In the exciting world of programming, mathematics plays a vital role, underpinning a wide array of operations and functionalities. C# programming is no exception. Whether for general programming or specialized fields like game development, C# developers often find themselves reaching for math functions to solve problems and build functionality. This tutorial unlocks the understanding of C# math functions through engaging and relatable examples.
Table of contents
What is C# Math?
C# Math is a built-in class in the C# programming language, within the System namespace. It provides rich mathematical functions that developers can use to carry out various mathematical operations.
What is it for?
C# Math class is a powerful tool for performing operations such as rounding numbers, generating random numbers, trigonometric calculations, and much more. These functions can be incredibly valuable in areas such as game mechanics, animation, physics simulations, and other areas where maths is integral.
Why learn C# Math?
Learning to effectively use C# Math will not only boost your competency as a C# programmer, but it can also open doors into specialized fields like game development. The use of math functions in games often contributes to creating compelling gameplay, realistic physics, and adaptive AI. Whether you’re a beginner or an experienced programmer, having a solid understanding of how to use the C# Math class will undoubtedly be a giant leap in your learning journey. So let’s dive in.
Basic Math Operations
The C# Math class comes with a suite of built-in functions to handle basic operations like addition, subtraction, multiplication, and division. However, it excels in delivering more complex functionaries as well.
// Addition int a = 5, b = 10; int sum = a + b; Console.WriteLine($"Sum: {sum}"); // Subtraction int c = 20, d = 5; int diff = c - d; Console.WriteLine($"Difference: {diff}"); // Multiplication int e = 4, f = 3; int product = e * f; Console.WriteLine($"Product: {product}"); // Division int g = 100, h = 20; int quotient = g / h; Console.WriteLine($"Quotient: {quotient}");
Advanced Math Operations
Besides basic operations, the C# Math class also provides functions for more advanced operations such as square root, power, rounding numbers, generating absolute value, and more.
// Square root double i = 9; double sqrt = Math.Sqrt(i); Console.WriteLine($"Square Root: {sqrt}"); // Power double j = 2, k = 3; double pow = Math.Pow(j, k); Console.WriteLine($"Power: {pow}"); // Rounding numbers double l = 5.7; double round = Math.Round(l); Console.WriteLine($"Rounded Number: {round}"); // Absolute value double m = -7.8; double abs = Math.Abs(m); Console.WriteLine($"Absolute Value: {abs}");
Trigonometric Functions
Trigonometry is fundamental in areas like game development, particularly in mechanics and animation. The Math class offers built-in functions like Sin, Cos, Tan, and their inverses for these purposes.
// Sin double n = 30; double sin = Math.Sin(n); Console.WriteLine($"Sin of {n} = {sin}"); // Cos double o = 45; double cos = Math.Cos(o); Console.WriteLine($"Cos of {o} = {cos}"); // Tan double p = 60; double tan = Math.Tan(p); Console.WriteLine($"Tan of {p} = {tan}");
Generating Random Numbers
Creating random numbers is incredibly useful for gaming or any other situation that requires variability. The Random class in C# allows you to generate random numbers without much hassle.
// Create a new instance of the Random class Random rand = new Random(); // Generate a random number between 1 and 10 int randNum = rand.Next(1, 11); Console.WriteLine($"Random Number: {randNum}");
Mathematical Constants
The Math class also provides common mathematical constants such as Pi and E (Euler’s number). These constants are crucial, especially in fields involving geometry and calculus.
