Welcome to this comprehensive tutorial on the C# programming language! If you’ve ever wondered about diving into game development or exploring the world of coding, this tutorial is the perfect steppingstone. What’s more? We’ve ensured that the tutorial remains engaging and straightforward, making it suitable for beginners and experienced coders alike.
Table of contents
What is C#?
C# (pronounced “C-Sharp”) is a powerful, object-oriented programming language developed by Microsoft. It’s chiefly designed for building a variety of applications that run on the .NET Framework, and it’s especially potent in building games, thanks to its integration with the Unity game engine.
The Purpose of C#
With C#, you can build an array of applications, including Windows clients, consoles, Web services, mobile apps, and even games. However, it’s its prowess for game development, particularly for Unity, that draws in a mass following.
Why Learn C#?
As a beginner, you might ask, why should I learn C#? The straightforward answer is its utility in game development. But there’s more :
- Unity Game Mechanism: Unity, one of the most in-demand game engines, utilizes C# scripts for game mechanics and behaviors, making C# an invaluable asset for aspiring game developers.
- Demand in Job Markets: The demand for C# developers remains relatively high in the job market, as hundreds of companies utilize C# for creating their products.
- Scalability: As your coding skills grow, C# is versatile enough to grow with you, allowing you to program complex and scalable applications.
Thus, whether you are starting your coding journey from scratch or wish to extend your skill set, learning C# provides numerous exciting opportunities and avenues for exploration and growth.
Getting Started with C#
Let’s kick off our programming journey in C# by exploring its basic constructs. Below are simple C# examples which address the fundamental concepts such as variable declarations, conditional statements, loops, and functions that form the backbone of the language.
1. Hello World in C#
Like with other programming languages, we’ll begin by displaying “Hello, World!” to the console.
using System; public class Program { public static void Main() { Console.WriteLine("Hello, World!"); } }
This code will output Hello, World! in the console when executed.
2. Variables and Data Types
In C#, we must specify the type of data we intend to store in any variable. This concept is known as statically typed. Here’s an example:
int myScore = 10; string myName = "Zenva"; double myAverageScore = 89.6; bool isAcademy = true;
Here, we have declared and initialized an integer (int), a string, a double, and a boolean (bool) variable.
3. Conditional Statements: If-Else
The ‘if-else’ statement is a fundamental decision-making construct in C#. It allows the program to evaluate whether a condition is true or false and perform operations accordingly.
int score = 20; if (score < 50) { Console.WriteLine("You failed!"); } else { Console.WriteLine("You passed!"); }
This code checks if the score is less than 50. If the condition is true, it prints “You failed!” otherwise it prints “You passed!”
4. Loops: For and While
For repeatable tasks, we use loops. Perhaps the most commonly used are ‘for’ and ‘while’ loops. Here’s how they work:
// 'for' loop for (int i = 0; i < 5; i++) { Console.WriteLine(i); } // 'while' loop int j = 0; while (j < 5) { Console.WriteLine(j); j++; }
Both these loops will print the numbers 0 through 4 on the console.
5. Functions: Methods in C#
Methods or functions are named blocks of code designed to perform a particular task when called. Here’s an example of a simple method.
public void Greet() { Console.WriteLine("Hello from Zenva!"); }
This method, when invoked, will print “Hello from Zenva!” on the console.
Expanding Your C# Skills
As you become more comfortable with the fundamentals of C#, you can start exploring more complex and exciting features of the language. In this section, we will go through examples involving classes, properties, inheritance, and exceptions.
Classes and Objects
At its core, C# is an object-oriented language. This means coding focuses around creating ‘objects’ from ‘classes’. Here’s an example of declaring a class and creating an object:
public class Player { public string Name { get; set; } public int Score { get; set; } } Player myPlayer = new Player(); myPlayer.Name = "Zenva"; myPlayer.Score = 100;
This code defines a ‘Player’ class with properties ‘Name’ and ‘Score’, and then creates an instance or object of the class.
