Unleashing the power of software testing can level up your development skills like never before and today we delve into one such advantageous tool – xUnit.net, or simply xUnit, when used in C# programming. This beautiful combination can streamline the way you test your C# projects, assuring high-quality and bug-free apps or games. So, why not hop on this exciting journey and gain innovation by adding a solid layer of testing to your programming efforts?
Table of contents
What is xUnit for C#?
Known for being a free, open-source unit testing tool, xUnit.net is designed for .NET Framework. This toolkit comes to aid when developers need to write tests for their C# code. It is part of the versatile family of xUnit frameworks that bring a fresh approach to writing automated tests.
A Tool for The Task: Why xUnit?
The name ‘xUnit’ signifies its lineage, which traces back to a broader category of frameworks that started with the renowned tool called JUnit. But why should you include xUnit in your C# projects? Here lie your answers:
- Firstly, xUnit is designed by the inventor of NUnit, another popular testing tool for .NET, ensuring its credibility and resourcefulness.
- It leverages a more modern and flexible approach compared to its counterparts, encouraging developers to write robust and usable tests.
- Adding to its components, xUnit supports .NET’s async programming model, which can be a real asset for developers in today’s evolving programming environment.
- Finally, its clean syntax makes writing and reading tests easier, making our coding journey more straightforward and enjoyable.
Now that you’re familiar with what xUnit for C# is and why it’s a crucial tool to learn, let’s not wait anymore and start writing some code!
Setting up xUnit in Your Project
Start by installing the xUnit testing framework in your C# project. Installing xUnit can be easily done through the NuGet Package Manager available in Visual Studio. Specify ‘xunit’ in the search bar and click on ‘Install’ to add it to your project.
// Go to Tools > NuGet Package Manager > Package Manager Console // Type the following command in the console: PM> Install-Package xunit
The next step is to install a test runner that visually displays our testing results. The ‘xunit.runner.visualstudio’ extension is ideal for this.
// In the Package Manager Console, type the following command: PM> Install-Package xunit.runner.visualstudio
Writing Your First xUnit Test
Now that we’re all set, let’s proceed towards writing our first unit test using xUnit.
// Your test method [Fact] public void MyFirstXunitTest() { // Arrange: Set input and the expected result int input = 10; int expectedResult = 20; // Act: Call the actual method int actualResult = DoubleTheNumber(input); // Assert: Check if the expected result matches the actual result Assert.Equal(expectedResult, actualResult); }
In this example, we marked our test method with the ‘Fact’ attribute, a distinctive feature of xUnit. The test takes an integer, doubles it, and then asserts that the result is as expected.
More Attributes: Using Theory
xUnit offers another crucial attribute – ‘Theory’, allowing us to run a single test with different input values. A single ‘Theory’ test can run multiple times with various data points, which is a big win!
[Theory] [InlineData(10, 20)] [InlineData(20, 40)] public void MyTheoryTest(int input, int expectedResult) { // Act int actualResult = DoubleTheNumber(input); // Assert Assert.Equal(expectedResult, actualResult); }
Dealing with Expecting Exceptions
At times, we want our code to throw exceptions as part of its normal functioning. In such cases, we use xUnit’s ‘Assert.Throws’ method to verify these expected exceptions. Let’s take a look:
[Fact] public void ExternalResourceUnavailableExceptionTest() { // Arrange string invalidResourceUrl = "http://invalid.url"; // Assert Assert.Throws<ExternalResourceUnavailableException>(() => AccessResource(invalidResourceUrl)); }
This test ensures that our method ‘AccessResource’ throws an ‘ExternalResourceUnavailableException’ when called with an invalid URL.
Organization with Collections
Organization is key! xUnit provides a means of organizing and executing test cases together through the concept of collections.
[Collection("Database collection")] public class DatabaseTest1 { public DatabaseTest1() { // Constructor code here } [Fact] public void TestMethod1() { // Test method code here } } [Collection("Database collection")] public class DatabaseTest2 { public DatabaseTest2() { // Constructor code here } [Fact] public void TestMethod2() { // Test method code here } }
In the above code, we have two test classes that belong to the same collection. Therefore, they will be executed together.
Class and Collection Fixtures
Context sharing can be managed generously in xUnit through Class and Collection Fixtures. The class fixture supplies a constructor (for setup) and an optional Dispose method (for teardown).
public class DatabaseFixture : IDisposable { public DatabaseFixture() { // Setup code here } public void Dispose() { // Cleanup (teardown) code here } } public class DatabaseTests : IClassFixture<DatabaseFixture> { DatabaseFixture fixture; public DatabaseTests(DatabaseFixture fixture) { this.fixture = fixture; } [Fact] public void Test1() { // Test method code here } }
Here, the DatabaseFixture Class creates a context at the beginning of each test method. The system calls Dispose after all test methods have run, providing a perfect pathway to clean up resources after testing.
