Welcome to this engaging tutorial on using the List.Find() method in C#. As you embark on your coding journey, almost inevitably you’ll encounter a situation where you want to sift through a list or collection of items to find a particular instance. That is where the List.Find() method steps in. In this tutorial, we will demystify the List.Find() method, making it a valuable and useful addition to your coding arsenal!
Table of contents
What is List.Find()?
The List.Find() method in C# is a powerful tool packed in a simple looking syntax. Think of it like a detective with the sole task of searching through a list to find an item based on a certain condition or criteria you specify.
What is it for?
If you have a list of game player scores or city names in a strategy game and you quickly want to find a particular score or city name on that list, List.Find() will be your go-to method.
Why should I learn it?
This method helps in trimming down lines of code and makes your code more readable and efficient. Therefore, mastering the handling of this method can enhance the speed of your applications and take your coding skills to greater heights!
Basic Use of Find()
Let’s start with the very basics. Here’s how you can use the List.Find() method.
List<int> numbers = new List<int> { 1, 3, 5, 7, 9 }; int num = numbers.Find(x => x > 5); Console.WriteLine(num); // Outputs: 7
In this very basic example, we use a lambda expression x => x > 5
as a criterion to find a number in the list that is greater than 5. The predicate x > 5 is true when tested with 7, hence 7 is returned.
Finding the first match
You may have noticed that List.Find() returns the first match in the list. Let’s illustrate this.
List<int> numbers = new List<int> { 2, 4, 6, 4, 8 }; int num = numbers.Find(x => x == 4); Console.WriteLine(num); // Outputs: 4
Although we have two 4s on our list, only the first 4 is returned because List.Find() stops at the first match.
Working with complex types
It’s also possible to work with more complex types in list. Consider this example.
class Player { public string Name { get; set; } public int Score { get; set; } } List<Player> players = new List<Player>() { new Player(){ Name="John", Score=80}, new Player(){ Name="Steve", Score=90} }; Player player = players.Find(p => p.Name == "Steve"); Console.WriteLine(player.Score); // Outputs: 90
We have a list of Player instances, and we want to find the Player that has the name Steve. Using the List.Find() method, we pass along the criteria as p => p.Name == "Steve"
to get our result.
Handling no match scenario
What happens when List.Find() doesn’t find a match in the list? Let’s see.
List<int> numbers = new List<int> { 3, 6, 9 }; int num = numbers.Find(x => x == 5); Console.WriteLine(num); // Outputs: 0
When no match is found, List.Find() returns the default value for the type of the list elements. In this case, it’s 0, because the default value for integer types in C# is 0.
If we were working with a list of classes or structs, a failure to find a match would return null. This is because null is the default value for object types.
Combining conditions
You can combine conditions using AND (&&
) or OR (||
) operators in the condition.
List<int> numbers = new List<int> { 1, 3, 5, 7, 9 }; int num = numbers.Find(x => x > 1 && x < 5); Console.WriteLine(num); // Outputs: 3
In this example, only 3 satisfies the condition of being greater than 1 and less than 5.
Finding last match
While List.Find() returns the first match in the list, if you want to get the last match, you can use the List.FindLast() method.
List<int> numbers = new List<int> { 2, 4, 6, 4, 8 }; int num = numbers.FindLast(x => x == 4); Console.WriteLine(num); // Outputs: 4
Whenever there’s more than one match for the condition, List.FindLast() returns the last occurrence, as shown in the example.
Find index of a match
If you would like to know the index (position) of the found element, you can use List.FindIndex(), which will return the 0-based index of the found element.
List<int> numbers = new List<int> { 1, 3, 5, 7, 9 }; int index = numbers.FindIndex(x => x == 5); Console.WriteLine(index); // Outputs: 2
In this example, since 5 is found at the second index (0-based) of the list, the FindIndex() function returns 2.