Properties in C#
C# introduced a concept called ‘Properties’. A property is a member of a class that provides a flexible mechanism to read, write or compute the value of a private field. Properties can be computationally intensive and can even hold the logic of a program. Here is an example:
public class Rectangle { private double length; private double width; public double Length { get { return length; } set { length = value; } } public double Width { get { return width; } set { width = value; } } public double GetArea() { return length * width; } } ... Rectangle myRectangle = new Rectangle(); myRectangle.Length = 5.0; myRectangle.Width = 4.0; double area = myRectangle.GetArea();
This code snippet creates a class, ‘Rectangle’, with properties ‘Length’ and ‘Width’. It also has a method, ‘GetArea’, that calculates the area of the rectangle. A rectangle object is then created and its area is computed.
Inheritance in C#
Inheritance allows you to define a class based on another class, thereby promoting reusability. It involves a ‘base’ class and a ‘derived’ class where the derived class inherits the members of the base class. Take a look:
public class Animal // Base class { public void animalNoise() { Console.WriteLine("The animal makes a noise"); } } public class Pig : Animal // Derived class { public void pigSound() { Console.WriteLine("The pig says: wee wee"); } } ... Animal myAnimal = new Animal(); myAnimal.animalNoise(); Pig myPig = new Pig(); myPig.animalNoise(); myPig.pigSound();
Here, the ‘Pig’ class derives from the ‘Animal’ class. Therefore, it can make use of its method ‘animalNoise’ in addition to its own method ‘pigSound’.
Exceptions in C#
When dealing with errors, C# includes the concept of exception handling. Exceptions are runtime errors that can be caught and handled using ‘try’, ‘catch’, and ‘finally’ blocks. This way, instead of letting your application crash, you can dictate how it should respond to errors.
try { int[] numbers = {1, 2, 3}; Console.WriteLine(numbers[3]); } catch (Exception e) { Console.WriteLine("An exception occurred: " + e.Message); } finally { Console.WriteLine("The 'try catch' is finished."); }
The above code tries to access an index of the ‘numbers’ array that does not exist. This results in an array out-of-bounds exception. Rather than crashing, the program catches the error in the ‘catch’ block and prints an error message. The ‘finally’ block executes regardless of whether an exception was thrown or not.
These concepts may seem a bit tricky initially, but with our easy-to-understand and engaging tutorials at Zenva, you’ll quickly gain fluency. Remember, becoming an expert in a language takes time and consistent practice. Keep pushing ahead and soon you’ll gain not only a deep understanding but also an appreciation for this powerful language!
C# Advanced Concepts
As we delve deeper into C#, we can explore several advanced concepts that can significantly boost your programming skills. ‘Polymorphism’, ‘Interfaces’, ‘Generics’, and ‘Delegates’ are four of these advanced concepts that every C# developer should have a solid grasp on. Let’s break those down with examples:
Polymorphism in C#
Polymorphism allows objects to take on many forms. The most common use of polymorphism in OOP occurs when a parent class reference is used to refer to a child class object. Take a look:
public class Animal { public virtual void animalSound() { Console.WriteLine("The animal makes a sound"); } } public class Pig : Animal { public override void animalSound() { Console.WriteLine("The pig says: wee wee"); } } ... Animal myAnimal = new Animal(); myAnimal.animalSound(); Animal myPig = new Pig(); myPig.animalSound();
Here, we use ‘virtual’ and ‘override’ keywords to modify the method ‘animalSound’ in the base and derived classes, respectively. When you call ‘animalSound’ on the ‘myPig’ object, it executes the overridden method in ‘Pig’ class.
Interfaces in C#
Interfaces enable you to encapsulate a group of related functions that can be adopted by any class. Different classes can implement the same interface while providing different behaviors. Consider the following example:
interface IAnimal { void animalSound(); } class Cat : IAnimal { public void animalSound() { Console.WriteLine("The cat says: meow meow"); } } ... Cat myCat = new Cat(); myCat.animalSound();
This piece of code creates an interface ‘IAnimal’ with a function ‘animalSound’. The ‘Cat’ class implements this interface and provides its own implementation of the method.