Let’s see how to access these constants:
double pi = Math.PI; Console.WriteLine($"Pi: {pi}"); double e = Math.E; Console.WriteLine($"Euler's Number: {e}");
Since we’ve now access to Pi, we can utilize it to calculate the circumference and area of a circle:
double radius = 5; // Calculate circumference double circumference = 2 * Math.PI * radius; Console.WriteLine($"Circumference: {circumference}"); // Calculate area double area = Math.PI * Math.Pow(radius, 2); Console.WriteLine($"Area: {area}");
Performing Comparisons
Now, let’s look at Math.Max and Math.Min, which allow us to easily perform comparisons and find the maximum or minimum of two numbers:
int x = 5, y = 10; int maxNum = Math.Max(x, y); Console.WriteLine($"Max: {maxNum}"); int minNum = Math.Min(x, y); Console.WriteLine($"Min: {minNum}");
Ceiling and Floor Functions
The Math class contains functions for rounding up and rounding down numbers – Math.Ceiling and Math.Floor:
double q = 5.7; double ceil = Math.Ceiling(q); Console.WriteLine($"Ceiling: {ceil}"); double floor = Math.Floor(q); Console.WriteLine($"Floor: {floor}");
Logarithm Functions
Finally, the C# Math class provides logarithm functions like Math.Log, which calculates the natural (base e) logarithm of a number, and Math.Log10 for the base-10 logarithm:
double r = 100; // Natural logarithm double log = Math.Log(r); Console.WriteLine($"Natural Logarithm: {log}"); // Base-10 logarithm double log10 = Math.Log10(r); Console.WriteLine($"Base-10 Logarithm: {log10}");
Finding the exponent with Math.Exp
The Math.Exp function is used to find the exponential of a specified number:
double s = 3; double exp = Math.Exp(s); Console.WriteLine($"Exponential: {exp}");
Calculating the remainder with Math.IEEERemainder
The Math.IEEERemainder function is used to compute the remainder resulting from the division of a specified number by another specified number. It’s an alternative way of performing the modulus operation (%):
double t = 10, u = 3; double remainder = Math.IEEERemainder(t, u); Console.WriteLine($"Remainder: {remainder}");
Math.Truncate vs Math.Round
It’s also important to understand the difference between the Math.Truncate method and the Math.Round method. Math.Truncate is used to calculate the integral part of a decimal or double number, removing any fractional digits. On the other hand, Math.Round rounds a decimal or double number to the nearest integral value, and to the even number if it’s midway between two other numbers.
double v = 5.7, w = 5.2; double truncateResult = Math.Truncate(v); Console.WriteLine($"Truncate: {truncateResult}"); double roundResult = Math.Round(w); Console.WriteLine($"Round: {roundResult}");
Solving quadratic equations
The Math class in C# can also be used to solve equations. As a final demonstration, let’s solve a quadratic equation using the quadratic formula (x = [-b ± sqrt(b² – 4ac)] / 2a):
// coefficients double a = 1, b = -3, c = 2; if ((b * b - 4 * a * c) < 0) { Console.WriteLine("No real solutions exist"); } else { double x1 = (-b + Math.Sqrt(b * b - 4 * a * c)) / (2 * a); double x2 = (-b - Math.Sqrt(b * b - 4 * a * c)) / (2 * a); Console.WriteLine($"Solutions: {x1}, {x2}"); }
By harnessing the power of the C# Math class, we can see that equations involving square roots, negative values and real solutions can all be tackled, helping us solve real programming challenges.
Where to go next
Now that you’ve gained a solid understanding of C# Math and seen its power and versatility in action, it’s time to go further on your learning journey. Mathematics is a cornerstone of a multitude of programming fields, and mastering it can open a vast range of opportunities.
We at Zenva recommend leveraging your newfound knowledge into more complex applications with game development. Unity, often used in conjunction with C#, is a powerful game engine that can help you create stunning 2D and 3D games. Our Unity Game Development Mini-Degree covers a wide range of aspects in game development. Mastering Unity with your current C# skills could lift to a whole new level, opening doors to high-paying job opportunities.
You may also explore our broader collection of Unity courses on our Unity page. At Zenva, we’re always ready to support you on your journey from a beginner to professional. Bite-sized lessons, interactive quizzes, and real-world projects – all in a flexible schedule – are some of our hallmarks to ensure an engaging learning experience. Buckle up and keep learning!
Conclusion
Mastering C# Math fuels you with the power to solve an extensive range of programming problems. Whether it is game development mechanics, software calculations or data analysis, knowing how to tackle mathematical operations can smoothly open doors to amazing opportunities!
We at Zenva provide courses that are designed to turn you into an expert programmer, regardless of your current skill level. By understanding the significance of these fundamental mathematical functions, you are ready to take your next giant leap into more complex fields such as game development. Explore our Unity Game Development Mini-Degree and continue your exciting learning journey within an engaging and structured learning path. Keep up the good work and always keep learning!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
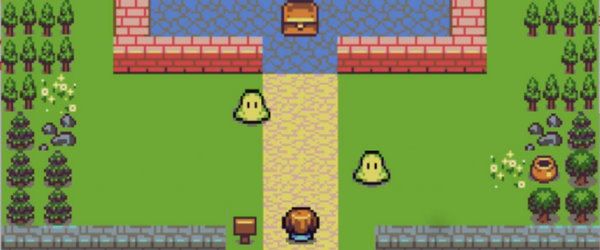
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.