynamic data collections and are integral to complex game development tasks. Mastering Lists and other advanced C# features can substantially enhance your programming skills, making you a formidable force in the gaming industry.
At Zenva, we believe in nurturing your talent and providing you with the best learning materials. We invite you to accompany us on this journey and explore our comprehensive Unity Game Development Mini-Degree. Whether you’re an aspiring game developer or a seasoned coder looking to ignite your passion for game design, the Unity Mini-Degree offers a challenging and rewarding ride. Your path to masterful game development starts here!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
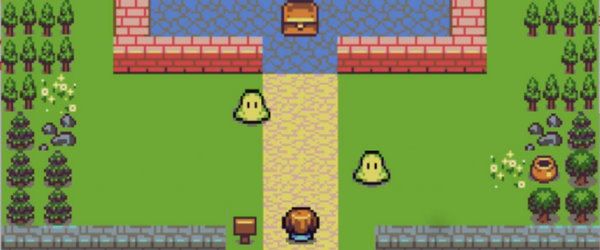
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.