Generic Classes
Generics allow you to define classes and methods with placeholders (type parameters) that get filled in when they’re used. This concept can increase code reuse, type safety, and performance. Let’s see an example:
public class MyGenericClass { private T genericMemberVariable; public MyGenericClass(T value) { genericMemberVariable = value; } public T genericMethod(T genericParameter) { Console.WriteLine("Parameter type: {0}, value: {1}", typeof(T).ToString(), genericParameter); return genericParameter; } public T genericProperty { get; set; } } ... MyGenericClass intGenericClass = new MyGenericClass(10); int val1 = intGenericClass.genericMethod(200); MyGenericClass stringGenericClass = new MyGenericClass("Hello World"); string val2 = stringGenericClass.genericMethod("Hello Zenva");
This code creates a generic class ‘MyGenericClass’ and creates two instances of it, one with int and the other with string type parameters. This makes it reusable for different data types.
Delegates in C#
Delegates are references to methods. They’re useful when you want to pass methods as parameters. They act as function pointers in C#.
delegate void MyDelegate(string msg); public class MyClass { public static void ClassMethod(string message) { Console.WriteLine("Call from MyClass.ClassMethod(): " + message); } } ... MyDelegate del = MyClass.ClassMethod; del("Delegate function call");
In this example, ‘MyDelegate’ is a delegate type, and ‘del’ is an instance of that delegate. ‘myClassInstance’ gets assigned a method (ClassMethod) that matches the ‘MyDelegate’ signaure. When ‘myDelegateInstance(“Calling Delegate”);’ is invoked, the ‘ClassMethod’ is executed.
These topics might seem overwhelming, but worry not. Here at Zenva, we believe in making complex topics readily approachable. Our goal is to ensure you have a firm grasp of the fundamentals before moving on to these advanced topics. Remember, consistency and practice are key to mastery. Happy coding!
Keep the Momentum Going with Zenva
As you’re well on your way to mastering C#, it’s the perfect time to delve deeper and harness the language’s full potential. We recommend taking your newfound skills a step further by exploring our comprehensive Unity Game Development Mini-Degree.
This mini-degree constitutes an all-encompassing myriad of courses aimed at teaching game development using the Unity game engine. You’ll gain valuable hands-on experience and nurture your creativity by crafting stunning games in 2D, 3D, AR, and VR. The in-depth courses cover a plethora of aspects relevant to game development, from game mechanics and custom game assets to enemy AI and animations. So no matter your experience level, this mini-degree offers an invaluable opportunity to elevate your GameDev skills.
If you’re thirsting for more, we’ve got you covered! Check out our extensive collection of Unity Courses to find the content best-suited to your learning needs. From a beginner eager to learn how to code, to a professional developer looking to broaden their skill set, Zenva has something for everyone. We provide a flexible learning experience, allowing you to learn at your own pace, anytime and anywhere. Your big break into the booming gaming industry is just a course away!
Conclusion
Discovering the world of coding and game development is an exciting journey, and learning C# is a significant milestone on that path. Here at Zenva, we’re dedicated to unlocking this journey for you, providing comprehensive, high-quality courses that empower you to explore and learn at your own pace.
Don’t stop here; seize the opportunity to delve further into the expanses of C#. Explore more about C#’s application in the thriving field of game development with our Unity Game Development Mini-Degree. Happy coding, learners, and may your journey with us be as fascinating as the destination!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
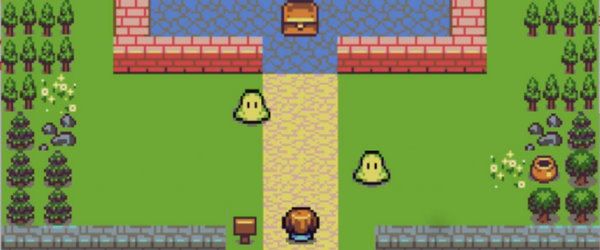
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.