Welcome to an exciting journey into the world of Eigen C++, a high-level C++ library for linear algebra! This tutorial is your chance to get hands-on with Eigen, understand how it works, and discover the power it lends to your programming toolkit.
Table of contents
What is Eigen?
Eigen is a powerful C++ library designed to simplify the complexities of linear algebra computations for programmers. It introduces the precision and speed of linear algebra computations to a broad range of possibilities for programming tasks, artificial intelligence, and game development offer.
Why should you learn Eigen C++?
Matrix manipulations, vector arithmetic, numerical solvers, and related algorithms are pervasive in computational tasks. Learning Eigen C++ empowers you to perform these complex calculations with relative ease, enhancing the versatility of your code especially in game physics and AI.
What can you do with Eigen?
From solving systems of linear equations to implementing transformations in 3D games, Eigen packs a punch when it comes to handling mathematical heavy-lifting. This positions it as a versatile tool not just for game developers but also for those delving into the realm of machine learning and AI. Now that you have a basic understanding of what Eigen C++ is and why it’s worth learning, let’s dive into some hands-on coding!
Setting up Eigen
First, we’ll need to download and install the Eigen library. Once we have the library installed, we can include Eigen in our C++ programs like any other header file. Assuming you have the Eigen library installed, here’s how you can include it:
#include <Eigen/Dense>
Working with Matrices
In Eigen, a matrix is a rectangular array of numbers. Here’s an example of how you can declare a matrix:
Eigen::Matrix3f m3;
This code creates a 3×3 matrix of floating-point numbers. We can also initialize a matrix at declaration like this:
Eigen::Matrix3f m3; m3 << 1, 2, 3, 4, 5, 6, 7, 8, 9;
The above code initializes 3×3 matrix with values ranging from 1 to 9.
Performing Matrix Operations
With Eigen, you can do all standard matrix operations. Let’s take a look at matrix addition:
Eigen::Matrix2f a, b; a << 1, 2, 3, 4; b << 5, 6, 7, 8; std::cout << "a + b =\n" << a + b << std::endl;
This code creates two 2×2 matrices, adds them together, and prints the result, which is another 2×2 matrix.
Working with Vectors
In Eigen, vectors are just special case of matrices. Let’s declare a 3-dimensional vector:
Eigen::Vector3f v;
The above code declares a 3-dimensional vector of floating-point numbers. You can also initialize a vector at declaration, similar to matrices:
Eigen::Vector3f v(1,2,3);
This will declare and initialize a vector with the given values.
Performing Vector Operations
Just like with matrices, you can also perform standard vector operations using Eigen. Here’s an example of vector addition:
Eigen::Vector3f v1(1,2,3); Eigen::Vector3f v2(4,5,6); std::cout << "v1 + v2 =\n" << v1 + v2 << std::endl;
This creates two 3D vectors, adds them together, and prints the result, which is another 3D vector.
How about scalar multiplication? Here’s an example:
Eigen::Vector3f v(1,2,3); std::cout << "v * 3 =\n" << v * 3 << std::endl;
This creates a 3D vector, multiplies it by a scalar (3 in this case), and prints the result.
Eigenvalues and Eigenvectors
Eigen can also compute eigenvalues and eigenvectors. These are important concepts in many areas, including machine learning and computer graphics. Let’s declare a 3×3 matrix and compute its eigenvalues:
Eigen::Matrix3f m; m << 1, 2, 1, 2, 1, 0, -1, 1, 2; Eigen::EigenSolver solver(m); std::cout << "The eigenvalues of m are:\n" << solver.eigenvalues() << std::endl;
This will print the eigenvalues of the declared matrix. Typically this process is used in advanced computations.
Conclusions
As we have explored, Eigen is a powerful tool for performing complex linear algebra operations in C++. If you are working in machine learning, computer graphics, game development, or any other field that involves heavy number crunching, learning Eigen can be a great asset.