Working with Mocks
For true unit tests, it’s sometimes necessary to isolate the system under test by replacing other parts of the software system with ‘mocked’ versions. Here is how we can implement mocks in xUnit using the popular Moq framework:
public interface IRepository { bool Save(string data); } public class TestClass { IRepository repo; public TestClass(IRepository repo) { this.repo = repo; } public bool SaveData(string data) { return repo.Save(data); } } [Fact] public void TestMethodWithMock() { var mock = new Mock<IRepository>(); mock.Setup(repo => repo.Save(It.IsAny<string>())).Returns(true); TestClass cls = new TestClass(mock.Object); Assert.True(cls.SaveData("sample data")); }
The Power of Assertions
Thanks to a robust assertions library, xUnit blesses us with an extensive range of assertion methods to verify test results efficiently.
[Fact] public void AssertionTest() { int expected = 5; int actual = Add(2, 3); // Assert with various methods Assert.Equal(expected, actual); Assert.True(actual > 1); Assert.False(actual < 1); Assert.NotNull(actual); Assert.InRange(actual, 1, 6); }
This test showcases the flexibility and power of xUnit’s assertion options that can amplify our unit testing to great extent.
Testing Equality and Collection Equality
Through Equality Assertions, xUnit allows us to verify if the output of our test is equal to the expected value. Similarly, through Collection Equality Assertions, we can compare two collections for equality.
[Fact] public void EqualityAssertionTest() { // Arrange string expected = "Zenva"; // Act string actual = GetCompanyName(); // Assert Assert.Equal(expected, actual); } [Fact] public void CollectionEqualityAssertionTest() { // Arrange List<int> expectedList = new List<int>{1, 2, 3, 4, 5}; // Act List<int> actualList = GetNumberList(); // Assert Assert.Equal(expectedList, actualList); }
As seen in the sample, these Equality Assertions can powerfully uplift our test coverage and integrity.
Custom Assertions
xUnit assertions aren’t sufficient for your unique scenarios? Don’t worry, xUnit provides a solution for that too! Just create your own assertion methods.
public static class CustomAsserts { public static void AreEqualIgnoringCase(string expected, string actual) { if(!expected.Equals(actual, StringComparison.OrdinalIgnoreCase)) { throw new Xunit.Sdk.XunitException("Strings not equal ignoring case"); } } } [Fact] public void CustomAssertsTest() { // Arrange string expected = "Zenva"; // Act string actual = GetCompanyName(); // Assert using custom asserts CustomAsserts.AreEqualIgnoringCase(expected, actual); }
This example introduces the concept of Custom Assertions, opening up a realm of flexible testing opportunities.
Code Coverage with Coverlet
To ensure that our codebase is well tested, it’s important to measure what proportion of our code executes during our test runs. For .NET, the industry standard solution for this is Coverlet.
// In the Package Manager Console, type the following command: PM> Install-Package coverlet.msbuild
With this installed, we can run our test coverage with a simple command:
// This produces a result.json file with detailed coverage numbers dotnet test /p:CollectCoverage=true
Conclusion
As we’ve explored, testing is a critical component of any software development lifecycle, and with tools like xUnit for C#, developing bug-free and maintainable applications becomes a breeze. Apart from simply learning C#, knowing how to validate your code with testing frameworks such as xUnit drastically improves your ability to deliver high-quality software.
At Zenva, we firmly believe in evolving with the tech industry’s ever-growing demands. And this doesn’t end here! There is a lot more to dig into with xUnit’s advanced functionalities. So join us, and let’s learn to code the smarter way.
Where To Go Next on Your Learning Journey?
Just learned xUnit for C# and excited for what’s ahead? Your development adventure is always continuing, and we suggest taking the next big step with Zenva’s Unity Game Development Mini-Degree. This robust program offers a comprehensive collection of game development courses using Unity, a versatile game engine used across multiple industries. The curriculum aims to bridge your journey from the fundamentals through to advanced topics, ensuring you gain access to high-paying job opportunities.
With practical projects, you’ll build real games, thereby strengthening your portfolio. The courses are regularly updated according to the latest industry practices and upon completion, you’ll earn valued certificates. This mini-degree is suitable for both beginners and experienced programmers. Thus, brace yourself for practical, relevant and flexible learning!
Looking for an even wider approach to Unity? Then, our broad selection of Unity courses can certainly be of interest! At Zenva, we provide beginner to professional courses in programming, game development, and AI. Whether you’re starting from scratch or aiming to upskill, we’ve got you covered. Here’s to your ongoing journey towards coding expertise!
Conclusion
Utilizing strong testing frameworks like xUnit for C# empowers us to step up our programming game exponentially, validating our code’s integrity at each development phase. Remember, the holy grail of software development isn’t just to code, but to code flawlessly!
Mastering xUnit is indeed an invincible asset in any developer’s toolbox. However, the voyage of learning doesn’t stop here. At Zenva, we are dedicated to fueling your journey further, with our comprehensive Unity Game Development Mini-Degree, which can propel your coding and game development skills to new exciting heights. So, why wait? Join us today, to code, create, and innovate!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
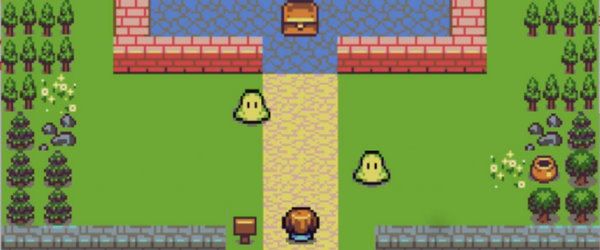
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.