Finding all matches
If you want to find all items that satisfy the condition, use List.FindAll(). This method returns a list of all matching items.
List<int> numbers = new List<int> { 1, 3, 5, 7, 9 }; List<int> evenNumbers = numbers.FindAll(x => x % 2 == 0); // evenNumbers now contains: 2, 4
In this example, we were looking for all even numbers in our list. The List.FindAll() method returned another list containing only the even numbers from our original list.
Diving Deeper into Complex Types
Let’s push the envelope a bit more with our Player class.
List<Player> players = new List<Player>() { new Player(){ Name="John", Score=80}, new Player(){ Name="Steve", Score=90}, new Player(){ Name="Tom", Score=85} }; List<Player> topPlayers = players.FindAll(p => p.Score >= 85); // topPlayers now contains: Steve, Tom
In the example above, we used List.FindAll() to find all players who have scored 85 or above. The method returned a new list containing only Player instances that met our criteria.
List.Exists()
Before we conclude, let’s talk about List.Exists(). This function will return true if there’s at least one item in the list that satisfies our condition.
List<int> numbers = new List<int> { 1, 3, 5, 7, 9 }; bool exists = numbers.Exists(x => x > 5); Console.WriteLine(exists); // Outputs: True
This function is particularly useful when you don’t care about the exact count or the elements themselves, and you just want to know if there’s at least one match.
Null Lists
Let’s see what happens when you try to use List methods on null List.
List<int> numbers = null; bool exists = numbers.Exists(x => x > 5); //System.NullReferenceException: 'Object reference not set to an instance of an object.'
That’s an error! Keep in mind that calling a method such as
Exists(), Find(), FindAll(), etc. on a null List will throw a NullReferenceException. Be sure to initialize your lists before you start searching.
Conclusion
As we’ve seen in these examples, the List.Find(), List.FindAll(), List.Exists() methods provide extensive power and flexibility to manage and manipulate list data within the C# language. As developers, we can leverage these methods to write concise, easy-to-read code, enabling us to build more efficient, high-performing applications. Time to explore these methods and find out the magic they bring to your coding skills!
Continue Your Learning Journey with Zenva!
Now that you’ve dipped your toes in the expansive ocean of coding, it’s time to dive deeper. At Zenva, we provide a range of beginner to professional courses in programming, game development, and AI. With over 250 courses available, our content aims to boost your career, whether you’re just starting your journey or looking to advance further.
Why not go a step further and explore the power of one of the world’s most popular gaming engines? You can dive straight into our comprehensive Unity Game Development Mini-Degree. It covers a wide array of topics such as game mechanics, cinematic cutscenes, audio effects and much more, opening doors to ample high-paying job opportunities in industries such as films and animation, automotive, healthcare, and engineering.
If you’re looking for an extensive collection of Unity topics, check out our Unity courses. With Zenva, you are not only learning, but also building a professional portfolio as you go. Remember, there’s no end to learning, so let’s get programming!
Conclusion
Congratulations on mastering the List.Find() method in C#! Your coding knowledge has just leveled up, and these methods are building blocks in becoming an adept developer. Imagine the amazing things you could do with even more advanced technical skills.
At Zenva, we believe in your curiosity and untapped potential. That’s why we offer over 250 comprehensive and accessible courses to help you along your learning path. Why not explore new realms with our Unity Game Development Mini-Degree? Success is awaiting, and it all begins with the next step. See you in the course!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
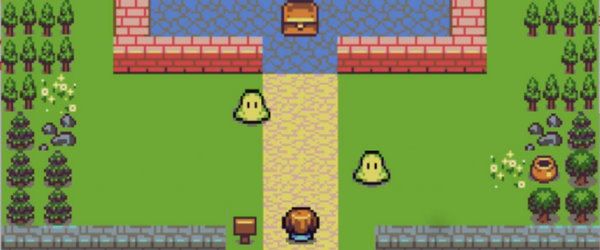
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.