Zenva strongly believes in the power of continuous learning and providing high-quality content. Therefore, we encourage you to delve deeper into Eigen, practice with the library, and discover the numerous possibilities it can offer you in your coding journey.
Matrix and Vector Multiplication
Perhaps one of the most common operations in linear algebra is the multiplication of matrices and vectors. Let’s take a look at how Eigen allows us to do this with simplicity and ease:
Eigen::Matrix2f a; Eigen::Vector2f v; a << 1, 2, 3, 4; v << 5, 6; std::cout << "a * v =\n" << a * v << std::endl;
This code creates a 2×2 matrix and a 2D vector, multiplies them together, and prints the result. The result, in this case, would be a 2D vector.
Solving Linear Equations
Solving systems of linear equations is a vital task in numerous fields, including computer graphics, physics simulations, and machine learning. Eigen provides a range of methods to do just that:
Eigen::Matrix2f A; Eigen::Vector2f b; A << 2, -1, -1, 2; b << 1, 2; Eigen::Vector2f x = A.colPivHouseholderQr().solve(b); std::cout << "The solution is:\n" << x << std::endl;
This example defines a system of two linear equations, solves it, and prints the result.
Matrix and Vector Transposition
A fundamental operation in linear algebra is the transposition of matrices and vectors. In Eigen, this task is realized briskly:
Eigen::Matrix2f m; m << 1, 2, 3, 4; std::cout << "The transpose of m is:\n" << m.transpose() << std::endl;
This example prints the transpose of the declared 2×2 matrix.
Eigen::Vector2f v(1,2); std::cout << "The transpose of v is:\n" << v.transpose() << std::endl;
This example transposes 2D vectors.
Matrix Determinant and Inverse
Determinants and inverses are important matrix operations used widely in simulations, image processing, and AI. Here’s how Eigen can compute these:
Eigen::Matrix2f m; m << 1, 2, 3, 4; std::cout << "The determinant of m is:\n" << m.determinant() << std::endl; std::cout << "The inverse of m is:\n" << m.inverse() << std::endl;
This code calculates and prints both the determinant and inverse of a 2×2 matrix.
Where to Go Next with C++?
Are you excited about furthering your journey into C++ and learning more advanced concepts? Nothing should stop you!
We at Zenva, provide a collection of crucial and exciting courses that can help you build an impressive portfolio in the tech-world. One such collection is our C++ Programming Academy. Through this program, we aim to simplify the learning process by building games, including text-based RPGs and clicker games. Our curriculum covers C++ basics, program flow, object-oriented programming, and even using the SFML for graphics and audio.
For an even wider selection, check out our catalogue of C++ Courses. With over 250 project-based courses, Zenva offers a comprehensive learning path from beginner to professional practice. So, continue with your journey into the exciting world of programming, and discover the endless opportunities that lie ahead. Happy learning!
Conclusion
That wraps up our exciting exploration of Eigen C++, a high-level C++ library for linear algebra. Whether you’re working on game physics or developing machine learning algorithms, Eigen offers a toolkit that enriches your programming abilities by facilitating intricate matrix and vector manipulations. Remember, any machine learning or game development task can be made easier with the right tools – and Eigen C++ is undoubtedly one of them!
Ready for further growth? Remember we’re here to guide you. Developing your skills with Zenva’s C++ Programming Academy will put you on a fast track to mastering C++ and unlocking your full potential. Get started on your journey today, and let Eigen C++ push your coding capabilities to new heights!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
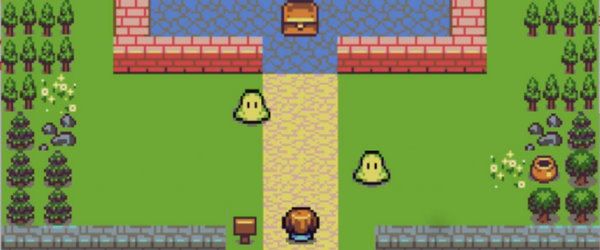
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.